Entity Framework Core 8 -Foreign Key Constraint failure.
Hello, I am playing around with EF code first and I am trying to model 3 tables:
I can add 1 Product entity to the DailyNutrition collection, but if I try to add more I am getting the "Foreign Key Constraint" error.
To be honest it's unclear to me which FK is referring to.
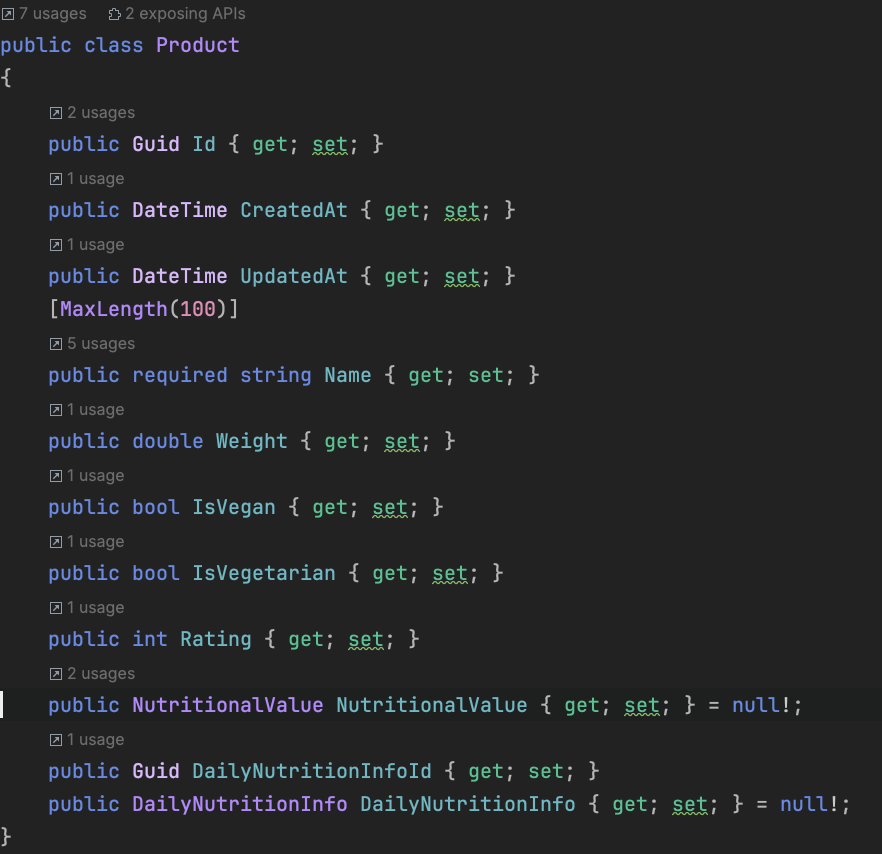
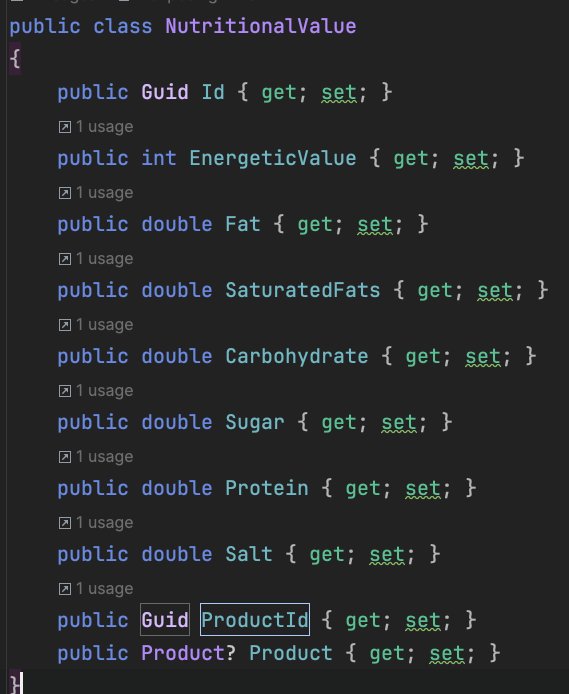
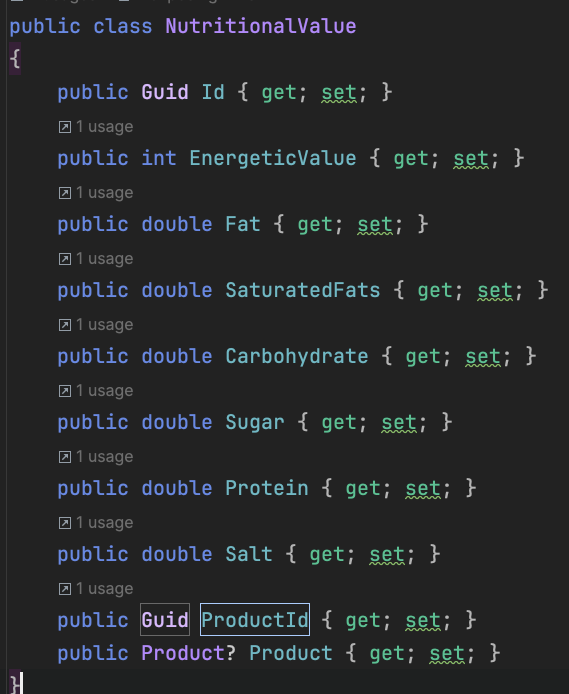
