✅ Index Outside Of Bounds System.Data.SQLite
UserModel: https://pastebin.com/wJBdA30Y
LoginModel: https://pastebin.com/QYMHxMbT
LoginViewModel: https://pastebin.com/XdS6P11W
DatabaseEngine function in question: https://pastebin.com/ipgtkTLd
I keep getting the error in the screen shot from the funciton in question on the line where I execute
and I don't know why. I have tried moving them to two separate while loops, and two separate model variables like
and I still got the error. Thanks in advance
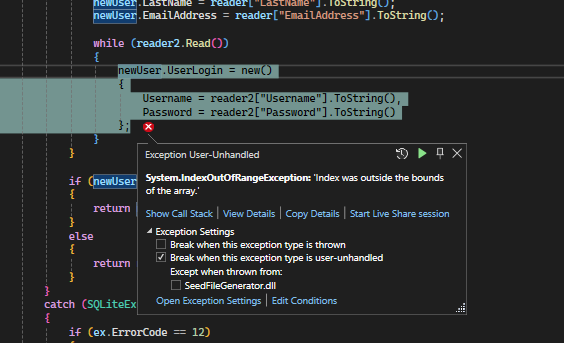
154 Replies
it probably refers to the column names you're trying to read from the reader
unrelated, but you shouldn't be doing this
just use
throw;
to rethrow the exception you already have
there are also a lot of disposable objects you aren't disposing
well, not a lot but the data readersUse
GetFoo()
, not indexer
Foo being the datatypeit also seems like you should be using a join instead of 2 queries
that specifically was from where I was trying to error code value and return specific messages based on it. I'll just throw the exception.
In response to
It probably refers to the column names you're trying to read from the readerIn my create database function, I create a table called
login
with columns Username
and Password
and in my code,
I read from login
by Username
for Password
and in the screenshot, you'll see the information is there.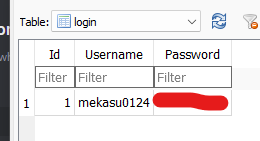
I have a basic understanding of using relative databases and that's about as far as it goes
so you're selecting
Password
but trying to get a Username
tooI'm here to learn how to do better
there is no
Username
column in the result set for that queryno selecting Password where Username = userLogin.Username
I hope password isnt in plain text - unrelated but important.
that doesn't affect what i said
you're only getting the passwords, not the usernames and passwords
so
reader2["Username"]
doesn't make senseit should only be getting 1 password if the username is a match to the usernames in the table
the error isn't about the number of records
it's about trying to access a column that doesn't exist
Check your query
I do apologize. I'm really confused on how when in the screenshot of the database, you can see the table and columns and that I'm calling them correctly
you're not
you are doing
SELECT Password...
then expecting the results to contain both Username
and Password
if you want the username too, you need to SELECT Username, Password...
ok so it should only be selecting the password given a username match, so how do I need to write it?
really this should be one query:
you have no reason to read the username back from the database because that's what you're using as your filter in the where
As per this, I've been trying to learn about salting passwords in C# in a playground and so far I've gotten to
but I wanted to get the login screen working first before hashing the password and dehashing the password as the functions to encrypt and decrypt the password will be going into an EncryptionGenerator class
this just absolutely blew my mind. Would you mind to show me what this looks like in my code with like some comments explaining it? If not, then would you mind linking a tutorial I can look at?
you put it in like any other query, then the result set you get back will have those 4 columns in it
it makes the database engine do the work of matching up your users and logins and gives you just the data you need in a single go
so like
i wouldn't squish it into one line, but yeah
ok I don't know how I would separate it
use triple quotes
yep lmao figured
also in general you should avoid
SELECT *
specifying an explicit set/order of columns to get back will avoid bugs and receiving more data than you care abouthttps://pastebin.com/8sQqRSZw how about this
ok, sounds good to me, any examples?
the query i just shared is one 😛
but you said to avoid Select and that one uses select
or am I missing the point
no
avoid
SELECT *
specificallyohhhh got you
^ ?
