Put and Delete Methods not working.
Program.cs:
using InventoryPro.Database;
using Microsoft.EntityFrameworkCore;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddDbContext<InventoryDbContext>(options =>
options.UseInMemoryDatabase("InventoryDatabase"));
var app = builder.Build();
app.Urls.Add("http://localhost:5555");
app.UseHttpsRedirection();
http:
@WebApiHost = http://localhost:5555
GET {{WebApiHost}}/
###
GET {{WebApiHost}}/items
###
POST {{WebApiHost}}/items
Content-Type: application/json
{
"Name" : "Example item 3",
"Description": "Description 3",
"Price": 25.50,
"Quantity": 30
}
GET {{WebApiHost}}/items/1
###
PUT {{WebApiHost}}/items
Content-Type: application/json
{
"itemId" : 1,
"Name" : "Example item 1",
"Description": "Description 1",
"Price": 25.50,
"Quantity": 30
}
###
DELETE {{WebApiHost}}/items/1
using (var scope = app.Services.CreateScope())
{
var dbContext = scope.ServiceProvider.GetRequiredService<InventoryDbContext>();
if (!dbContext.Items.Any())
{
dbContext.Items.AddRange(
new Item { Name = "Example Item 1", Description = "Description 1", Price = 10, Quantity = 100 },
new Item { Name = "Example Item 2", Description = "Description 2", Price = 20, Quantity = 50 }
);
dbContext.SaveChanges();
}
}
app.MapGet("/items", async (InventoryDbContext dbContext) =>
await dbContext.Items.ToListAsync());
//app.MapGet("/items/{id}", async (int id, InventoryDbContext dbContext) =>
// await dbContext.Items.FindAsync(id) ?? Results.NotFound());
app.MapPost("/items", async (Item newItem, InventoryDbContext dbContext) =>
{
dbContext.Items.Add(newItem);
await dbContext.SaveChangesAsync();
return Results.Created($"/items/{newItem.ItemId}", newItem);
});
app.MapPut("/items/{itemId}", async (int itemId, Item updatedItem, InventoryDbContext dbContext) =>
{
var item = await dbContext.Items.FindAsync(itemId);
if (item == null) return Results.NotFound();
item.Name = updatedItem.Name;
item.Description = updatedItem.Description;
item.Price = updatedItem.Price;
item.Quantity = updatedItem.Quantity;
await dbContext.SaveChangesAsync();
return Results.NoContent();
});
app.MapDelete("/items/{id}", async (int id, InventoryDbContext dbContext) =>
{
var item = await dbContext.Items.FindAsync(id);
if (item == null) return Results.NotFound();
dbContext.Items.Remove(item);
await dbContext.SaveChangesAsync();
return Results.NoContent();
});
app.Run();
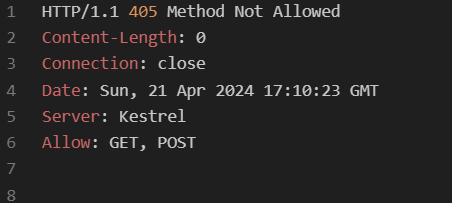
98 Replies
which request did you execute exactly to get that response
also $codegif
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/Put and delete
and what url
PUT {{WebApiHost}}/items

this is not the same
you're missing itemId in the url
do
PUT {{WebApiHost}}/items/1
for examplealright let me try
same issue
i think i had that on delete already too
its the same error for both i think
are you familiar with curl?
im not
i have a feeling you're not executing the request you think you are
hmmm ok, so what does that mean?
run this in terminal
see what you get
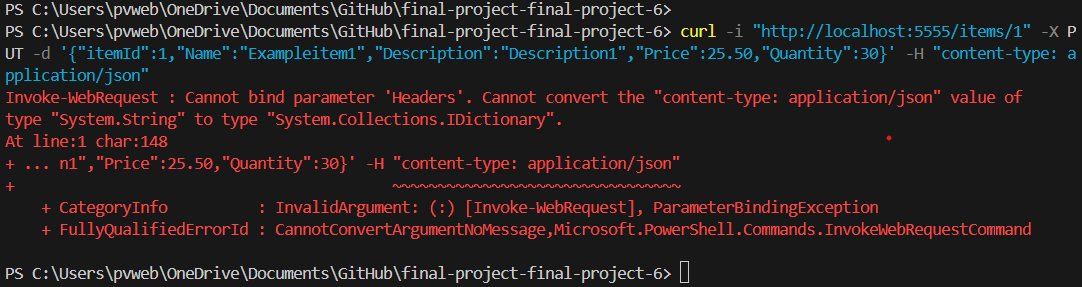
aa š¬
š„ŗ
or if you are familiar with postman/some other tool..