48 Replies
The error would specify what the actual missing constraint is
Could you tell me where to look for this?
I will paste screenshots where i've been looking for:
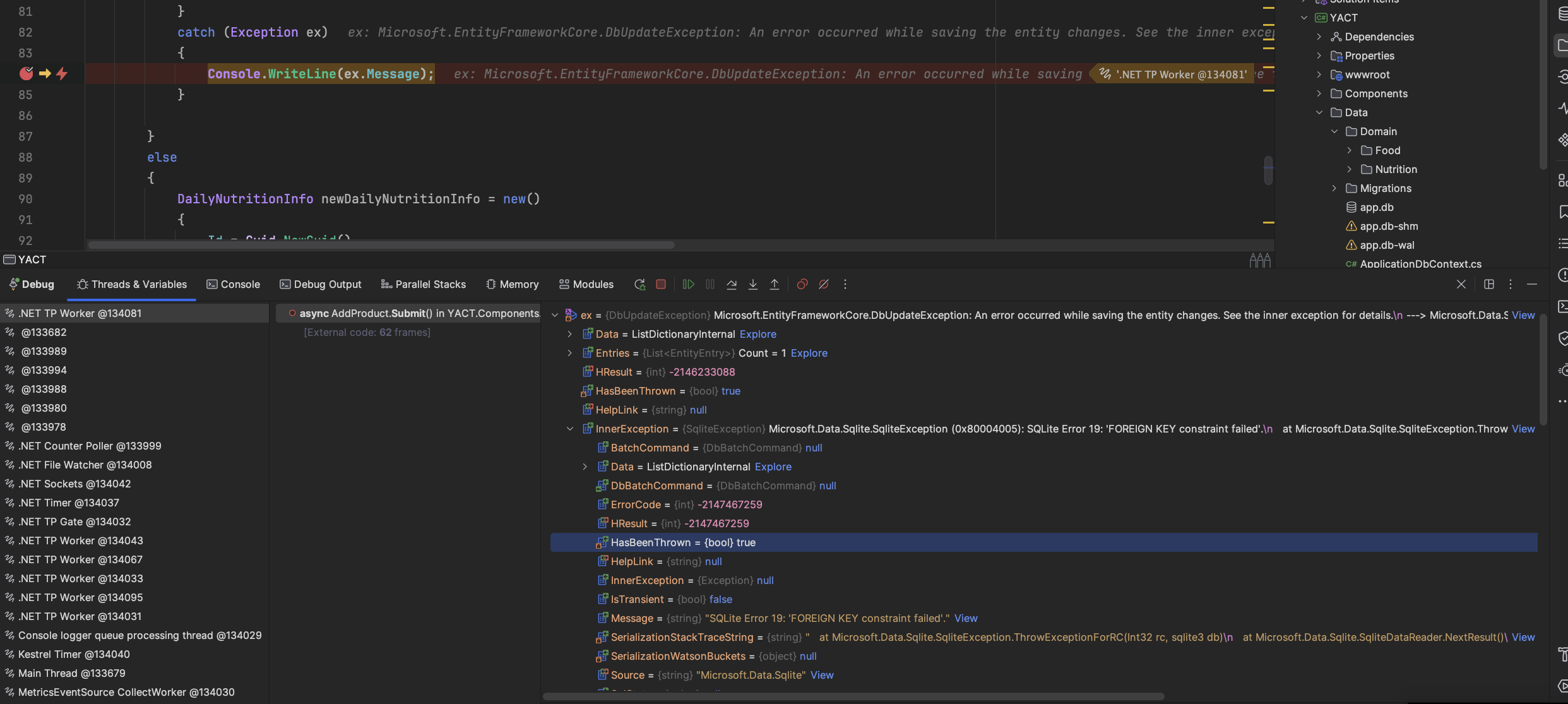
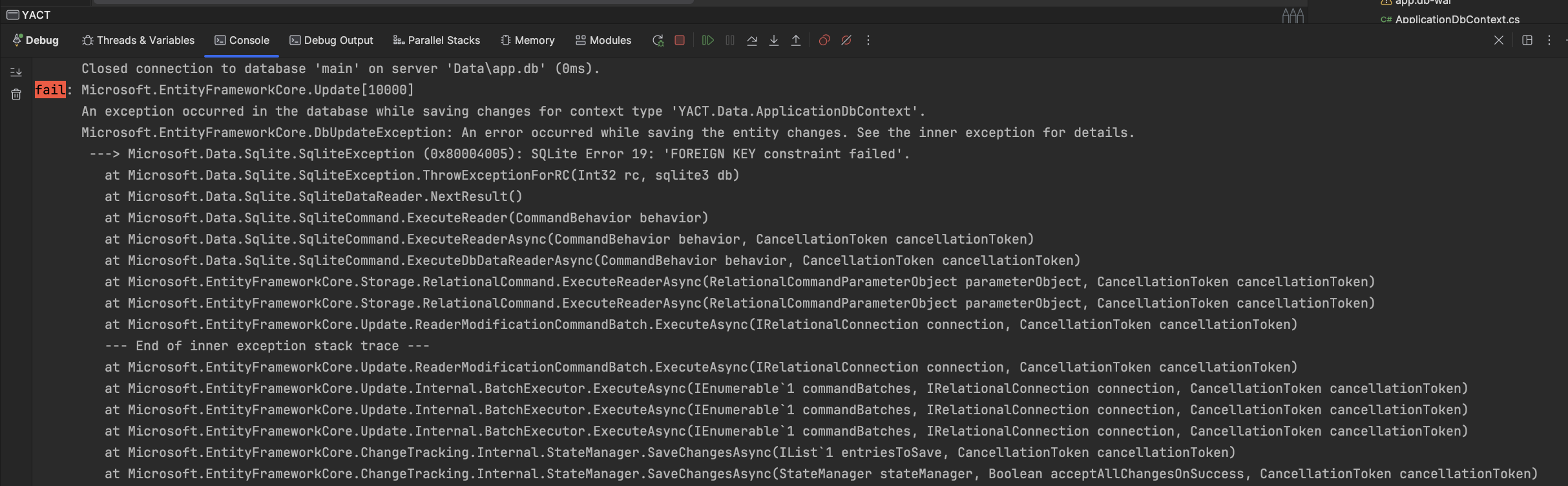
well the way u made it, its one to one
You mean Product - NutritionalValue?
DailyInfo - Product I hope its one to many
Will take a look in a bit
Well what ur saying is that X should accept more than one of Y
And at least from the 3 models u provided I did not see anything that would allow for that
Shouldn't DailyInfo have a collection of Products. And in turn each product shold have 1 NutritionalValue reference
if DailyNutrition is supposed to accept multiple products then yes
its what composes a one to many
https://learn.microsoft.com/en-us/ef/core/modeling/relationships/one-to-many
Yes, that's how I wanted it to be.
