OpenAPI-Swagger webflux Spring boot 3
I don't know why in my Swagger, I see only one of my two routes
31 Replies
⌛
This post has been reserved for your question.
Hey @Santo! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
Any idea please ? 😦
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
Can you show the corresponding controllers?
what exactly does it show?
You can find the source code here : https://github.com/Hunikel/Cocktail-API
This is the router (equivalent to Controllers from what I understood with ReactiveProgramming) : https://github.com/Hunikel/Cocktail-API/blob/main/src/main/java/com/santo/cocktail/router/IngredientRouter.java
Where did you specify the endpoints?
RouterFunctions.route(GET(BASE_URL) ...
Apparently this is the way today for reactive programming and endpoint declaration
oh ok
And what does the swagger UI look like?
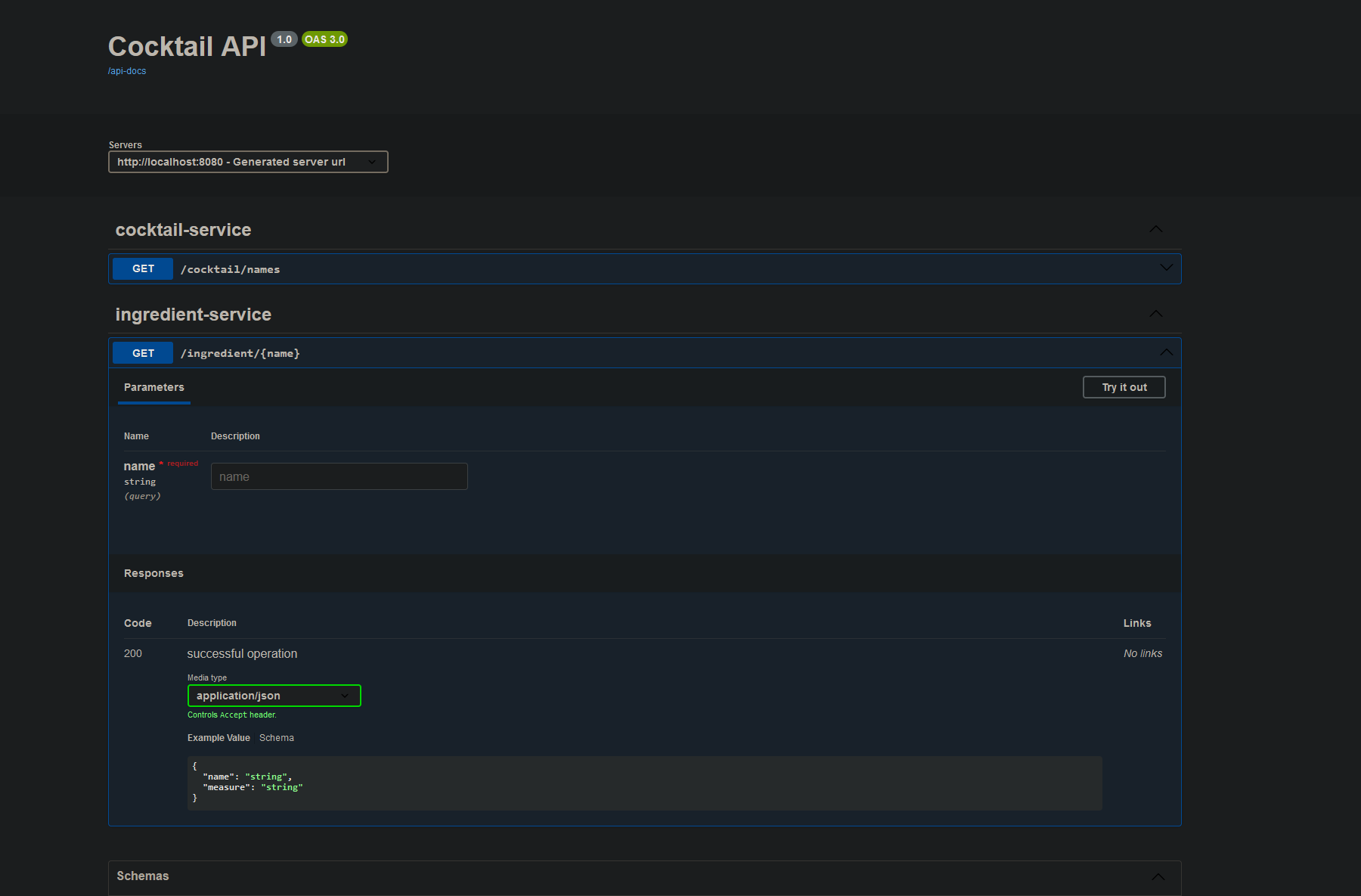
it seems to detect only one route
which route are you missing?
/ingredient
?
