NextJS + Pages plugin error
so here is my current code that is located
and i have screenshot the errors i am getting back from the turnstile plugin its triggering on lines 36/37
@/src/app/api/contact/route.ts
@/src/app/api/contact/route.ts
import turnstilePlugin from '@cloudflare/pages-plugin-turnstile';
import mailChannelsPlugin from '@cloudflare/pages-plugin-mailchannels';
const { MAIL_EMAIL, MAIL_NAME, MAILTO_EMAIL, MAILTO_NAME, TURNSTILE_SECRET_KEY } = process.env;
if (!MAIL_EMAIL || !MAIL_NAME || !MAILTO_EMAIL || !MAILTO_NAME || !TURNSTILE_SECRET_KEY) {
throw new Error("Missing .env configuration");
}
interface FormData {
name: string;
email: string;
phone: string;
message: string;
token: string;
}
export async function POST(req: Request) {
if (req.method !== 'POST') {
return new Response('Method Not Allowed', { status: 405 });
}
try {
const formData = await req.json() as FormData;
console.log('Received form data:', formData);
if (!formData.token) {
return new Response('Token is required', { status: 400 });
}
console.log('Rtoken:', formData.token);
// Verify Turnstile token
console.log('Verifying Turnstile token...');
const turnstileResponse = await turnstilePlugin({
secret: TURNSTILE_SECRET_KEY as string,
response: formData.token
});
if (!turnstileResponse.success) {
console.error('Turnstile verification failed:', turnstileResponse);
return new Response('Turnstile verification failed', { status: 403 });
}
console.log('Turnstile verification successful.');
// Prepare email data
const { token, ...emailData } = formData;
console.log('Preparing email data:', emailData);
const emailResponse = await mailChannelsPlugin({
personalizations: [
{
to: [{ name: MAILTO_NAME, email: MAILTO_EMAIL }],
cc: [{ name: emailData.name, email: emailData.email }],
subject: 'New Form Submission from ' + emailData.name,
content: [{
type: 'text/plain',
value: 'Form submission details: ' + JSON.stringify(emailData),
}]
},
],
from: {
name: MAIL_NAME,
email: MAIL_EMAIL,
},
respondWith: () => {
return new Response('Thank you for your message.');
},
})
if (emailResponse.ok) {
console.log('Email submitted successfully:', emailResponse);
return new Response('Email submitted successfully', { status: 200 });
} else {
console.error('Failed to send email:', emailResponse);
return new Response('Failed to send email', { status: emailResponse.status });
}
} catch (error) {
console.error('Error occurred in POST function:', error);
return new Response('An error occurred while processing your request. ' + error, { status: 500 });
}
};
import turnstilePlugin from '@cloudflare/pages-plugin-turnstile';
import mailChannelsPlugin from '@cloudflare/pages-plugin-mailchannels';
const { MAIL_EMAIL, MAIL_NAME, MAILTO_EMAIL, MAILTO_NAME, TURNSTILE_SECRET_KEY } = process.env;
