Vertical Slice Architecture and adding IdentityDbContext<TUser>
https://github.com/elianora/VerticalSliceTodo/tree/feature/initial-server
I'm following along with the vertical slice architecture template and I have a few questions about changing the
AppDbContext : DbContext
to be an AppDbContext : IdentityDbContext<MyUser>
:
- Where would the right place to put identity classes such as MyUser
be? As a feature slice?
- How can I handle the change messages that I'd normally inherit from BaseEntity
on my MyUser
class since MyUser
has to inherit from IdentityUser
? I don't want to mess up the logic in EventPublisher
27 Replies
(if there is an example of using this architecture with ASP.NET identity somewhere to look at that would be useful)
Identity stuff goes in your database right?
so it goes in the Data layer (one of the few layers a VSA project will have)
Hmm in the sample they have
Common/Domain/BaseEntity
and
Feature/Todos/Domain/Todo
so I was under the impression you still vertically split the domain objects
it makes sense that it would go in the data layerthey're db models, keep them together
also, what template?
the one from this repository (
dotnet new hona-vsa
):
https://github.com/Hona/VerticalSliceArchitectureGitHub
GitHub - Hona/VerticalSliceArchitecture: A small but opinionated Ve...
A small but opinionated Vertical Slice Architecture solution template for .NET 8 & C# - Hona/VerticalSliceArchitecture
which is from this video: https://www.youtube.com/watch?v=T-EwN9UqRwE
dotnet
YouTube
Vertical Slice Architecture: How Does it Compare to Clean Architect...
Is Vertical Slice Architecture the next big thing or just as cool new kid?
Enterprise software development requires you to choose the right architecture. This session with Luke Parker will provide a realistic dive into Vertical Slice Architecture (VSA) with .NET; showcasing the potential shift from Clean Architecture (CA) to VSA.
You will lear...
oh
hm
I don't like how he's split up the dbcontext everywhere
the splitting by technical concern within features is also kinda silly imo
@viceroypenguin | 🦋🐧🌟 ping
So essentially you're saying you think the DB stuff should be a horizontal layer
that exists across vertical slices
yes
I like this image as a summary
good image
OK
I think I also don't understand the
EventPublisher
/ MediatR well enough to wrap my brain around the right way to add ASP.NET identity with it?
Like I'm sort of understanding what the code is doing but it seems weird to me that the domain object has this StagedEvents
list property on it that isn't mapped to the DBi'm not the biggest fan of it, and luke has a bit more formality than I prefer, but it's a pretty good template.