it looks like you dropped my query in when you have to rewrite the whole method
you should only have one query and read the data just from that using either the names in the
SELECT
list or by indexI wasn't aware I had to change the function. sorry
oh ok. let me see if i can do that. one sec
when in doubt, $tias
the compiler and runtime will be better at telling you it's wrong than me reading raw text on pastebin
what about this https://pastebin.com/McrbAAvp
Index was outside the bounds of the array
on newUser.FirstName = reader["u.FirstName"].ToString()
. So I did something wrong with the column names?i personally wouldn't use the column names at all, it's redundant
you can get them with a 0 based index
i'd get rid of the loop too since you're only expecting one result
I need to get all the information since if the passwords match, I'm returning all the information otherwise I'm returning an emtpy model
what does that have to do with the loop
is this how you meant?

https://learn.microsoft.com/en-us/dotnet/api/microsoft.data.sqlite.sqlitedatareader?view=msdata-sqlite-7.0.0
the loop is about number of rows, you only have one row so there's no reason to loop
ok so how do I need to write it? Something like
?
you renamed the reader for some reason? that's not what i'm suggesting
look at the docs, specifically the part i screenshotted
I'm trying
ok I see the part that you specified but I don't know how to use it
it's a method on
SqliteDataReader
ok so reader.GetString("u.FirstName"); ?
you tell me, what do the docs say it accepts as an argument?
integer so I'd assume then reader.GetString(0);
right
that index refers to the order the columns are in in your
SELECT
so it would be ordered like
["u.FirstName", "u.LastName", "u.EmailAddress", "l.Password"]
or replace each one for its database valueif you were to treat the columns as an actual array then yes, that's the order they'd be in
ok so what step did I miss?
where?
reader.GetString(0);
is right if you want to get the first name for the rowok so then how would I get the last name? reader.GetString(1);?
yes, you'd index them just like you'd index an array
oh ok. bet
current code: https://pastebin.com/vSZnT29z
I'm not sure what I am doing wrong at this point. I thought I understood everything you said
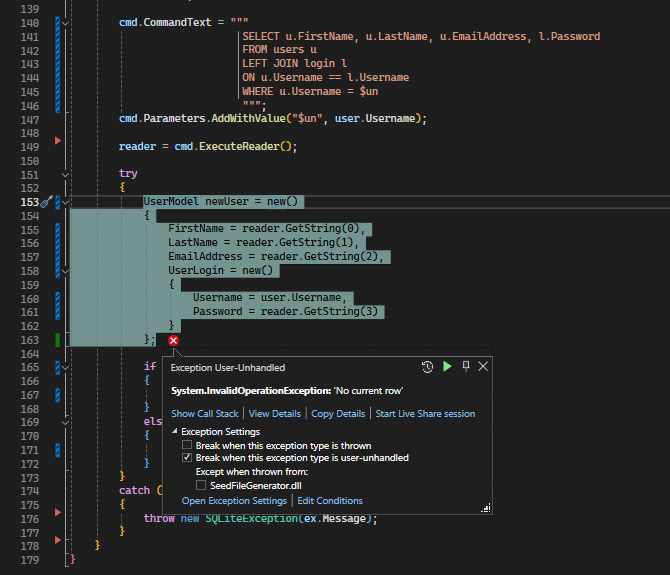
you still have to call Read() to advance the reader to the row
even if it's not in a loop
the reader starts "before" the first row if that makes sense
do I do that on the GetString()?
no, you do it like you were doing before
oh ok
just not in the loop
reader.Read();
then read
yeah
ok so I did reader.Read() before the model but this time instead of an error being thrown or anything like that, it just did nothing
what does "nothing" mean?
did you put a breakpoint and see if it set the properties of
newUser
like you expected?so using breakpoints in the database file and the login view model, It obtained my login inputs and passed them to the database function just fine. It queried the database and obtained the wanted results just fine. I ran the program, it got, passed, obtained the wanted data to/from the database, but then returned a null object. Then I remembered that the models used Id's as well so I go back and add in the Id fields to both models, and now instead of it breaking at point 1/4 it just breaks at spot 4/4 and has a null user model returned.
