✅ Item Returning Null From View Model??? Avalonia
MainWindowViewModel: https://pastebin.com/v5A0u9VJ
TermsOfServiceViewModel: https://pastebin.com/BQx4qd1D
I'm not understanding why when I click the run button to debug and run the program, that's when it deciedes to tell me that the model inside of the MainWindowViewModel's TermsOfService method cannot be null?
94 Replies
what's the full error? with line numbers etc
this is all it gives me but it's not until after I click the run button
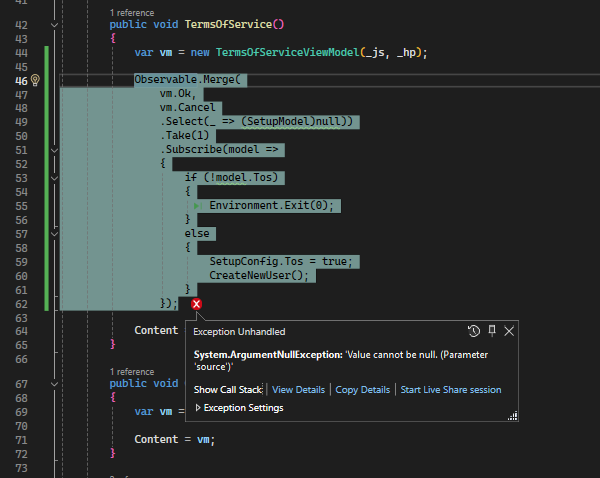
one of those methods has a parameter named
source
that you're passing null to when you shouldn't
(SetupModel)null
looks suspect to me
and the reason it's "deciding" to tell you it's null at runtime is because it's a runtime error, not a compiler error
it looks like you probably have compile time suggestions/hints about nullability, did you look at those?I don't know what those are or what they look like. apologies
ok so getting ride of the
.Select(_ => (SetupModel)nul))
like fixed it. Thanks for your helpthe green squiggles
you should do yourself a favor and add
<WarningsAsErrors>Nullable<WarningsAsErrors>
to your .csproj properties
then code that has sketchy nullability won't compile in the first placewhere does it go in the csproj file?
got it
that's making everything error.
every json serialization and it's usage beneath it is now an error
then you have a lot of sketchy nullability
as in, things that could be null that your code doesn't check for
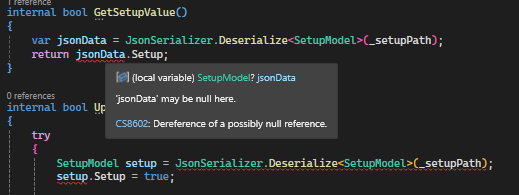
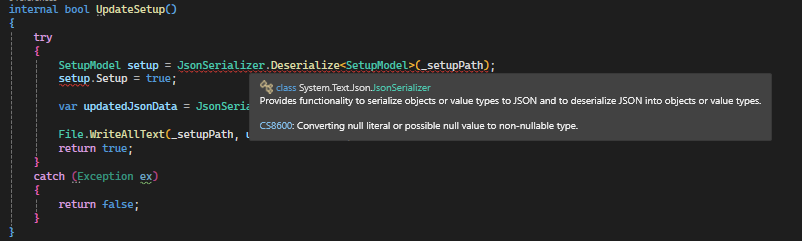
yes
deserializing json may return null, but your code doesn't check for it
they're all the same respectively
so how do I fix it?
which is a potential runtime crash
by adding null checks
ok I'll go research that
so googling this, I found https://www.thomasclaudiushuber.com/2020/03/12/c-different-ways-to-check-for-null/ is that what you're talking about?
Thomas Claudius Huber
Thomas Claudius Huber
C#: Different ways to Check for Null
What is the classic way to check if for example a parameter value is null? If you've developed with C# since a while, you might be familiar with this classic syntax: public static int CountNumberOfSInName(string name) { if (name == null) { throw new ArgumentNullException(nameof(name));…
if yes, then that means I need to build some type of universal error and exception handler
that is the gist of it
why does it mean that?