yes, DB is a layer that supports every feature. any feature can access anything in the db it needs to. there's really no reason to restrict whether a feature can access a particular table or not.
i'll be honest, i don't like MediatR. i avoid it like the plague. i like the concept of command dispatching, but using a central class for it is too much of a service-locator antipattern.
@ɐɹouɐᴉʅƎ if you have any more specific questions about VSA or how to organize, let me know. not sure what you're looking for here.
I think I grasp VSA well enough, just not the technologies that the template used
I'll try playing around with it some more to come up with more concrete questions
note that he is using immediate.handlers (which is my library), and he and i are talkign about how to migrate to immediate.apis (which is also my library)
so if you have questions about
[Handler]
, feel free to ask me anyway. 😄Will do 🙂
My goal is to get ASP.NET identity stuff to work with my project right now
and I was going to use the handler/endpoint pattern from the template for it
I think I'm getting it now
I updated the code to use
Immediate.Apis
and removed a ton of the boilerplate from that template
and removed MediatR
so now I have this folder structure: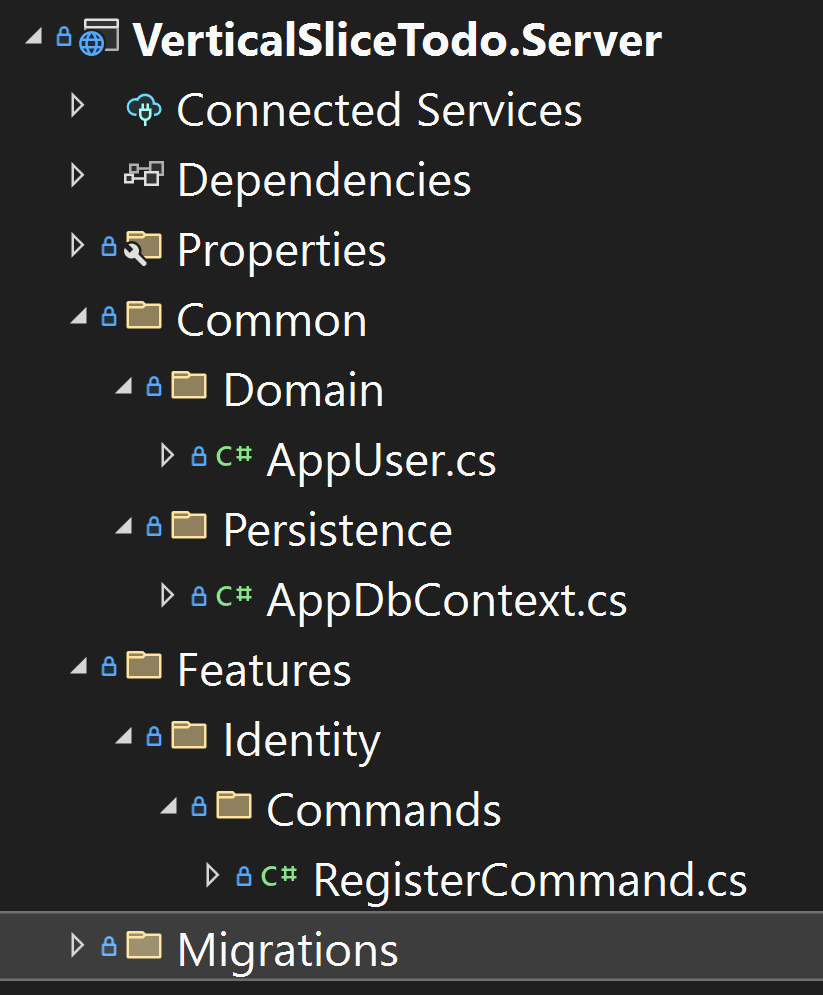
yup. very close to what i have:
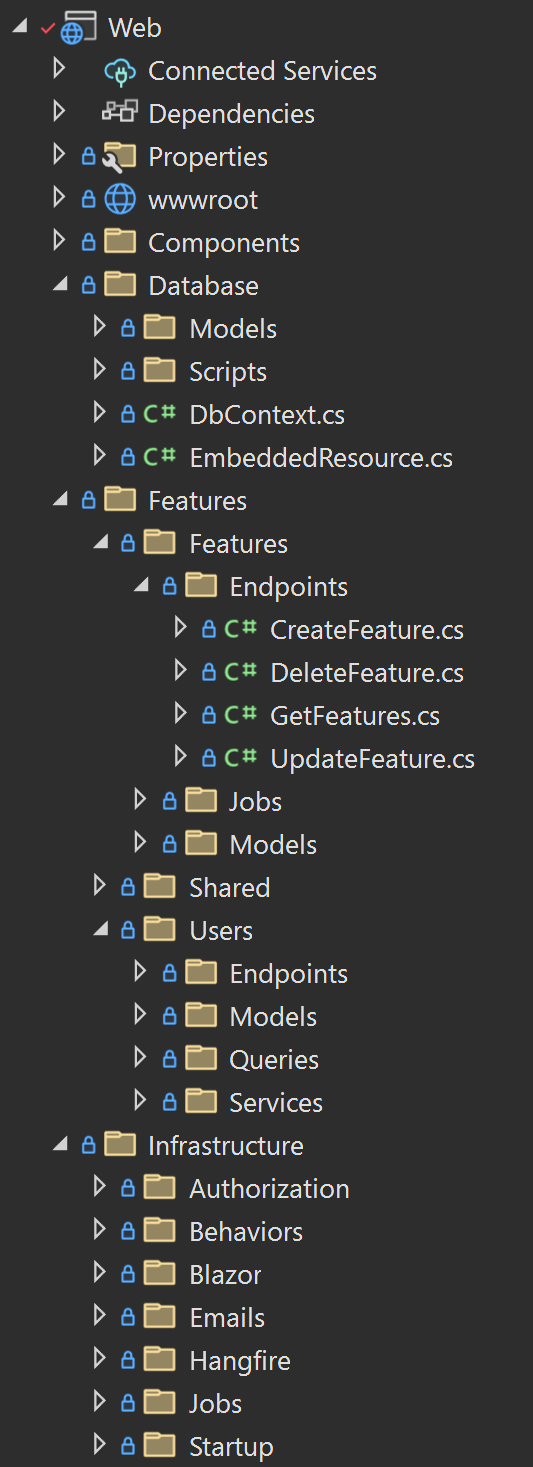
Now I just need to figure out how to actually do the auth stuff between the frontend and backend 😅
@🌟🐧🦋 | uınƃuǝdʎoɹǝɔıʌ quick question - how do I capture query params with
Immediate.Api
HttpPut
endpoints? Is there something similar to [FromQuery]
model binding for the record Command
?so currently, the query parameter is done as
[AsParameters]
, so each item inside of that is done as a parameter (i.e. it can come from query/route/body/etc., and can be marked with [FromRoute]
, etc.)
i'm pondering... if adding [FromBody]
on the object itself might make sense so that for an [HttpPost]
, you can make the whole thing a body and not have to make two objects (one for the query, and one for the [FromBody]
in my head I'd expect the API to look something like
problem is, that doesn't work well with the
Immediate.Handlers
interface
because the expectation is that there's a single parameter to HandleAsync()
, and everything else comes from DIOK
ah that makes more sense
is that how it works now? or are you saying that's how you'd like it to work?
if I'm reading this correct, this is how it works now
yes
what i would like to add is possibly:
if you only need body values
that makes sense to me