90 Replies
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
👍
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
"the hand"? Did you mean "a hand"?
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
The end goal is to simply safely handle secrets, passwords, and whatnot for other services
For example, I need to store the Google ClientSecret for the OAuth2 somewhere
For the record, this is my first time doing OAuth and just working with these user info access / user sign-in prompts
Please feel free to enlighten me throughout this process
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Give me a minute to think
Cuz my code does both KV service registration and SecretClient and I'm getting confused lol but I understand what's going on
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I don't believe I need the SecretClient after
Main
because I just need to register the other services as indicated so I likely don't need the service
Yeah, I likely don't need the 2nd and don't need hot reload configUnknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yea, it's the
AddAzureKeyVault
I've tested that and made it workUnknown User•12mo ago
Message Not Public
Sign In & Join Server To View
The problem is that I'm not sure how to use that to register the other services
Namely because I don't know how to get the service at the
Main
step to get the secrets to register the other servicesUnknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Hence why I asked all that I did yesterday
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
e.g. AddGoogleOpenIdConnect
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Oooh
You want me to pull config into the
IConfiguration
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Hmm, that may be an issue because I don't know if I can always rely on Azure for my configuration since I may deploy with something else
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I'm honestly not sure how to add the
AddGoogleOpenIdConnect
part but it'll look like something like this:
That's correct. I've been working with .NET Framework for my current main job for the last 5 years and 4 years before that 🥲
I've been pushing us to use .NET Core for my main job's application but alasUnknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Nope, and I don't need to know according to your analogy
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Got it so far
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
So to clarify, you're implying that
IConfiguration
is something that initially pulls its values from appsettings.json that then can be updated by other services, namely AzureKeyVault here, that other services can then read?Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Sorry, I didn't understand this
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Aaah, understood
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Could you provide me with a basic explanation of the HostBuilder or provide me with a link to read more? I'm not sure what that is and if it has anything to do with the WebApplication.CreateBuilder stuff
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Ooh, did you mean
IHostApplicationBuilder
?Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Got it
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Sorry, I got lost on this and above
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Sorry, still a bit confused. Are you asking if I literally have a car? If so, no
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yep
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Aaah ok
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yea, I get what you're saying - that there's a level of abstract that doesn't require the web portion
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I'm planning to build a web app as well as a mobile app that supports iOS and Android so I actually do need to know these differences
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
My friends and I are planning to use a .NET back-end and a ReactNative front-end xD
But yes, back to the code
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Regarding the registration of the KV, where did you specify the e.g. credentials to access the KV? I only see that I've added the Uri in the first line
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
For the record, I will likely be running this through e.g. VSCode since my friends/coworkers will be using that
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yea, I think I've already done all of that in Azure
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Lemme double check that I've aded the app as a managed identity
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yea, already registered
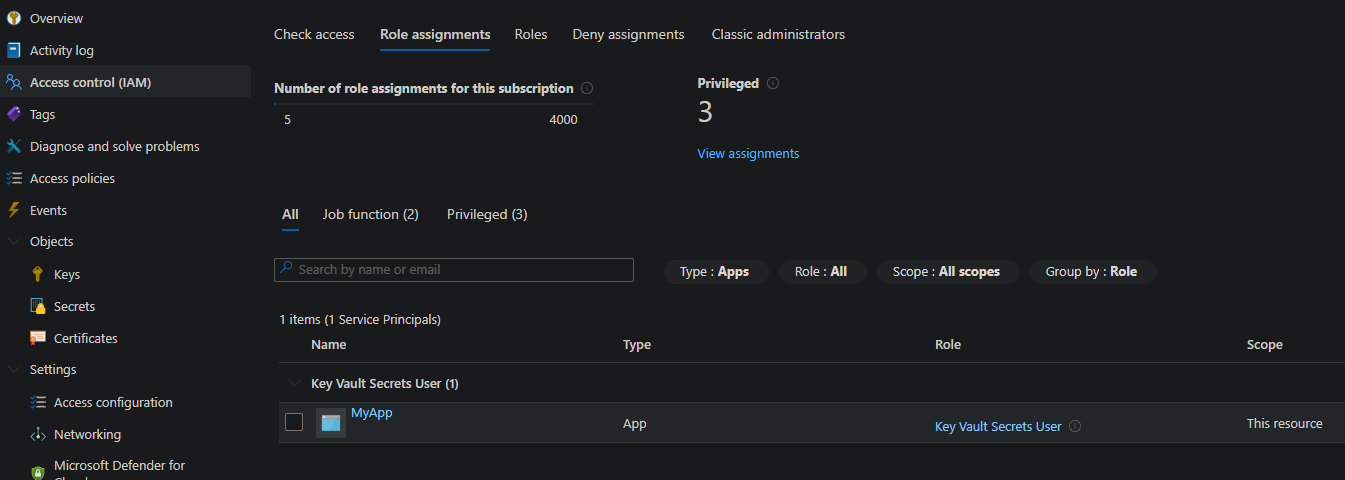
No, we'll not be using AppService
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
We're not certain yet but we'll not be using Azure for everything
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I think we want to be able to use multiple hosting services
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Our app may run in an on-prem environment so we can't always rely on Azure
i.e. none of our config / secrets will be loaded via Azure
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yea
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Yeah ok
I think I get the basics of what you're saying
I'm going to have to do a lot of reading on the fundamentals
This is all new to me as you can clearly tell
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I'll take note of everything you've mentioned here
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Unfortunately I can't comprehend everything but I generally get what you're warning me about
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
I really appreciate the help but unfortuantely it's going above my head due to my lack of experience xD
I'll keep at it, thank you so much for your help again tonight
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
xD
It's Tuesday 12:15AM for me
Oh by the way, given the fact that you're extremely knowledgeable even on the fundamentals, do you have a blog or some knowledge base I can checkout?
Or a YouTube channel or anything?
Just curious xD
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Ah alright
I've got to sleep but thank you so much again!
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Will do, I'll keep it up for now and write down everything later
@TeBeClone You here by any chance?
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
Aight, hope everything's going alright for ya
If you get a chance and would be open to answering a couple more questions, please let me know. Thanks again ❤️
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View