Local vs Prod Dev (with a twist)
I'm trying to setup separate dev scripts but a little differently, because i don't want the dev script to be limited to the webflow.io domain, rather just whenever the localhost is available.
So if the localhost is running, then it loads the relevant script from localhost.
If the localhost is not running, then it loads the public script instead
Now, i've got this kind of working using fetch with some try catch to ensure that it doesn't just give up when the localhost is unavailable. My issue with fetch though is that it prints out a nice big CONNECTION_REFUSED error even if i try to catch and print the error in a
log
instead of an error
For example:
Still displaying the error but in a console log just for demo purposes as opposed to console.error14 Replies
This is what i ended up on, it didn't solve the main issue, but it at least works instead of erroring out
does this work?
revent any logs in console but is showing
null
which you probably don't want
or this?
Both still print that connection refused error, for some reason it always seems to ignore the catch
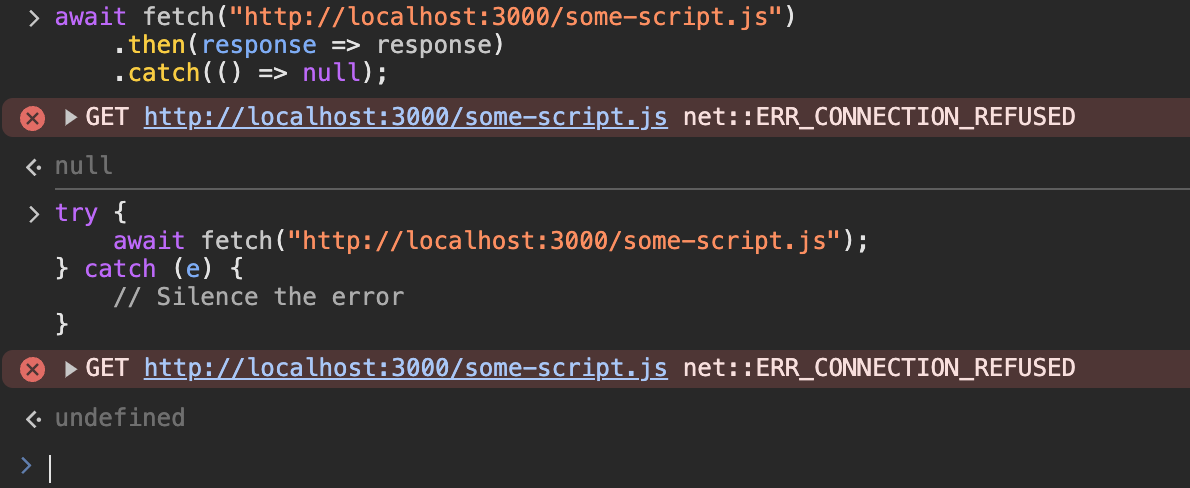
I was reading a recommendation someone made on stack overflow where they said you could overwrite the window.console function globally to ignore certain messages LOL
lol
how about this? 1:26
https://youtu.be/aCeD7GymetY?si=E66e6BuEPUwOet7g&t=86
Web Bae
YouTube
Separate Staging and Production Code in Webflow
Here's a little javascript snippet to serve different code depending on whether it's the staging domain or the production domain - super handy if you need to iterate on your code without worrying about publishing your in-development code to production and to maintain continuity on the live domain.
// FREE STUFF
💪 My ULTIMATE Webflow Resource L...
i guess that doesn't really work either
not exactly what you are looking for
I've also seen this done by using a boolean flag IS_DEV in localStorage
This is actually what sparked me to start 😂
but then you have to manage that local storage variable
ooooh i never considered using local storage
wonder if i could throw up local storage if the ip is mine
could use a url param too
both solns are still more manual tho
oh the url param is good too!
I really don't mind a more manual thing, i just hate constantly adjusting the webflow scripts 😂
agreed 🤝
Just a little update, but i ended up using localStorage combined with an applescript automation for triggering the localstorage variable on and off
Applescript automation (i'm triggering this with a raycast script and/or a streamdeck action)
Next steps would be seeing if i could automate the page script variable by making sure the filenames are the same as the slug of the current page, and then i wouldn't need to edit the script on the page, it would just be dynamic