How to get rid of nullable warning?
What are the usual ways to get rid of these warnings? Do I have to check if everything is null before doing something? That would mean I would have to add a lot of if statements all the time which I hope could be prevented
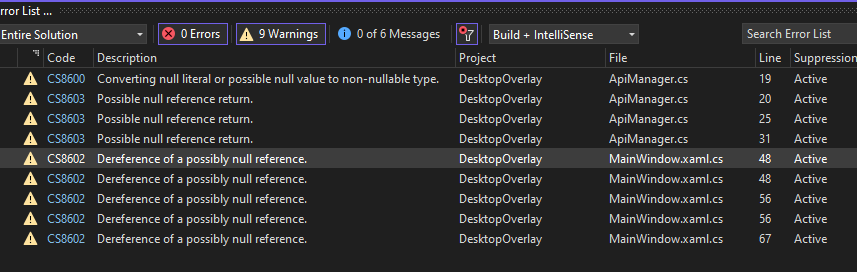
7 Replies
Do null checks and handle null cases
Null coalescing operator
Null conditional operator
Null forgiving operator
you can either do null-checks or assert that the relevant variable/field is never null
with annotations
if the compiler knows something can never be null (it's given a value in a constructor), it won't give you these warnings
So I have this code where I get data from an api which is nested
in some objects I can retrieve it using:
weatherData.Hourly.Time
So this is a nested object but if I want the time value, I cannot just check if weatherData is null
. I also have to check if weatherData.Hourly is null
.
But then my code ends up like this
Some nested if statements just to check if each object is not null
I dont like that solution
Any other options?
if you are specifically wanting Time, you could also do
I have the same issue for this line
T model = JsonConvert.DeserializeObject<T>(responseBody);
Using a if function to check if responsebody is null does not seem to workUse System.Text.Json instead...
You may also consider using httpfactory instead to avoid exhaustion problems with using + httpclient
https://learn.microsoft.com/en-us/aspnet/core/fundamentals/http-requests?view=aspnetcore-8.0
https://docs.microsoft.com/en-us/dotnet/standard/microservices-architecture/implement-resilient-applications/use-httpclientfactory-to-implement-resilient-http-requests
Make HTTP requests using IHttpClientFactory in ASP.NET Core
Learn about using the IHttpClientFactory interface to manage logical HttpClient instances in ASP.NET Core.
Use IHttpClientFactory to implement resilient HTTP requests - .NET
Learn how to use IHttpClientFactory, available since .NET Core 2.1, for creating
HttpClient
instances, making it easy for you to use it in your applications.