I'd rather write once and re-use 100 times than to create new handlers in each file that is going to cause this problem
the purpose of making nullable warnings errors is so you're forced to deal with them before they become NREs
ok so then how would I properly deal with the ones that are erroring in this scenario?
it depends what you want the behavior to be if the json deserializes to null
do you want to return a default value? throw a more descriptive exception?
I'd rather have it call the function to attempt to create the file and then recall itself to get the values it needs
then that's what you should do
keep in mind this is just the case where the contents of the file is literally the text
null
and doesn't account for other errors like the file not existing at all or containing invalid dataso I tried to do this with handling a null value. It calls a function to handle what I'm wanting, however, I can't apply
&&
between a SetupModel and void, which I know, but I'm not sure what to do from here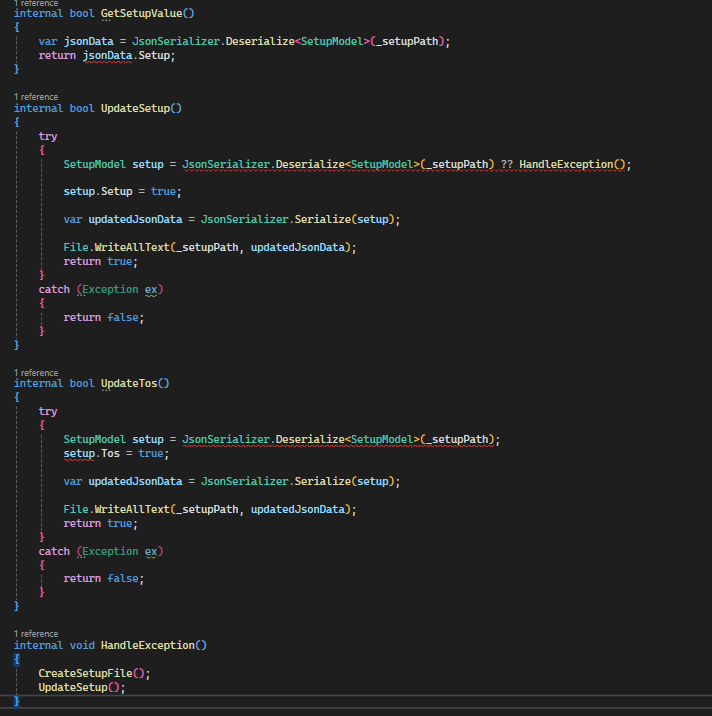
if you want to use the null coalescing operator then the right hand side has to return the same type as the left hand side
if you don't want to return something from that method, you can't use that operator
oh ok. I got you. one sec
well nvm no i don't
the only exception is if you wanted to throw an exception on the right hand side
right like
JsonSerializer.Deserialize<SetupModel>(_setupPath) ?? NullReferenceException;
, right?throw new MyException()
but yeahwould I be able to get away with something like
throw new NullReferenceException(HandleSetupException())
to have it trigger my function to do what I want it to do?no, that's not how exceptions work
oh. I'm doing my best. promise
throwing an exception basically means bailing out of the method immediately, and if it isn't caught by any calling code your program will just crash
basically for things the method isn't capable of recovering from itself
nvm. I already have it done in a try/catch
in the TermsOfServiceViewModel it's telling me that my reactive commands must contain a non-null value when exiting the constructor
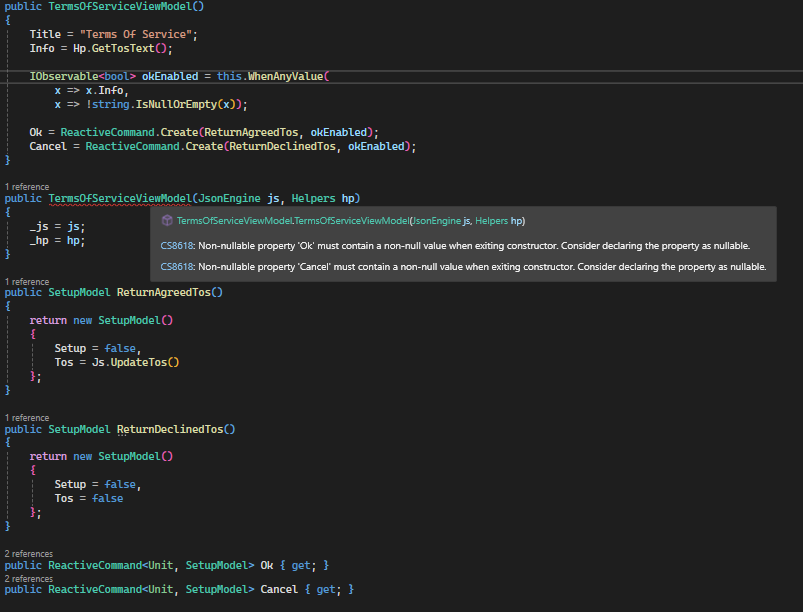
which is true if the members aren't declared as nullable
why do you have 2 constructors that are doing substantially different things?
