error shows that No command matching ban was found.
ban.js:
interactionCreate:
const { ActionRowBuilder, ButtonBuilder, ButtonStyle, SlashCommandBuilder } = require('discord.js');
module.exports = {
data: new SlashCommandBuilder()
.setName('ban')
.setDescription('Bans a user from the server.')
.addUserOption(option => option
.setName('target')
.setDescription('The user to ban')
.setRequired(true)
)
.addStringOption(option => option
.setName('reason')
.setDescription('The reason for the ban')
.setRequired(false)
),
async execute(interaction) {
const target = interaction.options.getUser('target');
const reason = interaction.options.getString('reason') ?? 'No reason provided';
const confirm = new ButtonBuilder()
.setCustomId('confirm')
.setLabel('Confirm Ban')
.setStyle(ButtonStyle.Danger);
const cancel = new ButtonBuilder()
.setCustomId('cancel')
.setLabel('Cancel')
.setStyle(ButtonStyle.Secondary);
const row = new ActionRowBuilder()
.addComponents(cancel, confirm);
await interaction.reply({
content: `Are you sure you want to ban ${target} for reason: ${reason}?`,
components: [row],
});
},
};
const { ActionRowBuilder, ButtonBuilder, ButtonStyle, SlashCommandBuilder } = require('discord.js');
module.exports = {
data: new SlashCommandBuilder()
.setName('ban')
.setDescription('Bans a user from the server.')
.addUserOption(option => option
.setName('target')
.setDescription('The user to ban')
.setRequired(true)
)
.addStringOption(option => option
.setName('reason')
.setDescription('The reason for the ban')
.setRequired(false)
),
async execute(interaction) {
const target = interaction.options.getUser('target');
const reason = interaction.options.getString('reason') ?? 'No reason provided';
const confirm = new ButtonBuilder()
.setCustomId('confirm')
.setLabel('Confirm Ban')
.setStyle(ButtonStyle.Danger);
const cancel = new ButtonBuilder()
.setCustomId('cancel')
.setLabel('Cancel')
.setStyle(ButtonStyle.Secondary);
const row = new ActionRowBuilder()
.addComponents(cancel, confirm);
await interaction.reply({
content: `Are you sure you want to ban ${target} for reason: ${reason}?`,
components: [row],
});
},
};
const { Events } = require("discord.js");
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (interaction.isChatInputCommand()) {
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(
`No command matching ${interaction.commandName} was found.`
);
return;
}
try {
await command.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.commandName}`);
console.error(error);
}
}
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
} else if (interaction.isStringSelectMenu()) {
const selectMenu = interaction.client.selectMenus.get(
interaction.customId
);
if (!selectMenu) {
console.error(
`No select menu matching ${interaction.customId} was found.`
);
return;
}
try {
await selectMenu.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.customId}`);
console.error(error);
}
}
},
};
const { Events } = require("discord.js");
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (interaction.isChatInputCommand()) {
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(
`No command matching ${interaction.commandName} was found.`
);
return;
}
try {
await command.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.commandName}`);
console.error(error);
}
}
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
} else if (interaction.isStringSelectMenu()) {
const selectMenu = interaction.client.selectMenus.get(
interaction.customId
);
if (!selectMenu) {
console.error(
`No select menu matching ${interaction.customId} was found.`
);
return;
}
try {
await selectMenu.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.customId}`);
console.error(error);
}
}
},
};
37 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!command handler?
i made the buttons work a long time ago but now i forgot
u mean where did i load the commands from?
