Need help with basic LWJGL 2.9.0 demo loading on CheerpJ
Hello, I am trying to get a very simple LWJGL 2.9.0 Demo working via CheerpJ, but am running into an error
Error: window.lwjglCanvasElement is not set or is not a canvas
. I've attached a picture of my project structure as well as the the error itself and some other relavent things. My desktop application is a NetBeans project using JDK 8. Here's the source code of the triangle that I found online: 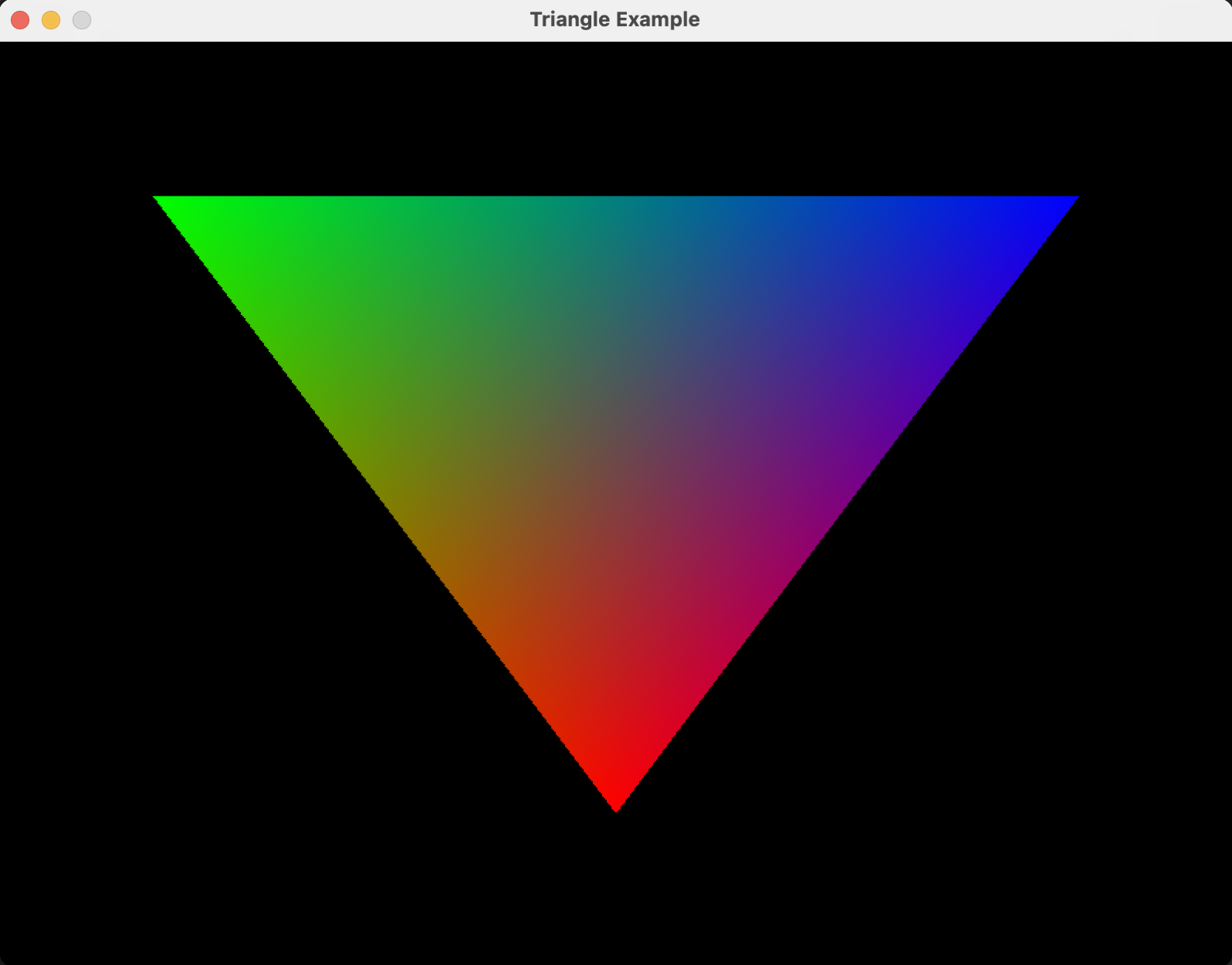
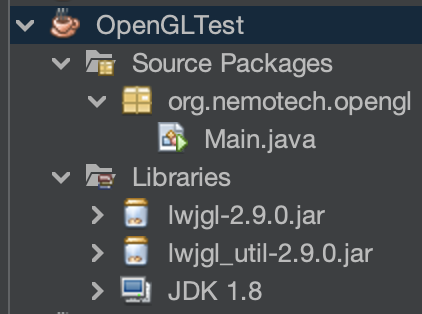
Solution:Jump to solution
1. add this HTML to the start of the document body
2. to the script, add the following line before the
cheerpjRunMain
call:...57 Replies
Here are the VM options I am using (just links native LWJGL binaries): Here is my
index.html
page:
I am using for the local HTTP server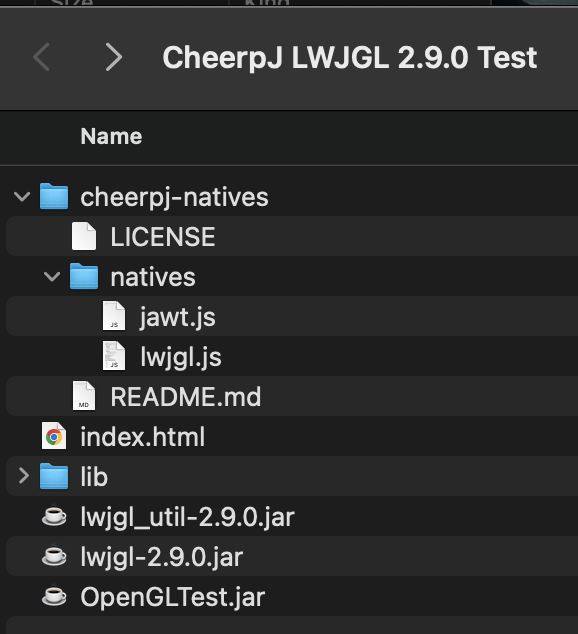
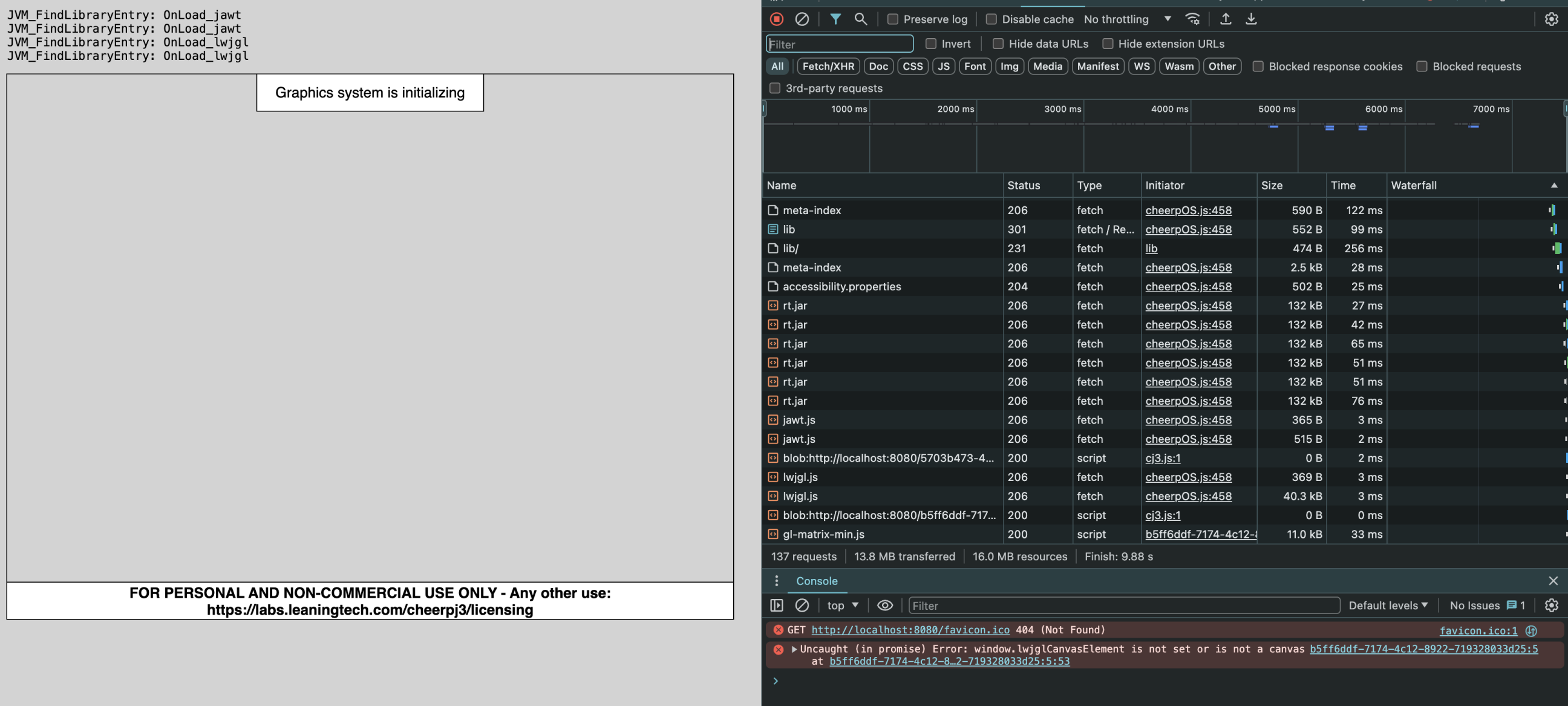
You need to simply initialize the global variable
lwjglCanvasElement
to the HTML5 canvas object that you want to use as your rendering area
Please keep in mind that the lwjgl implementation we provide is partial, consider using the browsercraft
demo as a guide on how it should be used.GitHub
browsercraft/minecraft-web.js at main · leaningtech/browsercraft