i'm going to bed so for the last question, i'm assuming you think the parameterless constructor is called all the time but you have to do that explicitly like
TermsOfServiceViewModel(...) : this()
There are two constructors per view model because if I only do
then I get the error
and a friend of mine who works in c# and avalonia said,
"If I remember right the previewer wants to have a constructor without any parameters so you could make one that just sets the db, hp, and user to some default value but in your code you would still use the contrcutor with paramters"
but I'm back to working on these errors that popped up and I've got
oh wow. for the first one it was as simple as
got the solution from here https://stackoverflow.com/questions/76723125/dereference-of-a-possibly-null-reference-despite-checking-that-the-value-is-not#:~:text=Current%20linters%20cannot,leg!.annotation!.nodes
Stack Overflow
Dereference of a possibly null reference despite checking that the ...
I have prepared a simple C# Fiddle, which calls an OSRM map matching service URL and parses the JSON response.
My problem is, that there is a line producing the warning CS8602: Dereference of a pos...
that is not the correct solution
that simply ignores the warning, it can still be null and throw a NRE
!
simply silences the error without actually fixing the potential problem
you need to check if jsonData
is nulllike that
yes
dont know what happened there, but I meant to say sweeet
that will return that default value instead of throwing an exception
ohhhh simliar to putting default values in parameters so that the program doesn't error and can have something to go off of
yeah you could compare it to that
how far off am I? lol
that's more of a syntax thing, this is a runtime behavior thing
but they're both still "default values" if you want to think of it that way
you don't know for 100% sure if
Deserialize
will return null or not, so you have to check it before trying to access any members to avoid a NREso like my reasoning for the comparison is like ok so with checking for null values. To prevent the program from erroring off a null issue, you have it check if the object is null and if it is then you return a default value. Comparing that to the default parameters, with default parameters, if the user doesn't enter anything there, but something needs to be there, you can always have the screen error and tell the user that the input can't be empty, but you can also have a second check with default parameter so in case it does ever allow an empty value, it has a default value to use which would be like a required item
that makes sense as well
so I made the changes for checking for null values and it didn't resolve the error. what did I miss?
i don't know what errors you have based on the source code
on the line
SetupModel setup = JsonSerializer.Deserialize<SetupModel>(_setupPath);
I am still getting the error Converting null literal or possible null value to a non-nullable type
ah, you have to make the type nullable
like
SetupModel? setup = ...
