Hi I Need Help In Two Dimensional Arrays
How do I check the values below and above the main diagonal in a square array?
78 Replies
Does this snippet explain it for you?
I Didnt Mean It Like That
if i want for example to check if all the values below the main diagonal are 0 how im i supposd to do this
Can i get some help
Talking about main-diagnal I think you mean a matrix.
First off, you need to determine it and next create a method for checking below and above.
You need
for
loops for this; looping through a 2D Array is done by nesting a for
loop into another, first loop will iterate through the row and the inner loop through column, like so
Is this it?
bro its like school type of somethhin
what is var
Type inference
Don't worry about it, tbh
mb idk why halve is var and halve is int
the Question is you need to code a method that returns a true of false if:
all the values below the main diagonal are 0
and all the values in the main diagonal and above it need 2 be and number other than 0
Now, that you know how to iterate through a matrix, the rest is up to you, to do the calculations and iterate through the main diagonal. I won't provide you the solution to your homework ¯\_(ツ)_/¯
this is what you mean by diagonal right
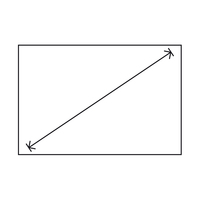
bro its like a 30 Q homework
i solved everything execpt this thing
yea when the row and col are equal
So what exactly are you stuck on?
Accessing the array? Figuring out the main diagonal?
no
my problem is
how to get the values below and above the main diagonal
how the structure of the for is going to be
Well, if the matrix has a cube shape and a side length of... 5
We know the diagonal is 0,0 to 5,5 right?
yes
row = col
If you visualize this array, you quite quickly see a pattern for what is below the diagonal and what is above it
yea
or am I spoiling
A little 🙂
he didnt have time to copy
😆
below the main diagonal the row > col
and above the row <col
Okay
@Kringe
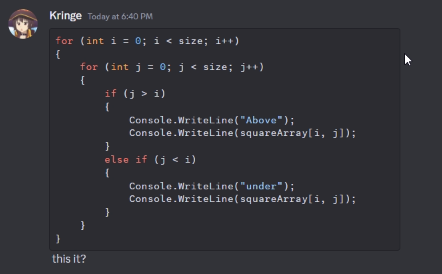
xD
Damn
i dont want it like that
i want to understand bruv
So now you know how to differentiate it
What's stopping you from understanding?
thats the spirit
idk what to do
tbh
I'm unfortunately on a phone atm, otherwise id write a program that prints the matrix and uses colors to show above/on/below.
You know exactly what to do thou
Ill do it
you mean somethin like that
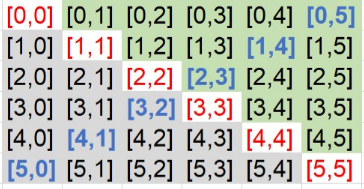
Sure.
do you also have to do something with the second diagonal or only the red one
i know what i need 2 do but not how to do it
only the red one
Combine some loops with an if statement?
And then you are more or less done...
the problem is with the loops
Do you know how to use breakpoints?
like yea
but why
Why are the loops a problem?
Look at the picture you posted
Set your breakpoint at the inner for loop and just loop through it I think you will understand how it works
i dont know how to form them
Well you have two options
im already lost my guy
Either loop through everything and use an if to decide if you want to continue or not
Or write your loops cleverly to only target above or below the diagonal
The second is better, but might be harder
Try using
x
and y
as variable names, like I did in my example, not i
(increment) or j
, makes things clearer.
Also, if using VS, set a breakpoint with F9
, run the code and step through it with F10
.
Do it, you'll understand that way.the second one is the one im supposd to do
i will do it
just going to eat
rn
Is that because we talking about squares in this context?
Because we have coordinates in a 2d matrix, like
Vector2
, representing a single point of data.X/y are the standard coordinate names
y thats what I meant will do thanks
changed var to int and added coordinates for clarity
i will see it when i comeback
ty
or i will try to solve it when i comeback and if i need it ill look at it
i understand it now
soo if i want to check if all the values under are 0
i just loop the array and if(row > col)
thats it ?
Yes nice!
wait ima show you the solution
its wrong bro
how am i supposd to do it
Could you paste the code
static bool Q4P141(int[,] mat)
{
for (int i = 0; i < mat.GetLength(0); i++)
for (int j = 0; j < mat.GetLength(1); j++)
{
if (i > j && mat[i, j] != 0)
return false;
if (i < j && mat[i, j] == 0)
return false;
}
return true;
} the purpose of the method is to check of all the values below are 0 and above and in the main diagonal are different from 0 aka any other number
} the purpose of the method is to check of all the values below are 0 and above and in the main diagonal are different from 0 aka any other number
you are returning after checking the first value
all the values need to be 0
soo if one value is not 0 you can return false
you can say
if(i>j)
if(ma[i,j]!=0)
return false
wait that was wrong so to clarify you only are checking the vals under the daigonal
right
under and above
and the above condition is
all the values in the main diagonal and above need to be any number but 0
ow mb
but i think you turned it around
can u check it for me
okay il make it a bit clearer
I also learned you should x an y for this
ill try it
if you want to include the diagonal
without the comments
@ahmadhija🌵 Im gonna go did you figure it out?
nah
its still wrong
past the code
and the array for me plz
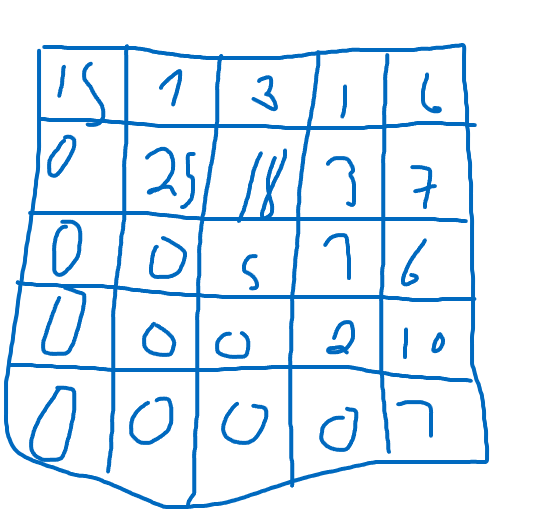
array
code is that
it should return true right
looks good to me
let me try it
sooo im the one who cant check
ty
soo if any q i can solve it with this pattern
Loop
if
and then what i want to do
depends on the question I guess
but everything working now?
yea
nice finally
luv u man
last thing y is row
and x is col
soo when y>x you are talking below
and x is the opposite