the code where you fill client.commands
oh idk i just followed that discord.js guide tbh
alr
i have Commands for prefix commands
ohhh ok that makes sense
let me fix it rq and ill tell u if it worked
now the console is showing this
node:events:497
throw er; // Unhandled 'error' event
^
TypeError: Cannot read properties of undefined (reading 'get')
at Object.execute (/home/jorrell/Desktop/Xen/Src/Events/Creates/interactionCreate.js:7:56)
const fs = require('fs');
const path = require('path');
const { Client, GatewayIntentBits, Partials, Collection } = require('discord.js');
const { Token } = require('../Config/bot_settings.js');
const chalk = require('chalk');
const db = require('../Database/connect.js');
const client = new Client({
intents: [
GatewayIntentBits.Guilds,
GatewayIntentBits.GuildMessages,
GatewayIntentBits.DirectMessages,
GatewayIntentBits.MessageContent
],
partials: [
Partials.Channel,
Partials.Message,
Partials.User
]
});
console.log(chalk.yellow.underline(`[CORE] Initalizing Xen. . .`));
client.database = {};
client.commands = new Collection();
const foldersPath = path.join(__dirname, '../Commands');
const commandFolders = fs.readdirSync(foldersPath);
for (const folder of commandFolders) {
const commandsPath = path.join(foldersPath, folder);
const commandFiles = fs.readdirSync(commandsPath).filter(file => file.endsWith('.js'));
for (const file of commandFiles) {
const filePath = path.join(commandsPath, file);
const command = require(filePath);
if ('structure' in command && 'execute' in command) {
client.commands.set(command.structure.name, command);
} else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "structure" or "execute" property.`));
}
}
}
client.SlashCommands = new Collection();
const slashCommandsPath = path.join(__dirname, '../Slashcommands');
const slashCommandFolders = fs.readdirSync(slashCommandsPath);
for (const folder of slashCommandFolders) {
const commandPath = path.join(slashCommandsPath, folder);
const slashCommandFiles = fs.readdirSync(commandPath).filter(file => file.endsWith('.js'));
for (const file of slashCommandFiles) {
const filePath = path.join(commandPath, file);
const slashCommand = require(filePath);
if ('data' in slashCommand && 'execute' in slashCommand) {
client.SlashCommands.set(slashCommand.data.name, slashCommand);
}
else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "data" or "execute" property.`));
}
}
}
const eventsPath = path.join(__dirname, '../Events');
const eventFolders = fs.readdirSync(eventsPath);
for (const folder of eventFolders) {
const folderPath = path.join(eventsPath, folder);
const eventFiles = fs.readdirSync(folderPath).filter(file => file.endsWith('.js'));
for (const file of eventFiles) {
const filePath = path.join(folderPath, file);
const event = require(filePath);
if (event.once) {
client.once(event.name, (...args) => event.execute(...args));
} else {
client.on(event.name, (...args) => event.execute(...args));
}
}
}
client.login(Token);
db().then((databaseClient) => {
client.database = databaseClient.database;
console.log(chalk.green('[DATABASE] Database connected and ready.'));
}).catch((error) => {
console.error('Error connecting to the database:', error);
});
const fs = require('fs');
const path = require('path');
const { Client, GatewayIntentBits, Partials, Collection } = require('discord.js');
const { Token } = require('../Config/bot_settings.js');
const chalk = require('chalk');
const db = require('../Database/connect.js');
const client = new Client({
intents: [
GatewayIntentBits.Guilds,
GatewayIntentBits.GuildMessages,
GatewayIntentBits.DirectMessages,
GatewayIntentBits.MessageContent
],
partials: [
Partials.Channel,
Partials.Message,
Partials.User
]
});
console.log(chalk.yellow.underline(`[CORE] Initalizing Xen. . .`));
client.database = {};
client.commands = new Collection();
const foldersPath = path.join(__dirname, '../Commands');
const commandFolders = fs.readdirSync(foldersPath);
for (const folder of commandFolders) {
const commandsPath = path.join(foldersPath, folder);
const commandFiles = fs.readdirSync(commandsPath).filter(file => file.endsWith('.js'));
for (const file of commandFiles) {
const filePath = path.join(commandsPath, file);
const command = require(filePath);
if ('structure' in command && 'execute' in command) {
client.commands.set(command.structure.name, command);
} else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "structure" or "execute" property.`));
}
}
}
client.SlashCommands = new Collection();
const slashCommandsPath = path.join(__dirname, '../Slashcommands');
const slashCommandFolders = fs.readdirSync(slashCommandsPath);
for (const folder of slashCommandFolders) {
const commandPath = path.join(slashCommandsPath, folder);
const slashCommandFiles = fs.readdirSync(commandPath).filter(file => file.endsWith('.js'));
for (const file of slashCommandFiles) {
const filePath = path.join(commandPath, file);
const slashCommand = require(filePath);
if ('data' in slashCommand && 'execute' in slashCommand) {
client.SlashCommands.set(slashCommand.data.name, slashCommand);
}
else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "data" or "execute" property.`));
}
}
}
const eventsPath = path.join(__dirname, '../Events');
const eventFolders = fs.readdirSync(eventsPath);
for (const folder of eventFolders) {
const folderPath = path.join(eventsPath, folder);
const eventFiles = fs.readdirSync(folderPath).filter(file => file.endsWith('.js'));
for (const file of eventFiles) {
const filePath = path.join(folderPath, file);
const event = require(filePath);
if (event.once) {
client.once(event.name, (...args) => event.execute(...args));
} else {
client.on(event.name, (...args) => event.execute(...args));
}
}
}
client.login(Token);
db().then((databaseClient) => {
client.database = databaseClient.database;
console.log(chalk.green('[DATABASE] Database connected and ready.'));
}).catch((error) => {
console.error('Error connecting to the database:', error);
});
interaction.client.commands.get
you still have to change it to SlashCommands herealr
like that?