Unmodified Minecraft 1.2.5 in the browser using CheerpJ - leaningtech/browsercraft
Thanks for the quick response. How should I do this? I know little to nothing regarding HTML/JS
There are a few steps, you need to create a
<canvas>
element, resolve it using getElementById
, and assign it with the global variable in JavaScript window.lwjglCanvasElement = getElementById(...)
.
I don't have the opportunity of giving you direct code right now, @Alex please provide some guidance when you can
But these are all very basic HTML/JS operations, so it could be a good chance of picking up the basics. You'll find countless tutorials on these.hello 👋
as Alessandro suggested, the LWJGL implementation expects you to tell it what canvas to use by setting window.lwjglCanvasElement to an instance of HTMLCanvasElement before you run cheerpjRunMain
Solution
1. add this HTML to the start of the document body
2. to the script, add the following line before the
cheerpjRunMain
call:
(one of the reasons this is required because any canvases managed by CheerpJ inside the display div use a 2D graphics context. LWJGL’s canvas needs to be 3D - and you can’t use multiple contexts on the same canvas element: https://developer.mozilla.org/en-US/docs/Web/API/HTMLCanvasElement/getContext)
hopefully this helps - @ me if you run into any trouble :)
Hey there, thanks for the response. I've edited the page with those 2 steps, but I'm getting a different error now: @Alex
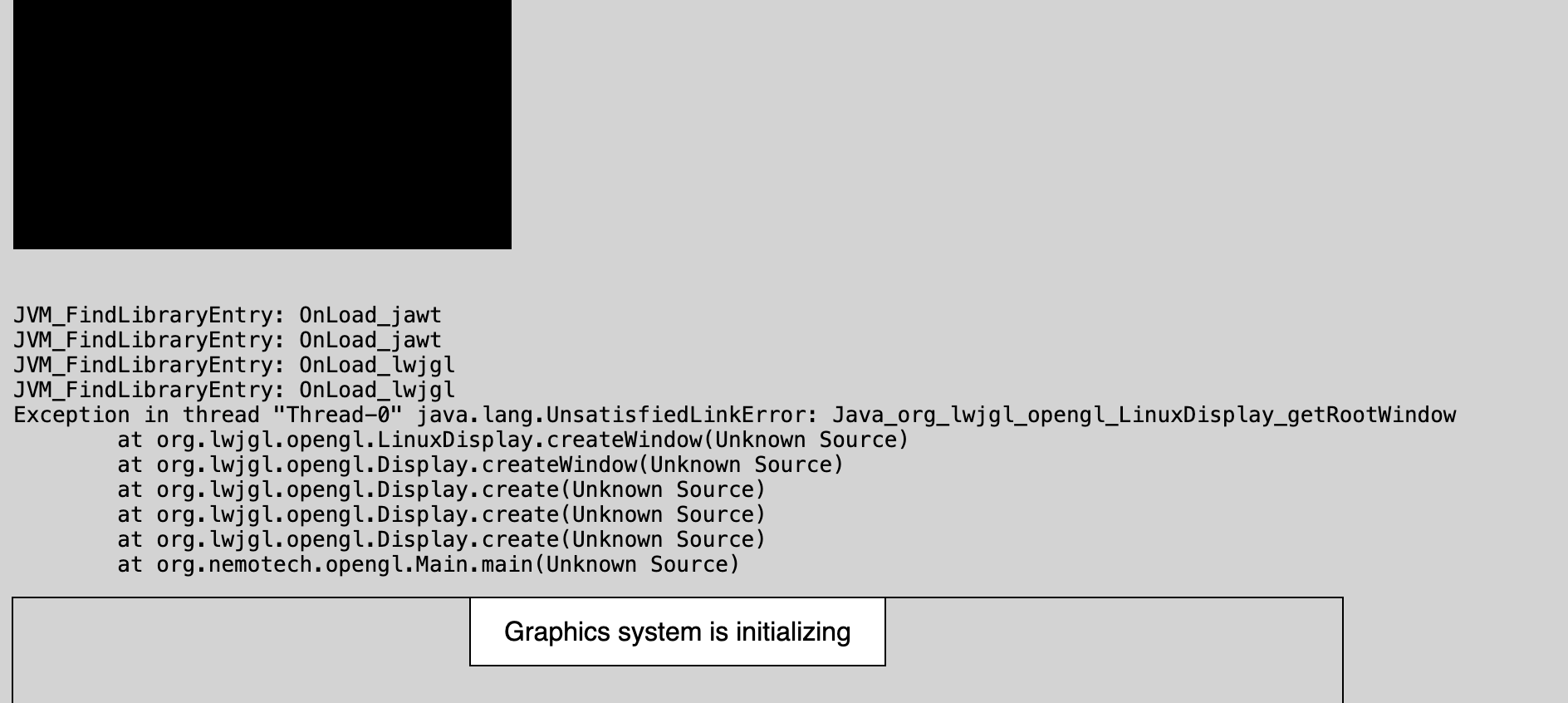
hm, so it looks like this isn’t going to be as easy as we thought
right now, the LWJGL implementation in cheerpj-natives is built so it supports only the functions that Minecraft uses
you could try to implement that function (
Java_org_…_getRootWindow
) inside lwjgl.jsOkay I gotcha, I assume just making it a stub function should suffice?
hopefully…. try returning null
Okay :) trying that
alternatively, since you have access to the Java source you could modify it in such a way that it doesn’t call that method at all (i.e. remove the call to Display.create)
my guess would be that you probably don’t need any display things at all actually
True, I had assumed that was necessary for CheerpJ, but maybe all
Display
calls can be omittedWas writing my message as you were writing this, yeah I'll try omitting them for now
ah, you're also going to need to give the canvas a width and a height:
This happens with all Display code commented out unfortunately, also it seems detached from the CheerpJ frame
