How to Create a Multi-Type List in Mojo?
Hi all!
I'm working with Mojo and need to create a list with elements of different data types (integers, strings, booleans, etc.). How can I achieve this? Are there specific collection types or methods in Mojo for mixed data types? Any guidance or documentation links would be greatly appreciated.
Thanks in advance!
17 Replies
You can try Tuple:
‘
def main():
var c = Tuple[Int, StringLiteral, Float64](1,"x", 2.2)
print(c.get1,StringLiteral)
‘
you could also try variant
fn main():
alias ListType = Variant[UInt8, Float64, StringLiteral]
var list = List[Type](6, 3.14, “b”)
you may need to explicitly convert to the variant type, and will need to import from utils.variantThis code raises an error!
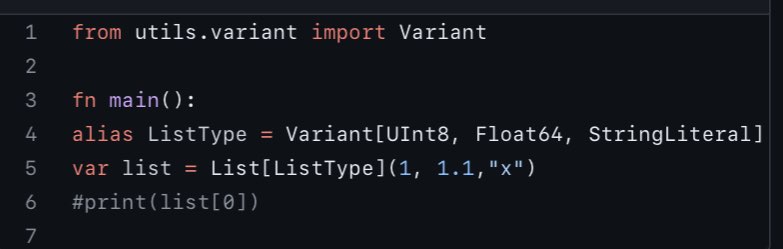
It doesn’t not compile with the mojo Playground:
https://docs.modular.com/mojo/playground
@benny For me it throws an error:
It doesn't work for
1
is converted to Int
and not UInt8
implicitly. Try this:
thanks for the fix sora, typed it on mobile :p
Thanks but it seems it's not printable.
I tried to print l, even doing a for loop on it and printing the for variable but none of them worked. Somewhere in the output errors, compiler says:
failed to infer parameter 'T', argument type 'Reference[Variant[SIMD[ui8, 1], SIMD[f64, 1], StringLiteral], 1, l]' does not conform to trait 'Stringable'
You have to dereference to print the value
List.iter() returns a Reference to each element, to extract the actual element, you must use the
[]
syntax, for example
Yes, I'm aware of dereferencing but it does not seem to work if the defined List has a variant type as the latest code Sora shared.
you you tried
item[].get[Stringable]()
The code that works will look horrible:
You could also use
x.take[...]()
to save a derefOMG, That's terrible! I don't know what the get method does and also cannot understand why in a single type list, just a dereferencing works, but here, we need such a tweak. Do you think it's going to be fixed?
Because I deeply believe that Mojo's collections are not well documented with examples or explanations and very very hard to work with. Modular wants developers to bring their ideas into Mojo but it's using Python's syntax while not keeping its beauty even after being open-sourced. I understand it's going to take a while but powerful easy-to-use datatypes are on top of priorities even before the Windows version release. Because I think we need a community-acceptable language before making it public for use. I mean it's still truly usable but not user-friendly at all in many cases…
However curious to know more about its future.
I appreciate knowing others' ideas.
P.S. Modular wants to bring a lovely language with a full dynamism basis (Python) to the language compilers world. That's wonderful and time-consuming, but are we even slowly getting there? I believe current clues about not resolved limitations show something else.
There is a certain price you need to pay for performance. Heterogeneous list is a difficult thing to optimise. Mojo might have one one day, but a better version of what we have here is also invaluable.
With enum on the road map, we can expect something like the following in the future (with place holder syntax):
If you are more dynamic leaning, something like the following may also work in the future:
But there is a price you have to pay for performance and type safety..
If you look at Swift, it's perfectly dynamically capable, and it's a statically typed language at core. So there is reason to believe that Mojo is only going to do better.
It's a bit off topic, but people have different backgrounds and would want different things from Mojo the language. I personally think "just another python" doesn't solve the problem Mojo is set out to solve: heterogeneous computing, unify Ai infrastructure etc. So, if you want to use Mojo for your daily Python use if you are not in the Ai niche, it's not going to happen for a long time.
I completely understand but things differ when people track mojo because of the revolutionary movements they state they can build. So then being Python's superset promise is a fake marketing statement when we are not going to have the available dynamism in Python right? I remember in one of the streams that Modular had, they said that they really look forward to running Python packages natively in Mojo but they are far from there at that moment. So this sight is not more than a dream when simple or complicated packages rely on this dynamism like pandas. When we can't parse them, we can't run them in now or the future, right?
It personally seems like a huge conflict between desires, modular dreams, their statements, and the reality we are facing as you also explained. I could say these to Rust core developers but it certainly matters what we expect from who. That's where the brand, the team, and their previous statements become considerable. Nevertheless, I appreciate any revolutionary movement forward for narrowing down the gap between dreams and realities.
What I can say is this: reasonable dynamism is a) certainly achievable, b) there is good precedence, c) it's on the road map, and d) we are not there yet. You can't skip ahead in time, but I also don't think the superset goal is anything fake as well.
The internal of Pandas is certainly not dynamic, it's C. And its higher level portion need not to be dynamic as well, just look at polars. It's what it is at least partially because Python doesn’t give you the choice when dynamism is not the best choice. Mojo does give you the choice.
I wonder if something like
List[Object](1, 1.1, "string")
Would work? It is the most similar to Python way