TypeError: Cannot read property 'KindeSDK' of undefined
// NPM
import {useState, useEffect} from 'react';
import {KindeSDK} from '@kinde-oss/react-native-sdk-0-7x';
import 'react-native-url-polyfill/auto';
// Interfaces
import ISession from './interfaces/ISession';
// Context
import {AuthenticationContext} from './context';
// Env
import {
KINDE_ISSUER_URL,
KINDE_POST_CALLBACK_URL,
KINDE_POST_LOGOUT_REDIRECT_URL,
KINDE_CLIENT_ID,
} from '@env';
export const AuthenticationProvider = ({
children,
}: {
children: React.ReactNode;
}) => {
const [currentSession, setCurentSession] = useState<ISession | null>(null);
useEffect(() => {
const client = new KindeSDK(
KINDE_ISSUER_URL,
KINDE_POST_CALLBACK_URL,
KINDE_CLIENT_ID,
KINDE_POST_LOGOUT_REDIRECT_URL,
);
console.log('Client = ', client);
const checkAuthenticate = async () => {
// Using `isAuthenticated` to check if the user is authenticated or not
if (await client.isAuthenticated) {
const userProfile = await client.getUserDetails();
const {permissions: userPermissions} = await client.getPermissions();
const claims = await client.getClaims();
setCurentSession({
user: userProfile,
permissions: userPermissions,
claims,
});
} else {
console.log('Client is not authenticated');
setCurentSession(null);
}
};
checkAuthenticate();
}, []);
return (
<AuthenticationContext.Provider value={{session: currentSession}}>
{children}
</AuthenticationContext.Provider>
);
};
// NPM
import {useState, useEffect} from 'react';
import {KindeSDK} from '@kinde-oss/react-native-sdk-0-7x';
import 'react-native-url-polyfill/auto';
// Interfaces
import ISession from './interfaces/ISession';
// Context
import {AuthenticationContext} from './context';
// Env
import {
KINDE_ISSUER_URL,
KINDE_POST_CALLBACK_URL,
KINDE_POST_LOGOUT_REDIRECT_URL,
KINDE_CLIENT_ID,
} from '@env';
export const AuthenticationProvider = ({
children,
}: {
children: React.ReactNode;
}) => {
const [currentSession, setCurentSession] = useState<ISession | null>(null);
useEffect(() => {
const client = new KindeSDK(
KINDE_ISSUER_URL,
KINDE_POST_CALLBACK_URL,
KINDE_CLIENT_ID,
KINDE_POST_LOGOUT_REDIRECT_URL,
);
console.log('Client = ', client);
const checkAuthenticate = async () => {
// Using `isAuthenticated` to check if the user is authenticated or not
if (await client.isAuthenticated) {
const userProfile = await client.getUserDetails();
const {permissions: userPermissions} = await client.getPermissions();
const claims = await client.getClaims();
setCurentSession({
user: userProfile,
permissions: userPermissions,
claims,
});
} else {
console.log('Client is not authenticated');
setCurentSession(null);
}
};
checkAuthenticate();
}, []);
return (
<AuthenticationContext.Provider value={{session: currentSession}}>
{children}
