HELP WITH getClass() method
Consider this class:
what is the difference between using getClass() and using MyClass.class,
I heard somewhere that getClass() can cause unexpected behaviour when a class extends MyClass but why would that happen ?
49 Replies
⌛
This post has been reserved for your question.
Hey @userexit! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
You may call getClass() on any object, including one of which you don't know what's it class
Example:
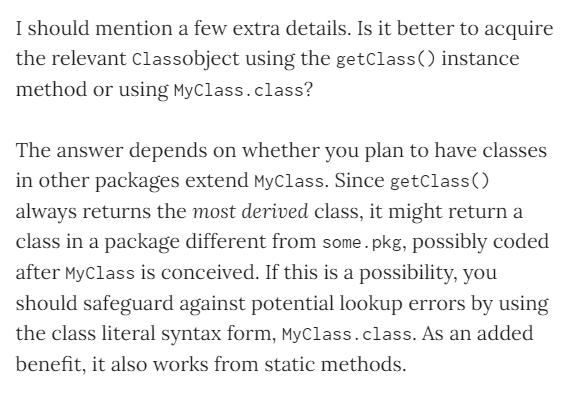
then what is this guy here saying
?
@Kyo-chan
He's saying "I'm a beginner"
If the class you want is MyClass, use MyClass.class
If the class you want is that of an object you got, use getClass() on that object
I think he means if you have for example:
and somewhere in your code you have:
Shape shape = new Rectangle();
and you do shape.getClass(); you get the Rectangle class and if its in another package then you cant find resources anymore in your package
lamo
do you think this is what he means
?
He doesn't mean much other than how if you use getClass() on an object whose variable type isn't a final class, then the possible results are more than just the one.
...... And yeah, that's the point. It's not an issue.
ah okay
and btw
getClass().getResource() will try to find it in the package of the class
and getClass().getClassLoader().getResource() will try to find it outside right
what I dont get is how can you have package based resources
there is only 1 resource folder in java
for example running this:
doesnt throw a null error
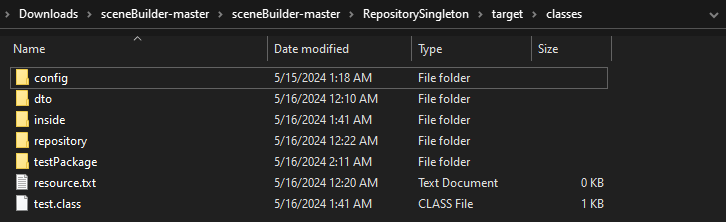
because it looked for it here
Actually, the resources folder is a Maven thing to better organize project. Natively, Java has no such notion
outside all packages
if I delete getClassLoader() I get an error
if I delete getClassLoader() and I put the resource inside the testPackage folder I get no error no more
yeah so do you know how I can put resources in a specific package only ?
Isn't that exactly what you just demonstrated you know how to do?
yes but only after compilation
because then I get the folder of the package in the target/classes folder
and I can do wahtever I want with it
but before compiling
How can I decide a resource will go inside a package folder
Just put the resources in folders named exactly like that inside the resources folder
(Though nobody but the Java base library team does that)
so inside src/main/resources
I put a folder named testPackage
and inside it it will put all the resources inside the testPackage inside target/classes ?
yup
why do you say that ?
I often mention various things that are true in this world. Don't mind it
no but I mean is there any logicla reason for which normal people wouldnt do ut
There doesn't exactly seem to be a point to bother with that. There is a little bit more of a point with Java 9+ modules isolation, but even then you can just name folders rather than actual packages.
isnt getting resources an enough point ?
You can get resources without putting them in packages, and with putting them in packages named differently to the ones you put your classes in.
Otherwise Maven would have simplified putting resources in the same packages as classes
really how can you get such resources
Again, did you not demonstrate you know how?
I mean u can have a folder inside resource named: jeff
and then do getClass().getClassLoader().getResource("/jeff");
but do u have naother way ?
@Kyo-chan
I don't remember all the details, but if you include the starting / I don't think you need the classLoader
why, what does the starting / change lol
It changes where it looks for the resource
yeah so if you do /jeff
where will it look
In a package named jeff
even wi thout classloader
ill check
damn
ur right
why does a / change so much ?
does it mean look from behind ?
