using namespaces and file paths in php properly
having mutiple issues with file paths and using namespaces and not sure why it seems they wont load.
here is code
50 Replies
here is model code
directory structure
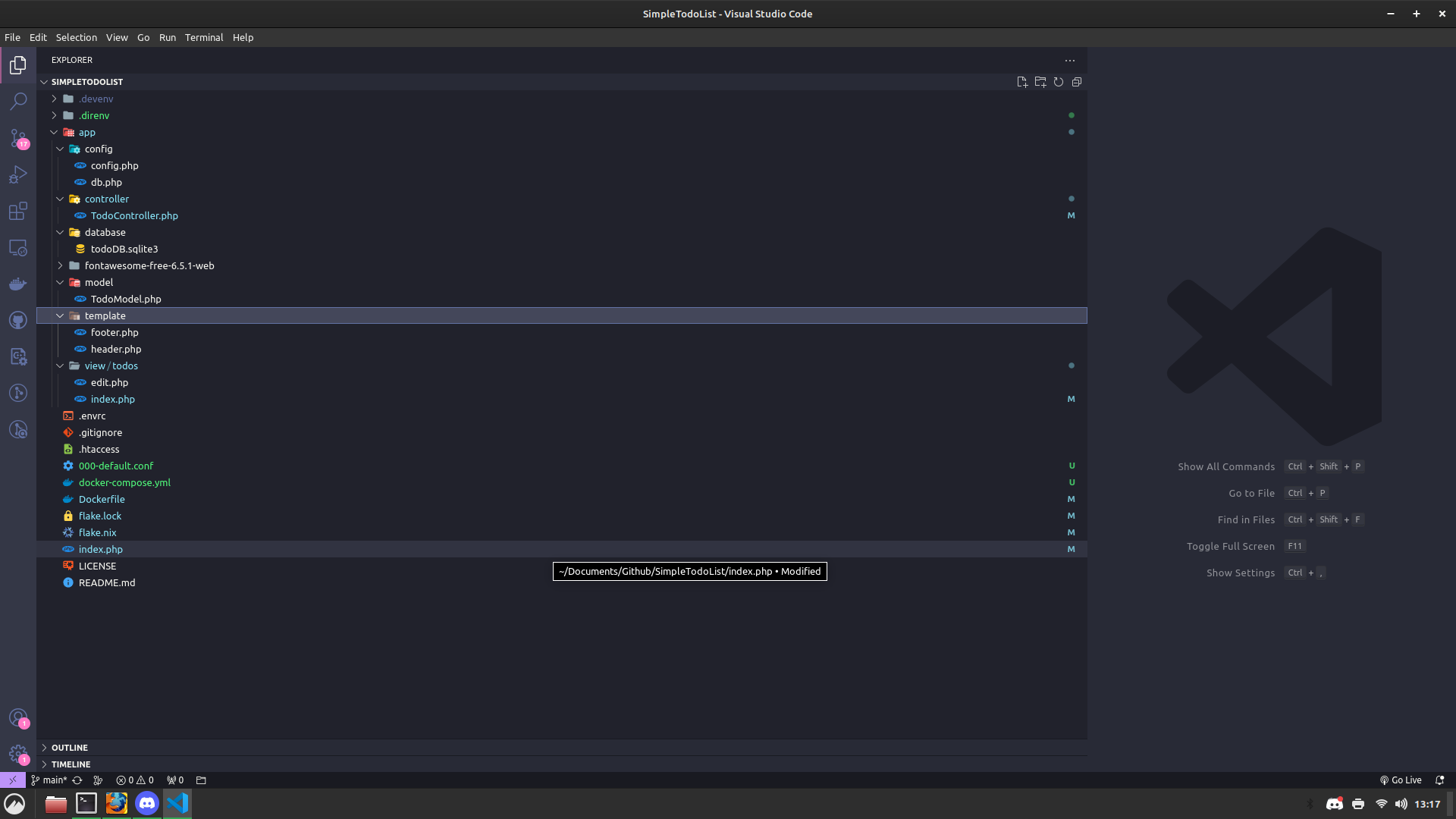
Why MD/ ? also never capitalize paths
MD/ is your project folder?
or is that some part of the namespace I'm just not keen to?
set_include_path('app/model/'); //require '../model/TodoModel.php';You realize both these paths are not even the same right?
actually I removed those
took out all the md
why did you even have it xD
that makes me think you don't understand paths at all mate
typically I don't think you would even be calling for /app/
but you would want to do /app
being at the
root/
of your projectidk how php handles that because it seems different
in go it was very easy
in php it's not
again...
paths are paths man
in php you have namespaces, require, include,
so what
ive tried them all and nothing has worked
What are you trying now since you changed the above
that's closest I got so far
it says it cant find "model\TodoModel"
that's model folder with TodoModel
both controller and model folders are in the app folder
trying
include ../model/TodoModel.php
, require ../model/TodoModel.php
and use model\TodoModel
all yield the same error
if trying set_include_path("app/model/")
and others have failed
even full paths didnt workomg mate...
you really don't understand paths
have you tried /app/model?
š
like I said
yes
it failed
/model/filename?
yep
looks like you need to learn how then š
you do know that
../
and
are not the same right?
and /
is root?yes
I use linux daily
yea I know that is why I'm laughing
cause I would think you know this shit
well php is different
paths are paths man
idk how many times I gotta say this
if remove
use model\TodoModel;
this then the extends fails
if you try any combination of include, or require with the path to the folder it still can't find it
you get errors like thisSo you have to import the correct path
b4 use will work ya?
LIke you have to give the namespace the correct path before you can use its shorthand
there's an example
right cause where is it looking for
model\TodoModel
....
you calling it from inside /app/controller yes?you cant use backslashes in keyword
use
it only allows forwardso its looking for
/app/controller/model/TodoModel
but you want /app/model/
Am I wrong in seeing this ?no that is what im trying to achieve but the way php does things im not reaching it correctly
wait I read that wrong so its doing this
use model/TodoModel
yields an unexpected ;
error so syntax fail/app/controller/app/model
xD../
would take you one level out of controllers
putting you in app
which has model
folder in itdo you need to define
__DIR__./model/
or something?yet php doesnt acknowledge it
ive tried that too
Opensource.com
Use autoloading and namespaces in PHP
PHP autoloading and namespaces provide handy conveniences with huge benefits.
read that too
Scroll down the PSR-4 bit
you need composer
Well mate idk what to tell you don't use namespaces then
it's part of php now so it's needed
All of these examples use
/
root paths thoughim used to the php 5 days this is way different it's like Java now
usually I dont have trouble with paths
this is just a weird one
its not weird you just have to fingure out your mental block
paths work the same anywhere
You just need to know how it handles the paths š
Like these do show it going outside of app too right?
idk hf with this š glad its not me
š
idk either this language has changed a lot and ive only used php 3 times in my life
mainly it's Java or C
yea mate.. your name spaces look like they need to start with \namespace
from all the examples I see
the whole
/
to \
drives me a bit nuts š¤£yeah php enforces
\
on namespacesyea idk man I can't be of anyhelp as I don't know it well enough but seems pretty clear cut š¤·āāļø
I would try an example from one these in your project
Get it working first then make your own
š
maybe jochem or epic will provide insight because im not gonna make any progress til this works
this could be all day
epic showed me with composer
that's what i know š
just never setup composer with php and apache in docker
lord even composer failed
yeah even in the same damn folder it cant find it
that's beyond me