or just use varoh ok. ty
now I'm having a problem on run-time with obtaining the json file. It's in
/bin/Debug/net7.0/
which is where it always goes when the program creates the file. I had this problem too when I had it set to private static readonly string _setupPath = "setup.json";
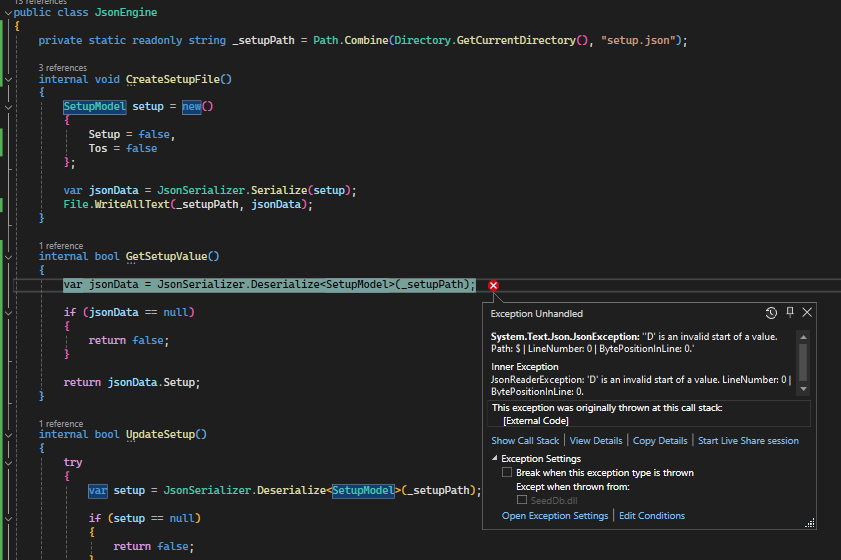
it's telling you the contents of the file are invalid json
specifically, the first character in the file is a
D
instead of the beginning of a json objectok so then did it write it wrong? This is the contents of the json file
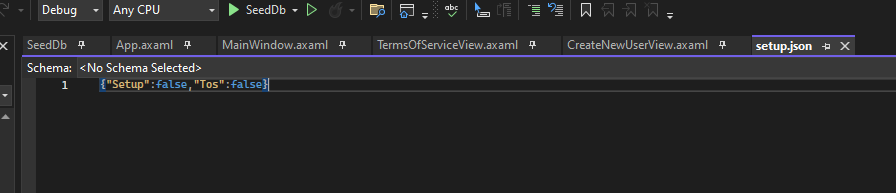
are you 100% positive that is the file it's opening?
because that doesn't start with a D and whatever it's trying to open does
it's because my programming files are on a flash drive that starts D:\
i don't see what that has to do with the contents of the file
oh
there is no overload that takes a file path
you need to pass it actual json or a stream
you lost me
you're telling it to take the literal string of "D:\My\File.json" and turn that text itself into an object
which obviously won't work
you need to either give it a string that contains the json you want to deserialize, or a
Stream
of the file you want it to readohhhhh
like that
yeah
TermsOfServiceView.axaml: https://pastebin.com/WJwxSg2n
TermsOfServiceViewModel.cs: https://pastebin.com/6vgc6Q9a
I'm getting the error
System.Xaml.XamlException: 'Unable to find public constructor for type SeedDb:SeedDb.ViewModels.TermsOfServiceViewModel() Line 12, position 4.' Line number '12' and line position '4'.
This goes back to that double constructor thing
I'm just not sure how to fix it, but I'm going to google it now
https://github.com/AvaloniaUI/Avalonia/issues/2593 it was an issue back in 2019 all the way up to 2023 but I haven't found a solution yet
9/9/22 using the double constructor was an option of a solution https://stackoverflow.com/questions/71103319/parameters-in-constructor-in-avalonia-window
and that's all I can find. I'm just going to stick with the double parameters.
so now I'm on this error and I can't find anything to help
I tried
but that threw extra errors on the MainWindowViewModel
doing that cleared up two errors, but I don't know what default is. I need default to be SetupModel.Setup = true for Ok and SetupModel.Setup = false for Canceldefault is null, so you're again just ignoring the error
Jimmacle
i'm going to bed so for the last question, i'm assuming you think the parameterless constructor is called all the time but you have to do that explicitly like
TermsOfServiceViewModel(...) : this()
Quoted by
<@901546879530172498> from #Item Returning Null From View Model??? Avalonia (click here)
React with ❌ to remove this embed.