alr
not sure why its still giving that error
i did
wow im slow lmao
const command = interaction.client.slashcommands.get(interaction.commandName);
const command = interaction.client.slashcommands.get(interaction.commandName);
node:events:497
throw er; // Unhandled 'error' event
^
TypeError: Cannot read properties of undefined (reading 'get')
at Object.execute (/home/jorrell/Desktop/Xen/Src/Events/Creates/interactionCreate.js:7:56)
node:events:497
throw er; // Unhandled 'error' event
^
TypeError: Cannot read properties of undefined (reading 'get')
at Object.execute (/home/jorrell/Desktop/Xen/Src/Events/Creates/interactionCreate.js:7:56)
const { Events } = require("discord.js");
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (interaction.isChatInputCommand()) {
const command = interaction.client.Slashcommands.get(interaction.commandName);
if (!command) {
console.error(
`No command matching ${interaction.commandName} was found.`
);
return;
}
try {
await command.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.commandName}`);
console.error(error);
}
}
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
} else if (interaction.isStringSelectMenu()) {
// respond to the select men0u
const selectMenu = interaction.client.selectMenus.get(
interaction.customId
);
if (!selectMenu) {
console.error(
`No select menu matching ${interaction.customId} was found.`
);
return;
}
try {
await selectMenu.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.customId}`);
console.error(error);
}
}
},
};
const { Events } = require("discord.js");
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (interaction.isChatInputCommand()) {
const command = interaction.client.Slashcommands.get(interaction.commandName);
if (!command) {
console.error(
`No command matching ${interaction.commandName} was found.`
);
return;
}
try {
await command.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.commandName}`);
console.error(error);
}
}
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
} else if (interaction.isStringSelectMenu()) {
// respond to the select men0u
const selectMenu = interaction.client.selectMenus.get(
interaction.customId
);
if (!selectMenu) {
console.error(
`No select menu matching ${interaction.customId} was found.`
);
return;
}
try {
await selectMenu.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.customId}`);
console.error(error);
}
}
},
};
it works appreciate yalls help frfr
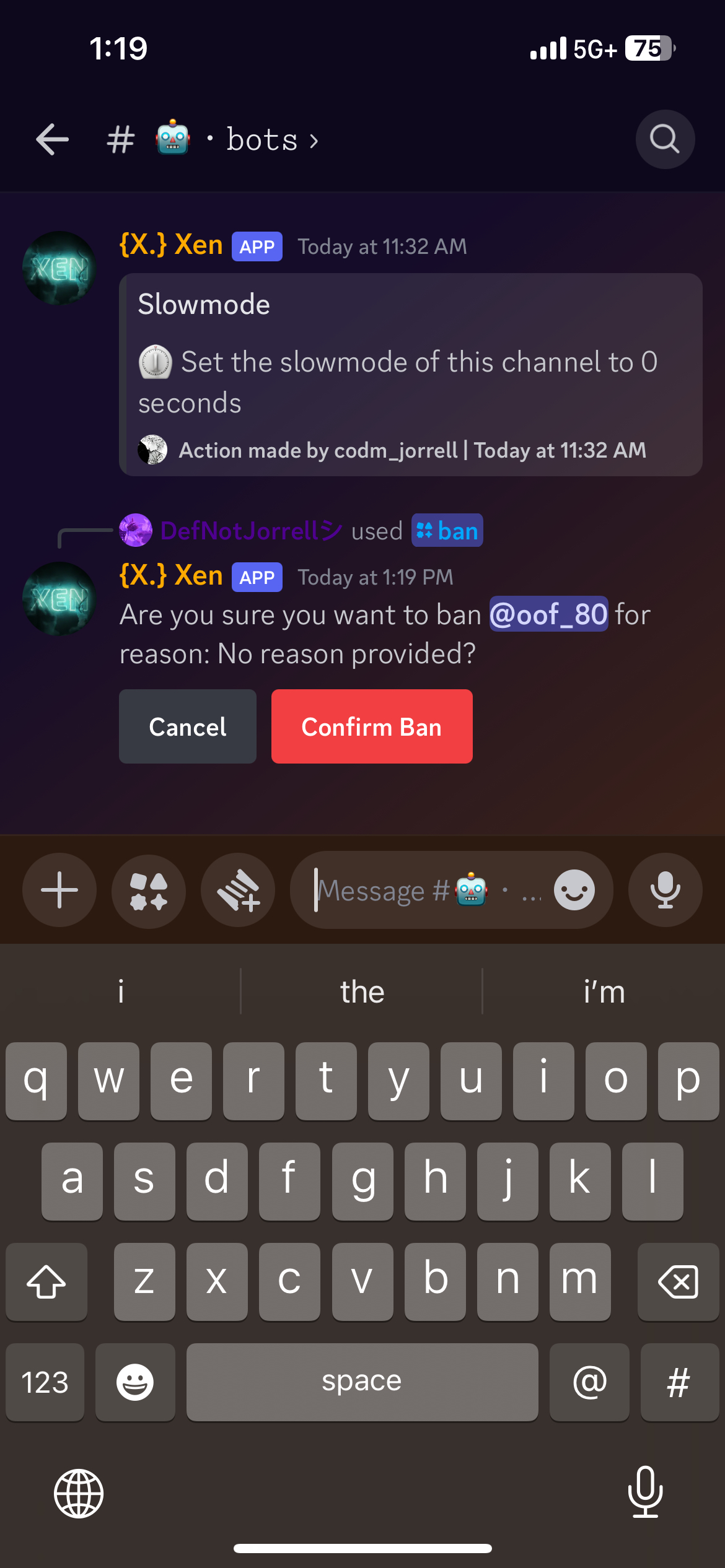
well then again
after i click the button it says this
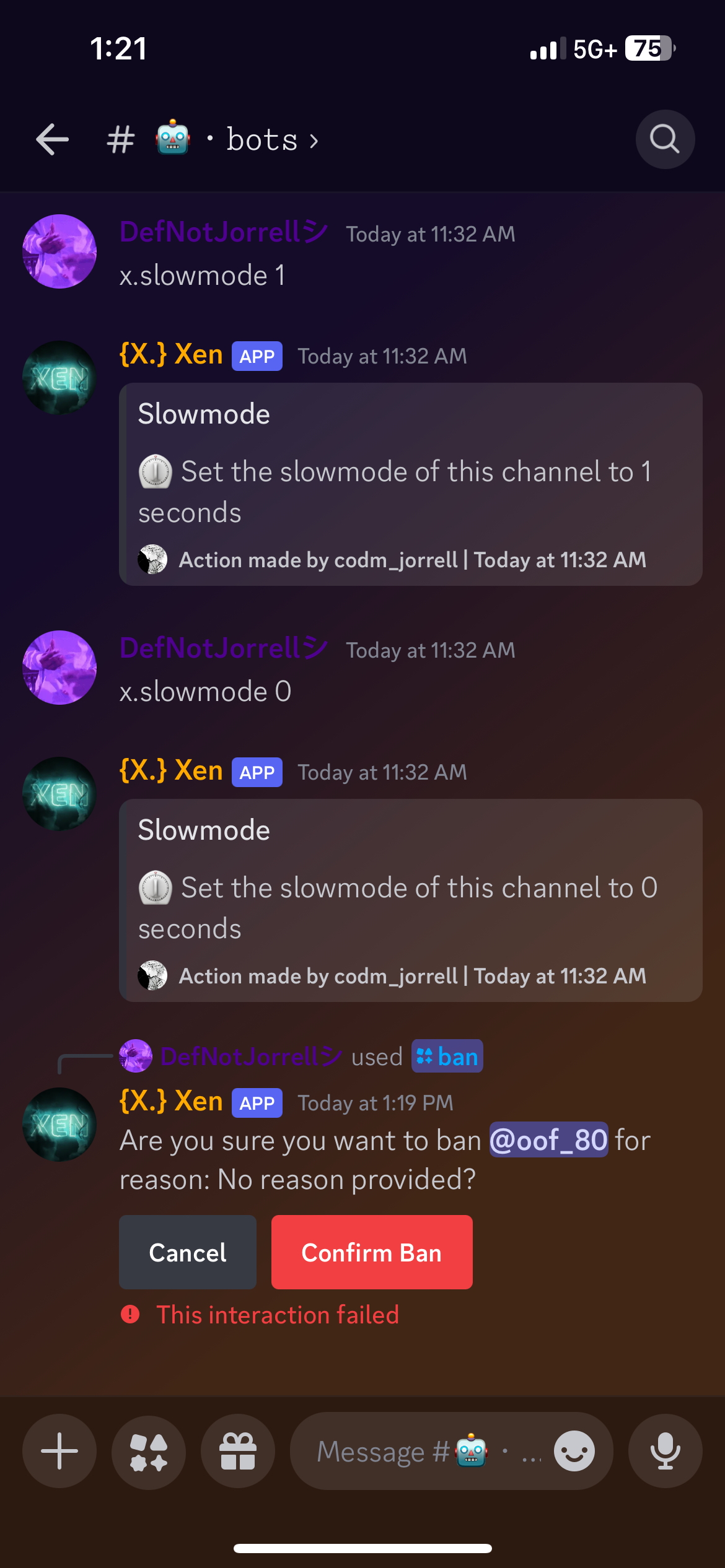
in console?
what happens in console
same as before
node:events:497
throw er; // Unhandled 'error' event
^
TypeError: Cannot read properties of undefined (reading 'get')
node:events:497
throw er; // Unhandled 'error' event
^
TypeError: Cannot read properties of undefined (reading 'get')