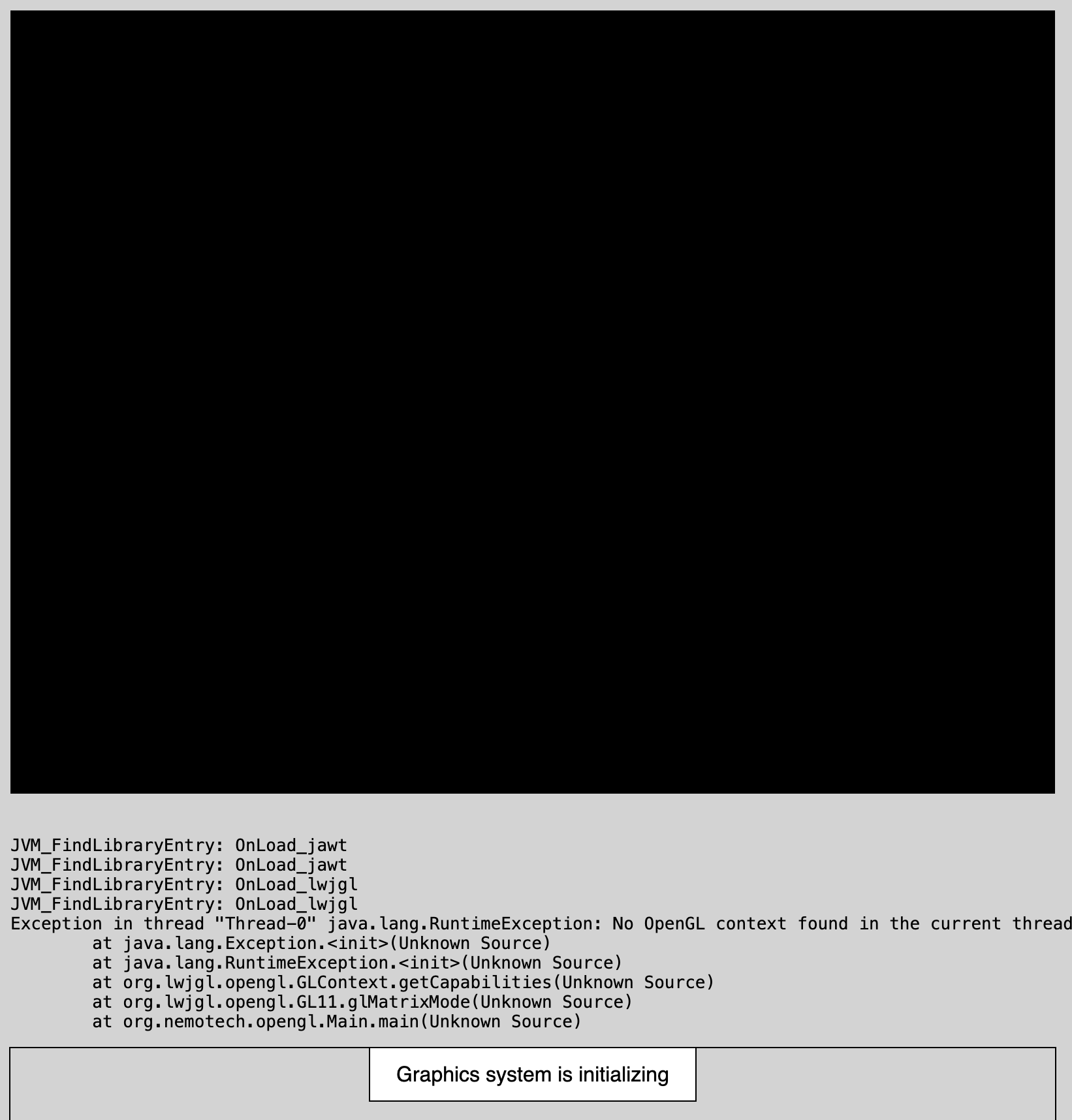
And adding a stub to
lwjgl.js
yields the same previous error message about the LinuxDisplay (with the Display code)hmm
here's where this is thrown in LWJGL itself: https://github.com/search?q=org%3ALWJGL+%22No+OpenGL+context+found+in+the+current+thread%22&type=code
also it seems detached from the CheerpJ framethis is expected and fine; just hide the CheerpJ display. here's browsercraft doing exactly that: https://github.com/leaningtech/browsercraft/blob/main/minecraft-web.js#L146-L149
Okay gotcha
Hmmm
my thoughts exactly! 😛
give me a minute to look into this more
Okay take your time. I really appreciate the help
OK, it looks like I was wrong here, you do need something Display-y
so... I've gone and added LWJGL support to JavaFiddle so we can better test this
LWJGL test - JavaFiddle
// Example using LWJGL 2.9.0
// Warning: experimental!
import org.lwjgl.LWJGLException;
import org.lwjgl.opengl.Display;
import org.lwjgl.opengl.DisplayMode;
import org.lwjgl.opengl.GL11;
public class Main {
public static void main(String[] args) {
try {
Display.setDisplayMode(new DisplayMode(800, 600));
Dis...
it exhibits the same error you are seeing
there must be some other way of initialising GL that Minecraft 1.2.5 uses, since this error doesn't happen in browsercraft
Very nice :)
I'm not familiar enough with LWJGL myself in order to feel confident advising on this. Maybe if we could see how Minecraft does it
or alternatively try to implement that stub correctly
Yes I think it's definitely the way it initalizes it as well, as I tried using browsercraft source, but I changed downloading the client from Mojang to this JAR instead, which yields the same error
oh, really?
hm
Yeah
that throws my theory out of the window (pun intended)
Is that build of minecraft opensource?
Well, i suppose it wouldn't be but idk if it's obfuscated
java tends to be easy to reverse-engineer...
I mean I could try analyzing the source code of their client
it might not be helpful anyway, if this is the case
I'll try to implement getRootWindow properly
Sounds good
Yeah, I was just unsure if they used some sophisticated obfuscation tool such as Zelix Klassmaster, etc
I don't think they did, no
@ayunami2000 knows a bit about reverse-engineering Minecraft, I think, if we want to go down that road
OK just looks like very minor obfuscation, such as variable and class renaming. Control flow seems untouched as there's definitely not string encryption either.