current code: https://pastebin.com/B1xgTx07
ok so now I'm getting this issue
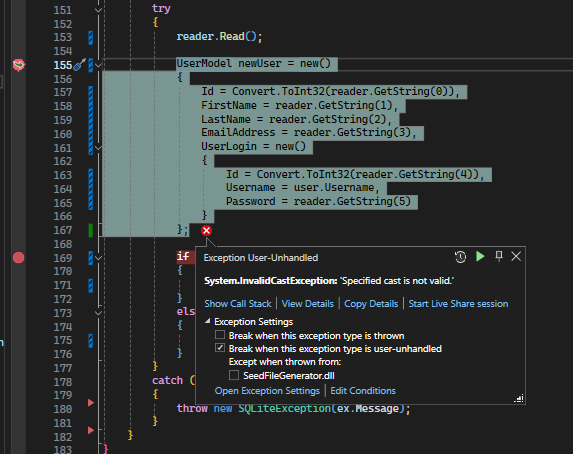
ok so upon further inspection,
reader.Read();
get all the correct wanted values, however, when I attempt to obtain those values inside the models, it's now a null object.
like when it breaks on reader.Read()
the hasRows
boolean is true
, but then when I step over to the next breakpoint on the newUser
user model, the hasRows
property of reader.Read()
is now false
. Past this, I'm lostdont use getstring for everything
if the type is a number say int
reader have other methods
i.e.:
reader.GetInt32(index)
its the same process of using GetString but u just choose the right method for the right type in the database
does that make sense?
https://learn.microsoft.com/en-us/dotnet/api/microsoft.data.sqlite.sqlitedatareader?view=msdata-sqlite-7.0.0#methods
there is a list with all the options u have to use with the reader
