Decoding message.
hey i need help with creating a code that will decrypt a messege with a couple of rules.
firstly the original messege was changed by their letters.
each word turned into its reverse, for example "hello" turned to "olleh".
secondly every space line (between words) were changed with a random number.
as if hey man turned into hey9man ( without the first rule).
lastly it got changed with other letters. i have the dictionary to each letter.
problem is i cant solve it. i was thinking of doing something with a dictionary but cant solve the other conditions.
190 Replies
so you have written some code?
Show us what you got so far
We won't write the code for you, but we can help you figure out your problems
Are you sure your rules won't cause problems? For example, " I have 10000 ponies" may cause some ambiguity, unless you trust that the first digit before/after a given word is always meant to be a whitespace.
yeah but i bet there arent numbers in digits
or maybe if it's a fixed number of digits for the random number technically it's feasible
for sure
I would represent each "rule" as a Func<string, string> I think
So you create a function that executes one rule on a given string, and returns the result
then you can chain these rules on a string
Let me show you what i have written so far, @Fyren @dont @Pobiega
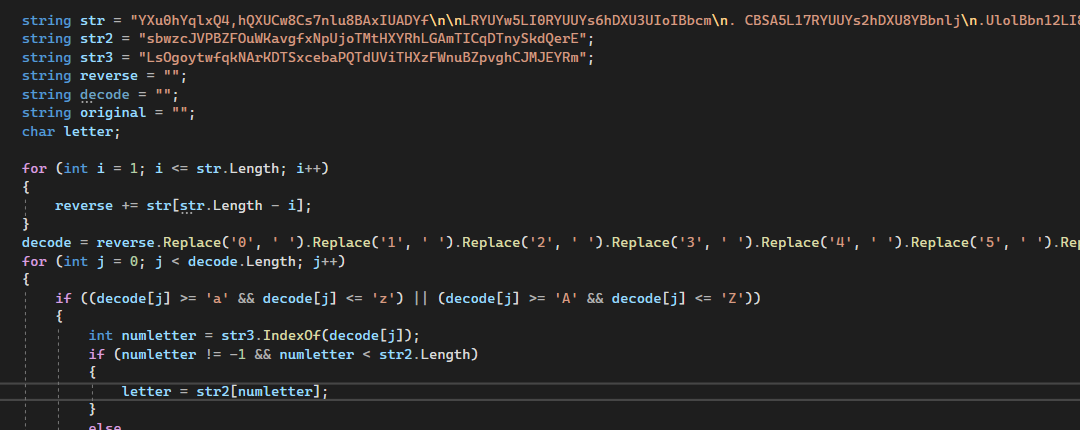
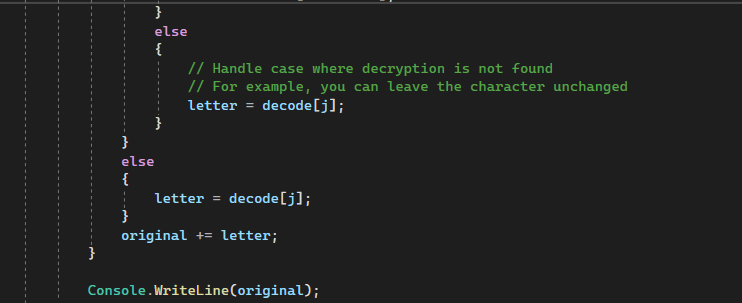
str is the incrypted message
str2 and str3 are banks of letters they act as dictionary
It looks like you're well under way to making sense of this. š What do you want feedback on?
well when i run it, the decryption seems way off
also its order looks reversed
check this out

i guess there might be an issue with str and str2 and 3
but it still has the reversed issue
I think this will be easier if we modularize it a bit. We generally try to clean up our code by making segments of code perform one single responsibility, and not multiple. This makes it both easier to understand the code and to maintain it.
"those! of more so lets"
shouldnt it be, "lets so more of those!"?
what would u suggest?
