Delete user
Hi guys im trying to delete a user from the database but i keep getting 403 cant seem to find the problem
im getting 403 error in postman deleting a user with the id
this is picture of me debugging it
Note: I have the user already in my database with the id
https://cdn.discordapp.com/attachments/653632542100160547/1221828244882591804/image.png?ex=6613ff3a&is=66018a3a&hm=31741475b0eb312cc675b6459d8bac920bd2fd3628527e3a2d0803432abb650e&
53 Replies
⌛
This post has been reserved for your question.
Hey @Itsurran! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
403 means you're not authorized or using the wrong url
I'm authorized doe and have the right url
sec gonna try again
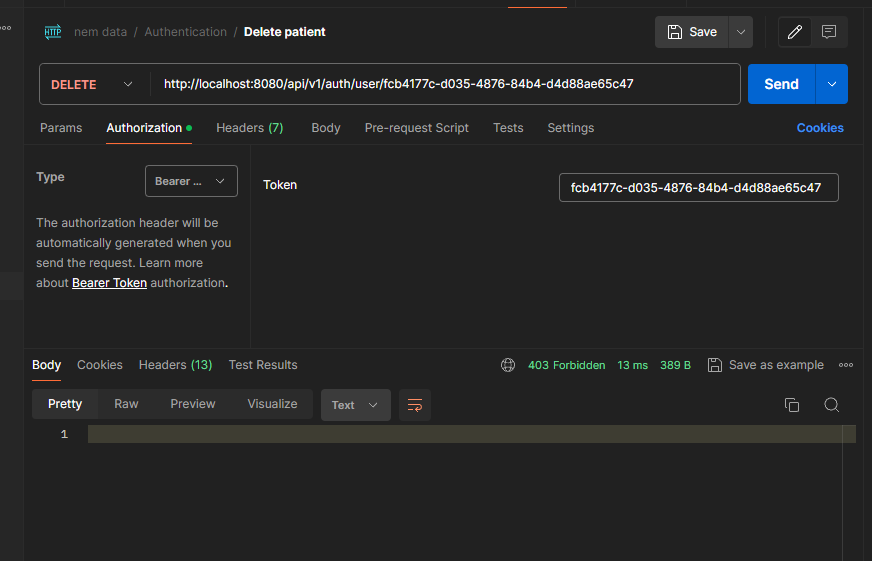
@RequestMapping("/api/v1/auth")
@DeleteMapping("/user/{id}")
Should be right url
Cant se whats wrong
Do you find anything wrong?
2024-03-25T15:55:50.516+01:00 DEBUG 23628 --- [nio-8080-exec-9] o.s.s.w.a.Http403ForbiddenEntryPoint : Pre-authenticated entry point called. Rejecting access
???????????????????????
im authorized tho
I'd need to see your code
This was in my debug console
Ah
No you're not 🙂
Bearer tokens expire
So might need to get a new one
Maybe i cant delete a user with the same user?
or?
Do you have a spring security config?
And your user controller?
I dont have user controller i have authenticationController
here is my userService
wtf can it be
Might be cors stuff
hm
No idea why you'd put that CrossOrigin header on your controller
the allowCredentials?
