Hono Oauth Provider with JWT issue
Hey everyone! I'm not sure if this is the right place for me to post about this but recently I wanted to try to use Hono and also saw there was a package for it
@hoino/oauth-providers
which I also wanted to try.
Now I noticed that in my main login.ts route where I use hono/jwt
package then I'm getting errors inside my google.ts file where I use the googleAuth from @hono/oauth-providers/google
. Currently I'm still using the basic stuff to figure out how things work and how I can use it so don't mind that.
This is my /routes/auth/login.ts
file
and this is my /routes/auth/google.ts
file
and my vscode shows an error on the 3 const in google.ts:
I'm not really sure why exactly this is happening and how to resolve it but I did notice that it comes from the hono/jwt
usage which has the following in it's index.d.ts which is also weird cause the oauth-provider package has something similar too:
not sure if this is a bug that I should report or why this is happening/how to resolve it, I'd appreciate any help on this! ^^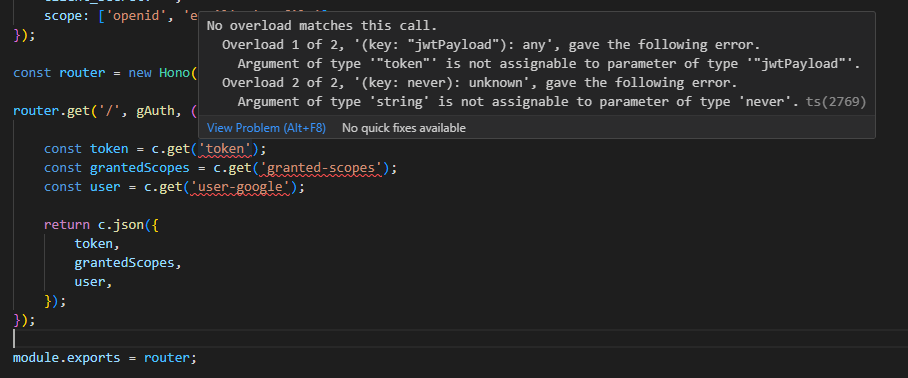
5 Replies
Update: I did found out that I can resolve it by adding the Variables type https://hono.dev/api/context#set-get
yet I'm not sure if this is the way it's supposed to work, it seems kinda odd to me because both already have these things defined, it's just that's it's not properly being picked up I guess
Context - Hono
Ultrafast web framework for Cloudflare Workers, Fastly Compute, Deno, Bun, Vercel, Node.js, and others. Fast, but not only fast.
Sorry no one got back to you. Yes you can post any questions here! The reason you had to define the types is because the middleware and routes can’t talk to each other in typescript and know which routes that middleware belongs to. Therefore you do have to declare in each instance of Hono any variables that may be set. You can also do this globally in case you have multiples routes using it across multiple files which you can see here https://hono.dev/api/context#contextvariablemap
Context - Hono
Ultrafast web framework for Cloudflare Workers, Fastly Compute, Deno, Bun, Vercel, Node.js, and others. Fast, but not only fast.
No worries, oh alright, I see thanks for this information/clarification ^^
I guess the thing that confuses me is that in
hono/dist/types/middleware/jwt/index.d.ts
is this:
so this is defining what you say and then the @hono/auth-providers
has this in its @hono/oauth-providers/dist/providers/google/index.d.ts
so it feels like this should just work out of the box without me adding anything extra
I did some extra testing and I assume this might be some strange override thingy going on? As I just checked, in the file where I have my Google endpoint for hono oauth providers I also have the sign from hono/jwt in there, if I move the line from hono/jwt above the one from google oauth providers, my vscode is not upset anymore :PikaThink:No you are right those types should be showing up. I know for sure the jwtPayload shows up as any for me, so I don't know what is happing on your end
I am reading through what you provided again (I was on my phone the first time) the jwt type is a known issue that will be resolved. For right now whatever you set it to cast the value. So
const token = c.get('jwtPayload') as myCustomType
Yeah I'm not sure, I initially resolved it like this ^ but now I noticed that if I move the
import { sign } from "hono/jwt";
above the import { googleAuth } from '@hono/oauth-providers/google'
it also seems to be fine