Does that change anything?It miss all the other routes
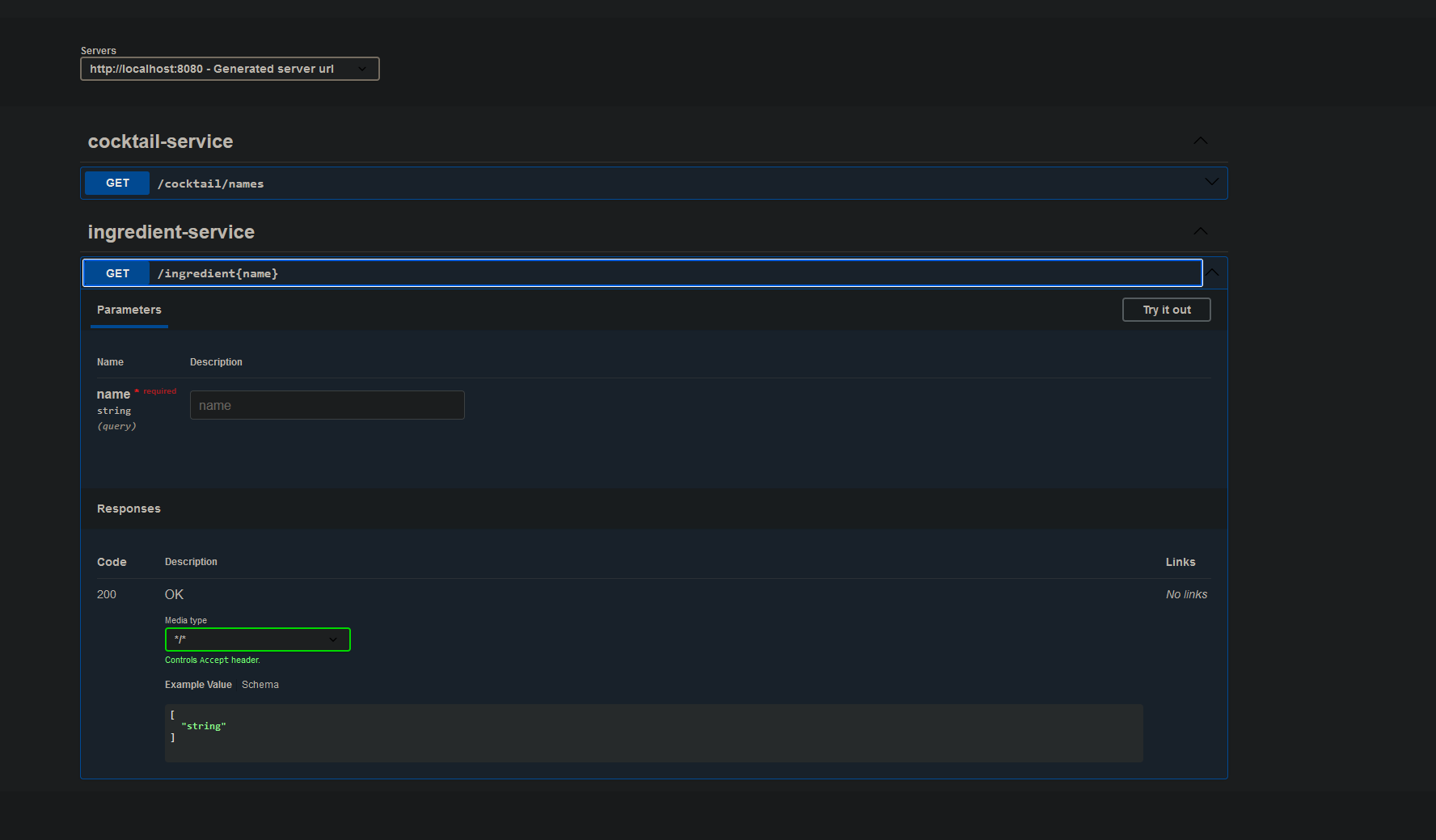
I changed
.andRoute(GET(BASE_URL + "{name}").
to .andRoute(GET(BASE_URL + "/{name}").
