How to use multiple schema definitions ( e.g. <catalog>.<schema>.<table> )
Hey guys,
I'm currently working on a new version for our graphql wrapper.
Currently we're using knex to generate the query - it works as expected but isn't typesafe with how we use it 🙈
I'm currently evaluating kysely and 99% seems to work.
Some details: We're using trino as datasource ( via their http api ). The expected SQL Query requires to specify also the catalog name in the
FROM
statement.
Short example:
I have tested some scenarios, but it seems I missed something.
Question: Is it possible to generate this kind of FROM
statement with kysely?
here the playground link: https://kyse.link/EaWQ3Solution:Jump to solution
You could actually get away with:
```ts
const test = db
.selectFrom<"person">(...
7 Replies
Have seen, that kysely provides a sql helper (
sql.id
).
Via:
I get the correct SELECT statement, but also some type errors 😕
Playground: https://kyse.link/sV7ww
setting the types seems to work:
https://kyse.link/fDkOU
Question: Is this the "way to go" ?
Note: it seems I missed something in the type definition, because I have now access to all fields from all tables ( tested it locally with two tables )Hey 👋
When passing expressions to
.selectFrom
you're required to alias them with .as
.
Try this:
or
Hi, thanks for the quick response - will give it a try
Solution
You could actually get away with:
or:
Will try the second approach since my current eslint setup will throw an error if there is an
any
(using the t3 turbo stack)
this works perfect! Thanks for the quick help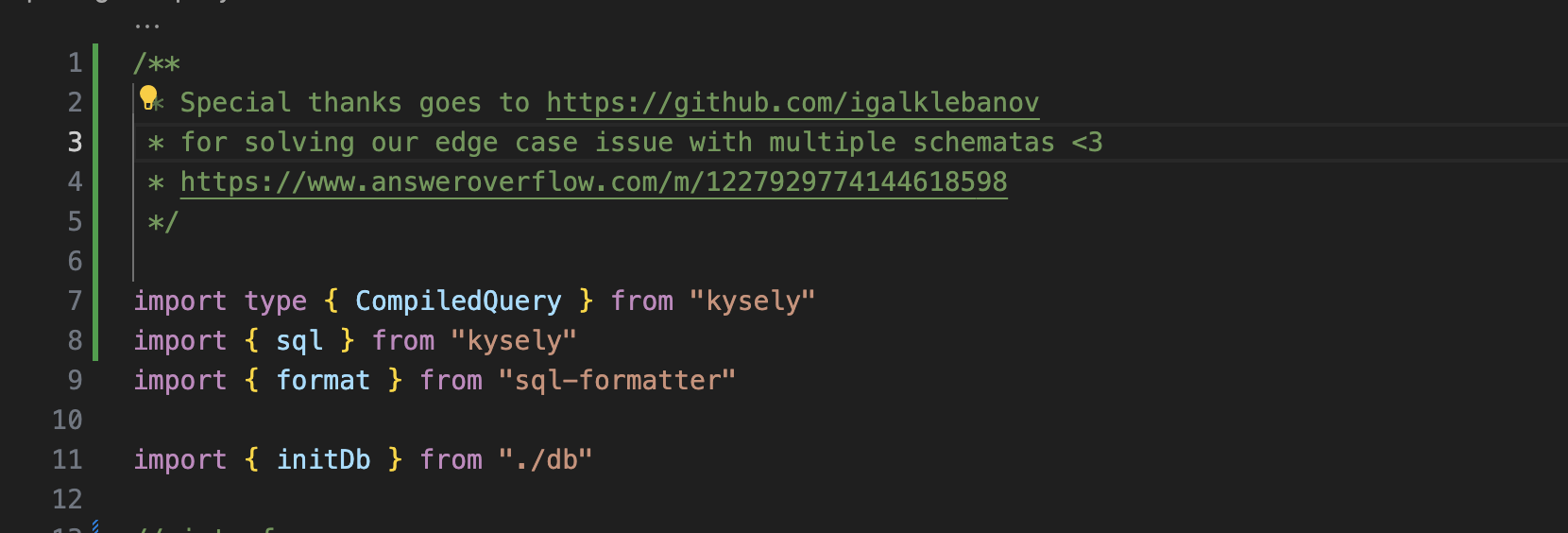
Updated the code and made it a bit generic:
the test shows how I want to implement it later in the graphql resolver
selectFields are "type-safe" and you can only select the fields which are defined in the provided table definition
hope this is not bad practices