i don't think you load buttons
in index.js
oh
wait am i supposed to load buttons in index.js?
possibly?
ima try it rq
i added client.buttons = new Collection into index.js and the console says this now
soo make a path to Events/Creates/interactionCreate.js then?
or make a new folder for buttons
tbh ima use collectors for the buttons seems easier that way anyway tbh
so collectors worked but the console still shows that same error
No button matching cancel was found.
No button matching cancel was found.
const fs = require('fs');
const path = require('path');
const { Client, GatewayIntentBits, Partials, Collection } = require('discord.js');
const { Token } = require('../Config/bot_settings.js');
const chalk = require('chalk');
const db = require('../Database/connect.js');
const client = new Client({
intents: [
GatewayIntentBits.Guilds,
GatewayIntentBits.GuildMessages,
GatewayIntentBits.DirectMessages,
GatewayIntentBits.MessageContent
],
partials: [
Partials.Channel,
Partials.Message,
Partials.User
]
});
console.log(chalk.yellow.underline(`[CORE] Initalizing Xen. . .`));
client.database = {};
client.commands = new Collection();
const foldersPath = path.join(__dirname, '../Commands');
const commandFolders = fs.readdirSync(foldersPath);
for (const folder of commandFolders) {
const commandsPath = path.join(foldersPath, folder);
const commandFiles = fs.readdirSync(commandsPath).filter(file => file.endsWith('.js'));
for (const file of commandFiles) {
const filePath = path.join(commandsPath, file);
const command = require(filePath);
if ('structure' in command && 'execute' in command) {
client.commands.set(command.structure.name, command);
} else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "structure" or "execute" property.`));
}
}
}
client.SlashCommands = new Collection();
const slashCommandsPath = path.join(__dirname, '../Slashcommands');
const slashCommandFolders = fs.readdirSync(slashCommandsPath);
for (const folder of slashCommandFolders) {
const commandPath = path.join(slashCommandsPath, folder);
const slashCommandFiles = fs.readdirSync(commandPath).filter(file => file.endsWith('.js'));
for (const file of slashCommandFiles) {
const filePath = path.join(commandPath, file);
const slashCommand = require(filePath);
if ('data' in slashCommand && 'execute' in slashCommand) {
client.SlashCommands.set(slashCommand.data.name, slashCommand);
}
else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "data" or "execute" property.`));
}
}
}
client.buttons = new Collection();
const eventsPath = path.join(__dirname, '../Events');
const eventFolders = fs.readdirSync(eventsPath);
for (const folder of eventFolders) {
const folderPath = path.join(eventsPath, folder);
const eventFiles = fs.readdirSync(folderPath).filter(file => file.endsWith('.js'));
for (const file of eventFiles) {