if (!MAIL_EMAIL || !MAIL_NAME || !MAILTO_EMAIL || !MAILTO_NAME || !TURNSTILE_SECRET_KEY) {
throw new Error("Missing .env configuration");
}
interface FormData {
name: string;
email: string;
phone: string;
message: string;
token: string;
}
export async function POST(req: Request) {
if (req.method !== 'POST') {
return new Response('Method Not Allowed', { status: 405 });
}
try {
const formData = await req.json() as FormData;
console.log('Received form data:', formData);
if (!formData.token) {
return new Response('Token is required', { status: 400 });
}
console.log('Rtoken:', formData.token);
// Verify Turnstile token
console.log('Verifying Turnstile token...');
const turnstileResponse = await turnstilePlugin({
secret: TURNSTILE_SECRET_KEY as string,
response: formData.token
});
if (!turnstileResponse.success) {
console.error('Turnstile verification failed:', turnstileResponse);
return new Response('Turnstile verification failed', { status: 403 });
}
console.log('Turnstile verification successful.');
// Prepare email data
const { token, ...emailData } = formData;
console.log('Preparing email data:', emailData);
const emailResponse = await mailChannelsPlugin({
personalizations: [
{
to: [{ name: MAILTO_NAME, email: MAILTO_EMAIL }],
cc: [{ name: emailData.name, email: emailData.email }],
subject: 'New Form Submission from ' + emailData.name,
content: [{
type: 'text/plain',
value: 'Form submission details: ' + JSON.stringify(emailData),
}]
},
],
from: {
name: MAIL_NAME,
email: MAIL_EMAIL,
},
respondWith: () => {
return new Response('Thank you for your message.');
},
})
if (emailResponse.ok) {
console.log('Email submitted successfully:', emailResponse);
return new Response('Email submitted successfully', { status: 200 });
} else {
console.error('Failed to send email:', emailResponse);
return new Response('Failed to send email', { status: emailResponse.status });
}
} catch (error) {
console.error('Error occurred in POST function:', error);
return new Response('An error occurred while processing your request. ' + error, { status: 500 });
}
};
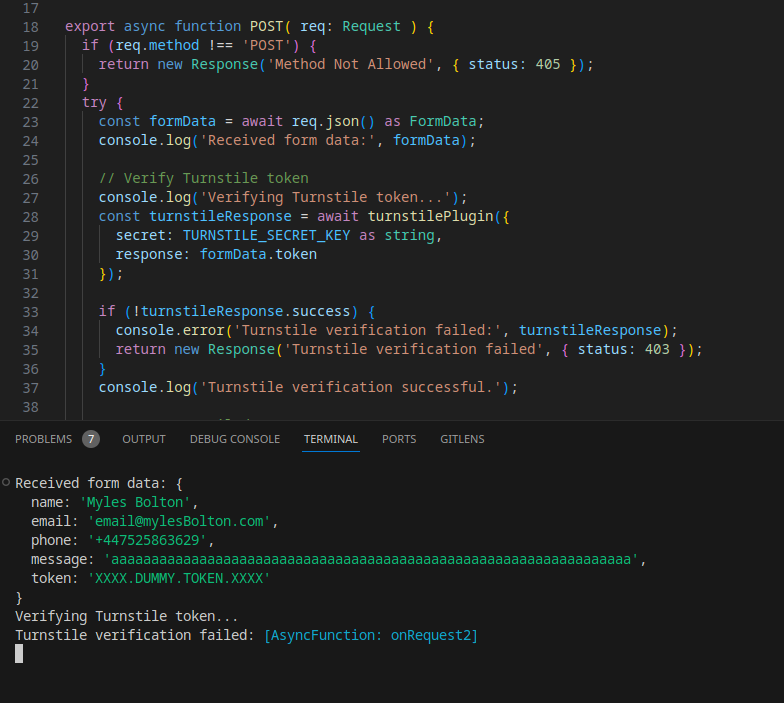
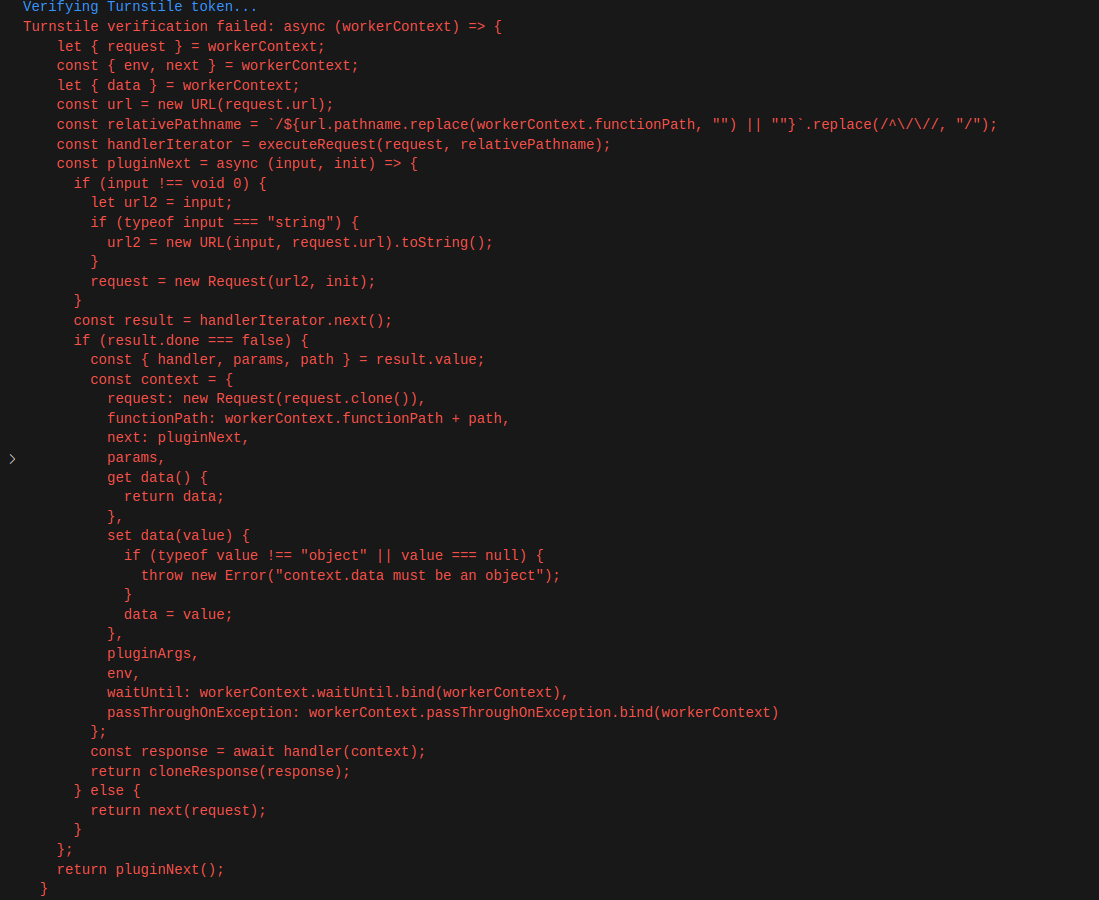
2 Replies
i dont mean to waste anyone's time with this im just trying to replace node-mailer in my code for my contact form
but i will probably end up reverting back to a server side validation https://developers.cloudflare.com/turnstile/get-started/server-side-validation/
ok ye i chaged to the server side validation now the mailchalles plugin is throwing this error
maybe i messed up my code somewhere
Failed to send email: async (workerContext) => {
let { request } = workerContext;
const { env, next } = workerContext;
let { data } = workerContext;
