excel modification
https://pastecode.io/s/ndzcjm6s
can i get some tips how can i improve my code
i am a complete beginner in c#
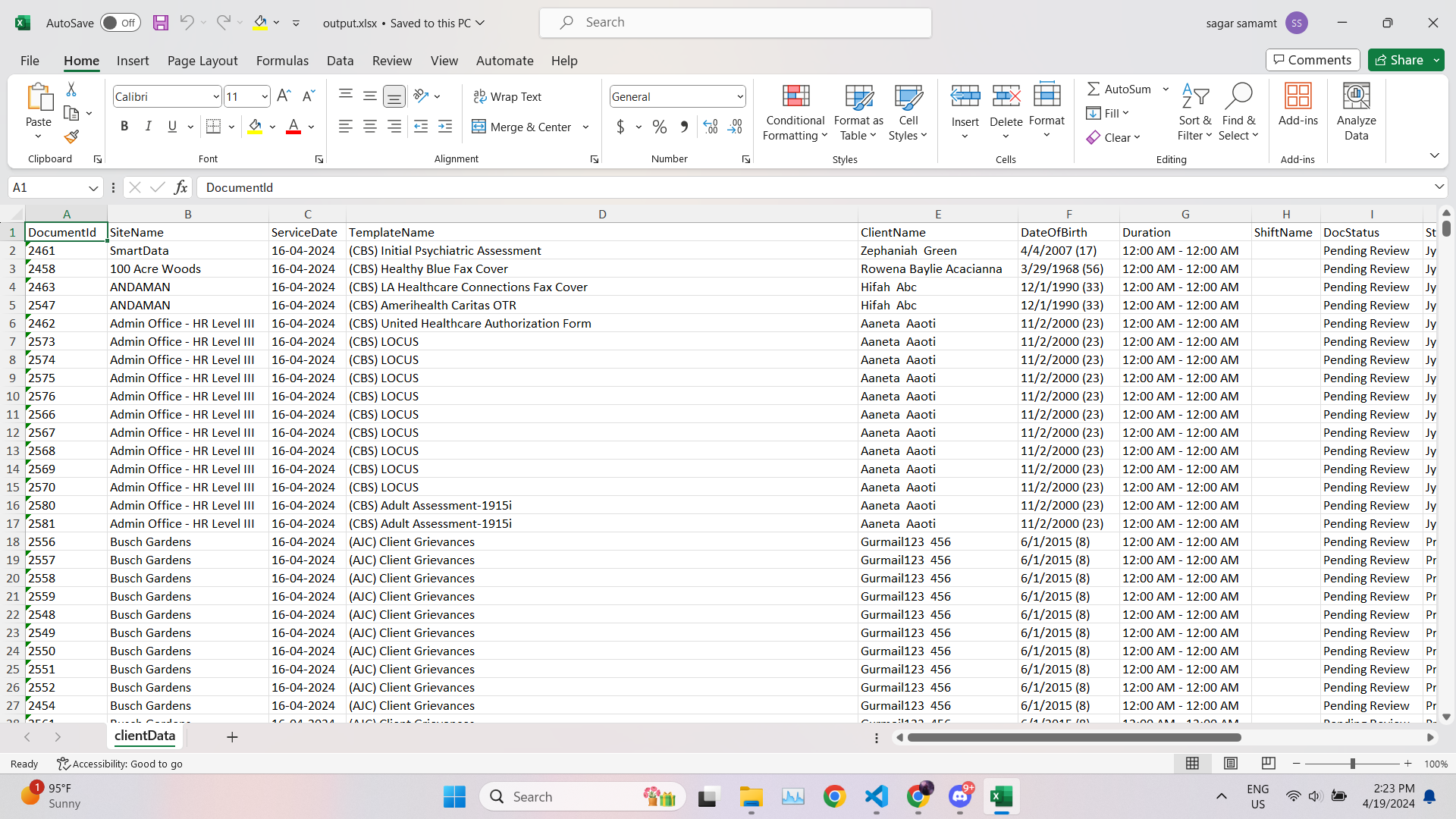
59 Replies
What code are you trying to improve? I just see an excel sheet
Edit: I see you added a pastebin link to the post now 👍
code looks fine already
You don't need the
JsonPropertyName
attributes if the property name matches the json name.
You have a lot of nullable properties. I would make these required
if they are, well, required in the JSON. Only leave them nullable if the property is optional.
