Get first item in list
I am having a very strange bug with my List<>
The following code works as intended - resulting
tallyTotal
of first person
in possiblePeople
:
just changing the last line breaks it:
it provides the attached error (image)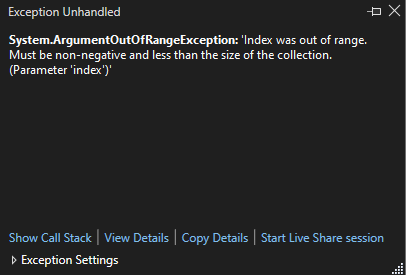
28 Replies
Does it rub the adding of items? Check with a breakpoint and checking the contents of the list once stoped
yeah verify the number of items in
possiblePeople
before using it
your code does not take the posibility of no person in people
having that tally
I would personally use var firstPerson = possiblePeople.FirstOrDefault();
and check that for null before trying to modify the valuelet me try that real quick
I don't know how it would be possible for possiblePeople to be empty, but I'll give it a go
well you should verify with the debugger, as BoxyBear suggested
I'm using visual studio, not visual studio code, as this is a WPF project
sure, I dont see how that changes anything I just said lol
VS has a very capable debugger built in
oh, I'm not too familiar with VS...
I'll have to play around with it for a bit
I'll just try this first
$debug
Tutorial: Debug C# code and inspect data - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
Ok i tested it
there definitely IS a person at index 0
i will send a series of screenshots
and
tallyTotal
is just a public int property?
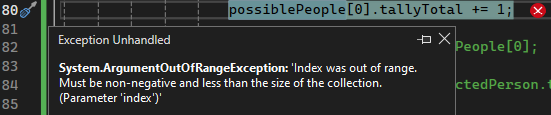
yup
if you run the program with the debugger attached but with no breakpoints, it should break when the exception is thrown (possibly thats what we are already looking at in the last screenshot)
Person object (heavily simplified)
you can then inspect the
possiblePeople
variable there
those are fields, not properties fyi
not the cause of your problem, but should be fixedoh yeah my bad
welp
it is empty
🙂
for whatever reason between these two lines of code it becomes empty

your code runs several times then
let me double check that I'm doing something very stupid here
and it works once or more, then fails at a later time
I can't imagine how that's possible
the code runs when a WPF button is pressed
just once the function is called
this project is VERY bare bones right now
hm, well unless you have shared mutable state for this class, thats the only explanation I can come up with
can you share the full source somewhere? github?
ah you're right actually
the code is nested in a foreach loop, and it looks like it does work the first few times, but then breaks at the end.
I didnt notice/forgot about the loop because it happens instantly...
woops
well tysm
🙂