Am I doing this right?
Hello, this assignment is confusing me very much, the basis for it has been done but I don't know if I have a certain section done correctly... This here is the overview
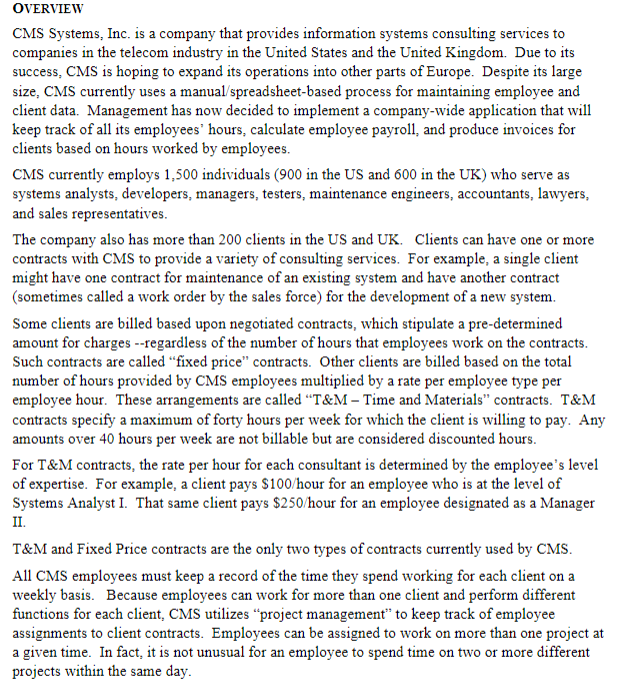
11 Replies

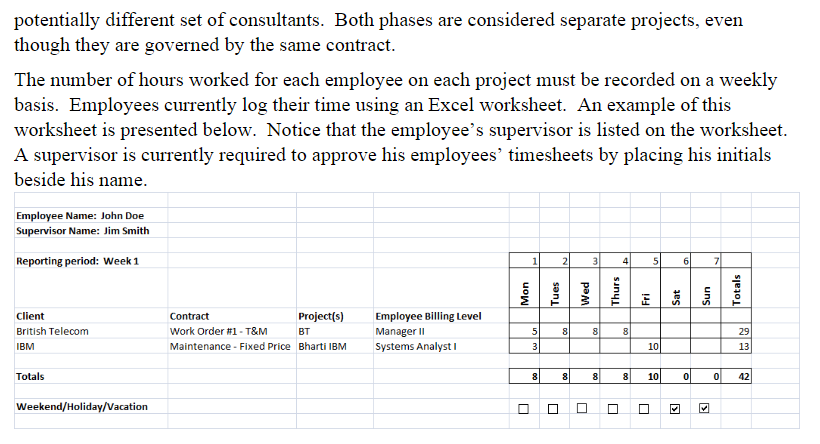
These are the instructions:
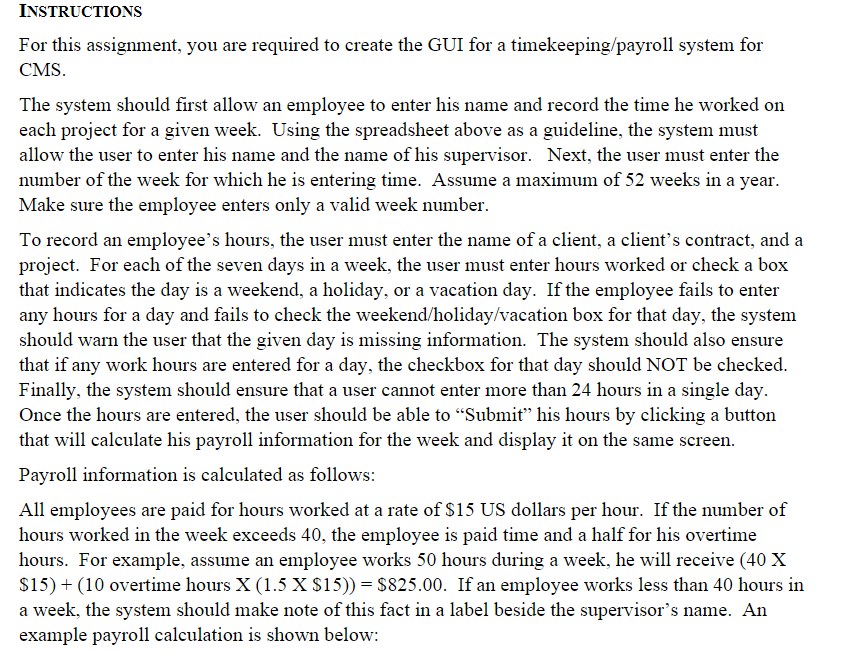
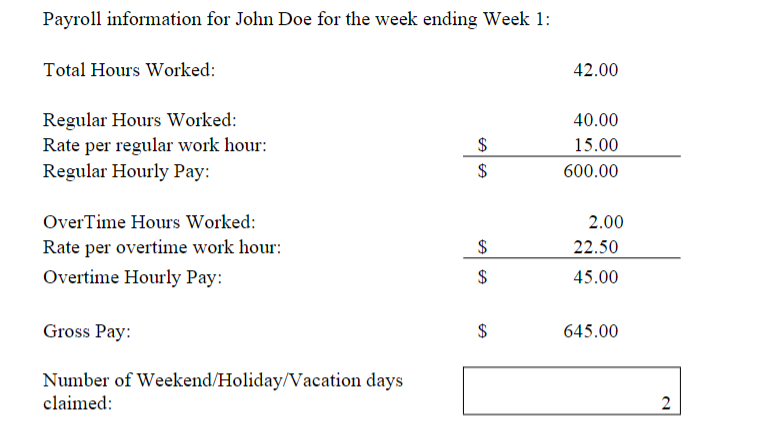
This is what has been done so far, I'm wondering if I am on the right path concerning the class TimeSheetEntry.
Especially where I have the days of the weeks listed
Is the TimeSheetEntry good so far or am I way off?
a few sidenotes, generally I don't use manual set/get methods, just use autoproperties
and second, the
TimeSheetEntry
looks a bit odd considering the user will input their daily hours every day. Edit: nevermind, assignment mentions "weekly"
I just shuffled through the assignment, but it feels odd to input every day of the week when you're inputting on Monday for exampleI don't know what autoproperties are, I hardly understand get/set methods so I'm just gonna stick to what I'm familiar with
So should I put things like "Week 1" instead?
Auto-Implemented Properties - C# Programming Guide - C#
For an auto-implemented property in C#, the compiler creates a private, anonymous backing field accessed only through get and set accessors of the property.
and as such, no
GetName
/ SetName
is needed as you can just reference the Name
itself
the current implementation is ok as isOk thank you