Create JSON objects from System.Text.Json
For a while now I've been trying to get away from dependencies that I don't need anymore, including Newtonsoft.Json, however now I have the following issue:
How could I create a JSON object like this
Usually I would have used JObject but that is no longer an option, so what's the equivalent in System.Text.Json (at best without creating a whole new Object / Class for the model)
69 Replies
creating models is honestly the simplest route... using JObject can cut corners but are as good as using dynamic and can cause more harm then good.
If u copy that JSON on visual studio and then go to Edit > Paste Special > Paste JSON as Classes it will generate all the model for you.
Its not always perfect and in most cases u might need to change some types or objects around, but for what u have above it produces:
You can use properly named properties in the C# model
we can change that
bool[]
to a List<bool>
if u feel more comfortable.
It could also be written as a Dictionary but due to the nature of your other objects being different types it would not be a good choice
yes you can use JsonPropertyName to rename the properies to whatever u want
but Since I dont know what the keys would be representing I opted not toAnd either configure the serialization to generate lowercase keys, or by annotating the properties with respective attributes that specify the target JSON name
but as an example
Just an FYI
This is just an example where u map the json name to however u prefer to name the properties
@Fabio from Human Resources let us know if that makes sense
My issue with models is that the JSON I work with is very dynamic, sometimes keys aren't even present etc
+ for libraries with a lot of REST endpoints for example I don't want to have like 200 objects that server as models
JSON is very forgiven an object not existing on the json doesn't break the model
but yeah Dictionary<Dictionary<string, object>> is what really your json is
But it might break the API request on badly written backend software
I mean if u can provide some examples I can show you how u can handle it
I don't have examples available at the moment
STJ have JsonArray, JsonObject, etc but I really think u should avoid those if possible
im sure there might be some cases u have very little options but models are things u will make once
I mostly just need something to construct a post request
For any responses or other JSON things I use Models
If you are making a request, I dont see why you wouldnt use a model you own.
because he says his requests very dynamically generated
to the point where all the keys are unknown at compile time?
to the point he doesn't want to write models for every of them
because anything other than that can be solved with models + dictionary<string,T>
but to me it doesn't make much sense because models you do it once
after u done all your models you're settle
so I am waiting for him/her to provide some examples so we have a better idea of what he is working with
👍
here is an example
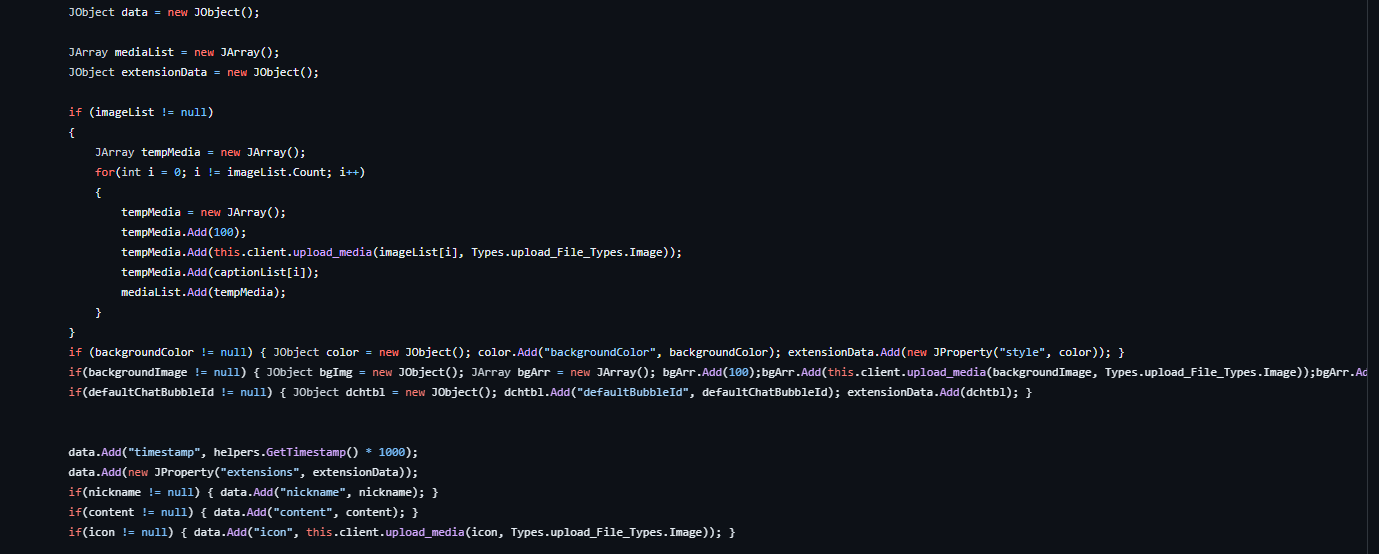
the code is ass but its like a yr old by now so :SCgetoutofmyhead:
i think that is one of the best examples i can give
IF models can be dynamic like that id gladly use them but i need something i can directly modify
sorry can you $paste it to the site below I have no means to copy from image to reuse that
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
sure thing
I'm pretty sure you can model that quite easily tbh
BlazeBin - ssahrkmzvphb
A tool for sharing your source code with the world!
