How do i make .txt file watcher with VS
i was trying to make .txt file watcher and i just added these functions;
but this RefreshList() code is too broken, my veriler.txt datas filled like this texts;
3 Replies
and
in the first example eighth one is sometimes can be empty or not
depending on how I fill them out, they are recorded in the "veriler.txt" file.
If I summarize the code along with its errors; when there is an update in 'veriler.txt', my DataGridView table named 'veriTablosu' is deleted and reloaded. However, when this table is deleted and reloaded, as shown in the example above, the 8th and empty data shift to the left, and the 9th, 10th, 11th, 12th, and 13th data take their place. Also, there is a code assigned to Cell_Click where data is sent to a total of 10 TextBoxes and 2 ComboBoxes located at the top whenever a cell is clicked. Somehow, this code also transfers the spaces around the '|' symbol in 'veriler.txt' to the 'veriTablosu' along with the data. As a result, spaces are added before and after the text in the total of 10 TextBoxes, and nothing is selected in the ComboBoxes as if that data were not there.
.
Thats why i need to help its too complicated for me
I don't really understand. What are you visibly seeing? Just doing a simple repro I'm getting something easy to use:
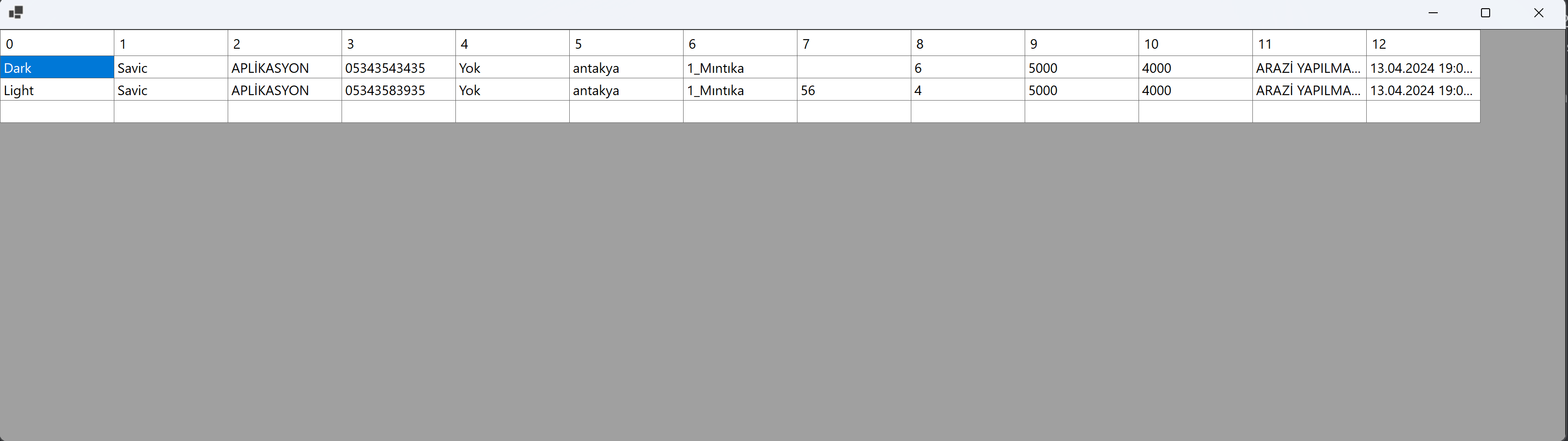
s_data
was just copied from what you had here