[✅] Receiving Game Controller Input while Minimized (WinForms C#) | Repost
Pretty general question here. How would I go about receiving input from my user while they aren't tabbed into the program or have a different window as their foreground window? I'm using the InputSimulator Nuget package (InputSimulatorStandard version), and I'd like for my program to send inputs to a game window when it receives controller input. My only issue, now, is that the controller input isn't received unless I have my application as the foreground window.
This timer runs when a checkbox is
on
, and is stopped when that checkbox is turned off
. It functions fine, but I can't receive said controller input unless the app is opened, which kind of ruins the whole point of this functionality.
I've experimented with trying to use something of similar function to GetAsyncKeyState()
, but as far as I know, nothing of such would work for a controller.
Previously, I created a thread regarding this question and received two answers; one to use RawGameController
rather than Windows.Gaming.Input.Gamepad
, and the other to check if my application receives input in the background. While the use of RawGameController
did work for the project, my program did not receive background input while minimized. Upon using Spy++ as another user recommended, I reaffirmed that my program could not receive input when minimized.
I've tried to run my inputs via a background thread, but to no avail, as controller input was still only received while my program was the foreground window. Would appreciate some help on what direction I should try going with this.21 Replies
i know about the win32 raw input api and that one sends messages to your window message loop. you can also make it het global messages, not only in your window. i feel like you have tried wappers of it here but idk why those dont seem to work that way?
wouldn't the win32 raw input api only work for a mouse/keyboard? I don't think it supports game controllers, though I could be wrong
the previous thread i had opened for this was: #Receiving Game Controller Input while Minimized (WinForms C#)
it should have other hid devices too but maybe game controllers are special
Raw Input - Win32 apps
This section describes how the system provides raw input to your application and how an application receives and processes that input.
to be quite honest im not sure how i could use it to implement a game controller, haven't seen any documentation on how to do anything like that or similar
hmm yeah idk
different than raw input: i got input from a simple wacom tablet by reading it as a file but i had to figure out what the bytes mean myself
that's what i mean
i have no clue how to read the bytes or even get them properly lol
GitHub
GitHub - QubitTooLate/RawWacom: Small app to use a Wacom tablet as ...
Small app to use a Wacom tablet as a mouse. Contribute to QubitTooLate/RawWacom development by creating an account on GitHub.
you think anything like that exists for game controllers too?
:rozoa_shrug:
ive tried looking
but i havent found any
you might be able to read some bytes like what i did there
but might not be useful
yeah
getasynckeystate() is the easiest way to get input while minimized
but that's only for keyboards
yeah
i mean i have this
that's just what GamepadButtons accesses
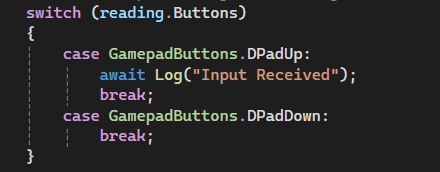
but i can't say i'm sure how i could use this to achieve the functionality im looking for
ds4windows does something similar but their entire process is vastly different since it's translating playstation input into xbox input
maybe a lowlevelhook if that input even gets sent trough that
yea i could try that
UPDATE:
@186 days until 🎃 @Yawnder - [▰▰▰▰▱] @Buddy, Citizen of Pluto
I apologize for the mass ping, but since you three were helping me, I owe a thank you. I've found my solution in the form of using the
SharpDX.XInput
nuget package.
The following usage has resulted in success, and the messagebox shows up regardless of whether or not I am focused:
:Thumbs:
Great!