What if you completely remove the andRoute
line (except the ;
)?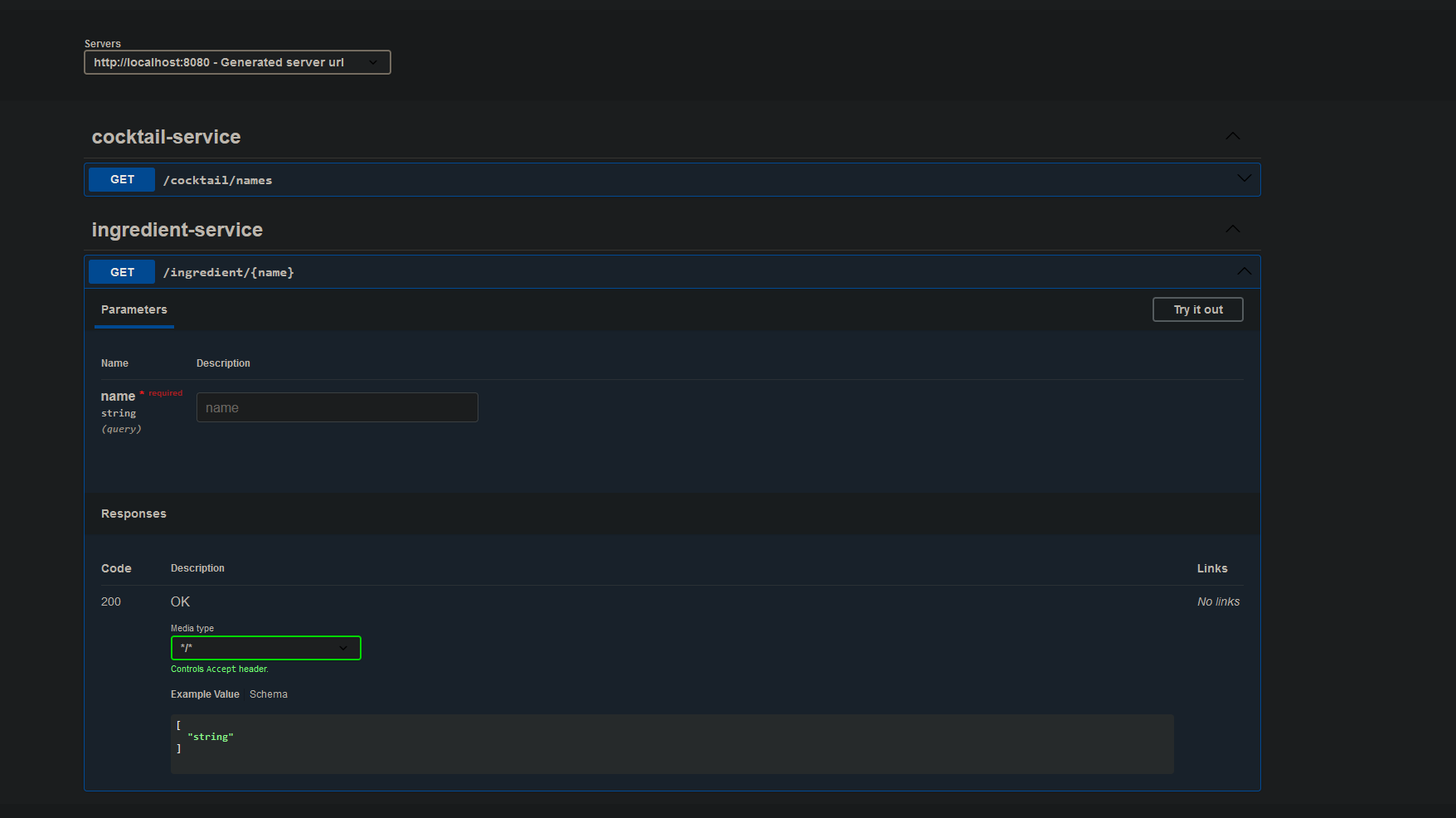
The swagger doesn't automatically detect the routes, I have to use the @Operation for it to detect it
I'm pretty sad with this compared to when the detection was automatic with RestController :/
https://springdoc.org/features.html
I tried their way here :
yeah looks like it: https://stackoverflow.com/a/62053579/10871900
Stack Overflow
How to use OpenApi annotations in spring-webflux RouterFunction end...
I am currently working on a project where I use spring functional web programming. I usually use annotations of swagger 2 in restController but with functional web programming I can not find where ...
Does it work without
RouterOperations
or not?no, even separating the RouterFunctions into multiple methods with a routeroperation each, the problem persists
Maybe you need to seet an
operationId
OpenAPI 3 Library for spring-boot
OpenAPI 3 Library for spring-boot
Library for OpenAPI 3 with spring boot projects. Is based on swagger-ui, to display the OpenAPI description.Generates automatically the OpenAPI file.
it's already there :
And does it work properly with the annotation present?
nope
Maybe it's a bug ... i'll try downgrading the version
I think you can still use controllers with reactive: https://github.com/springdoc/springdoc-openapi-demos/blob/master/springdoc-openapi-spring-boot-2-webflux/src/main/java/org/springdoc/demo/app3/controller/TweetController.java
GitHub
springdoc-openapi-demos/springdoc-openapi-spring-boot-2-webflux/src...
Demo for OpenAPI 3 with spring-boot. Contribute to springdoc/springdoc-openapi-demos development by creating an account on GitHub.
If you completely remove the second route, does the first one show up?
this is the recommanded way for spring boot 3 😅 but yeah i'll try
if i remove the second route the first one shows up yeah
Do you have different operationIds for both routes?
yes
maybe try specifying the method inside the
Operation
as wellFound the culprit ... I removed the following (beanClass and beanMethod everywhere) and now everything shows
Idk why ... normally that's what's written in the doc, or it's not up to date
Post Closed
This post has been closed by <@231028402025529344>.