Unsure what this error means
I'm building an Azure Function on .Net 6 and this return snippet is giving me this error. I am new to C#
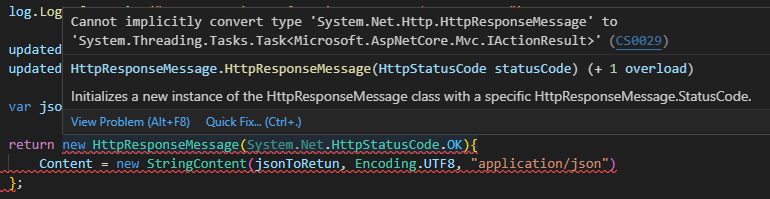
7 Replies
it means that a HttpResponseMessage is not a Task<IActionResult>
can you share more code for context?
sure its not long at all one second
you have two mistakes here. one is that your method is not async. the other is that HttpResponseMessage is not what you use to respond from a web API
using System;
using System.Net;
using System.IO;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
using System.Net.Http;
using System.Text;
using Microsoft.Azure.WebJobs.Extensions.CosmosDB;
using System.Linq.Expressions;
using Microsoft.Azure.Cosmos;
namespace Company.Function
{
public static class getVisitorCounter
{
[FunctionName("getVisitorCounter")]
public static Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
[CosmosDB(databaseName:"AzureResume",containerName:"Counter", Connection = "AzureResumeConnectionString", Id = "1", PartitionKey = "1")] Counter counter,
[CosmosDB(databaseName:"AzureResume", containerName: "Counter", Connection = "AzureResumeConnectionString", Id = "1", PartitionKey = "1")] out Counter updatedCounter,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
updatedCounter = counter;
updatedCounter.Count += 1;
var jsonToRetun = JsonConvert.SerializeObject(counter);
return new HttpResponseMessage(System.Net.HttpStatusCode.OK){
Content = new StringContent(jsonToRetun, Encoding.UTF8, "application/json")
};
}
}
}
i'm not familiar with azure functions but afaik you need an actual ASP.NET controller to use IActionResult
i was reading about async and using await. if use async it gives an error for the updatedCounter. I was ignoring it for now
but there's IResult for minimal apis that definitely exposes the right response types
this looks like a relevant example https://learn.microsoft.com/en-us/azure/azure-functions/functions-scenarios?pivots=programming-language-csharp#build-a-scalable-web-api
but you'd want to use https://learn.microsoft.com/en-us/dotnet/api/system.web.mvc.jsonresult?view=aspnet-mvc-5.2` instead of OkResult