How to implement code-level caching service?
How to use caching on code-level? I need to cache the
getAuthUser(userId)
function result on code level to avoid querying D1 again and again as the getAuthUser is a middleware used on all the API routes.
So, I built a get/set caching function using a fake url, user/1, user/2 etc. But it doesn't seems to be working and I see undefined
in cache.match(cacheKey)
response always.
3 Replies
What does cache.put return?
And you're not showing how the two funcs are implemented which might be relevant
This is how I am using this cache function.
The
cache.put() : Promise<void>
is a void function, so it returns undefined
Ok, I get it working. So issue resolved, but It doesn't expire.
Even when I set
s-maxage=30
header, the match return the value after 30s as well.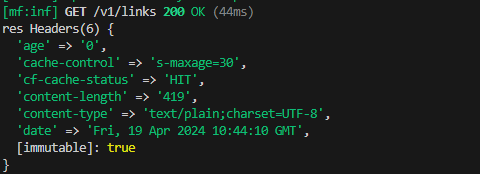