right. I saw that on the docs after posting my question. I changed it to doing so with the Id's on both models, however, I still have the same issue. The
reader.Read()
is getting the wanted values just fine, but when I try and put them into the models, they become null and I don't know why. I'm fixing to try and set each item from the database equal to <type> <variable>
setup and then use the variables in the modelssee here, in screen shot #1, it has the wanted values, but in screenshot #2 no sooner than I say
int userId = reader.GetInt32(0);
it's now null.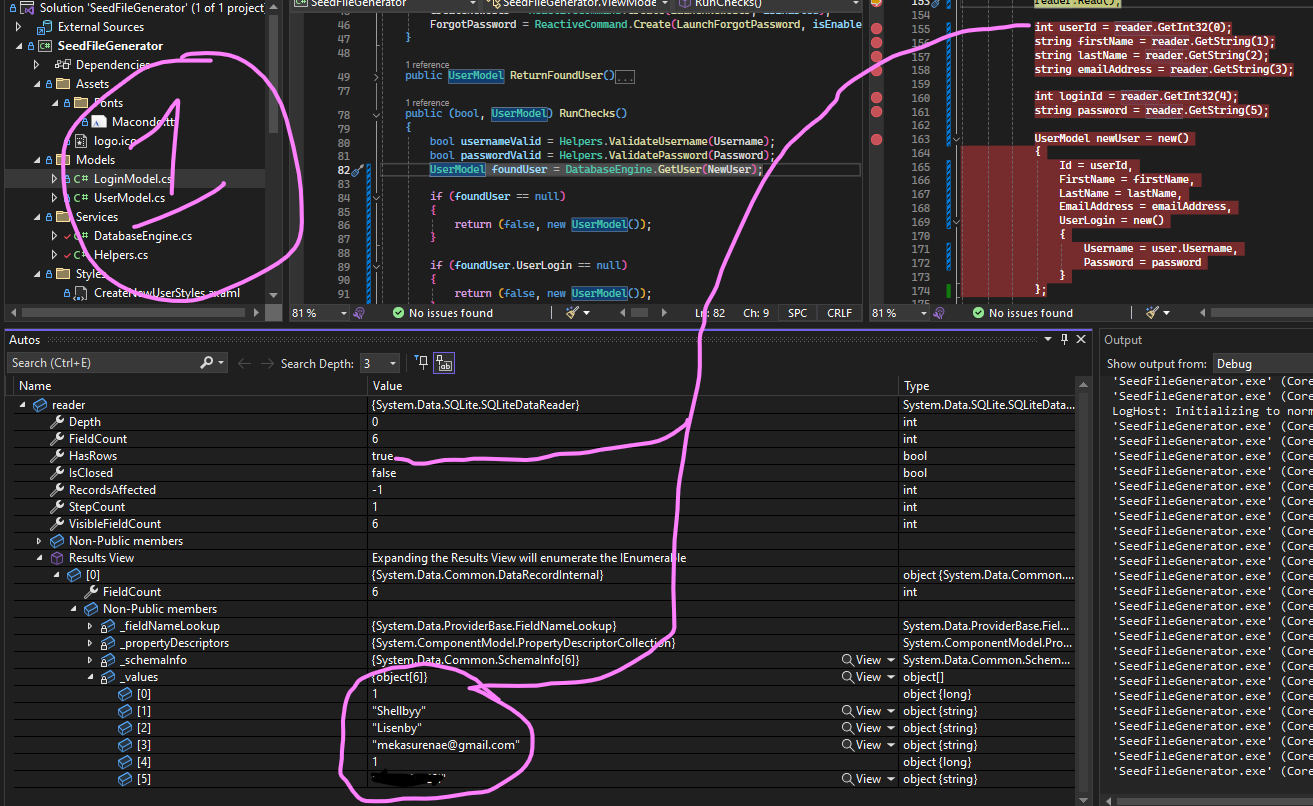
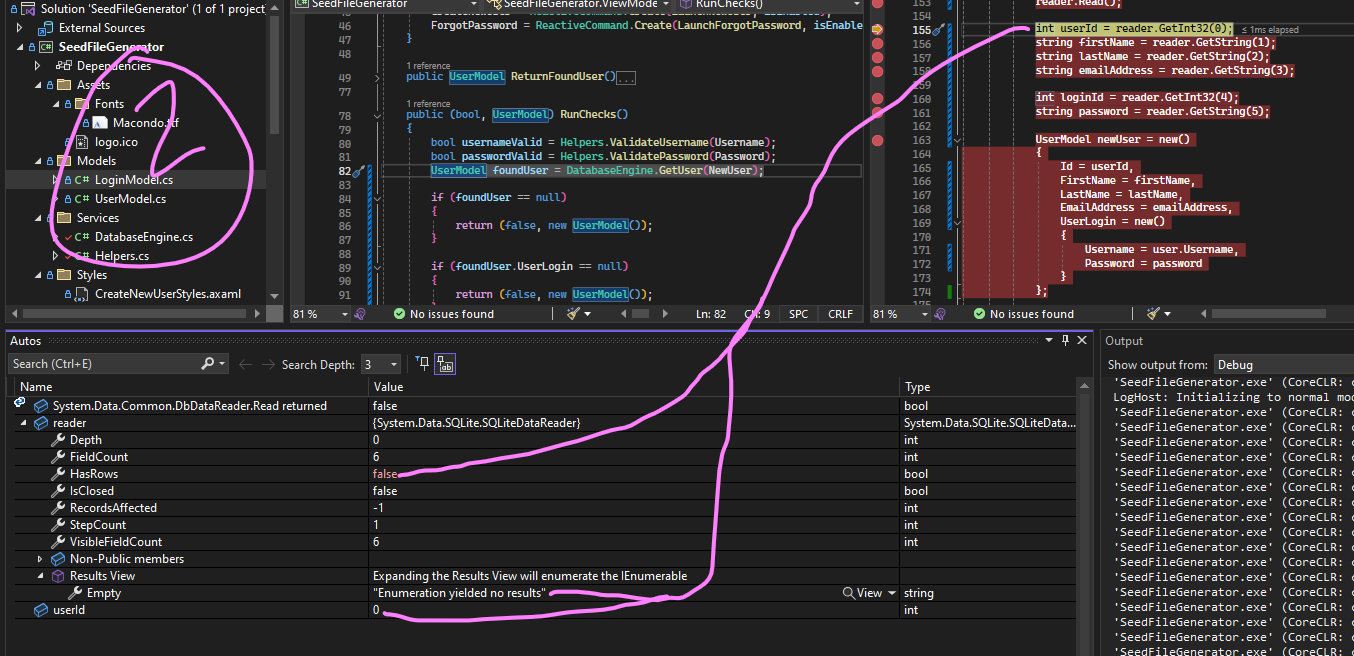
that makes sense if you ask me. Your result only contains one single row
so when you call
reader.Read()
that row is read, and the result set contains no further rowsso then how am I supposed to write it? I'm honestly confused lmao I thought I was doing it right by indexing the reader....