Fyren's idea of making individual methods for each step is a good one
you can then test that each step works as expected
(downloading sdk, didn't have .net on this laptop. I'll chime in in a bit)
Are you familiar with how methods work in general @Muchacho ?
i am most likely sure that the issue is with the Strings. but other than that the code looks fine logiclly i tried to run it without some lines but each and every one seems to be important as without it, it just wouldnt run or have errors. the only issue im facing is with the reversed words output
sure
you suggest that i use a method for each step?
Allright, then the gist of my idea is this:
That method performs one step of your decoding task.
i can try, i would have to agree it will be clearer
yes
the way i see it
there are 3 steps
Then you reduce the complexity of testing this - you can work on that individual segment of this task until it works as expected. like @Pobiega said
first step is to reverse the letters i suppose and the second one would be changing numbers to space lines and then decoding
i will try that :)
Allright, so - there's one helpful thing here for you
types often have static methods.
When I say
type
I mean stuff like string
, char
, int
. These are all types.char
for example has an easy way to check if a given char
is a digit, a letter, or a whitespace. This can help you with your second rule. Take a look at this:
.IsWhiteSpace(char c)
is a method that takes a char
as argument, and returns true if it is whitespace. That will let you know when you need to replace something with a digit.but i need to do the opposite tho
Yep, and that is my original concern I posted further up.
Let's say "I have 1000 ponies". that
1000
will really become 400012
or something similar (because we are reversing 1000 and adding two more digits around it)
So I guess knowing when a digit is a number and not a whitespace in disguise is part of this challenge
But still - modularize this. Tackle each challenge one step at a time, don't try to solve all problems at once. šbut there are only digits and not numbers in the incrypted code, that means that there are no actual numbers in the original message
they only act as space lines
Ah - so you are sure that every digit is a disguised whitespace?
Yes.
Okay. š Then it becomes a lot easier!
The
char.IsDigit
method lets you detect whitespaces, then you can swap them out with an actual whitespace.
Then do something like this, so you can focus on only this problem.so i can run it on the whole string and change every digit to a space line? using this char.IsDigit ?
char.IsDigit only tells you if a char is a number. And since you know that a number represents a whitespace, what it really does is tell you that you need to perform the replacement - it doesn't do the actual replacement for you, this is just the part that lets you know when to do it.
yeah
glad to know there is a method for that
A
string
is a word, right? "hi my name is" is a string. What you can see is that a string is really a lot of char
s. If you want to get technical, you can say that a string is really a collection of chars.
Are you familiar with for-loops?
or foreach?yeah sure
you can do that on a string, and perform operations on individual chars
for (int i =0; i < str[length]; i++)
{
if (Char.IsDigit) then i change that char to a " ";
changing that [i] i mean
@Pobiega suppose a stringbuilder will be a better choice here, right?
if you want to build up the new string iteratively, yes
It'd be the solution I'd be drawn towards, but I feel like I am overlooking something simpler. Whats your thoughts on this?
Don't want to implode @Muchachos mind
I'm a bit out of the loop, what particular step are we on?
Replacing any digit with whitespace in a string
hm, is that true thou? isnt it just the first and last of any chain of digits?
They assured us that the input strings do not contain numbers, and any digits found are whitespace
okay
then sure
i would go for a loop that goes fromo zero untill the str length and then for each index i would check for if char is a digit, if it is, then that char at indexer location would be changed to a spacle line if not then levae as is
SB is the right call here, but its also overkill for this usecase
so its perfectly fine to just + a string
pretty much
but instead of mutate the original string, we build a new one
i would rather do it in steps tho
it would be logical to go the oppsite way of the decryption
We will do it in steps, you're right about that š Your suggestion is correct, it's just we cannot change the original string. That's why we gotta build up a new one in parallel.
since it began by reversing words and then adding numbers and then changing the letters.
my idea would first be changing the letters back, chagning nubmers to space lines and then reversing.