I will remove the reference to the related entity and test how that works out, should be fine since there are only 2
It seems it's related to the DailyNutritionInfo - Product relationship
well u have to remove/update the migration with the updates or modify the table
ofc that depends how ur doing it dbfirst or codefirst
I am doing it codefirst, I've been dropping the db and creating a fresh migration for each change
sure, can you $paste your models to the below site?
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
or if u got it working just ignore it 😛
Will do, I didnt get to make it to work yet
BlazeBin - nhjclnsxalbs
A tool for sharing your source code with the world!
Should I also paste the code where I am trying to create these entities? Maybe I am doing something wrong there
BlazeBin - tzuvdmmgkyir
A tool for sharing your source code with the world!
that cuts me the job of doing it my self to test so thx
Thanks a lot btw.
I guess I can just paste the whole component. Will update
BlazeBin - tcaeoqnibbqx
A tool for sharing your source code with the world!
it would be something like this
But this way there will be a one to many between products and NutritinalValue
ah my bad u want it to DailyNutrition
the idea is the same
I have added this, but it behaves the same
but wasn't the issue that u were trying to add more than one nutritionvalue to products
rrr products to nutrition value*
No it's the other way around
I can add 1 product to a dailyNutritonalInfo
But when I try to add more I get that fk issue
I have completely commeted out and made a migration without the nutritional value part
ok let me update sec
updated the above
It's the way I have it also
I guess this means I am not creating it properly in blazor
that should work, let me give it atry
works for me
well
Could you share how you created the entities before saving them?
ah ok I see the problem u should not set the id
the db sets it
mm unsure if sqlite handles guid thou
Which one? On the Product?
Or on each product for the DailyNutrition FK?
for example
in this example it will give both an id
now say u do
this 2nd product will be using the already existent nutrition
Oh OK, then should I assign the reference to the existing nutrition
if u don't want to create a new item yes
No, I want it to be added to an existing dailyNutrition for the currentDay
Let me try it out

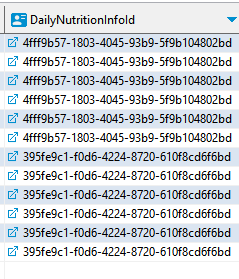
first one is dailynutrition created so u can see 2
second one are products using referencing the 2
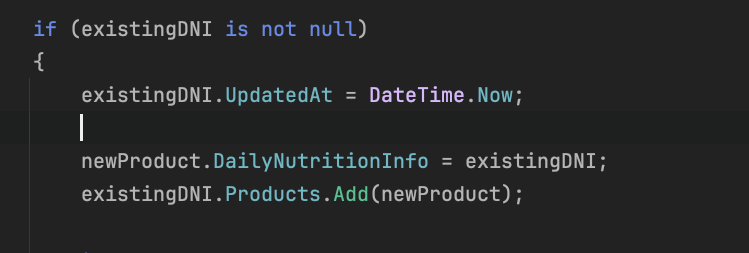
But if I add a reference to the Product of the DailyNutrition
Should I also update manualy the lists of products inside DailyNutrition?
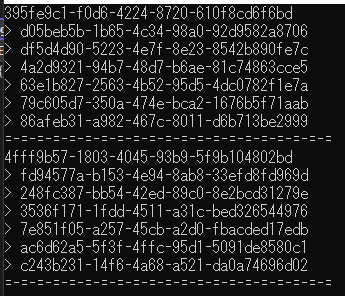
if you're doing it the opposite way yes
u would be adding a product to it
u dont need to do both thou
if u already set either the GUID on DailyNutritionInfoId or gave it the reference on DailyNutritionInfo
then u dont need to add it to the collection
and if u add product to the collection then u dont need to add the references to product as I understand it
i.e.:
if I do
this is fine, if I do
this is also fine
also fine
you dont need to do all, just one of the above
It worked
Thanks a lot
This was the issue, I was doing both things
cue the FK constraint issue
if u do both they collide
That's a relief
if u have no further questions throw a /close to mark it as solved 😉
I don't have any questions about this right now. Will continue fighting adding Identity now 🙃 .
Thanks a bunch!
well unhappily I won't able to help u with identity asp.net side but gl