lemme set up a test project real quick. Have not worked with raw ADO in many years
haha ok. I just want to become a lot better at databases than I currently am. I'm only good enough to write to/pull information from a relative database, and you see how well that's going 😂
we all gotta start somewhere 🙂
and learnign how to write SQL queries is good
completely 100% side question. If I was to put advertisements into my application that only I ever used, and I let that application just run and run and run and it's loading ads every so few minutes or whatever and playing them, would I still get paid from the ads, or would that be illegal? lmao
Sounds illegal
but I'm not sure
absolutely. I've been listening to this Talk Python to me podcast, and the most that I hear of what people are looking for (mind you this was back in 2015) is those who are excellent with linux servers, database queries and other workings, and basically all backend stuff
this works just fine for me
can you show the most recent command/reader code you have?
https://pastebin.com/bRc0c5fr this is my entire database file.
and what method are we looking at?
I had nearly this exact thing before I moved onto the complicated query statement
GetUser method.
GetUser
?
k
hm, that seems fine at a glance
I'll emulate that database structurebefore I had the compound statement, I had
I had this before the complicated select statement that I have now and I was getting an out of bounds error on run-time when I would attempt to login to my app, however, after re-writing to what it is now in the GetUser function, I have the problems in the screenshots I provided before this conversation today and I can't figure out why the values go from being there in the
reader.Read()
to them suddenly being null on the next step which we've identified is an issue on how I'm reading the information from reader
works absolutely fine here
only thing I changed was the table names
wanna hop to #vc-2 and screenshare?
yea we can
this
arion
REPL Result: Success
Result: byte[]
Compile: 309.642ms | Execution: 19.890ms | React with ❌ to remove this embed.
The client shouldn't have access to the database when the server is hosted somewhere
Usually its like this (+ some more complicated steps for bigger projects)
Client -> Web API -> Database
so how would I apply that return style to this function?
you can use the non-generic
Result
so just
? It's an insert function so it doesn't return anything but a t/f value
or is a ternary thing.
return Result.IsSuccess ? cmd.ExecuteNonQuery() : Result.Failure;
look at the methods defined on it
oh ok so
return Result.Ok();
for the happy flowbet tyvm
in my app.axaml.cs, how would I go about setting the application to the max_width and max_height of the screen it's being viewed on? Like a
mainWindow.ShowMaximized()
or somethingI don't this you can do this in the app.axaml.cs but you can do it for the individual windows
eg.
In the window axaml.cs you can also call
WindowState =
from code
you could inherit the Window class to have it apply some logic beforehand, eg.
then your Windows inherit that instead of just : Window
oh ok bet
or just put that line in the constructor of the windows you want to start maximized
that's really not what you should use inheritance for
so I'd just put it in the code behind for the screen that I want to be maximized? That's every screen except for literally 1 😂
#copyAndPastFTW
as someone who uses programs, i would be pretty annoyed if something kept opening fullscreen windows
how many windows do you have? is there a reason you're not just replacing the content in a single window?
as someone who uses programs, I would be pretty annoyed if something kept opening fullscreen windowsit's not a public application. I'm building it specifically for myself and I prefer full screen 🙂
how many window do you have?right now, just the 1. I just started the project last night lol
is there a reason you're not just replacing the content in a single window?I am lol I use the MainWindowView to display content. I bind it to a Content variable in the view model and I load each "screens" view model and display it when a function is called. Like so it goes in the
.axaml.cs
file then? If yes, how should it be written?If we're still talking about the WindowState, it can be done in any of the views files, that being, .axaml or .cs
if in cs, anywhere in the constructor (preferrably after
InitializeComponent();
)
if in the .axaml file its just another parameter in the <Window > tag
(Something along those lines)
You can also remove the window frame typically associated with most windows if you want (though there are reasons not to do that)
The property is called "SystemDecorations" and the enum for that is "BorderOnly" or "None"
There's also another thing you could do, thats to extend the window into the window area
that would look like this:The property for that is
ExtendClientAreaToDecorationsHint="True"
what do you mean by
. . . extends the window into the window area?