No but i think i changed my localhost let me retry someting
nvm same error
with this ?
run this in terminal?
yes
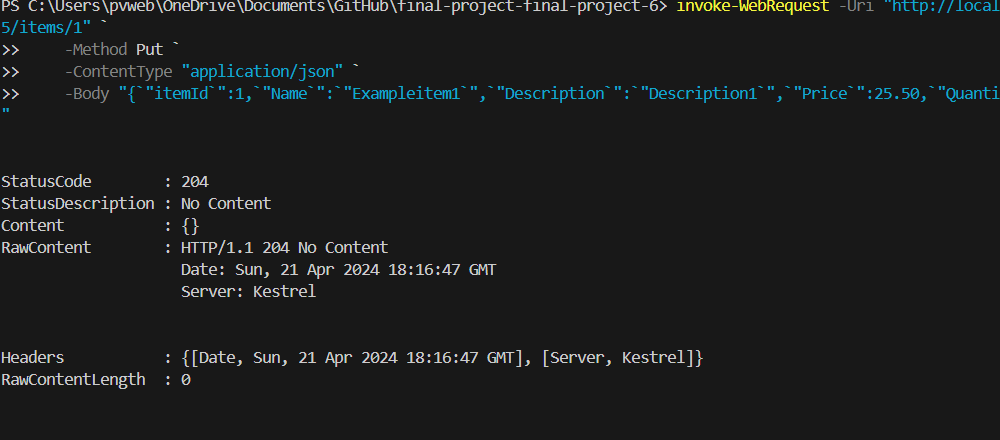
š
so the issue is with how you are executing the requests
which editor/tool are you using to do it
vs? rider?
visual studio code
REST Client - Visual Studio Marketplace
Extension for Visual Studio Code - REST Client for Visual Studio Code
yeah
paste full .http file you use -> $code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/
my put message is not found
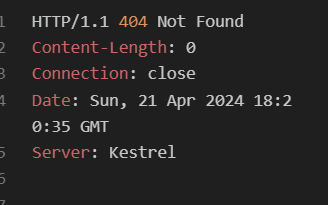
from this?
Whenever I send a new request
like I was doing before
not from that
ok thats another issue
ok
you click here to run that put request?
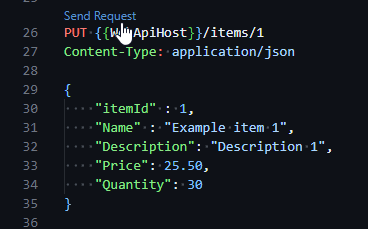
yes!
strange it works fine for me
oh wait
try restart vscode š
now its happening on the first get too
ok 1 sec
still happening
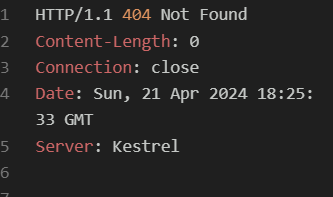
this from first get
second get works
ok but let's fix method not allowed issue first
kk
you still get method not allowed on put?
204 no content now
ok that means its fixed
and delete?
same thing
ok
which endpoint is 404 not found
//app.MapGet("/items/{id}", async (int id, InventoryDbContext dbContext) =>
// await dbContext.Items.FindAsync(id) ?? Results.NotFound());
this?
