I'm having trouble with a library project
So I'm doing a library project and I've been tackling it step by step. All the apps need to do is create book cards from user input and display them in a grid but I haven't been able to add them to my array.
Here's the codepen: https://codepen.io/norwyx/pen/rNbZOmE?editors=0011
A little bit of context: all the functions at the bottom are pure UI stuff so not that important. But at the top I have things like the Book object that has some default values inside the constructor and then the properties. The Library object with the books constructor (the array Im having problems with) and the helper functions suck as addBook, getBook, inLibrary, and removeBook.
But what I'm facing is that I'm able to correctly get the info from the form, and successfully create the object (check the console, I left a console.log). But then when I need to push it into the library.books array, it is not working and I'm not storing the object anywhere.
Help would be very appreciated since I've been trying stuff for 2 days and no shot, so now I need some help. Thanks in advance.
50 Replies
you're using the wrong tool for the job
instead of an array, use a map
then map the book objects to the book name
an object could work too
also, it didnt work because you do a filter on the books array to see if it exsist. if it not exist it will return an empty array, that is still considered truthy
yeah, an array is truthy
or falsy
depends on how you check
the way you're doing, it is always truthy
the array.find method would work, but indeed. a map would be even better
yes, it is a tiny bit more annoying to use, but makes things a lot easier
adjusted to use a map (with title as key)
this.books = this.books.delete(title);
<-- delete updates the map in place
that line is wrong
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map/delete#return_value
you're setting this.books
to a boolean
instead, do return this.books.delete(title);
i would even use a private value
just add #books = new Map();
and you're set
and all references to this.books
become this.#books
oops, you were right
were? 😲 i am right 🤣
but yeah, the class looks clean af, super easy to read and not error prone
haha, yea.
i wont overkill with too much class options for now. book could even extend the library class