sry, meant more like, extends the UI into the window area
like the screenshot
so in my user control, if I set the usercontrol.background to blue it'll, extend the blue up to the top of hte window bar like the screenshot instead of having a white title bar and blue background user control?
like this, the UI area is where the window decorations are
i believe so
oh ok
lemme see what i did here on my event watcher window
okie dokie
yup, just set the background property
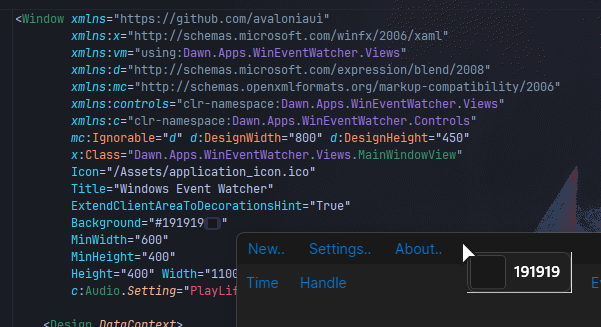
sweet tyvm
:okHand:
another question if you don't mind. I'm moving over from Avalonia.ReactiveUI to CommunityToolkit.Mvvm. I know how to do screen navigation in Avalonia.ReactiveUI by using Observable.Merge()'s, however, I can't find anything to show me how to do screen navigation using CommunityToolkit.Mvvm
Personally, I've never used the Mvvm toolkit, i've never had a need for it
Though speaking of Reactive, I am a big fan of System.Reactive, i love the IObservable extensions and most of the things relating to IDisposable of the package
It's pretty close to one of my other favourite packages (Vanara.PInvoke xD)
I just hate writing all the boiler code. I believe I can use them side by side that way I can keep the navigation that I'm used to using, and I don't have to write as much boiler plate code
Doesn't Visual Studio have Live Templates?
rider does, i use it to generate my avalonia boilerplate
I have no idea. The only reason I'm even using Rider is because I updated Visual Studio to it's latest version and it broke the axaml previewer in the IDE and I can't figure out how to get it back. I've tried literally removing every installation and file/folder I could find on my system for Visual Studio and re-install and that didn't work. I tried to install 2019 alongside it, but the installer for 2019 kept saying that a newer version was installed so it can't install a later version. So again, i went through the entire computer and removed everything I could find of Visual Studio and Visual Studio 2022 and it still wouldn't work. restarted the computer multiple times, 2019 still kept detecting a newer version and refusing to download so I setup vscode for c# and avalonia development and that's fine, but I liked the simplicity of Visual Studio so I'm giving rider a try, but now I'm having the issue of my tags won't auto complete. In the axaml, if I type
<Grid>
and it just stays like that. it doesn't autocomplete the closing tag like vs and vscode do.Do you have the Avalonia Rider plugin?
https://docs.avaloniaui.net/docs/reference/jetbrains-rider-ide/jetbrains-rider-setup#install-the-avalonia-plugin
I do but see in that snippet you have to select the component, but in visual studio I didn't have to do that. Once I pressed the
>
button to close the opening tag, it auto closed it for meAh, yea I get the same issue on that. You could open an issue on their github to try to get that added
I've honestly just been needing to wipe my computer and start over so that's what I just did lmao I'm going to download vs 2019 now and see what happens
why not the latest version?
because I like having the axaml previewer in the editor with me instead of every time I make a change, having to run/reload the project and wait for it to rebuild, etc.
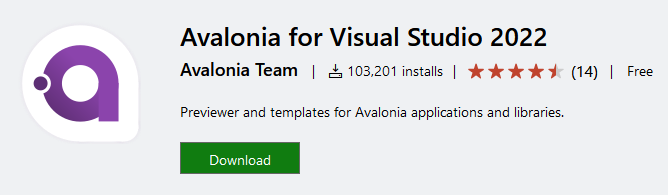
there is a project called avaloniahotreload
if u can set it up and it runs u should get a nice hot reload
the designer is really not good reference for the most part
I just dont remember the github, I think its this one https://github.com/AvaloniaUI/Live.Avalonia
GitHub
GitHub - AvaloniaUI/Live.Avalonia: In-app live reload for Avalonia ...