I think so?
well do you have item in the database that you try to get?
do post and then try get
also... dont ever use in memory with ef core š¬
is that going to be an issue?
yes read warning https://learn.microsoft.com/en-us/ef/core/providers/in-memory/?tabs=dotnet-core-cli
In-memory Database Provider - EF Core
Information on the Entity Framework Core in-memory database provider
it even says on the side

its for a school project, It won be for production
add Id = something.. because probably in memory doesn't automatically assign Id
oh really ok one sec
i mean even for development... its more pain in the ass than worth. it's really easy to use something like sqlite provider even
Same issue
what did you set Id to? š
1 and 2
and what is your get request
GET {{WebApiHost}}/items/1
?
Method not allowed
405
wtf...
š
I really appreciate you trying to help me btw
try this
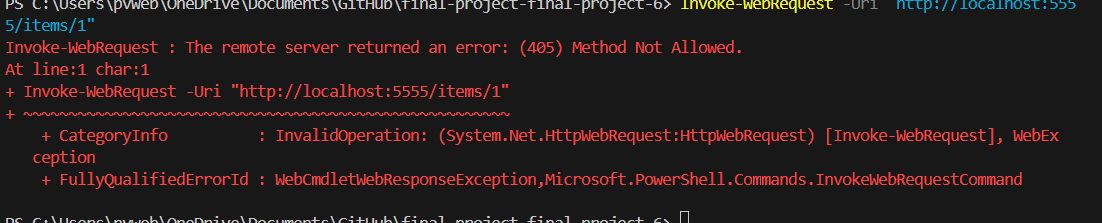
can you post your program.cs again in $code pls