const filePath = path.join(folderPath, file);
const event = require(filePath);
if (event.once) {
client.once(event.name, (...args) => event.execute(...args));
} else {
client.on(event.name, (...args) => event.execute(...args));
}
}
}
client.login(Token);
db().then((databaseClient) => {
client.database = databaseClient.database;
console.log(chalk.green('[DATABASE] Database connected and ready.'));
}).catch((error) => {
console.error('Error connecting to the database:', error);
});
const fs = require('fs');
const path = require('path');
const { Client, GatewayIntentBits, Partials, Collection } = require('discord.js');
const { Token } = require('../Config/bot_settings.js');
const chalk = require('chalk');
const db = require('../Database/connect.js');
const client = new Client({
intents: [
GatewayIntentBits.Guilds,
GatewayIntentBits.GuildMessages,
GatewayIntentBits.DirectMessages,
GatewayIntentBits.MessageContent
],
partials: [
Partials.Channel,
Partials.Message,
Partials.User
]
});
console.log(chalk.yellow.underline(`[CORE] Initalizing Xen. . .`));
client.database = {};
client.commands = new Collection();
const foldersPath = path.join(__dirname, '../Commands');
const commandFolders = fs.readdirSync(foldersPath);
for (const folder of commandFolders) {
const commandsPath = path.join(foldersPath, folder);
const commandFiles = fs.readdirSync(commandsPath).filter(file => file.endsWith('.js'));
for (const file of commandFiles) {
const filePath = path.join(commandsPath, file);
const command = require(filePath);
if ('structure' in command && 'execute' in command) {
client.commands.set(command.structure.name, command);
} else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "structure" or "execute" property.`));
}
}
}
client.SlashCommands = new Collection();
const slashCommandsPath = path.join(__dirname, '../Slashcommands');
const slashCommandFolders = fs.readdirSync(slashCommandsPath);
for (const folder of slashCommandFolders) {
const commandPath = path.join(slashCommandsPath, folder);
const slashCommandFiles = fs.readdirSync(commandPath).filter(file => file.endsWith('.js'));
for (const file of slashCommandFiles) {
const filePath = path.join(commandPath, file);
const slashCommand = require(filePath);
if ('data' in slashCommand && 'execute' in slashCommand) {
client.SlashCommands.set(slashCommand.data.name, slashCommand);
}
else {
console.log(chalk.underline.red(`[WARNING] The command at ${filePath} is missing a required "data" or "execute" property.`));
}
}
}
client.buttons = new Collection();
const eventsPath = path.join(__dirname, '../Events');
const eventFolders = fs.readdirSync(eventsPath);
for (const folder of eventFolders) {
const folderPath = path.join(eventsPath, folder);
const eventFiles = fs.readdirSync(folderPath).filter(file => file.endsWith('.js'));
for (const file of eventFiles) {