also yes i know i butchered the exception thing by just throwing another one.
yeah I dont really see anything really dynamic in there
RestClient and RestRequest are part of RestSharp
all u showed above have proper naming and types
if i can make a model make certain keys just not appear i can gladly use models
cause sometimes certain keys cant exist with others etc
I see like what happens if u send an empty color for example
does it break
i think it will throw Invalid Request on HTTP Code 400
the api used in that example is very sensitive
and i want my approach to be "bulletproof"
k I will try to model this its missing a bit of information but lets see
oki
u dont happen to have the json counterpart to help visualize it right?
you mean an already constructed JSON?
yeah
i can make one
brb
also i just saw that having imagelist and caption list as 2 seperate lists is stupid when i can make it a Dictionary :SCgetoutofmyhead:
Pobiega
REPL Result: Success
Console Output
Compile: 507.351ms | Execution: 54.468ms | React with ❌ to remove this embed.
tada
key ignored
you can tell the serializer to ignore any key where the value is null
interesting
is there also a serializer option to space out the JSON?
since i also use Model classes for things like my config, id rather have my config.json file to look like this
than
yep
@leowest i think this will do
WriteIndented = true
thanks
ill test out some stuff later but i think the approach you sent here should work
models would also make the Medialist stuff easier i think
ill see
what does this give u? an URL? this.client.upload_media(icon, Types.upload_File_Types.Image)
also am I crazy you dont do anything with your media u generate it but never add it to data or extensiondata
yes client.upload_media returns an URL
I imagine it being so what this
I could probably make Style a Dicitionary there
but due to different types it contains its not a good idea
and BagroundImage also have mixed types like int, etc
so string would fit the best there given your API can accept it
the array contains the image link, the media type, the image text (like for rendering but it goes unused for anything thats not a post) and something else
wouldnt List<dynamic> or List<object> do the trick?
I mean you're not even including the media at that point
just List<string?>? at that point
hm
you have int, u have null and have urls all mixed
unless you can have a Property for the image
the only way u can go thru there is using string
using dynamic would not benefit u in anyway
oki
thanks
but yeah u still need to fine tune the models and see if the json matches
but I would say its close to what u need
and I will u tell u, that is not even the nastier JSON i've seen
there are some json that have 1 object being 2 different things like a list and a unique type
for example where u need to write custom converters to check whether its an array or not
and redirect it to the right model
a model being some weird Union of both types
and it populating the right type
I'll do some tests later, it'll probably work
Will be easier to make changes to anyways
@Pobiega anything u would change or u think its fine?
looks fine at a glance. I'd remove most if not all the
JsonPropertyName
(JsonDefaults.Web handles the casing), and use records in most places
in places where you have "if X is set, Y must not be set" scenarios, create constructors for each of those scenariosI usually keep those in case changes occur to property names but fair
Thats a valid choice. I just personally dislike the bloat 😄
mmmm like Style for example? but Style is by default there but its body may not be
yeah that one might need some tweaking, depending on exactly that should work
must the style prop be there, even if it has no body? etc
that is what I get from his code
well, if
backgroundColor
and backgroundImage
are both null, it wont be added as far as I can tell
but I dont know if thats a valid invocation of this method
in general, this code doesnt really follow the "dont make invalid states representable" ruleyeah