In-app live reload for Avalonia applications. Contribute to AvaloniaUI/Live.Avalonia development by creating an account on GitHub.
ok so maybe vs 2019 wasn't what I was thinking. VS 2022 is on version 17.9.6. How do I go to an earlier version of vs 2022? I don't see it on their downloads page
Visual Studio
Free Developer Software & Services - Visual Studio
Free offers: Visual Studio Community, Visual Studio Code, VSTS, and Dev Essentials.
left side one purple
right. that's goign to download version 17.9.6. I need to find the VS 2022 version older than that for when the axaml previewer wasn't broken
unless you're on preview
which is 17.10.0 preview 3.0
axaml designer is not broken on latest
only on preview and if it is as I have not tried mysefl
but u can use https://github.com/Kir-Antipov/HotAvalonia
GitHub
GitHub - Kir-Antipov/HotAvalonia: 🔥 Supercharge your Avalonia devel...
🔥 Supercharge your Avalonia development experience with hot reload capabilities - Kir-Antipov/HotAvalonia
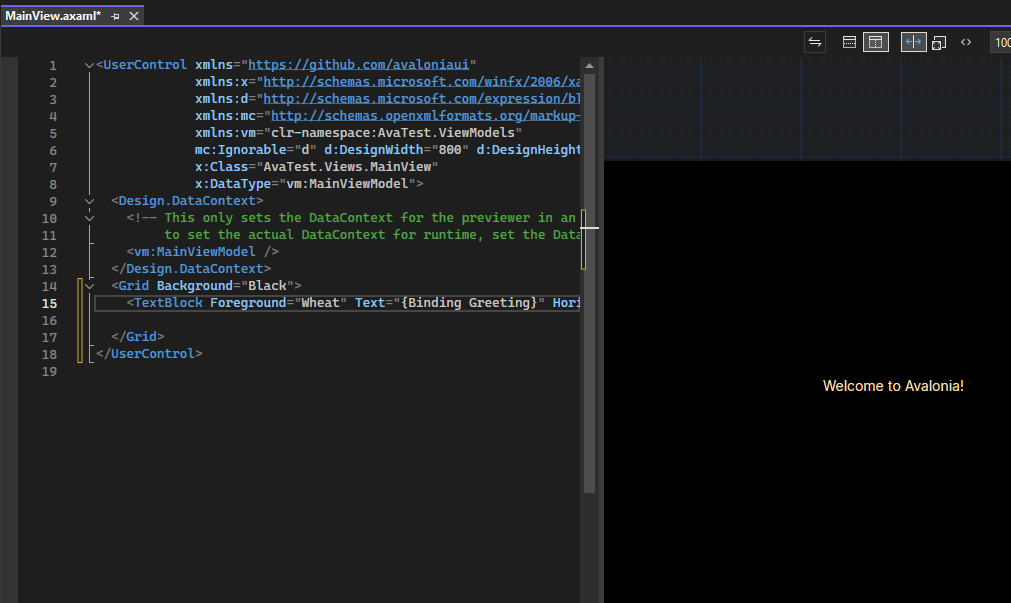
so i added this to my project, and omg it's pretty cool lol I'm cloning the repo for future use
when it works is very helpful sometimes its wonky but I never had much issues with it
right right
ok so another question. To change the task bar icon of my avalonia application in visual studio, i'd just got to my project settings, scroll down to pick an image and that was it. However, I'm using VSCode. How do I do it from there?
I dont use vscode so can't help u there sorry.
what about in rider?
found it
right click project > Properties > Application > Resources > Application icon. It's right there bottom right as soon as you click properties
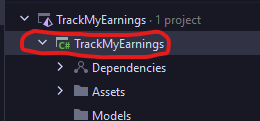
ultimately all of those settings are stored in the
.csproj
file, it might be beneficial to look those up so you don't have to rely on any built in IDE tools to change themoh I didn't know that. thanks!