@Kyo-chan
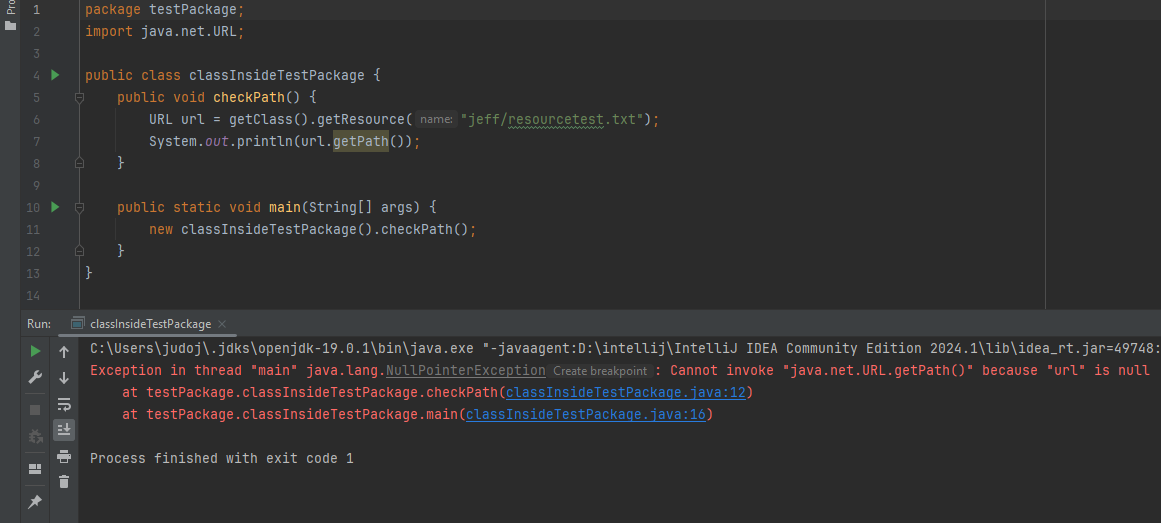
I guess when u do jeff/resourcetest.txt
it tries to find folder jeff in the current folder which is the testPackage folder
if u do /jeff/resourcetest.txt it tries to find it on the parent
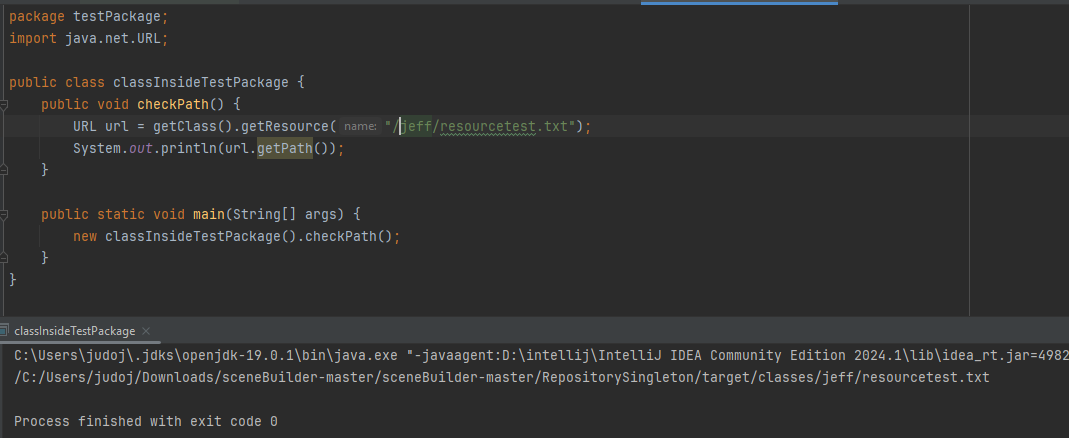
so what if i have a package inside a package
wait why does it work
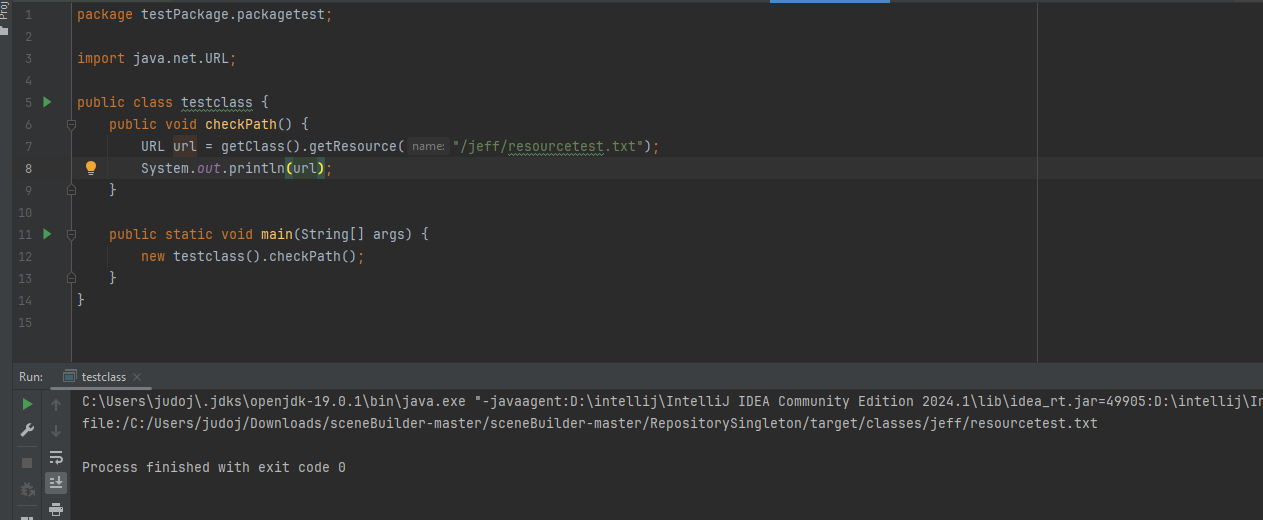
?
does adding a / simply get me all out, inside the target/classes folder ?
yes it does
what if I have ar esource in parent package that I want to access
can I access it without using getClassLoader ?
also justl earned using getSystemResource deos the same
nice damn
It means look from the root
what do u use to look from behind
so i can look in resources of parent package
I don't do that. I'm not convinced there is something for it. I'd try .. just in case
yeah
URL url = getClass().getResource("..New Text Document.txt");
this here doesnt work
wait
adding a slash works
URL url = getClass().getResource("../New Text Document.txt");
@Kyo-chan
My bad, I assumed that as obvious
can I chain double dots ?
I don't know, but it would be very weird if you couldn't
so
....
this here puts me 2 packages abvoe ?
../../name.text
Not very used to navigate filesystem trees, are we?
yeah not used to none of that
yeah it works
i can double dot chain
i guess triple, quadruple etc all work the same
I was in a state of confusion but thanks to this conversation im in a state of clarity now, thank you for the help @Kyo-chan
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.