Discord.net help - Trying to access a users roles
Does anybody know a method I can use to access a users discord roles whilst I am logging into my application so I can either allow them into the app or disallow them?
If you have any guides on how I could do this or some examples that would give some ideas on how I can do this as currently my method is not working.
Here is how it looks.
Just looking for more ideas about this approach. My brain is starting to run out of them.
Tia
58 Replies
what exactly doesn't work? The code you show is a valid option for getting user roles
Let me run it and grab the error. I'll just be a second
This appears when I hit the break point here however it shows there is a count of 10 roles. This makes it return as false
Its like it can't see the roles?

Thats the count. Its just not showing me what 10 roles there are.

π so what does your logging output? seeing as you loop through the roles collection before that
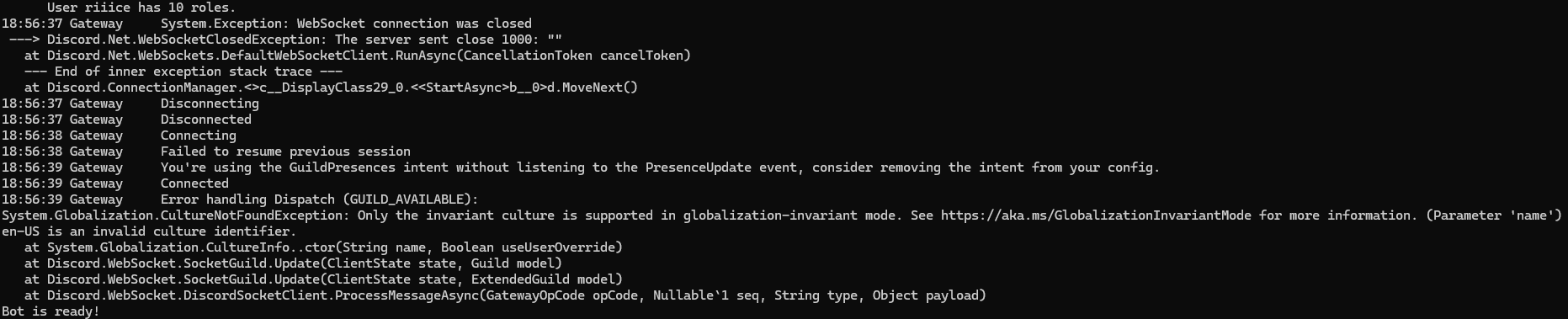
Doesn't seem to get the role and Id
uh...which part of those logs is related to this section of code?
The 10 roles at the top it seems
I don't see log in ur code snippet
oh
sorry, i see
so if you debug (use the debugger) does it just skip the
foreach
Yeah its skipping this whole section here

π
Yeah I am totally lost. I wonder if you can't do Oauth but I would be suprised if thats the case
nothing in this snippet is related to OAuth though... you have a
DiscordSocketClient
assuming that's what _botService.Client
returns... and that fact that you successfully get a guild and a user should mean the client is already logged in and readyYeah its logged in and ready but if you don't have a specified role I want it to (at some point) send you to another page
so it just sends the user to that
But currently its not even capable of extracting the data from whatever the role info is stored in
Got the socket setup here
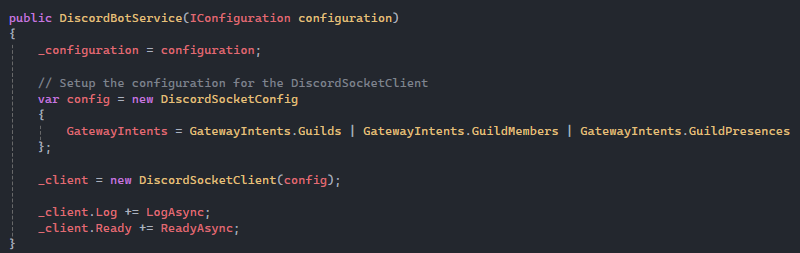
what async work does this method actually do?
Literally this
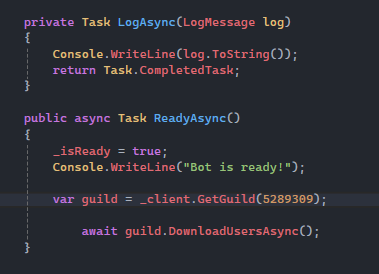
no, i mean the
IsUserInServerWithRole
why is it returning a Task<bool>
is there other code that you left out?
cause these no awaits in your snippitJust this
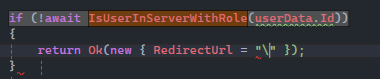
π€
inside of the method, now how u call the method
^ in this
its called in my HTTP Post
So when I make a post to do the exchange its called within that
I don't think u get what i'm asking
I think I might be confused
this method
private async Task<bool> IsUserInServerWithRole
has async Task<bool>
yet it does not do any async work
why is it not just private bool IsUserInServerWithRole
Let me try it
Got an error about "Get Awaiter"?
where?
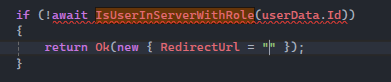
remove await
still this issue :cry_cat: inside of my Roles
Also skips the actual check for the role :/
which check?