i went the opposite way
oh okay yeah
okay i will try this and let yall know what happens :)
thanks
@Fyren alright
i have got it to be more organized
swell
let me show you my methods
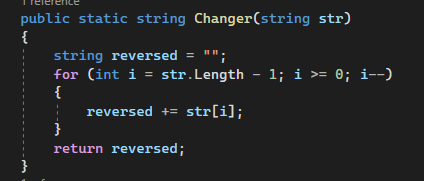
this will reverse
the string
So did you intend to reverse the entire string, or only the individual words of the string?
the entire string
this is a test
would become tset a si siht
ok goodnow this is what i call stage2
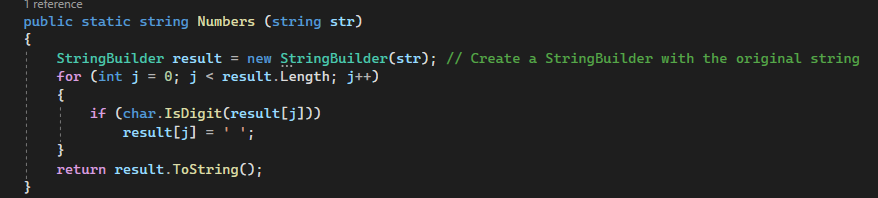
this is wat changes the digits to space lines
Ok hang on, wait a bit
I want to address something in your step 1.
How you name your methods and variables matter, a lot š When you start working with code professionally you'll have coworkers who haven't looked at your code. But when they need to look at your code, they need to understand it quickly. So we must help them to be able to do that.
One of the best ways to do that is to ensure our methods and variables accurately convey their purpose
You've named your method
Changer
. If I only look at the method declaration, i.e. this:
Then I'd be utterly confused.why?
Assume that I am your colleague. You've worked on this project towards a deadline that's on next thursday.
Then you fall ill, and I have to finalize it. To me it isn't clear what
Changer
does, and I have to step into the method and look at its implementation and exactly what it does. This takes time.
Consider this alternative:
by looking at this method declaration it's immediately clear what happens. It reverses a string, presumably the input, and returns a string - presumably the reversed one.alright then
i agree
changer however is the only "hard to get" one
This may sound and look like a minor thing, but trust me it matters a lot. If our codebase spans 50.000 lines of code and they all are this confusing, then you'll wanna punch a wall. trust me, I am there currently XD
next ones are more understandable and for the future i will write specific named methods
does numbers look alright?
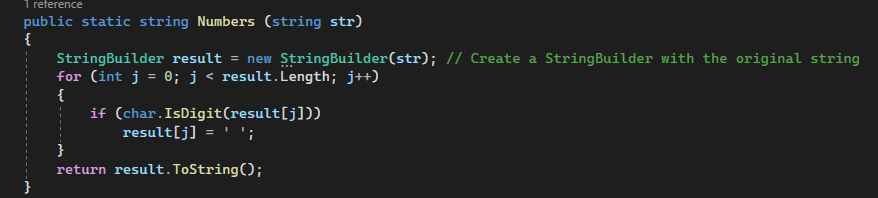
Yea I'd say so. It seems to do what you want to, naming is the only thing š
hhahah
dont worry i will change the names :)
lastly
stage 3
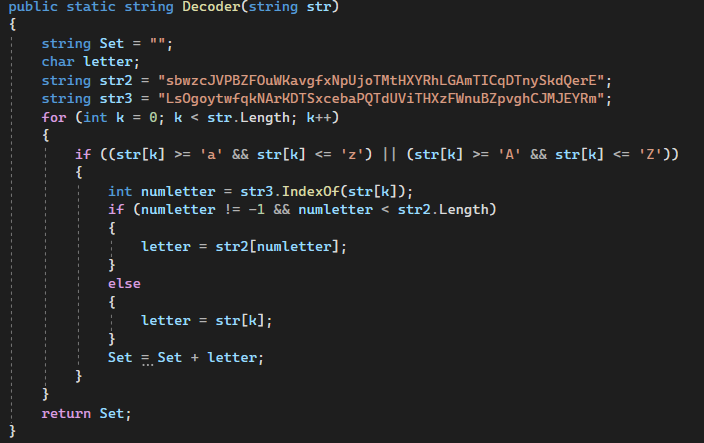
Can you in your own words write what this method does?