const filePath = path.join(folderPath, file);
const event = require(filePath);
if (event.once) {
client.once(event.name, (...args) => event.execute(...args));
} else {
client.on(event.name, (...args) => event.execute(...args));
}
}
}
client.login(Token);
db().then((databaseClient) => {
client.database = databaseClient.database;
console.log(chalk.green('[DATABASE] Database connected and ready.'));
}).catch((error) => {
console.error('Error connecting to the database:', error);
});
node:events:497
throw er; // Unhandled 'error' event
^
TypeError: Cannot read properties of undefined (reading 'get')
at Object.execute (/home/jorrell/Desktop/Xen/Src/Events/Creates/interactionCreate.js:24:49)
node:events:497
throw er; // Unhandled 'error' event
^
TypeError: Cannot read properties of undefined (reading 'get')
at Object.execute (/home/jorrell/Desktop/Xen/Src/Events/Creates/interactionCreate.js:24:49)
const { Events } = require("discord.js");
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (interaction.isChatInputCommand()) {
const command = interaction.client.SlashCommands.get(interaction.commandName);
if (!command) {
console.error(
`No command matching ${interaction.commandName} was found.`
);
return;
}
try {
await command.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.commandName}`);
console.error(error);
}
}
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
} else if (interaction.isStringSelectMenu()) {
// respond to the select men0u
const selectMenu = interaction.client.selectMenus.get(
interaction.customId
);
if (!selectMenu) {
console.error(
`No select menu matching ${interaction.customId} was found.`
);
return;
}
try {
await selectMenu.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.customId}`);
console.error(error);
}
}
},
};
const { Events } = require("discord.js");
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (interaction.isChatInputCommand()) {
const command = interaction.client.SlashCommands.get(interaction.commandName);
if (!command) {
console.error(
`No command matching ${interaction.commandName} was found.`
);
return;
}
try {
await command.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.commandName}`);
console.error(error);
}
}
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
} else if (interaction.isStringSelectMenu()) {
// respond to the select men0u
const selectMenu = interaction.client.selectMenus.get(
interaction.customId
);
if (!selectMenu) {
console.error(
`No select menu matching ${interaction.customId} was found.`
);
return;
}
try {
await selectMenu.execute(interaction);
} catch (error) {
console.error(`Error executing ${interaction.customId}`);
console.error(error);
}
}
},
};
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
yea
it is
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
oh
so add this in my index.js?
