Issue with Modal -- "Something went wrong. Try again."
I've been trying to get the response from a modal, but nothing seems to come down the websocket at all and it just states that something went wrong.
The modal shows up just fine, but on submit it's failing. Any help would be appreciated!
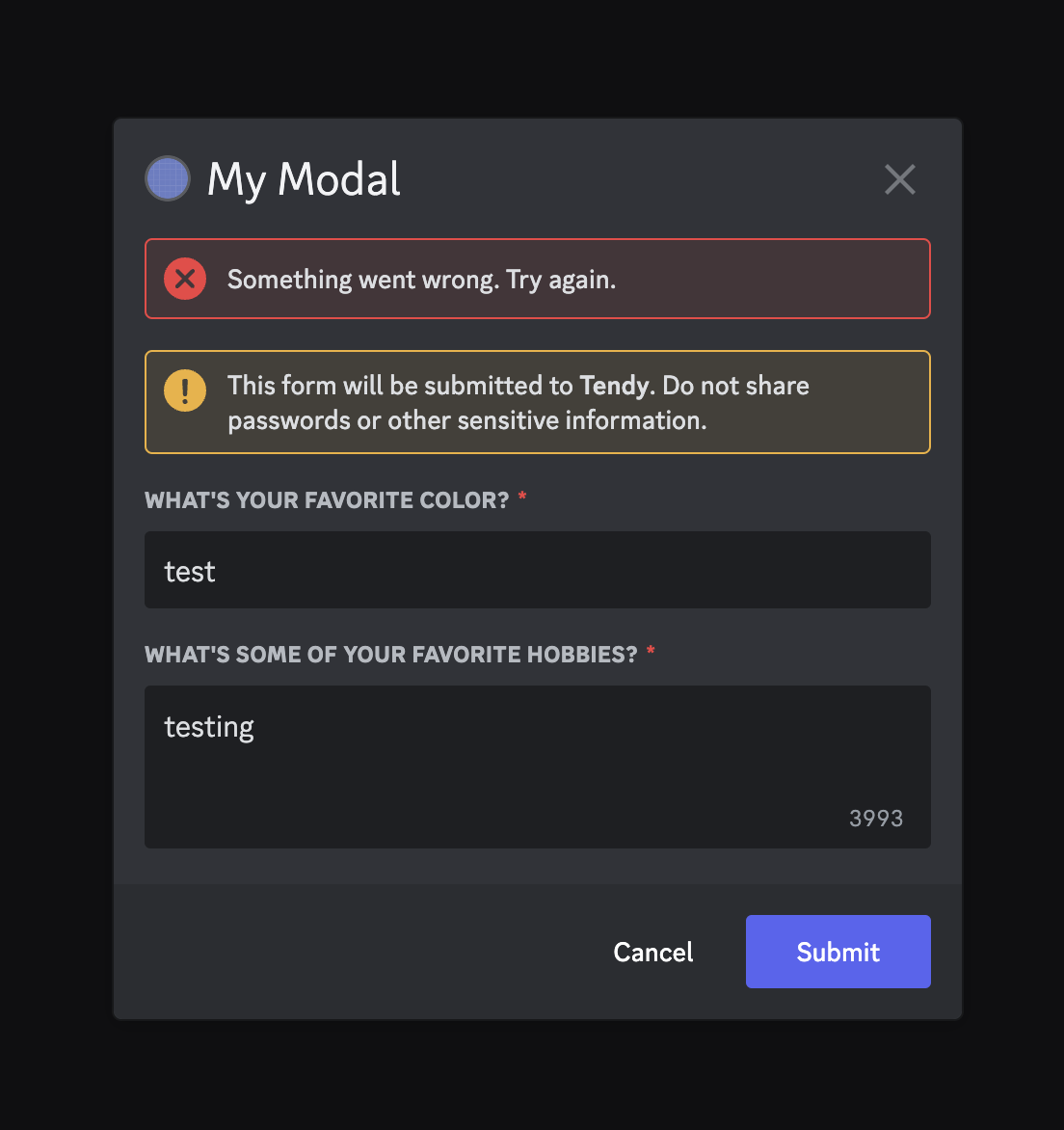
9 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!You need to acknowledge the modal interaction like you do any other interaction
Right now, you're just console logging it
PS: you should use a unique name for that variable: right now,
interaction
is used by both 1) the interaction that interactionCreate
emits with and 2) the modal submit interactionoh i see -- how do i acknowlege the modal interaction? I'll go snooping in the docs again
i think using
interaction
in both places should be ok. It's scoped inside that Events.InteractionCreate
listener -- right?interaction
at the top is normal, but I would call the modal one something like newInteraction
to be different, but that's up to you
ModalSubmitInteraction instances have options like .reply()
to acknowledge themwhere would the reply() go? inside of the
Events.InteractionCreate
handler? becuase I'm not even seeing that code runRight now, the modal interaction only exists in the callback of
so just call
interaction.reply(...)
inside thereit never makes it in there
my terminal says "A" then the modal shows, i submit the modal and it shows the error message i mentioned in the topic, and "B" is not in my terminal
just seems like nothing comes down the pipe at all when i hit submit in the modal
could it be a permission issue or does that not make sense?
do you have the
Channel
partial? discord.js needs it to properly receive messages in DMs, so it might also be needed for interactions in DMs to function properlyi didn't, but i added them just now and same result
if anyone else has ideas, it would be much appreciated