Also, can you copy the code in to discord here, easier for me to paste it into my editor š
firstly loops from index k to the length of the string inputed to the method. Checks to see if a char is a letter both lower and upper. after that we go to str3 where we check the postion of the letter.
we get that position and then we go str2 and change it depending on the dictionary (since the banks are in order of letter = other letter) and then so on we build a new string using the letters decoded and summing it to set which we will lasly return as a string.
ofc
public static string Decoder(string str)
{
string Set = "";
char letter;
string str2 = "sbwzcJVPBZFOuWKavgfxNpUjoTMtHXYRhLGAmTICqDTnySkdQerE";
string str3 = "LsOgoytwfqkNArKDTSxcebaPQTdUViTHXzFWnuBZpvghCJMJEYRm";
for (int k = 0; k < str.Length; k++)
{
if ((str[k] >= 'a' && str[k] <= 'z') || (str[k] >= 'A' && str[k] <= 'Z'))
{
int numletter = str3.IndexOf(str[k]);
if (numletter != -1 && numletter < str2.Length)
{
letter = str2[numletter];
}
else
{
letter = str[k];
}
Set = Set + letter;
}
}
return Set;
}
this is decoder method
Allright. So, readability is one of the most important metrics in code quality.
First of all, the code has to do its job š if it doesn't, then the rest doesn't matter. So obviously that's more important.
But readability is imo #2 on the list of important things.
Your
if
-check can be vastly simplified
lol yeah i agree i just dont know all of the shortcuts and simplifications and i do them manually. thats what i also originally did with the space and digits...
Remember, the goal when you write code that you show to a colleague is that they have no clarification-questions they need to ask you.
If they don't, it means your code is self-explanatory
That's perfectly fine - don't worry too much about this
just keep it in the back of your head as a "some day I want to do this"
yeah whenever i do write a code i would need to share i also add // and notes there to make it easier to understand.
Right - in my opinion we should add comments when we talk about why we do something. If you have both a line of code and a
//
comment explaining what the code does, then the line of code could theoretically be made clearer so that you didn't need the //
comment.
Okay, let's carry onyeah sure
alright
Generally when we refer to the position of something in a list/collection, then we call that position an index š
so numletter == index
oh
so for example:
words[1] is "age" (because indexing is 0-based, i.e. starts from 0 instead of 1)
and age has index 1 in words
so what should i change?
Ah that was just me sidetracking
Okay, can you explain the intent with str2 and str3?
You want to replace the individual characters in
str
with the letters at the same position from str2
?str2 and str3 both make the dictionary.
as lets look aat this
{ s: L, D;o and so on } i have built str2 from the first char of each group and str3 as the second char so they are built in order.
i want to replace individual chars from str using str2 ans str3, so if we run into a letter in str.
say for example a. i go for str2 and look for a, that position in str3 will be the decoded char.
Ahh, okay. So if I encounter an
s
, it should be replaced with L
?yes
and that L will be added to set
Okay, this is a great opportunity to learn a new data structure. Have you encountered
Dictionary
before?which will build the decryption string
yeah
i did
it didnt work well with us :EZ:
XD
Haha
well
Fair enough, but what you're doing here is really what a Dictionary is designed for
im sure i can use it here
but yeah
that wouldnt matter then which approach i use
although i agree
it was made for a reason
dictionary
This comes back to readability
Maybe it doesn't matter to you right now, but if you need to perform a business critical fix in 7 months and encounter code that had 0 regards for readability
then I wouldn't wanna be in your shoes
besides, some structures are heavily performance optimized for their usage
this is a way to combine your two lists into a dictionary.
i would just add at the // this is an execution instead of a dictionary method. i used it becuase it didnt work well for me in this specific case. either way this loop is designed the same as a dictionary so you can replace it or change it how you want according to the terms of blah blah as i told u//
fair, fair
could u maybe help me build a dictionary
and i can overcome my hard relaion ship with it
š
Yea, the way to do it is very straight forward actually. If you just want the quick fix, then you can use the
.Zip()
-method I did above.