const url = new URL(request.url);
const relativePathname = `/${url.pathname.replace(workerContext.functionPath, "") || ""}`.replace(/^\/\//, "/");
const handlerIterator = executeRequest2(request, relativePathname);
const pluginNext = async (input, init) => {
if (input !== void 0) {
let url2 = input;
if (typeof input === "string") {
url2 = new URL(input, request.url).toString();
}
request = new Request(url2, init);
}
const result = handlerIterator.next();
if (result.done === false) {
const { handler, params, path } = result.value;
const context = {
request: new Request(request.clone()),
functionPath: workerContext.functionPath + path,
next: pluginNext,
params,
get data() {
return data;
},
set data(value) {
if (typeof value !== "object" || value === null) {
throw new Error("context.data must be an object");
}
data = value;
},
pluginArgs,
env,
waitUntil: workerContext.waitUntil.bind(workerContext),
passThroughOnException: workerContext.passThroughOnException.bind(workerContext)
};
const response = await handler(context);
return cloneResponse2(response);
} else {
return next(request);
}
};
return pluginNext();
}
Failed to send email: async (workerContext) => {
let { request } = workerContext;
const { env, next } = workerContext;
let { data } = workerContext;
const url = new URL(request.url);
const relativePathname = `/${url.pathname.replace(workerContext.functionPath, "") || ""}`.replace(/^\/\//, "/");
const handlerIterator = executeRequest2(request, relativePathname);
const pluginNext = async (input, init) => {
if (input !== void 0) {
let url2 = input;
if (typeof input === "string") {
url2 = new URL(input, request.url).toString();
}
request = new Request(url2, init);
}
const result = handlerIterator.next();
if (result.done === false) {
const { handler, params, path } = result.value;
const context = {
request: new Request(request.clone()),
functionPath: workerContext.functionPath + path,
next: pluginNext,
params,
get data() {
return data;
},
set data(value) {
if (typeof value !== "object" || value === null) {
throw new Error("context.data must be an object");
}
data = value;
},
pluginArgs,
env,
waitUntil: workerContext.waitUntil.bind(workerContext),
passThroughOnException: workerContext.passThroughOnException.bind(workerContext)
};
const response = await handler(context);
return cloneResponse2(response);
} else {
return next(request);
}
};
return pluginNext();
}
found this so im going to use this instead of the plugins
https://github.com/Le0Developer/cloudflare-discord-mailing/blob/master/src/send.ts
GitHub
cloudflare-discord-mailing/src/send.ts at master · Le0Developer/clo...
Cloudflare Workers + Route to Workers + Mailchannels => Discord - Le0Developer/cloudflare-discord-mailing