This one^
does it skip the
foreach
itself or just the if
?Just the if. It goes to the user.Roles then the in then back out
also are you actually using the debugger or just relying on the logging?
I am using the debugger
It shoes the count of roles so in this case = 21. But then it wont match the id with the ids
so it knows ids are there
just not able to access
that doesn't make sense though... if you have a collection with items, it should simply iterate through that collection
Thats what i thought but when I try to access the collection I get
Empty = "Enumeration yielded no results"
maybe unnecessary, im unsure what intents are privileged and what arent, but have you verified whatever youre doing doesnt require opting in within the Discord developer dashboard?
I dont think it makes sense since you are allegedly getting the 10 roles and can see them in the debugger, but might be worth playing around with? π€·ββοΈ
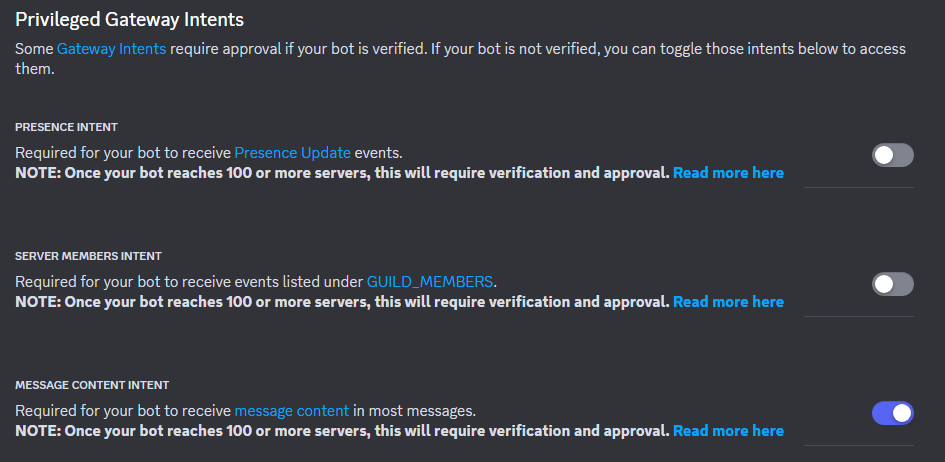
I have all of them checked π¦
Actually, not this one. Unsure fully what it does

if you add that before you logger line and put a break point on the logger. Does the array have anything?
Empty = "Enumeration yielded no results"
Still has the count thoug
screenshot
its not letting me, just comes off when I go to screenshot
In visualiser I get this
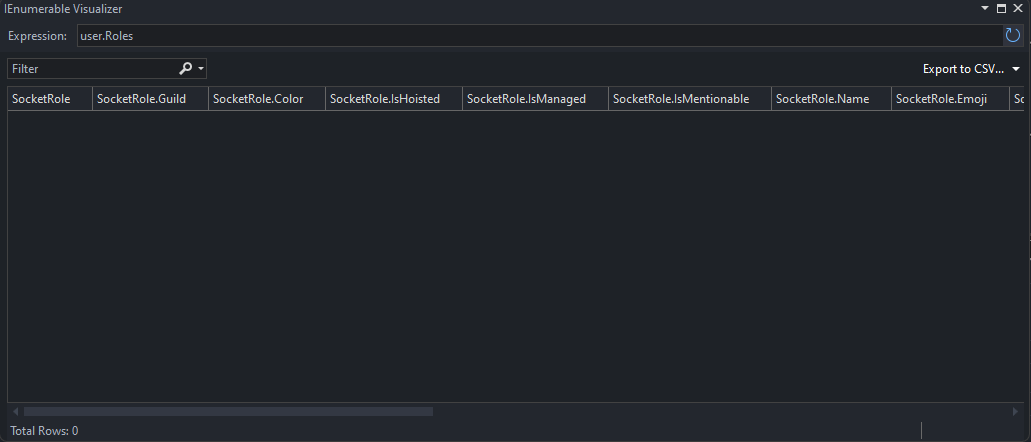
yeah, idk... this is weird. As far as I can see the code is correct
I've been stuck on and off for about 2 months
I am about to give up and go to js at this point :CryingCool:

There you go
thats whats popping up
not the user.Roles.... the array
var roles = user.Roles.ToArray();
what does roles
show
πΆββοΈ
gg
:cowboy_cool_cry_mild_panic: Yep, its really not looking good
do you have the code sitting anywhere public? I can try taking a quick stab at it locally
I don't no currently its filled with personal info and stuff of that matter π¦
I would be happy to go into a call and go through what I have so you can see where I am and what its doing?
No promises, but sure.