switching the loop of k?
this is the manual way, where I associate
a
with b
and c
with d
Well, Zip takes two collections and merges them individually. It naively assumes both collections are the same lengthso zip would only work in my specific case
yep
and not generally
alright
Right, it's a specific tool
so i can just change the loop?
to this Dictionary<char, char> characterDictionary =
str2.Zip(str3)
.ToDictionary(pair => pair.First, pair => pair.Second);
Well no what we're doing there is just constructing an actual dictionary, whereas you're kindof doing it manually
so we havent' got to your loop yet
So, the way we use a dictionary, is we pass it a socalled
key
and get a value
in return.yep
In my example,
a
is a key that is associated with the value b
.
So if we do this:
then the value will be 'b'
and in my example ecah key is sassociated with the value just under it ( this is where i suspect zip will come to play, if they are the same length).
Yes, precisely
so you are kind of doing the dictionary operation in a complicated way
So you can use the dictionary to get the new letter for any given letter
the problem is i wasnt really given a dictionary, but just a string
That's fair - is this for an assignment?
this is some challenge my teacher gave us for our homework he didnt ask for us to hand it in or something but i loooked at it and it was very interesting so i thought i would try it
he sent us a picture and then all of the details there
problem is, it was a PICTURE
Okay. So I see - you find a matching letter in
str3
, and use the index of that to look up its replacement in str2, right
Hahaso you couldnt copy paste the texxt there
dear lord
a picture
Yeah...
i had to use some sorta scan
which i doubt is 100% correct
Okay, well this doesn't change much - you can choose to use a Dictionary, for the learning it's a perfect match to your usecase
but whats improtant to me is the logic
and to learn
thats why i tried it
it also seemed cool tho :)
yep!
i didnt know about the zip tho
so thx for letting me know about it
anyways
this is my main
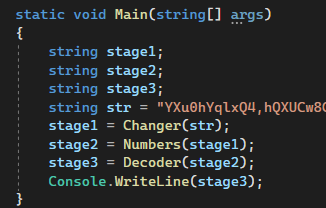
str is the encryted msg they gave us
If all of your methods there do their work, then your main method should succeed.
Oops hang on
okay - you really had this down without much of my help, so I'll just post my suggestion here
yeah that seems more effective
if i were to know some more methods this is what i would most likely do
Yea I get that impression too š
:)
somehow tho when i run it
You were on the right track, your thinking was spot on
it doesnt have space lines
RIght - I didn't test this, so idk if it works or not yet lol
Aight lets fix
What's the input and what's the expected result?
the expected result is a well understandable message with meaning, but the input is in an image so i most likely wrote it wrong
if i were to send you a png of the input text what would u do in order to make it an actual text
Oh, no I made an assumption that isn't right
We can't actually use a Dictionary here like I thought
your teacher laid a clever trap
what is it?
So a Dictionary is a thing that holds unique keys with values (don't have to be unique).
That means a dictionary can contain every unique letter as keys. So that'd be at most 26 keys for the english alphabet (its 26 right? can't remember)
but if you look at str3, that's not a unique list
we see capitalized 't' three times (
T
)i checked it
str2 and str3
have both 52 letters
which is 26*2 with upper and lower
and they both have not repetead letters
True, but your teacher has made some letters occur multiple times
really?
that proly was my mistake then
let me check
i did check tho, every letter is there once
not more than once

Or did I mix it up?
dang
is this the value, and not the key?
no no
ur right
that is my mistake
but how can i do it 100% accurate from a png?
Ha, well
oof
admirable effort
wasnt even mine lol
i scanned it in some site
but yeah
i have the png tho
Okay so just to be sure - the text I just pasted as an image, is that the reference letters? as in these letters are to be replaced with letters from the other string?
i can send you what the teacher sent us

that is probably where i made a mistake
for str2 i wrote every even char
so like wrote s, skipped L wrote b, skipped s
and for str3 i wrote every odd char, the opposite
Okay. If we assume your str2 and str3 are correct, we go back to how you were initially doing it.
sure
Do you know what happens here?
