Trying to get a game object to disappear and re-appear when a certain number of items are collected.
I have a game object that when you collide with it, it takes you to the next level, but i would like it so it can only be seen when all three of the items are collected.
15 Replies
(for some context i have to get the item from a different script)
i will paste the current code for it
public class itemcollection : MonoBehaviour
{
static public int item = 0;
[SerializeField] private Text itemText;
public void OnTriggerEnter2D(Collider2D collision)
{
if (collision.gameObject.CompareTag("item"))
{
Destroy(collision.gameObject);
item = item + 1;
itemText.text = "Fuel: " + item;
}
}
}
this is the script for the item
public class Finish : MonoBehaviour
{
public void Update()
{
if (itemcollection.item == 3)
{
gameObject.SetActive(true);
}
else
{
gameObject.SetActive(false);
}
}
this is what i have to make it disapper and re appear
You'll probably get more detailed answers in #game-dev, and it's been ages since I used unity, but this is one way you could try doing things:
Make a script that is attached to the items you are collecting. This script will have a reference to the goal object you are toggling visibility for.
When you collect / collide / etc with those items, your script will run and notify the script attached to your goal that you have collected an item. This could be state for individual items, or just a counter for the number you've collected.
Add logic in your goal object's script to disable/enable itself as needed based on the items collected.
thank you for that, i understand some of that, but when i say im new to c# i have so very little knowledge of it at all that I dont know how to even pass something by referance
You're not passing stuff by reference. You're using the unity inspector to assign a specific object to a variable in your script. In this case, you'd be assigning the goal object to variable on item collection script
i think my problem may be even simpler than that
when i run the code the object disappers
but when i collect all three objects it doesnt reappear
Not sure in that case since I don't know exactly how you've got your scripts setup in the scene. It's ugly, but you can add debug output in your
Finish
script to log how many items it thinks you've collected, and that might help you figure out what broke.IT HAS GONE FROM BAD TO WORSE
i have done something (im not sure what) and now the player is destroyed instead of the item
ok i have fixed it by just pasting the code above phew
can u collect more than 3?
also I dont see itemcollection or gameObject in your Finish
normally it would look something like
and set that to the object
item collection is the other script im trying to referance from'
u dont know how to do it? u got it working? do u have an updated code?
I will be back in a few and check this again
is there any chance we could hop into a call so i could try and explain it?
it isnt working, i have tried a different solution by creating a new script but when i try and drag it onto the spaceship i get this error message 'Can't add script component 'ShowSpaceship' because the script class cannot be found. Make sure that there are no compile errors and that the file name and class name match.'
(the script class has the same name as the file)
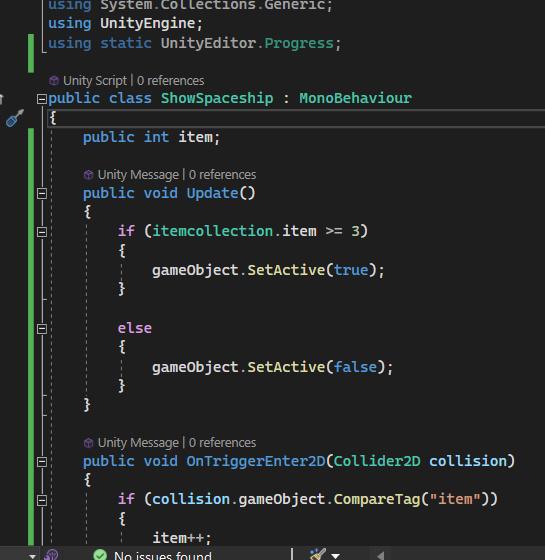
https://stackoverflow.com/a/51714475 take a look at this and see if it helps you fix the errors you're having
Stack Overflow
Can't add script component because the script class cannot be found?
Yesterday I updated unity from unity5 to 2018.2.2f1. Unity scripts are not loading after Update 2018.2.2f1.
Once I try to play the Scene the scripts are not loaded and I can't add the script again...
sorry I dont do voice chat and many here don't either
just got back will review the above
@vWest sorry on the delay, what is your object or w/e that have the itemcollection?
and did u attach ShowSpaceship to anything?