setNickName limitations
Hi everyone!
I have a bot that changes its nickname every time a websocket is updated (around 10/15 seconds) and I can't find the problem why it stops changing.
As a test, I made this method that I call every 1 second and you can see that after change #20 it stops updating.
As I checked on the Discord developers discord insert spiderman meme here the API has a limitation that "it is returned in the response headers", but I don't know how to handle it from discord.js. Can anyone help me?
Output
7 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
Hahaha
I understand that it is being filtered by Spam, and my intention is to lower the frequency... the problem is that no one tells me what the limitation is 😕
Ratelimits are dynamically assigned by the API based on current load and may change at any point.
- The scale from okay to API-spam is sliding and depends heavily on the action you are taking
- Rainbow roles, clock and counter channels, and DM'ing advertisements to all members are all examples of things that are not okay
Thank you so much! I'll look at this and come back for more help 😁
Hello, this is the solution I found so that the bot can change its nickname freely and manage the API limits:
Where
And
Thank you for your feedback! Do you have any recommendations for making a ticker style bot?
Currently my bot offers the bitcoin price near in real time
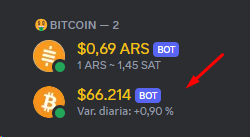
Ratelimits are dynamically assigned by the API based on current load and may change at any point.
- The scale from okay to API-spam is sliding and depends heavily on the action you are taking
- Rainbow roles, clock and counter channels, and DM'ing advertisements to all members are all examples of things that are not okay
Mmmmmmmmm..... I see
Maybe I'm abusing your cordiality and your time, but do you have any idea how to display information near real time on Discord?
Wouldn't sending 1 message every 2 or 3 seconds also be spam? 🤔
Roger that
In short: Discord is not made to display information in (near) real time