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/but.. its commented š
no wonder it doesnt work
Operator '??' cannot be applied to operands of type 'Item' and 'IResult'CS0019
If i uncomment it
ok 2 things
dont use FindAsync.. use FirstOrDefaultAsync(x => x.Id == id)
and then do something like
if you can see the argument for FindAsync is..
object
. its not type safe. it's extra easy to shoot yourself in the foot and send something you dont intendapp.MapGet("/items/{id}", async (int id, InventoryDbContext dbContext)=> {
item = await dbContext.Items.FirstOrDefaultAsync(x => x.Id == id)
if (item is null)
return Results.NotFound()
return Results.Ok(item) } like this?
return Results.Ok(item) } like this?
yes
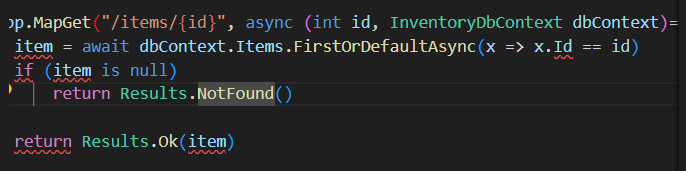
;
? š
also instead x.Id neets to be x.ItemId
per your model
hover with mouse it should hint you errors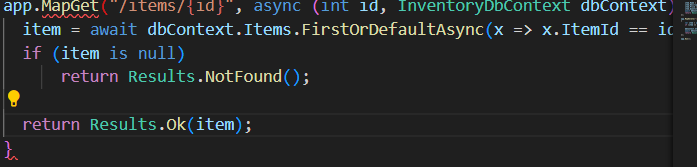
whats the error it says
mapget takes 3 arguments
can you copy or full screenshot of this
its cut off
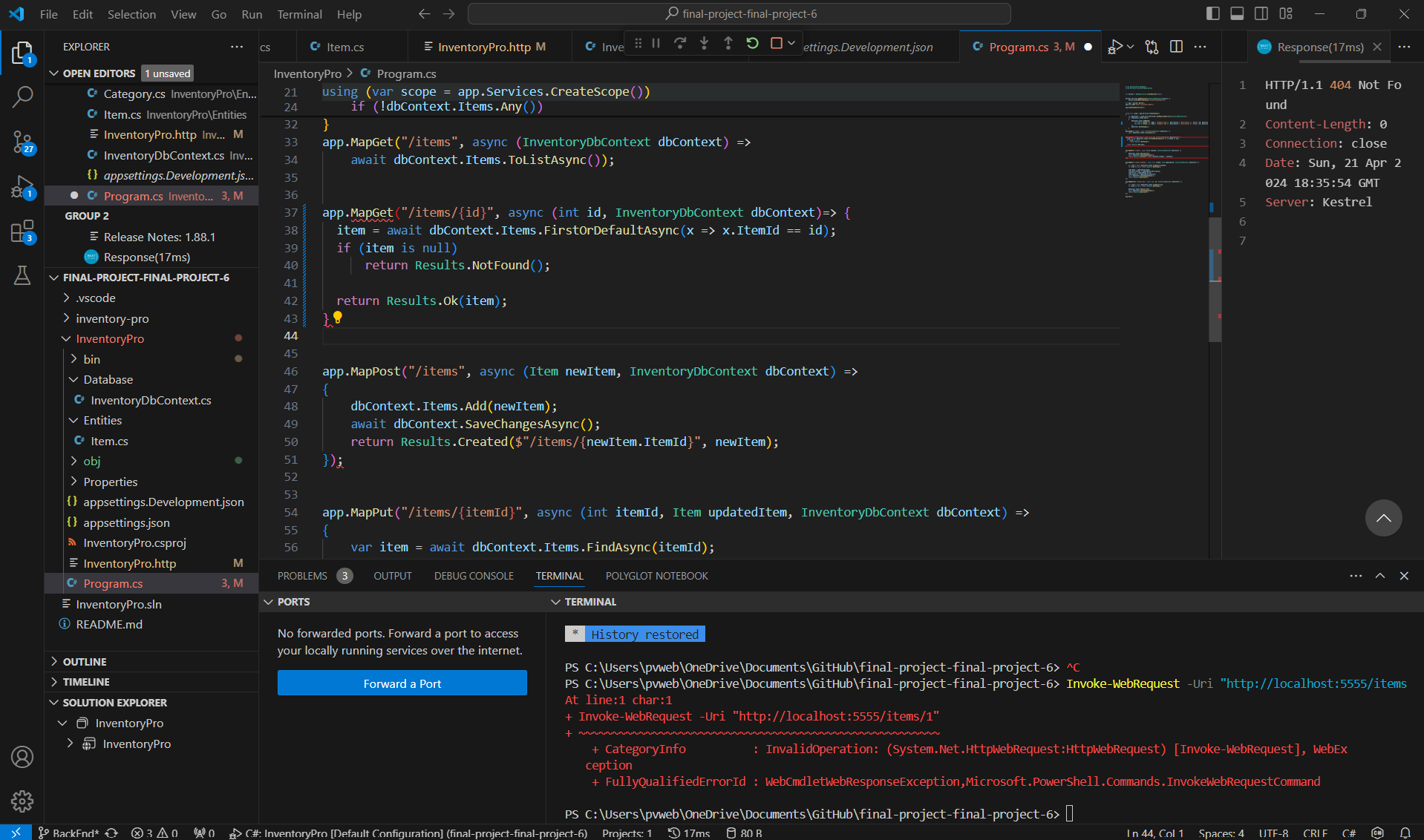