nah, this is fine at is it
think so too
i think so too
So I've never seen Maps. Now I can't seem to display the information I get. I'm able to see the info from the form but I can't seem to access where the books are stored. Why is this line not working?
Please keep in mind I have never seen Maps
show us the updated codepen
Sure
that still uses an array
Nah i switched it for a map

maybe thats why?
I fixed it, no longer that error but still can't find the library with the books inside
this is the issue
you're adding a function
In the last line?
no
Tbh I just googled how to access info from a map and copy pasted SO
library.addBook(newBook)
<-- herefucking hell
How didn't i read that
thats crazy
const newBook = getBookFromInput
<-- no parenthesys, which means that you are setting newBook
to be a functionFuck, i tought that when creating the functions the way i created them wasnt necessary to do it
Pretty dumb from me i guess
you just didn't run the function
no running = no new book
I just saw that way of creating functions when learning es6 and though it looked cleaner
so the parenthesis should fix it? what about seeing stuff from the map
is that line correct?
console.log([...library.books.entries()])
yes, it is
ok
let me try this
it's not the best way, but it is correct
What would be a more accurate way to do it?
because it works
but what would you do?
trying to learn best practices
for(let [title, book] of library.books.entries()) { .... }
it's not a matter of "best practices", but a matter of not creating an useless arrayBtw look at how it is displaying
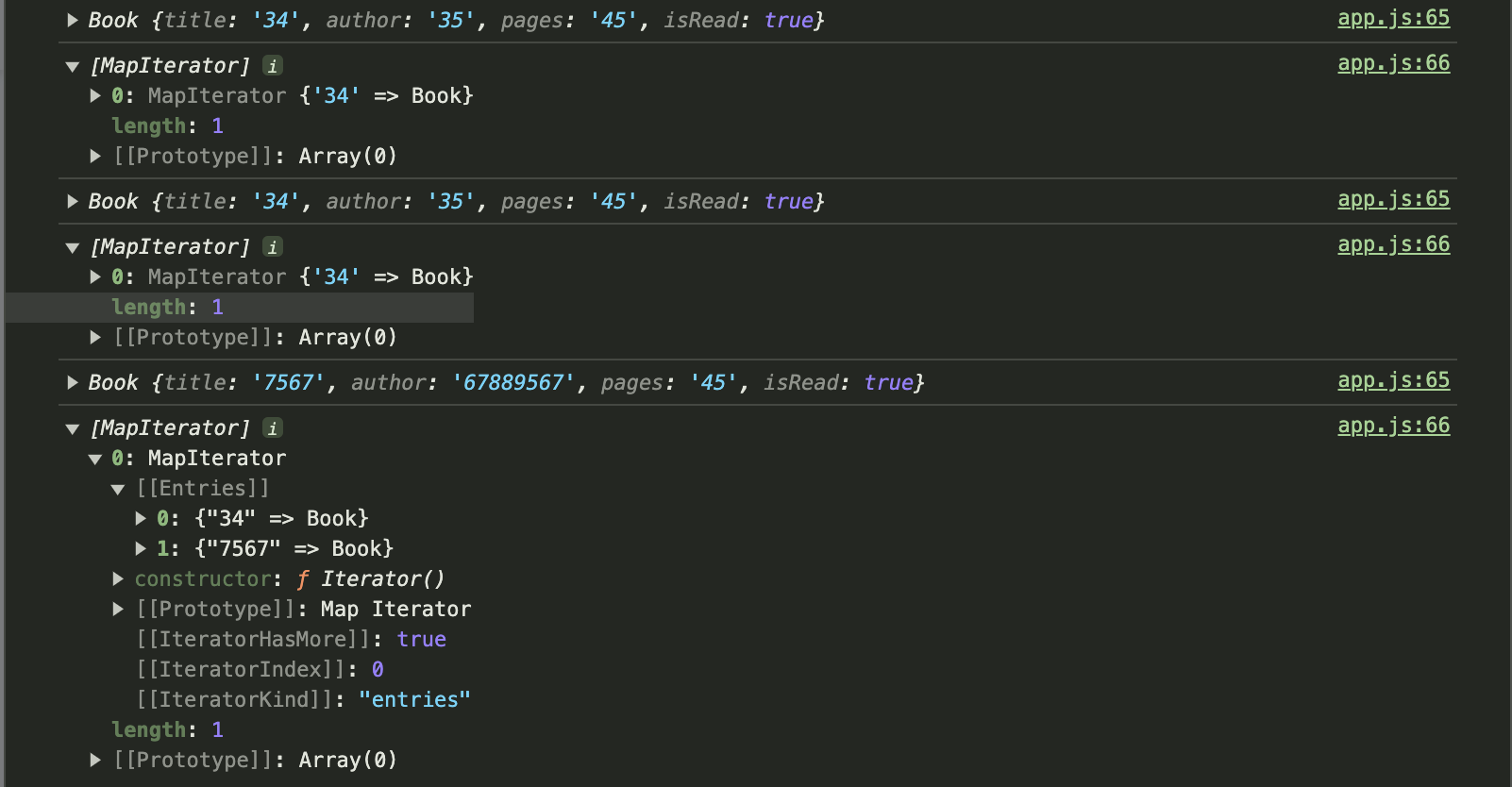
seems about right?
Yeah yeah just showing you
About what you said i removed the [] and now it shows the following way
That means now Im not creating a useless array?
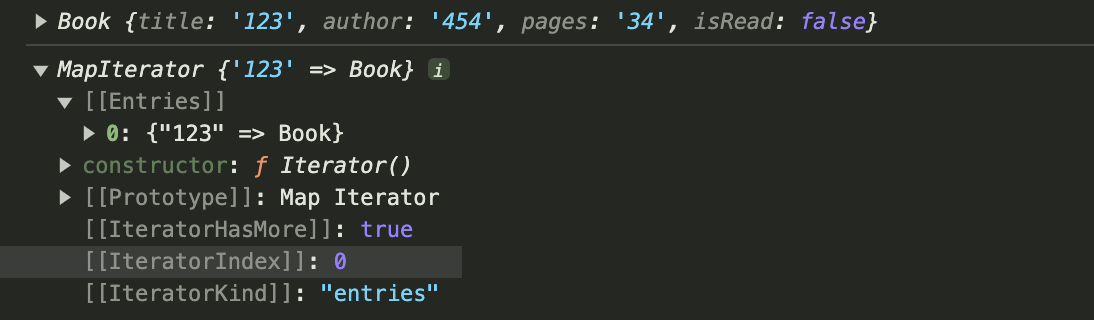
if you don't need the key, then that is fine
Ok seems about right bro. Thank you so much and also thanks to Mark. I truly learnt a lot by just seeing you two talking
Have a good night
Or morning wherever you are 🙂
it's way way into the night here
Go to bed @ἔρως i'm already up
i just woke up :/
then we have the same schedule 😉
im trying to fall back asleep
good luck with that. i give it another 30 minutes to try so too, otherwise I'm making my morning coffee
too early for morning