You can use this syntax to avoid having to nest using:
using var workbook = new XLWorkbook();
oooh I see you are using the properties for headers. Mmmmm
I'd have to ponder that. I don't think relying on property order is a great strategy, but I don't know of a better one offhand.I guess I would use the DisplayAttribute for that instead. https://learn.microsoft.com/en-us/dotnet/api/system.componentmodel.dataannotations.displayattribute?view=net-8.0
DisplayAttribute Class (System.ComponentModel.DataAnnotations)
Provides a general-purpose attribute that lets you specify localizable strings for types and members of entity partial classes.
so all this code here:
Could be probably handled better with another property instead.
in fact you might consider moving all the properties to a DTO object and then having another object that wraps it that fomats the data for display.
This is called the decorator pattern.
since you aren't doing anything fancy with the exceptions other than logging and exiting, you might simply move all the code out of main into a seperate function, and then handle the catch there.
https://pastecode.io/s/m4n86vem
how about this one guys since i reduced lots of condition statement
i was trying to name them in first letter capital
that why i definaed json property to give them new name
Yeah, you don't need to bother with that if you set the
PropertyNameCaseInsensitive
property, which you did.
But actually I think that is the default, so you don't even need to bother with that.https://pastecode.io/s/d3nfzmk1
json data
is this good enough as beginner
I mean if it does what you want, sure. The only major thing I would point out is the nullable type issue.
so how should i ressolve it
required?
mark properties that should not be null as
required
stj will then throw if the property is not presentshould not be null or that is not null in my json data?
if it is required it should not be nullable
like documentId
values that should always have a value in your json data should probably all be marked required
even struct ones
this sill cause STJ to throw if they are not present during deserialization.
one of the problem is i am deleting some colums like firsta nd second name
so, is the composite name present in your json?
what do u mean
if are talking about clientfirst and last name them yes they r present in json data
no I mean properties like
clientName
no
i added them
I would then consider doing a two-object approach like I said.
clientname and duration
a JSON based DTO object
and another object that presents the data formated.
which wraps the DTO object
can u give me example
any example
something like...
This gives you a deterministic order as well.
DocumentDTO should be matching with json data keys like in my json its documentId:10
if you set that property, stj will figure it out.
PropertyNameCaseInsensitive will turn off this one
it works just fine
thanks
how can i access display names
[Display(Name = "Document Id", Order = 0)]
reflection
what
something like...
you would probably want to hydrate this together with the property object though.
let me send u my code in few min
Then you would access them via like...
this look lethal
lethal bad? Good? Your using reflection either way here.
Adding the order property just makes order deterministic.
this too good for me even if i understand ill just use properties let me send u
because i have given this work as a beginner and they probably think i copied from somewhere
good
can i iterate through DocumentDisplay
foreach(var entry in dtoList.Select(dto => new DocumentDisplay(dto)) {
foreach(var prop in propertyNameArr.Select(element => element.Property)) {
var propValue = prop.GetValue(entry);
// set propValue into cell
}
}
not like this something more simpler
in this case i have to use .
sure. You used reflection earlier, so I gave a reflection based solution
can we just iterate through it
well, that's what I showed.
The issue with the code you had earlier is that iterating properties in the way you were is not (AFAIK) deterministic.
That is, the order the properties are enumerated is not guranteed to be the same.
The way to avoid this is to either A. Order them by a deterministic attribute, such as order, which I showed above.
B. Manually order them.
https://pastecode.io/s/0iraj2qk
i am very proud of code since i am not even using display name that you told me
but it does helped me solve addind and removing unnecessary columns
but its too hasrd for me to get
can i remove Display since i am using it?
looks fine, though you could have
ServiceRate
just be a decimal
and avoid parsing it.i was thinking if i can just get all the values through iteration but this is just too much for me understanf rn
this is very similar to what you are doing, but just pulls values from a property attribute as well. I can go over what it's doing if you like.
Unhandled exception. System.Text.Json.JsonException: The JSON value could not be converted to System.Decimal. Path: $[0].serviceRate | LineNumber: 49 | BytePositionInLine: 23.
public decimal ServiceRate { get; set; }
if i did that i am getting error
sure if i can understand
ahh, looks like ServiceRate is sometimes null in your data. Unfortunate.
yeah it is
You might try making it nullable. But I'm not 100% on how STJ will handle that with a struct type.
that's why i was using it as string
let me check
using ?
yeah.
Works.
but nullable struct types have some different properties
iil just use string
explain
how familiar are you with linq?
noob
just started c# yesterday
mmm.... might be to much to explain then
what is this for btw?
nah its fine i'll just use this
my friend introduce me to some company
they were looking for backend and ui dev
i am new in c# so they gave me this json data and told me to convert into excel
with my basic knowledge i somehow managed to make it i am just now improving