avoid
!
unless you really understand why you'd use it, because it literally just silences the warning and will lead to runtime NREs laterwhat?
i mean in the definition of this class itself
you said
TermsOfServiceViewModel(...) : this()
so I thought that's what you meantif you want your constructor with arguments to do the stuff the parameterless constructor does too, you have to put that on the definition of the constructor with arguments
right?
no
you still want 2 constructors don't you?
oh good gravy
not if I don't have it
I don't recall having too last year anyways when I started learning avalonia
you told me why you have 2 constructors earlier
the problem you're having is that your "designer" constructor sets up your commands but your "real" constructor doesn't
right because that's what I have to do now to avoid that specific error. Last year when I started learning C# and Avalonia, I didn't have to use two constructors. For example, my first project was a Todo Project and I didn't need two constructors
which leads me to believe you want the code in the parameterless constructor to be run for all constructors
which you do by adding
: this()
to the other constructorsyes, I would rather have one constructor as you see from the snippet above then to have two, but even on my first project from last year, I didn't need to do
: this()
on it so I guess I'm just confused on why it's that way now?it's not that way now, this is just how constructors work
if you want one constructor to call another constructor this is the syntax for it
I understand that. Ok to be more specific. I didn't have to have two constructors or a constructor with
: this()
on the end of it. It worked just like shown above with zero problems. I'm confused on if "... this is jus thow constrcutors work" then why didn't it give those same errors on that project back then?i can't possibly tell you that without seeing that code
in general the avalonia designer needs a parameterless constructor so it knows how to create a viewmodel for the designer
in your current code right now, if you use the
new TermsOfServiceViewModel(_js, _hp)
constructor it will
1. not set the title
2. not set the info
3. not set up your commands
and by extension
4. not do what you want
which is why i guessed that you want the parameterless constructor's code to run for that constructor too
have you tested any of this code to confirm it works? it seems like you're writing a bunch of code that's accumulating errors that you should have noticed beforeI never had to deal with any of the errors that I've had to deal with before you gave me that line to put in the .csproj file
then you didn't test the code
because the errors i gave you are errors that would have occurred at runtime otherwise
like clicking Ok or Cancel on that viewmodel and nothing happening because the commands aren't actually set up
or getting a NRE and it crashing
or things like the title and info text not being set
ok so how do I fix these reactive commands in my TosVM? https://pastebin.com/HBxmYfwn
i told you
your 2 constructors are doing entirely different things, is that really want you want?
no
then you have to add what i suggested
I just want one constructor
well you can't have that if you want the designer to work
ok so which constructor gets
: this()
?
parameterless or otherwhich constructor needs to call the parameterless constructor?
like that?
yeah
ok so here. It was set to
var fileText = File.ReadAllText("TermsOfService.txt");
but the program couldn't find the file, so I added the private static readonly string at the top and it's still having issues finding the file. The file is actively in /bin/Debug/net7.0/
System.Xaml.XamlException: Could not find file 'D:\Programming\CurrentProjects\C#\Modules\SeedDb\TermsOfService.txt'.
not sure what you need help with on this one
either move the file to where it's looking or change where it's looking to where the file actually is
that's the part I'm struggling with and am unsure of how to fix. What I did was I right-clicked the project and selected Add > Add Existing Item. I selected the tos file from
D:\Programming\UniversalFile\law\TermsOfService_SeedDb.txt
and clicked the drop down on the file picker's window Add and selected Add As Link. I updated the tos files properties inside of vs to be AvaloniaResource and Copy If Newer, however, it wouldn't show up in the bin/Debug/net7.0 folder so I tried changing the properties of the tos file to Resource and that didn't work, but when I changed the tos file's properties to Content, it now builds the file into the bin/Debug/net7.0 folder, however, when I run my project, or I build it to get the axaml preview, it tells me that it still can't find the file
don't worry about it. I'll just use a python script to write it into the database and I'll just pull the text from the database