JUnit, can I create an EntityManager or even a session without running the server?
33 Replies
⌛
This post has been reserved for your question.
Hey @Rag...JN 🌌 🦡 👽 💰! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
Location
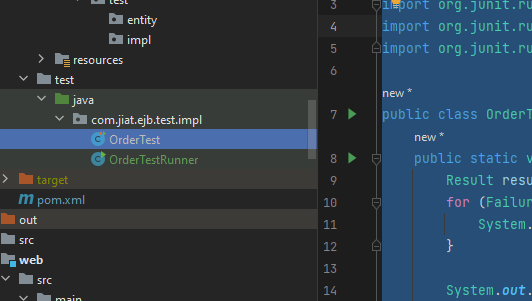
Problem
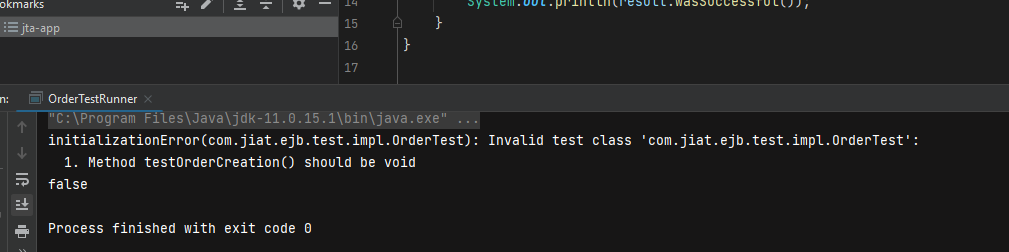
alright
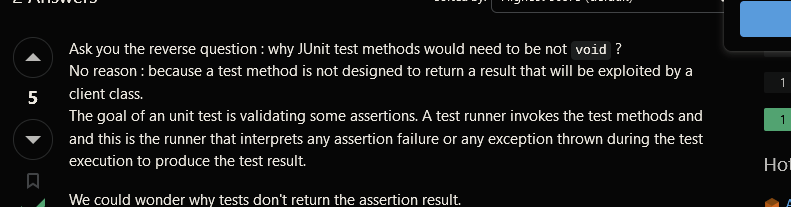
Yes
ok so but now my test is failure
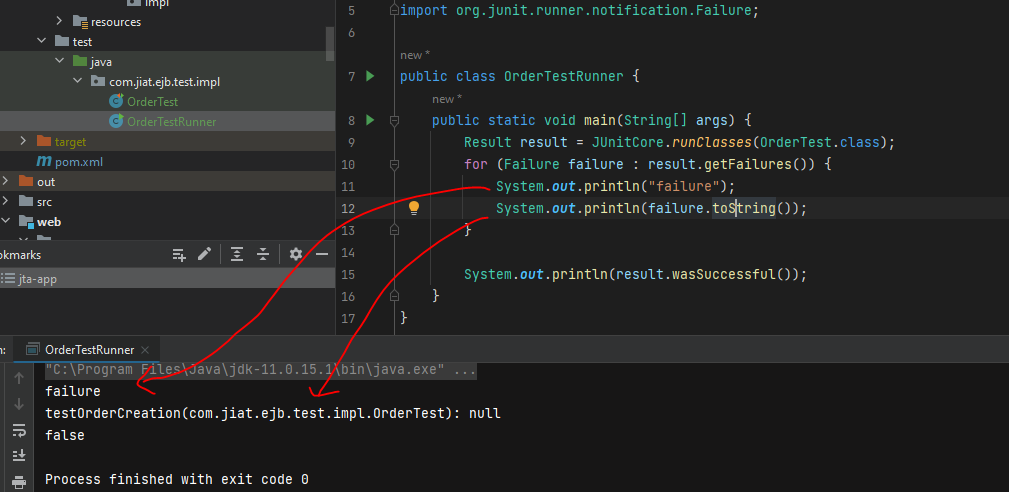
how can I know why this is it?
Because you return boolean for some reason.
I just changed it to void
Oh, I see.
same class with void
You're meddling with pure JUnit. That's unusual. Let me look this up.
failure.getException().printStackTrace();
ok
null pointer exception is the cause
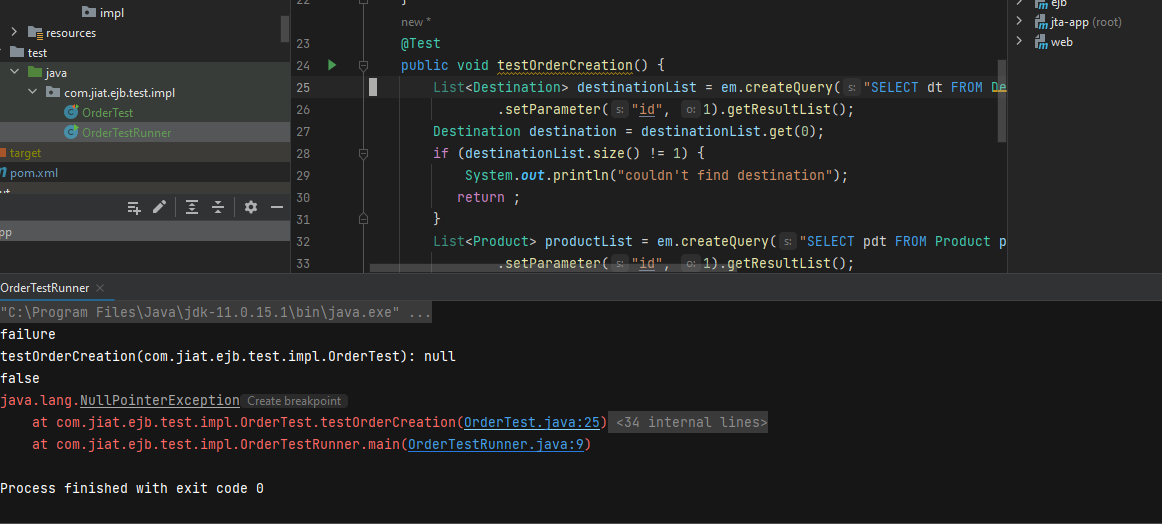
but there is clearly a Destination with id 1
Probably there's nothing there to interpret the PersistenceContext annotation.