The entire @CrossOrigin
Because its wining in my frontend
I've never seen or used that
when i do want to register a user
or authneticating
should i do a /help
I'm out of ideas tbh
But it's usually CORS 😄
I mean it's weird you made a video trying to delete, and you didn't demonstrate that your token system is supposed to work at all, with a GET
What do you mean? he token system works since the other endpoint that require authentication gets a response
I just copied the token that i got from the authentication to use in my bearer
You didn't show that
Ok sec lemme show better
https://gyazo.com/0ffce47783ef23a9aa033482e4034f64
https://gyazo.com/e9f4f144a66da66ae606bc1da3fda525
https://gyazo.com/5aad63fe9f8322cf0ebe2da325fa228f
Sorry, gyazo doesnt let me record longer than this
buts its step for step
i register first
then i authenticate
i get the token from the database
and then try to delete
.... Try to get rather than delete
wrosk
works
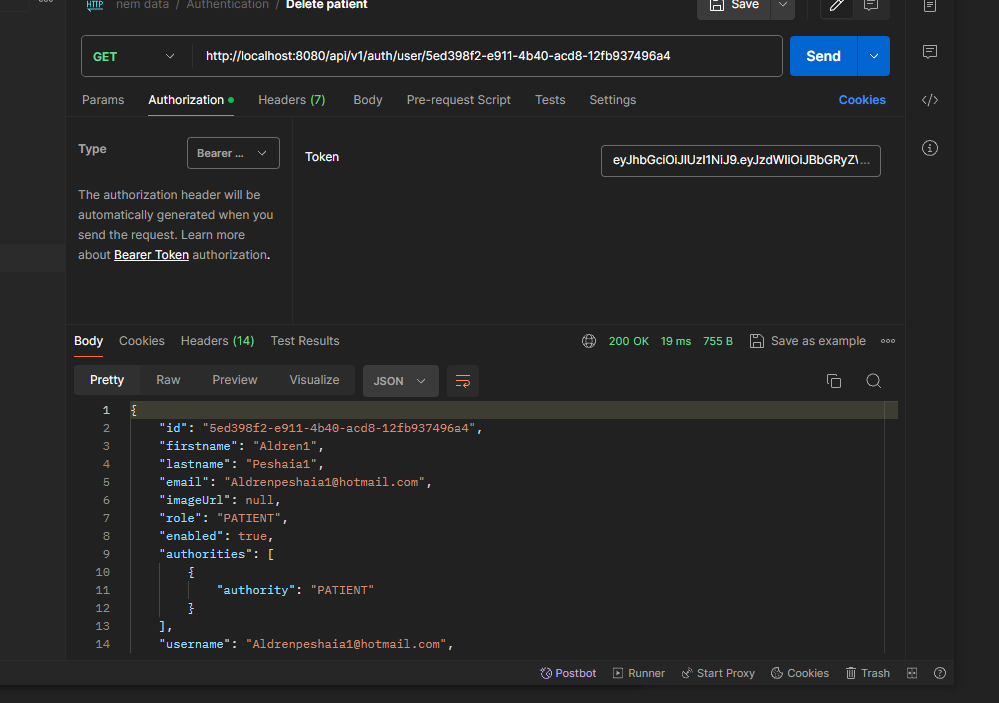
Then it can't hurt to make a summary, that mentions that GET works and not DELETE, and yes invite other people to try and help, in case they've seen weird things and have good ideas
What do you mean exatcly?
of ur last senteces
I mean that you should describe clearly your situation, such as you know the GET works, it's the DELETE, same URI same authentication, that doesn't and gives a 403 instead
True but i never tried to get since u mentioned it.
Beside that, sure, you have a right to hope for help from other people who may have good ideas
Should i try to ping?
Does it sound helpful?
If you or tjoener are out of ideas i think then yes.
Write a summary, and mention that help is still needed
Unread channels are written in bright white. People can't miss that someone needs help, they can only choose to ignore so
Thanks for the heads up
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
Can you try using verbose logging for Spring security?
and for the same endpoint, you are getting a 403 on DELETE but not on GET even though CSRF is disabled?
Yes, true.
Ok sec
Ohh i know the problem
update or delete on table "users" violates foreign key condition "fkj8rfw4x0wjjyibfqq566j4qng" for table "token"
Cant delete a user when it has a token for some reason
yes
but you can configure to delete the token with it
ahhhhhhh fk me
true
(cascading)
So like ON DELETE CASCADE?
yes
try that
yes sec
Working now! thanks @dan1st | Daniel ❤️
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
Post Closed
This post has been closed by <@265561438452580353>.