</AuthenticationContext.Provider>
);
};
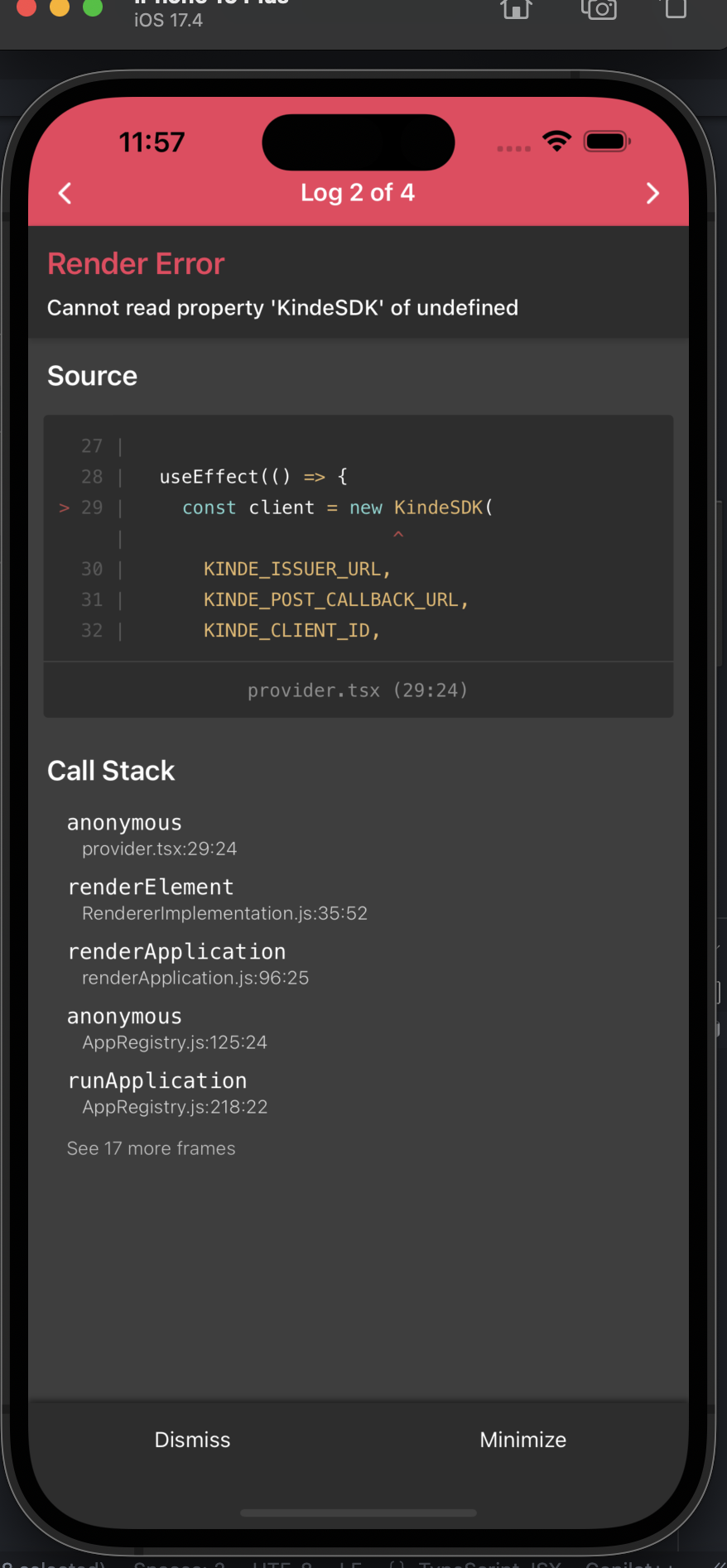
4 Replies
ERROR TypeError: Cannot read property 'NativeModule' of undefined, js engine: hermes
at AuthenticationProvider (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:162140:24)
at DeviceProvider (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:162098:24)
at App (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:124622:54)
at RCTView
at View (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:59306:43)
at RCTView
at View (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:59306:43)
at AppContainer (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:59148:36)
at JawabwalaMobileApp(RootComponent) (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:110763:28)
ERROR TypeError: Cannot read property 'NativeModule' of undefined, js engine: hermes
at AuthenticationProvider (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:162140:24)
at DeviceProvider (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:162098:24)
at App (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:124622:54)
at RCTView
at View (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:59306:43)
at RCTView
at View (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:59306:43)
at AppContainer (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:59148:36)
at JawabwalaMobileApp(RootComponent) (http://localhost:8081/index.bundle//&platform=ios&dev=true&lazy=true&minify=false&inlineSourceMap=false&modulesOnly=false&runModule=true&app=com.opinionatedinnovations.jawabwala:110763:28)
ERROR TypeError: Cannot read property 'KindeSDK' of undefined
This error is located at:
in AuthenticationProvider (created by App)
in DeviceProvider (created by App)
in App
in RCTView (created by View)
in View (created by AppContainer)
in RCTView (created by View)
in View (created by AppContainer)
in AppContainer
in JawabwalaMobileApp(RootComponent), js engine: hermes
ERROR TypeError: Cannot read property 'KindeSDK' of undefined
This error is located at:
in AuthenticationProvider (created by App)
in DeviceProvider (created by App)
in App
in RCTView (created by View)
in View (created by AppContainer)
in RCTView (created by View)
in View (created by AppContainer)
in AppContainer
in JawabwalaMobileApp(RootComponent), js engine: hermes
Hi @acadmaniac,
Thanks for reaching out.
I will get my React Native expert team mate to look into this issue.
I'm sorry but we had to move to supertokens because we're in the process of spinning up a quick MVP and we did not have enough time to wait for a resolution. We searched this error on google as well and found no relevant results.
I wish you guys all the luck though!
Hi @acadmaniac,
Thanks for letting us know this.
Good luck to you too!