Cannon not following mouse?
Hello!
I'm trying to have my cannon change position based on where the mouse is, but it always chooses to the same angle when the mouse is clicked no matter its placement on the screen. Does anyone know what is causing this? And what I need to fix and with what?
C# in Unity
5 Replies
Just quick coding convention.
C# does not use
_
except for backing fields.
so _Fire()
would be Fire()
Also what perspective is the camera?
First-Person?
Or top-down?Sideways? I'm trying to make the game angry birds styled in a way
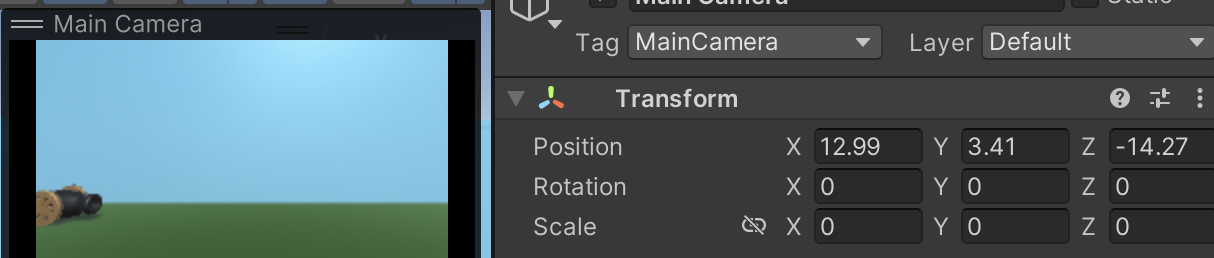
the cannonballs fire out of the cannon when the mouse is unclicked after being held down, so the fire function works, its just the angle of the cannon never changing
I just realized I believe I was having issues because of my projection settings on my main camera being incorrect which was throwing off everything else
Thank you for the help, I really appreciate it!
:blobthumbsup: But I barely if ever helped, either way. No problem!
If you have no further questions, please use /close to mark the forum thread as answered