client.buttons = new Collection();
const buttonsPath = path.join(__dirname, '../Buttons');
const buttonFolders = fs.readdirSync(buttonsPath);
for (const folder of buttonFolders) {
const folderPath = path.join(buttonsPath, folder);
const buttonFiles = fs.readdirSync(folderPath).filter(file => file.endsWith('.js'));
for (const file of buttonFiles) {
const filePath = path.join(folderPath, file);
const button = require(filePath);
if ('data' in button && 'execute' in button) {
client.buttons.set(button.data.customId, button);
}
}
}
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
alr
node:fs:1508
const result = binding.readdir(
^
Error: ENOENT: no such file or directory, scandir '/home/jorrell/Desktop/Xen/Src/Buttons'
at Object.readdirSync (node:fs:1508:26)
at Object.<anonymous> (/home/jorrell/Desktop/Xen/Src/Core/index.js:70:26)
thats what came from the console
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
this is what happend when i used the collectors
so basically remove
from interactionCreate?
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
if (interaction.isButton()) {
const button = interaction.client.buttons.get(interaction.customId);
if (!button) {
console.error(`No button matching ${interaction.customId} was found.`);
return;
}
try {
await button.execute(interaction);
} catch (error) {
console.error(
`Error executing button interaction with customId: ${interaction.customId}`
);
console.error(error);
}
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
ill remove it and run it again to see what happens
ok it works with no errors
:spincat:
so ima just use collectors when im using buttons and also can collectors be used for modals select menus etc?
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
oh ok
thanks for the help
can u use collectors with modals?🤔
:method: ChatInputCommandInteraction#awaitModalSubmit()
Collects a single modal submit interaction that passes the filter. The Promise will reject if the time expires.
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
ok
when i type something in the modal and press submit nothing happens
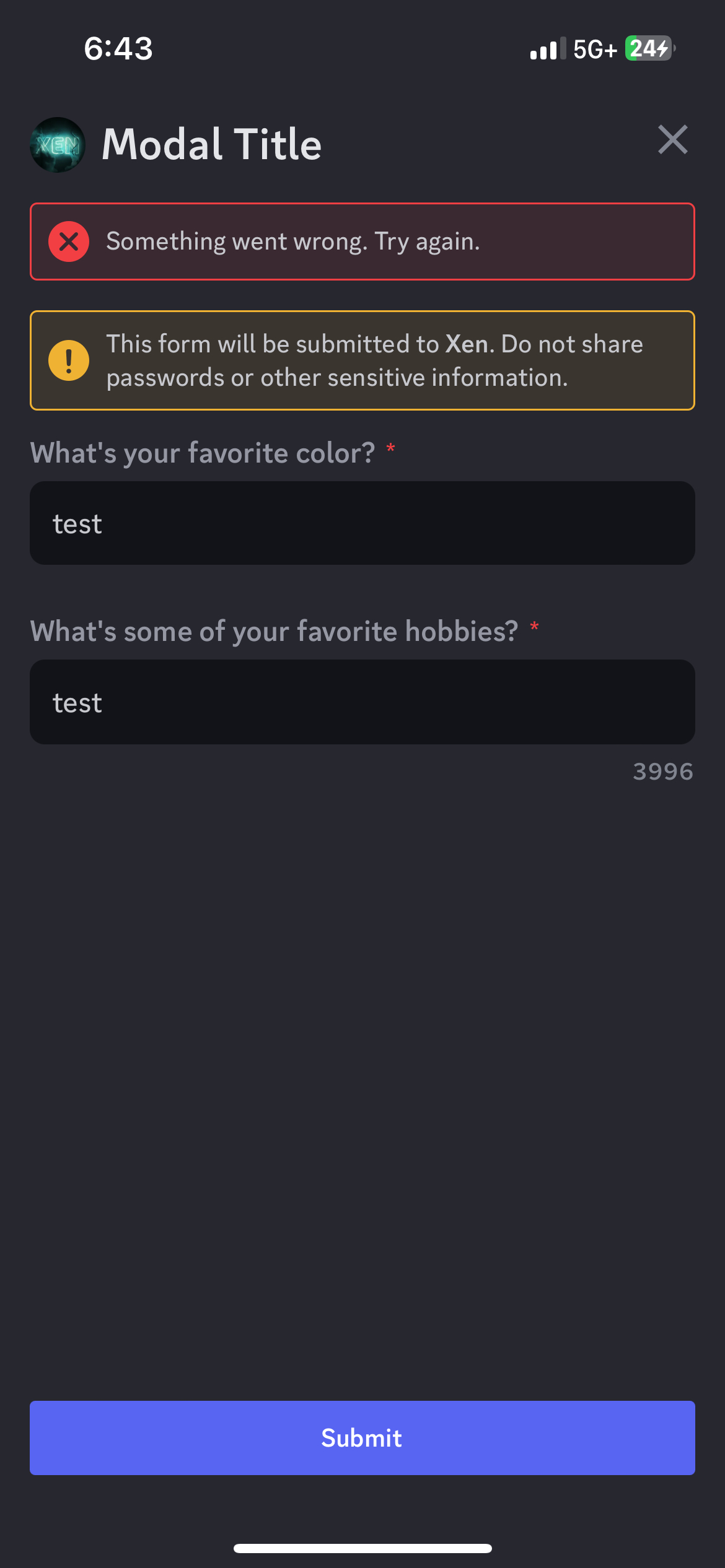
