Reaction collector not working
Reaction collector does not seem to work for me , i am expecting to be able to collect reactions on a message or embed.
Here is the slash command i created
and the results i get in console after reacting:
User xxxx used the /ping command.
Collected 0 items. Reason: time
I have verified permissions on the developer site: Permissions are: application commands, bot, manage messages, send messages, read messages. I have many other slash commands and functions working.
I have tried this with an embed and regular message. I have tried to listen for any reaction, as i thought it could be the type of thumbs up.
Looking at the discord guide this should work:
https://discordjs.guide/popular-topics/collectors.html#await-reactions
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
13 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!I’m seeing a message component collector in your code, but not a reaction collector
Documentation suggestion for @maccrackalackin:
:class: ReactionCollector (extends Collector)
Collects reactions on messages. Will automatically stop if the message (Client#event:messageDelete messageDelete or Client#event:messageDeleteBulk messageDeleteBulk), channel (Client#event:channelDelete channelDelete), thread (Client#event:threadDelete threadDelete), or guild (Client#event:guildDelete guildDelete) is deleted.
thankyou, i will review this and see if can get it to work
I have worked through this i think, but i am getting this still after i react : User xxx used the /ping command.
Collected 0 reactions. Reason: time
here is the adjusted code with a reaction collector:
does anyone have an insight into why the collector is not working?
Do you have the GuildMessageReactions intent?
yes it is inside my client constructor, i believe that should be correct:
I would try changing
:thumbsup:
in your collector filter to the unicode value instead 👍
I also think you might need the GuildMessages intent & Reaction partialI have made the code simpler by taking out the specific reaction, and included any:
I have given the bot Adminstrator permissions and application.command + bot command scope for testing.
Added some extra console logs for testing. Add and removed the bot again :
These are the console results after reacting to the message.
User XXXX used the /ping command.
Reaction collector started.
Collected 0 reactions. Reason: time
What i do notice is the 'reaction' inside the collector is declared but never used. so i am not sure if that filter/collector is correct
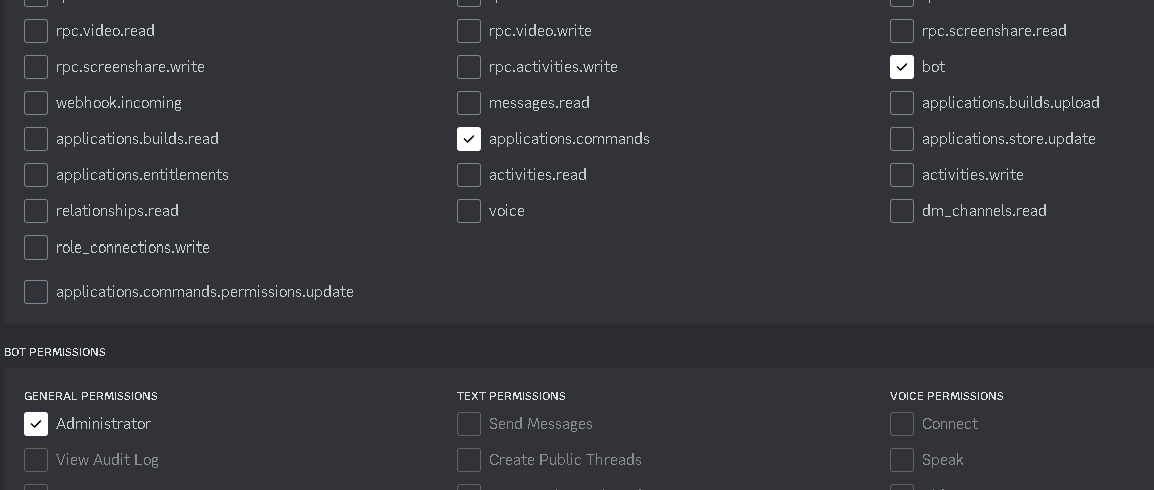
Unknown User•13mo ago
Message Not Public
Sign In & Join Server To View
thanks for the input, i apprecciate it it alot, i changed the filter with the same results however. I did have the bot scope, i just didnt have administrator untill recently to make sure i had no permission issues. I have other slash commands working perfectly with a lot of functionality, its just a simple react collector that doesnt seem to work.
Unknown User•13mo ago
Message Not Public
Sign In & Join Server To View
Documentation suggestion for @maccrackalackin:
:event: (event) Collector#ignore
Emitted whenever an element is not collected by the collector.
Thats a good idea. i tried the ignore event before and after the end event to see if it made a difference , Alas it did not, I think i coded it correctly but it did not seem to write to console anything:
User xxx used the /ping command.
Reaction collector started.
Collected 0 reactions. Reason: time
is there a working example of the reaction collector i can test, or if someone can test this code to see if it works. Otherwise i am not sure how else to troubleshoot this