Long running process in Blazor Server
I have a Blazor Server app, and I have a page that will kick off a long running process. I am calling an async method without an await in order to get the response back quickly, and it is starting the processes fine. But it seems like whenever there is a long running syncronous task running in the background, it will lock the UI thread. How can I make this process run in a way that doesn't block the UI thread?
Here's a sample project to show what I mean:
40 Replies
Every time the loop hits the await, it does return control back to the UI, but then it locks again the next time it does the
Thread.Sleep(5000)
I looked into using BackgroundServices, but not sure if that's what I need in this case, since it's not something that is always running while the application is running, but only when a job is submittedUnknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I'm not exactly sure what you wanna achieve, but starting
LongRunningJob
doesn't make it run on a different Thread just being marked as async
.
You need to create a new Thread
, either by the low-level Thread
class or, better, by creating a Task
, something like Task.Run(() => LongRunningJob);
Yeah, I guess I don't have much concept of what "background" means
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
So
Task.Run
is different than just calling a method with no await, I assume?Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yeah, that's what I'm asking, I mean. What do I use to offload that?
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yes, it does create an actual new Thread; you can check this by setting a
Thread.Current.Name = "Foobar"
and pause the process in Debug
. You'll see all the running Threads
, one will be named Foobar.Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I gotcha. I guess I always assumed that I only needed to do Task.Run if I was trying to run a sync method as async
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yeah, I don't use Thread in a real world situation, was just looking for something that would lock syncronously
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Well I'll try out Task.Run and I assume that'll do what I was trying to do
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Thanks to you both
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
No, I don't
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Sweet, thanks
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Oh cool, I'm interested in knowing more about that, so I'll look more into Enqueue for backgroundQueue
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Ahh Channel, I think a friend told me about that, and I couldn't remember the class
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Oh neat, then just write to the channel with a new job request?
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Cool, that's not too complicated
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yeah, that's what I'm doing now anyways. I'm just using a SemaphoneSlim as a queue, but maybe that would be better
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Opinion: first article looks better as an introduction than the devblog one, but but should still be read in that order
https://github.com/tkp1n/ndportmann.com/blob/master/posts/2019-01-03--system-threading-channels/index.md
https://devblogs.microsoft.com/dotnet/an-introduction-to-system-threading-channels
https://www.stevejgordon.co.uk/an-introduction-to-system-threading-channels
Video:
https://docs.microsoft.com/en-us/shows/on-net/working-with-channels-in-net
To make it a bit more clearer, here's what I mean:
Will show up in the List,
Debug
->Windows
->Threads
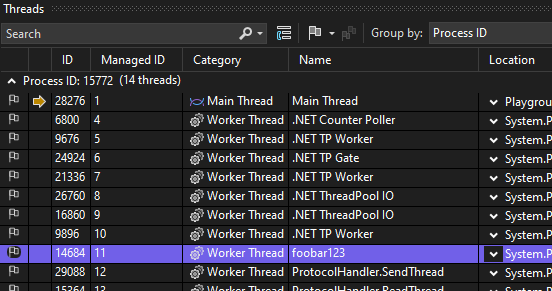
As seen, it's not blocking the
Main Thread
/UI Thread
.
When working with Threads
and alike, it's very helpful to have the Threads
window open in the debugger, it let you identify in which Thread
you're currently in and so on.Oh neat, I'll have to watch that
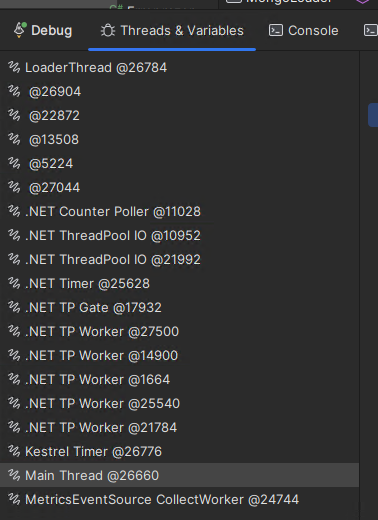
Seems to be working for now as I expected. May need to play around with my hacky queueing functionality, but for now, it's not locking my UI thread, so I'm happy
To make it even more clearer;
Task
is just a wrapper for Thread
. Thread
is rarely used these days and considered being low-level, it's useful when in need to set the ApartmentState
, etc. - Yes, as @TeBeClone mentioned, today you use Task
in 99% of the cases.
Task
handles a lot of things you had to do yourself when using the Thread
class.