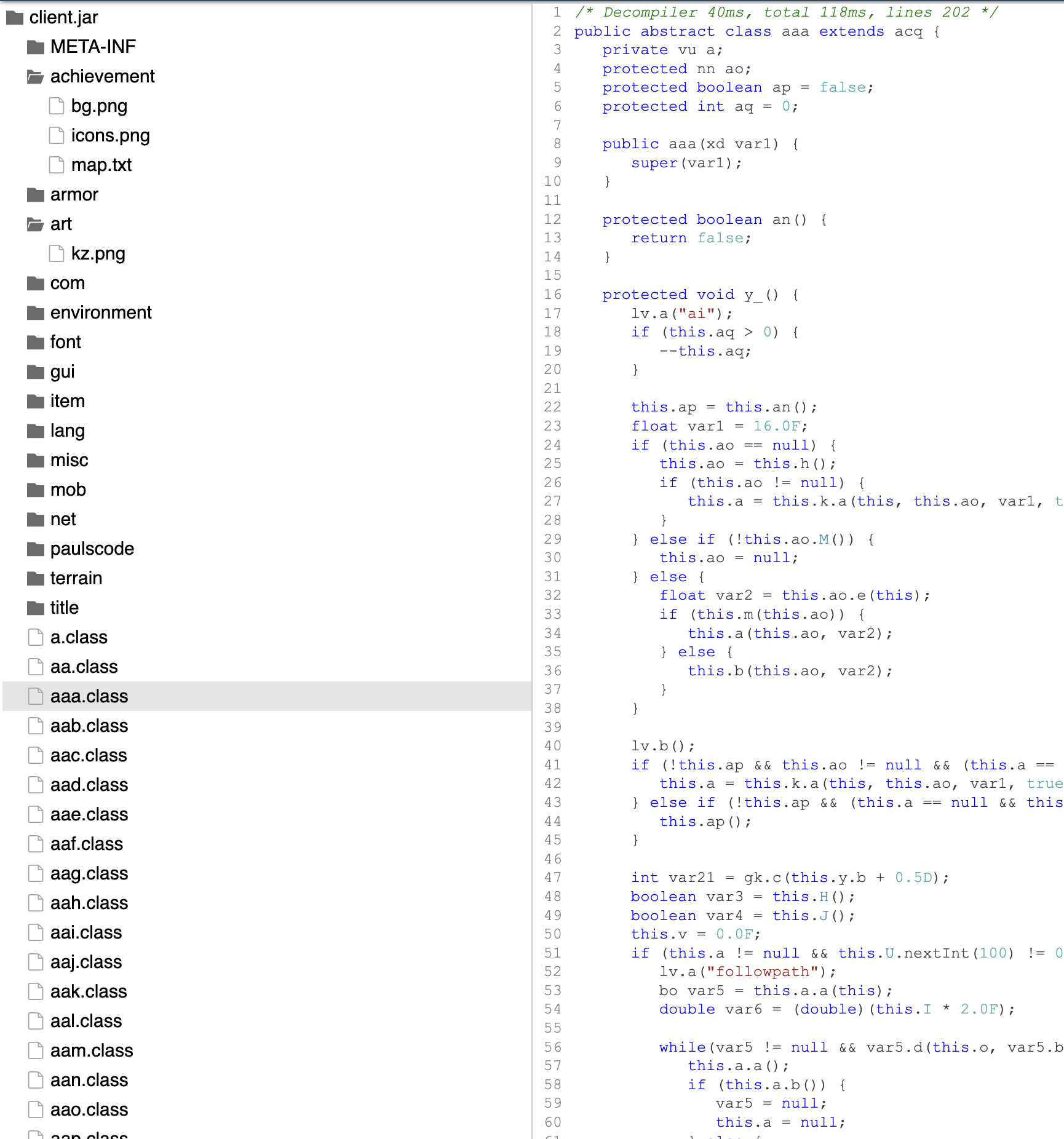
Now to find the classes that communicate with LWJGL ...
Would probably be easy to just use
grep
to find them, but that's if we really need to see what they didupdate on this: i will need to revisit this another day if thats OK
Sure no problem 🙂
I wonder if a
return 0
would work
I'm pretty sure the LWJGL impl just returns 0 for the other get window functionsUnfortunately the same thing.
Exception in thread "Thread-0" java.lang.UnsatisfiedLinkError: Java_org_lwjgl_opengl_LinuxDisplay_getRootWindow
, which is the same as if I don't have the stub at allLook up MCP
For deobfuscating Minecraft
Might be overkill at the moment given that I just want to render 8 lines of code for a 2D triangle. I might go down that route for a larger project, once I can get this triangle working
I'd suggest an even simpler example like just changing the glClear color to red. There is no implementation for glVertex2f in the natives.
