Flex-item not shrinking and causing overflow
Hello, I'm working on a simple UI for utility tool and I need bit a help with css, basically I got a div that lists all files inside a scripts folder, however the span item (so the file name) just doesn't shrink causing the whole container to overflow
Like this
https://mentally-insane.pixeluted.com/BOXe6/PELotUxU44.png
I'm using svelte for this project, so this is the code for the div
I don't know what code you need, but feel free to ask for more code and I will send it.
Also the main container is not overflowing https://mentally-insane.pixeluted.com/BOXe6/HaRiYaSa84.png
Just the container that contains the search button and the list of files (this overflow didnt start until I started implementing the files)
https://mentally-insane.pixeluted.com/BOXe6/HAjAjEhe20.png
Thanks for any help!
47 Replies
I suspect it is the editor together with the file explorer that is causing the issue.
does the left part have a fixed / min-width.
It might be easier for us if you copy the rendered html and css to a codepen or sandbox
ohh that is possible?
sorry im new to this all
could u tell me how to do it?
yea, just inspect the element, right click on the body tag and copy
got it
do i have to copy it over somewhere?
you can do it on codepen.io (you need an account to be able to save it) then you can share the link
hmm the html tag doesnt include css
since im using vite
css you can copy seperatly from you components
like copy all the css from compoments to one css?
yea
k
yeah that doesnt look good
I don't think I can share the UI this way
The two parts have set width
one is 75% and the other is auto
to fill whatever is left
is it a flex container as well?
inneed
remove the percentage width and try flex: 1
think this is the issue
90%is a lot. on the tab editor. make that flex 1
remove the flex-grow on the explorer
yeah it now doesnt scale how i wanted to and the overflow wasnt fixed https://mentally-insane.pixeluted.com/BOXe6/WaZASAsA60.png
yeah the width is there bc i want it to be bigger
than the other part
maybe better would be flex-basis for this
so tab-editor: flex: 1 (that will take all available space)
explorer: just empty (remove flex-grow) so it will be the smallest size possibly
ok does the effect i wanted but still overflows
oh yeah it just doesnt shrink at all
when i removed the media query
that set width to lower values
yea, there must be more set values then. really hard to tell without having access to the whole thing
maybe try codesandbox and upload the project there
does it work with tauri apps?
not sure, i normally don't use it. maybe someone else can chime in to help you share it
well the code is public
for the project
maybe this could be also why
yeah i removed the width and didnt help but didnt broke it
so it wasnt needed
so what has set width hmm https://mentally-insane.pixeluted.com/BOXe6/hemOxIvO00.mp4
I don't see any more set widths in the project
well, seeing the code editor isnt shrinking makes me believe something is forcing the width.
can you screenshot that part in the inspector
editor?
the editor is just div which uses monaco
ok it is monaco causing the issue
i just disabled it settuping the editor
and it scales normally
god damm you microsoft devs
seems like someone got the same issue
https://stackoverflow.com/questions/69280251/how-can-i-make-the-monaco-editor-size-to-its-parent-div
Stack Overflow
How can I make the monaco editor size to its parent div?
So I am trying to create a layout with two instances of the monaco editor side-by-side. I'm working with react, and I'm using the @monaco-edior/react component.
I have created the desired layout u...
monaco editor is the reason its being forced
but idk why it just doesnt to shrink
@MarkBoots so I got possible solution, that is make another container at the same position size as the real container
so that doesnt get affected by monaco editor width and height
and i can then update it myself in js side
however i have trouble getting the mirror container properly working https://mentally-insane.pixeluted.com/BOXe6/roDAKobA84.png
Got it fixed, thanks @MarkBoots https://github.com/microsoft/monaco-editor/issues/3393
GitHub
[Bug] automaticLayout doesnt shrink to container within flex layout...
Reproducible in vscode.dev or in VS Code Desktop? Not reproducible in vscode.dev or VS Code Desktop Reproducible in the monaco editor playground? Not reproducible in the monaco editor playground Mo...
a good. sorry wasn't able to respond anymore yesterday. Glad you still manage it
👍 quick question, do you have any idea why is this not causing a scroll bar appear on my x axis?
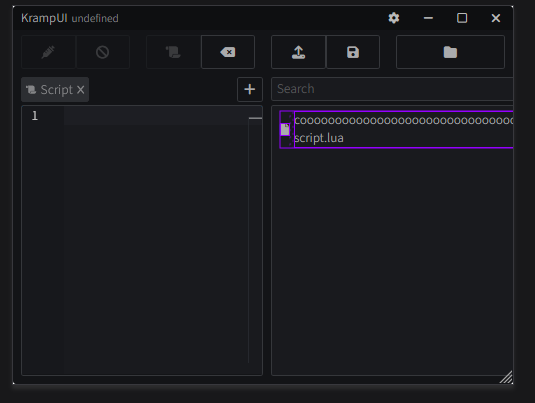
hard to tell, but probably there is an overflow: hidden on it somewhere
interesting
Figured it out, however bc the name is too long, it uses a lot of the space so they layout is bad
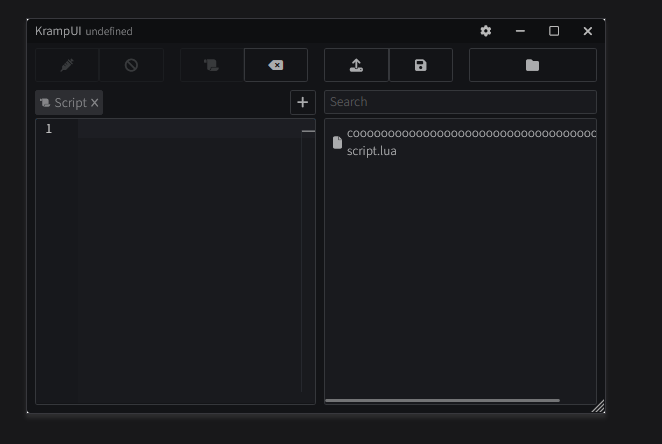
if you get what i mean
Fixed