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
const { SlashCommandBuilder, ActionRowBuilder, ModalBuilder, TextInputBuilder, TextInputStyle, ModalSubmitFields } = require('discord.js');
module.exports = {
data: new SlashCommandBuilder()
.setName('modal')
.setDescription('Shows a modal'),
async execute(interaction) {
try {
const modal = new ModalBuilder()
.setCustomId('modal')
.setTitle('Modal Title');
const favoriteColorInput = new TextInputBuilder()
.setCustomId('favoriteColorInput')
.setLabel("What's your favorite color?")
.setStyle(TextInputStyle.Short)
.setRequired(true);
const hobbiesInput = new TextInputBuilder()
.setCustomId('hobbiesInput')
.setLabel("What's some of your favorite hobbies?")
.setStyle(TextInputStyle.Paragraph)
.setRequired(true);
const firstActionRow = new ActionRowBuilder().addComponents(favoriteColorInput);
const secondActionRow = new ActionRowBuilder().addComponents(hobbiesInput);
modal.addComponents(firstActionRow, secondActionRow);
await interaction.showModal(modal);
await interaction.submitModal(modal)
}
catch (error) {
console.log(error)
}
}
}
const { SlashCommandBuilder, ActionRowBuilder, ModalBuilder, TextInputBuilder, TextInputStyle, ModalSubmitFields } = require('discord.js');
module.exports = {
data: new SlashCommandBuilder()
.setName('modal')
.setDescription('Shows a modal'),
async execute(interaction) {
try {
const modal = new ModalBuilder()
.setCustomId('modal')
.setTitle('Modal Title');
const favoriteColorInput = new TextInputBuilder()
.setCustomId('favoriteColorInput')
.setLabel("What's your favorite color?")
.setStyle(TextInputStyle.Short)
.setRequired(true);
const hobbiesInput = new TextInputBuilder()
.setCustomId('hobbiesInput')
.setLabel("What's some of your favorite hobbies?")
.setStyle(TextInputStyle.Paragraph)
.setRequired(true);
const firstActionRow = new ActionRowBuilder().addComponents(favoriteColorInput);
const secondActionRow = new ActionRowBuilder().addComponents(hobbiesInput);
modal.addComponents(firstActionRow, secondActionRow);
await interaction.showModal(modal);
await interaction.submitModal(modal)
}
catch (error) {
console.log(error)
}
}
}
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
alr