The program should connect 2 sorted arrays into 1 sorted array
The program should connect 2 sorted arrays into 1 sorted array (and sorting should be done during connection). The error of my program is that it outputs zeros instead of a connected, sorted array.
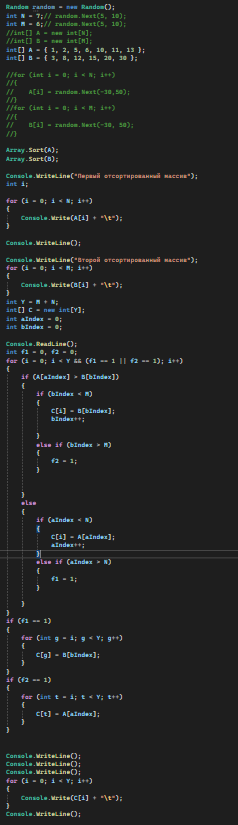
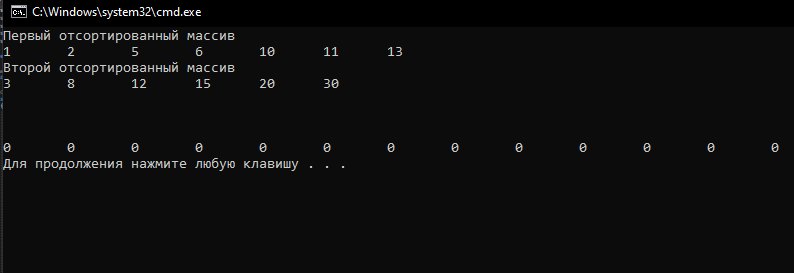
6 Replies
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/
f1 and f2 are initialised to 0. So
(f1 == 1 || f2 == 1)
will evaluate to false straight away. It's a bit hard to follow what you're trying to do with all the single character variable names.it seems like you are trying to track if one of the arrays are finished but, you don't actually need those variables
You could do something like this
since I know that the a index and the b index are going to be less than C's length, I can break as soon as one of those is finished. Then I can add whatever the remaining one has left in it. If A is exhausted, then aIndex < A.Length will be false and that else will be skipped. Then it will add the rest of what is in B.
You can shorten the body of the if/else incrementing to be like my while statements, or expand the while statement, but they should be consistent either way. If you have any questions, feel free to ping
Thank you