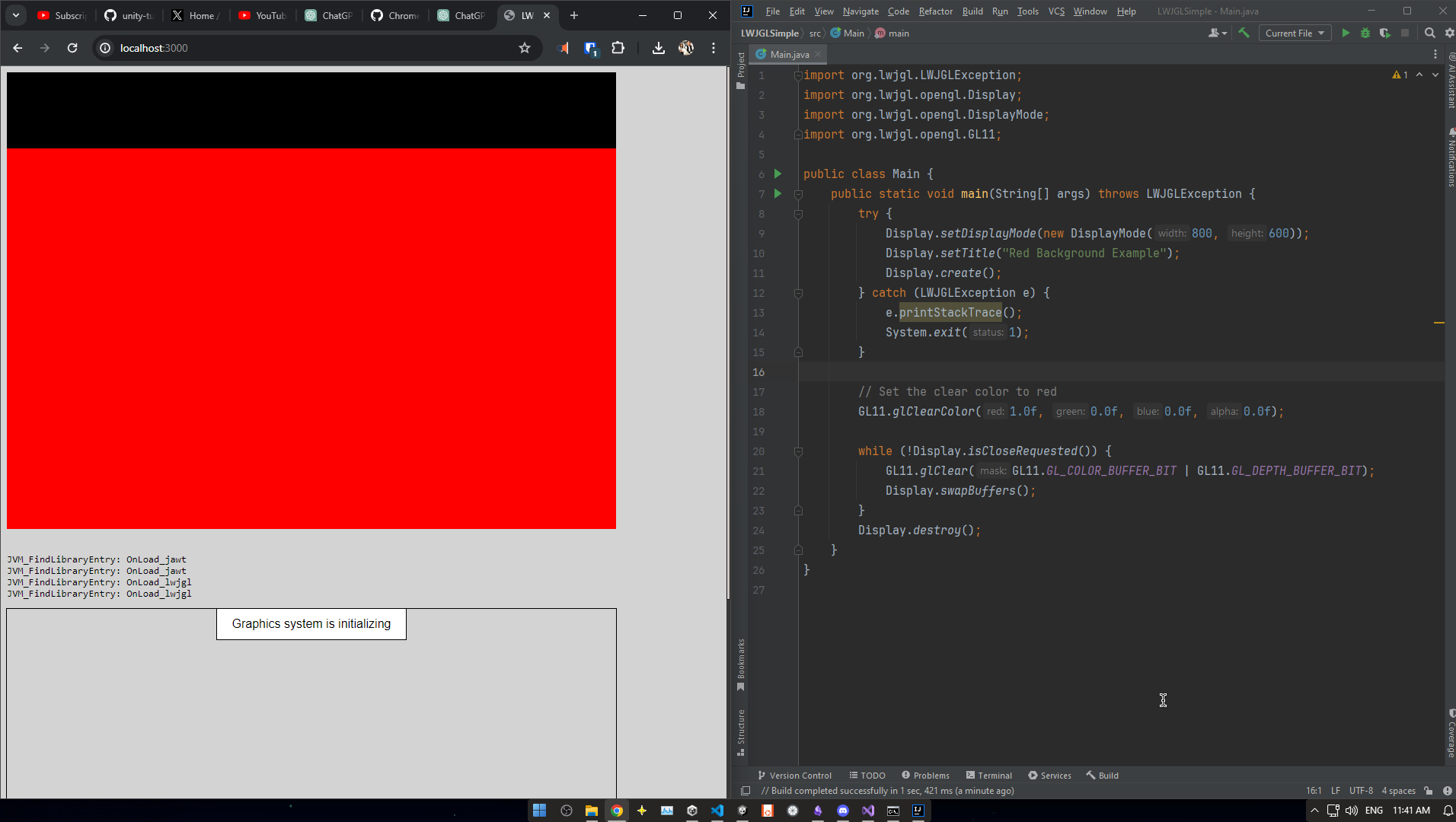
you can stub out LinuxDisplay_getRootWindow and LinuxDisplay_nSetWindowIcon as suggested above.
nice!