you are missing ) at the end
look at MapPost below
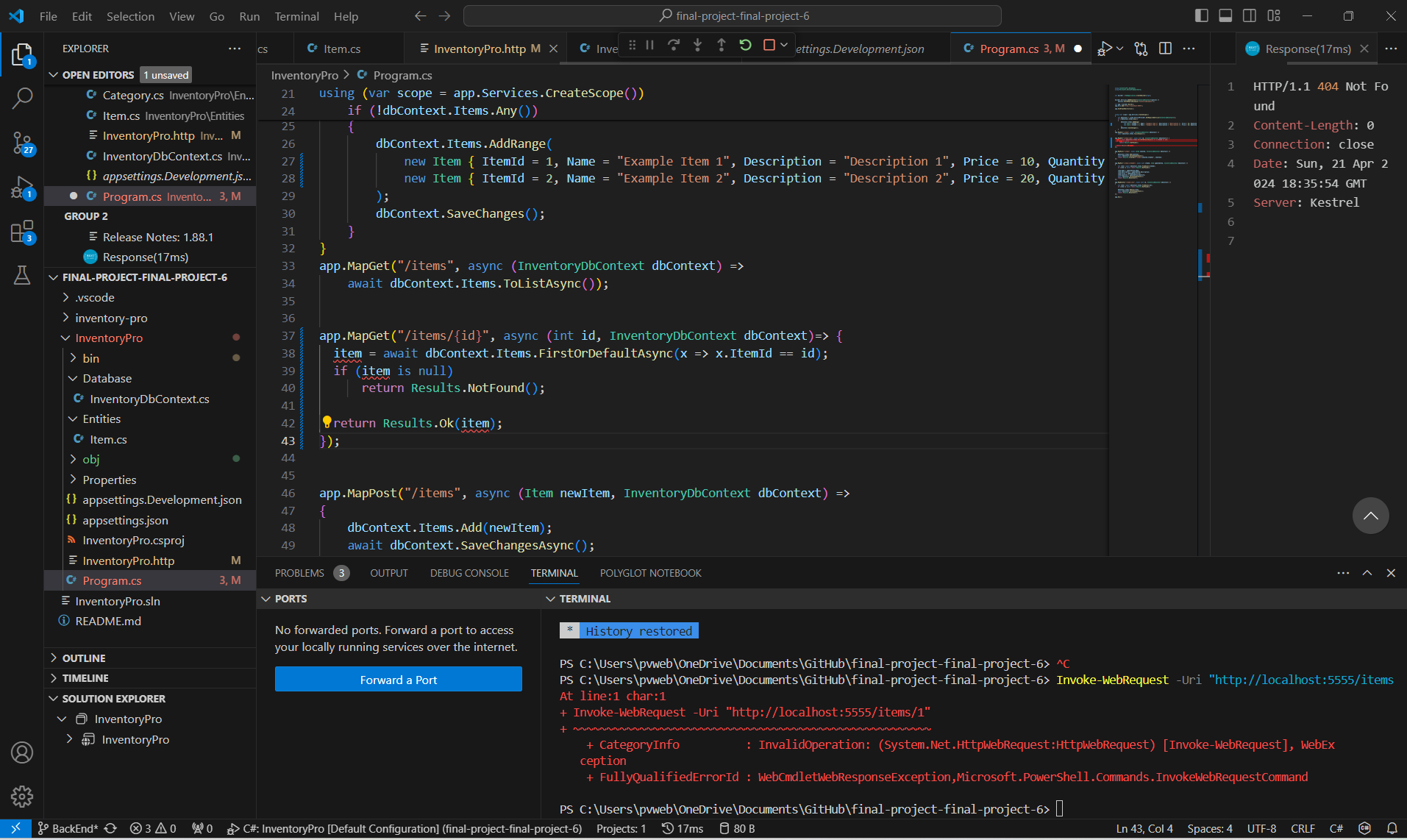
Item does not exist in current context
š
well duh. you didnt declare it anywhere
var item =...
ok yay! it works
now we are back at 204 not found
on put and delete
204 no content ssorry
but?? thats OK
did you write this code yourself or..?
no it was a team member, they were having trouble debugging it
you return NoContent here.. which is 204
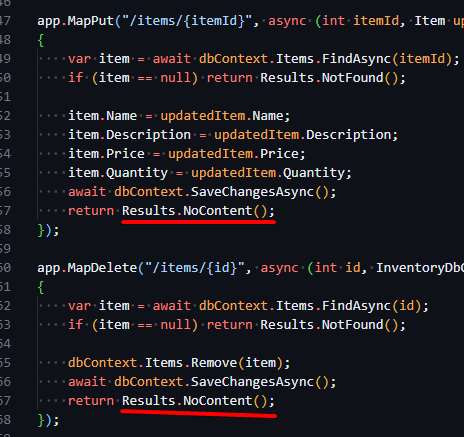
so that means it executed all the way
ahhh so its working gotcha!
sorry lol
yes
š
What was the root issue (simplified lol)
well for method not allowed.. i dont know. you werent executing the requests right.. since the commands you ran in terminal worked ok
i dont use this vscode extension so idk if there are some gotchas.. or something to be aware of
i recommend you learn to use some tool like postman/insomnia/other...
it might help usually
compare what we changed... š it should tell you what were the issues
ā¤ļø