here
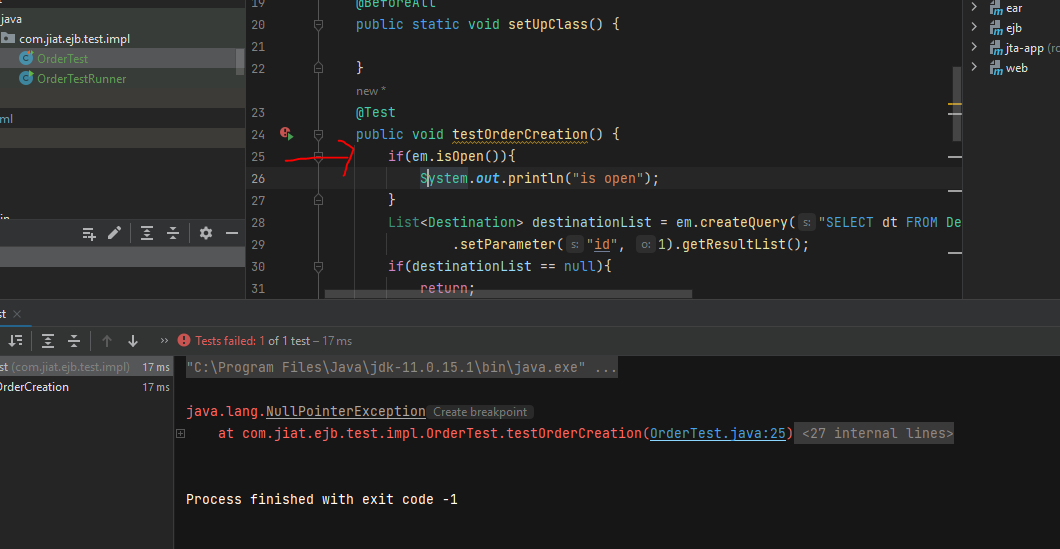
so it is not even there in the first place
EntityManager is null
Arquillian needed?
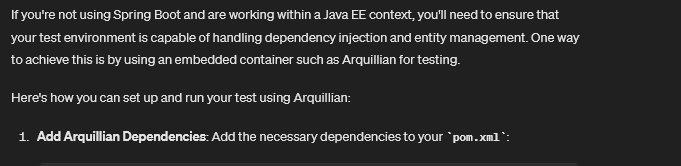
No idea, I wouldn't use a single thing that you're using except Java.
why?
The requirement is to use JUnit for the assignment
see
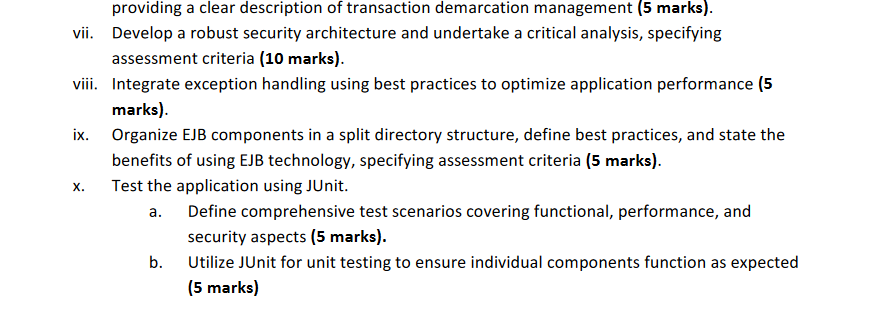
Java EE is getting less and less popular and nowdays it's usually a red flag indicating a shitty project.
yah we had a convo today in general
I'd use JUnit, but you're apparently using JUnit 4, which I wouldn't pick, because JUnit 5 is more enjoyable to work with.
idk really, the tech is too old
yah but 5 has like a lot more configurations so I chose 4 because just one or two dependencies
If you asked that a month ago I could've look up, because I had access to a Java EE project with JUnit tests.
also I just started to work with JUnit 3 horus hours ago.
read an article started to implement into the project
where else can I apply JUnit beside the EJB because this thing requires a lot of configuration
at last to get some marks
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.