yeah, these just need stubs. happy to take a PR on cheerpj-natives
Okay cool, this seems to be working 😎 . Yeah looks like 2d drawing calls aren't implemented yet. I modified the code to draw a small red square (since it looks like quads are supported, but tris are not) with 3F calls instead, but keep running into
GL_INVALID_OPERATION: Vertex buffer is not big enough for the draw call
. I've tried making vertexBuf
larger than 32, but no matter what size I set it to, it results in the same thing. I'll try tinkering around with it some more and see if I have any luck What it looks like rendered on the desktop: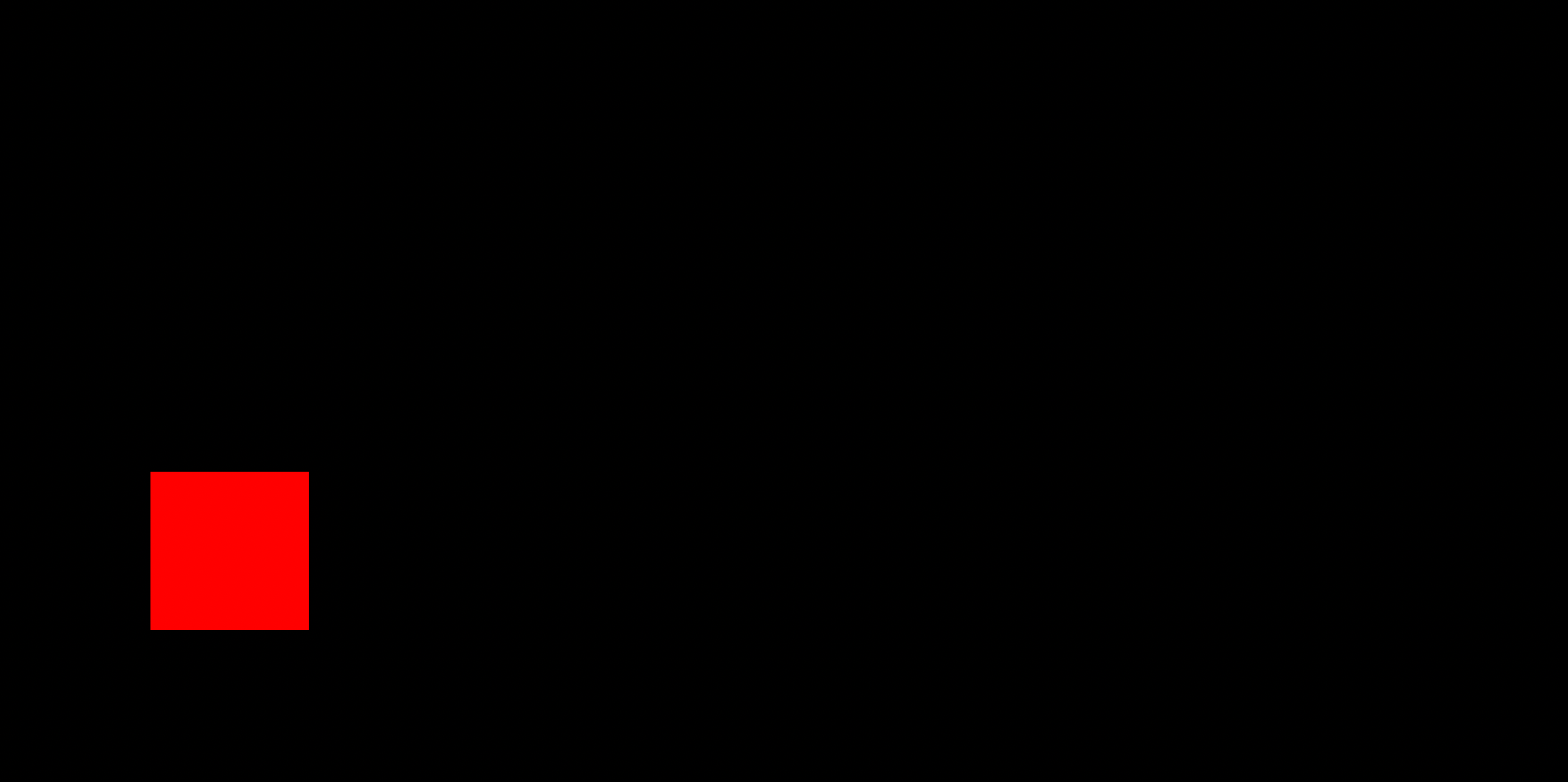
I think that tri fan and strip should be supported, just not TRIANGLES (it hits a
debugger
)
I had a play about with this this morning and it feels to me that it might be more sensible to look into supporting modern LWJGL3 and OpenGL ES (ie WebGL) instead of trying to hack together a compatibility layer for GL11->WebGL. (alternatively, we do that, but seriously - the current implementation is mostly hacked together just to support a specific version of Minecraft)
depends on your usecase @sean. perhaps you could explain what you’re aiming to build in the end?That sounds better, and Minecraft does support LWJGL 3 if you use a patched asset index API
Probably not for 1.2.5 tho
My long term goal was to port a legacy LWJGL 3d landscape and model editor that I wrote a few years ago for a game I'm working on. I thought it would be really neat to have it browser based
that sounds cool; let's make a thread in #cheerpj about it
not a problem, we only picked that version because it used GL1 iirc