Have you seen this
? :
function before?this is similar to what i did but im not sure about the ?
This is exactly what you did, just formatted a bit differently š
this is what is called a ternary operator
which is a different style of
if-else
if referenceIndex != -1
then replacementChars[referenceIndex]
else null
the main differece is that it assigns a result immediately.i understand
but what is the difference between that and the last code
dont they achieve the same thing?
The difference is that the ternary operator is an
expression
. All expressions compute a value / return a result.
A regular if (condition) {} is a statement
. Statements don't return a value, but they do something.
I generally always favor expressions over statements, and I could go on for hours about why. But you're right, both are viable and correct approaches.
the ultra giga super short version is that expressions do one thing, whereas statements do something
and some people find the former alternative more reasonable and reliable.
... but dont think too much about that honestly, just consider it an fyi
This should work, meaning the two string lists you gave me were correct.
Does this make sense?i just got back from a little break, let me read it rq
i think it does
decodedString += replacementChar ?? currentChar;
here
Cool. I personally didn't learn
+=
or ??
until after a while, but I suspect you can guess what happens based on the previous code you had šcan u explain what this line does?
Yes
But first - since the code works, can you guess what happens?
its the alternative for my set
so i guess
Yep.
+=
is shorthand for decodedString = decodedString + somethingElse
yeah that one is known for me but what exactly is ??
So that's a default value of sorts.
If you have something that can be null, and a default fallback value that is not null, it's common practise to do
var iAmNeverNull = thisMightBeNull ?? thisIsNeverNull;
the double questionmarks ensures that if the left value is null, the right one is used insteadalright
that makes sense
this is also down to personal preference / team code style. You don't have to do this if you don't like it.
But it is more concise in my opinion.
yes
so
can u help me find a way to check my mistake
on the str2 and str3
like
this is all coming down to how well i copy
is there a way to get the textt from that image?
i guess i would have to try to do it manulally for most accuracy
grind it out I guess, 3-5 minutes of staring at the picture š
We can probably simplify it further:
okay!!
got it
the words kinda make sense
but
its still reversed somehow and there are no spacelines
look

Yep I too have an error in the substitution. Here's my result:
Seems not all letters are covered in the reference list
they are i checked
let me try
are u sure the encoded is right?
i even get something wierder
Can you send me your current str2 and str3?
string str2 = "sbwzcJVPBZFOuWKavgfxNpUjoiMtHXYRhLGAmTlCqDInySkdQerE";
string str3 = "LsOGoytwfqkNArKDlSxcebaPQIdUViTHXzFWnuBZpvghCjMJEYRm";
okay. str3 does not contain all possible letters.
This is str3 sorted:
oof
there is some thing wrong in the middle
i will check rq
also T,T?
1 sec
it only has lowercase
i
, triple uppercase TTT
no lowercase l
it says here
lowercase
should be l

what about g?
Oh, right. No capital G either
this is hella confusing me
Haha
wait a sec il resend it so i dont scroll like crazy
Well, it makes sense! We are using a list of letters to find a replacement letter. But if the initial list is incomplete, we will only have a one-way conversion

i am going to sit on this until i get this right
Allright. I need to go for dinner now, hope I didn't confuse you too much haha
I'll check back later
okay
thx
i am sending here my progress
string str2 = "sbwzcJVPBZFOuWKavgfxNpUjoiMtHXYRhLGAmTlCqDInySkdQerE";
string str3 = "LsOGoytwfqkNArKDlSxcebaPQIdUViTHXzFWnuBZpvghCjMJEYRm";
this all works
one last problem
space lines dont work and i suspect its from the Decoder method
most of my question are answered tho :)
if you have anyway of fixing this please lmk
thank you very much fro helping and for your time
holy cow 380 messages
why you call them spaces lines
it's just space
how does it not work? im not home rn, but can take a look this evening
im not really sure, the output doesnt have spaces :