Hey all, I am trying to make curl calls
Hey all, I am trying to make curl calls using PHP and want to start the session using the login API, however, I am getting status code 204 in the response and it seems to be a successful call, I checked the response headers and it contains JSESSIONID and clienId but session cookies are not getting set for the user whose credentials I have shared in the request body.
Later I will use it to display the embedded iframe using the JavaScript API library by initializing the session using "window.thoughtspot.initialize".
Is this process in the correct path?
Any suggestions or fixes are appreciated.
Thanks,
7 Replies
Please use the Thoughtspot Embed SDK, it would make your life easy https://developers.thoughtspot.com/docs/tsembed
Embed ThoughtSpot
Getting Started
I have already embedded and used SDK in the React app, and everything was working fine with that, but now the requirements have changed and we want to implement it in PHP.
Any help will be appreciated.
The SDK is pure javascript, so it should work fine with PHP as well. You would need to add script tags in the output markup and do everything in there
Can you provide some sample php which you are working and I can try and suggest an approach
$url = 'https://<host>/api/rest/2.0/auth/session/login';
$data = array(
'remember_me' => 'true',
'username' => 'username',
'password' => 'password',
'org_identifier' => '0'
);
$data = json_encode($data);
$headers = array(
'User-Agent: ---',
'X-Requested-By: ThoughtSpot',
'Authorization: token',
'Content-Type: application/json'
);
$api_request = array(
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => $data,
CURLOPT_HTTPHEADER => $headers,
CURLOPT_HEADER => true,
CURLOPT_VERBOSE => true,
);
$ch = curl_init();
curl_setopt_array($ch, $api_request);
$api_response = curl_exec($ch);
$http_status = curl_getinfo($ch, CURLINFO_HTTP_CODE);
$header_size = curl_getinfo($ch, CURLINFO_HEADER_SIZE);
$response_headers = substr($api_response, 0, $header_size);
echo "Response Headers:\n$response_headers\n";
if ($http_status == 204) {
echo "API request successful. HTTP Status Code: $http_status";
} else {
echo "API request failed. HTTP Status Code: $http_status";
}
curl_close($ch);
If you have some samples using JavaScript, can you please share it with me?
Additional information:
I have noticed that automatic cookies are not getting set after a successful call to the thought spot login API.
So we have tried setting cookies manually using,
setcookie('JSESSIONID', '7b61523a-49d3-4d73-a1a1-7a44409ed2da', strtotime('2024-04-23T10:21:59.556Z'), '/', 'q1media.thoughtspot.cloud', true, true);
But it's not working, we have tried removing the domain from the cookie and it's getting set but for the localhost domain, not the thoughtspot.
@ashish do you have any updates for me ? I shared a sample with you.
The link I shared above has the javascript code samples of using the SDK. You already know how to use the JS SDK. You can just use php to emit JS code. Something like https://www.geeksforgeeks.org/how-to-run-javascript-from-php/
GeeksforGeeks
How to run JavaScript from PHP? - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
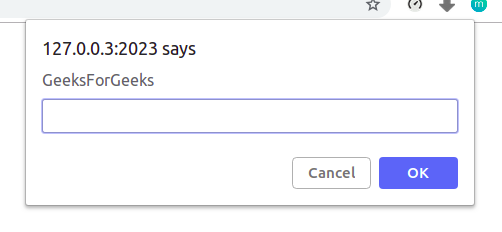
Hello@ashish,
Now I am trying using JavaScript, but when I send a request to the session login API and later initialize the session via SDK init, then it shows 401 (Unauthorized) for 'info' and 'isactive' requests of the session, I think so cookies which are required for this are getting blocked, please check the screenshot for better understanding.
Thank You!
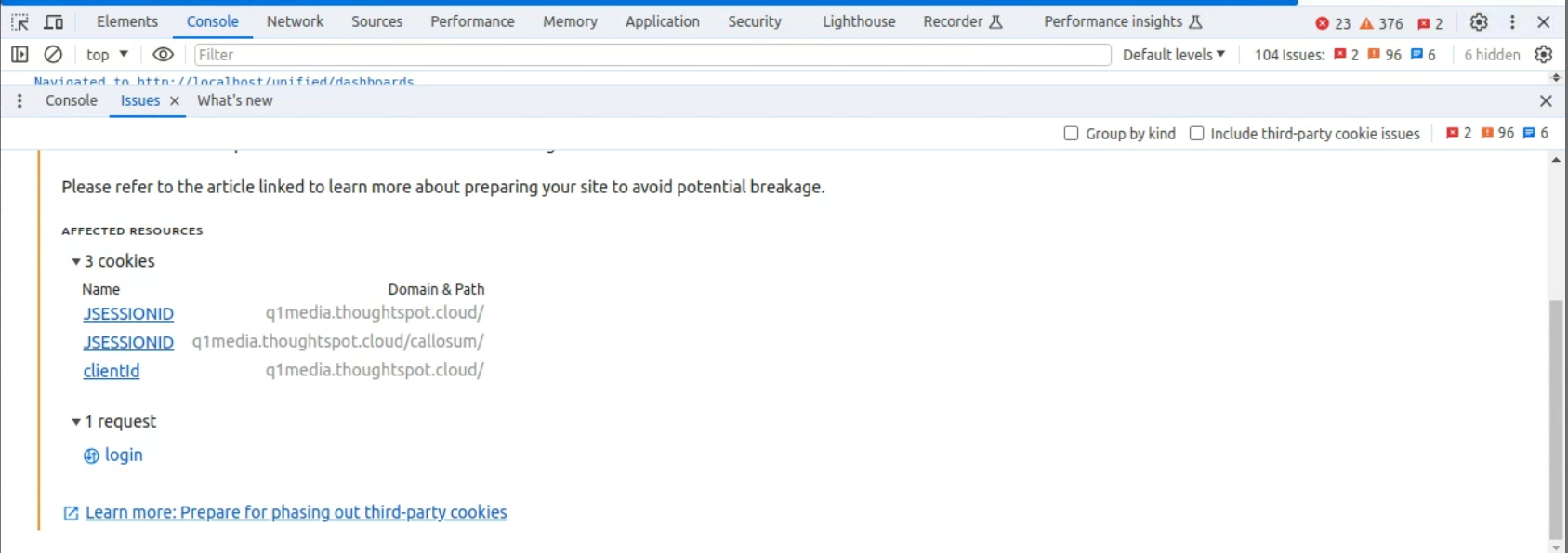
If you are using the SDK you do not need to use the
login
API. Just use the init
and pass the required credentials to that.