Plugin system not casting properly
I'm trying to make a plugin system for my app, which does this:
The
IPlugin
instance is null, so calling plugin.Initialize()
throws an NRE. The thing is, it should work just fine, given my plugin code:
And yes, they both reference the same exact interface, defined in GyroShell.Library.Interfaces
.
Lastly, I've tried debugging this and nothing should go wrong. It tries to cast the PluginRoot
class to an IPlugin
, which should work since it properly implements the interface, but it just fails. No exceptions are thrown.40 Replies
I'd do
if (typeof(IPlugin).IsAssignableFrom(type))
Okay, I'll try this
There's not an NRE anymore, but now it just fails to cast. Once again, no exceptions are thrown, and it should work just fine
The test plugin references GyroShell.Library, so it's definitely implementing the correct interface
if you expect the cast to succeed, use a C style cast instead of
as
so you get an invalid cast exception if something unexpected happensOkay
Well
that will give you more info than it just returning null
if (typeof(IPlugin).IsAssignableFrom(type))
it never gets past here though
This is before the cast can be donethen the cast isn't failing
It thinks that you can't cast
PluginRoot
even though it implements the interface properlyAre you sure it's even processing your plugin's dll?
I believe so, when I tried debugging, the module showed up along with its respective Types
Set a breakpoint on the LoadFromAssemblyPath line, and set a breakpoint on the
typeof(IPlugin).IsAssignable...
line with a condition of type.Name == "PluginRoot"
And then check the debugger local variables for the type and see if it's correctOkay. Also, I tried the C Style cast and got a generic exception out of it:

Maybe there's a version difference since you compiled the PluginRoot and the CLR doesn't like it
do you have multiple types called
IPlugin
?nope, just one
trying this rn
@br4kejet
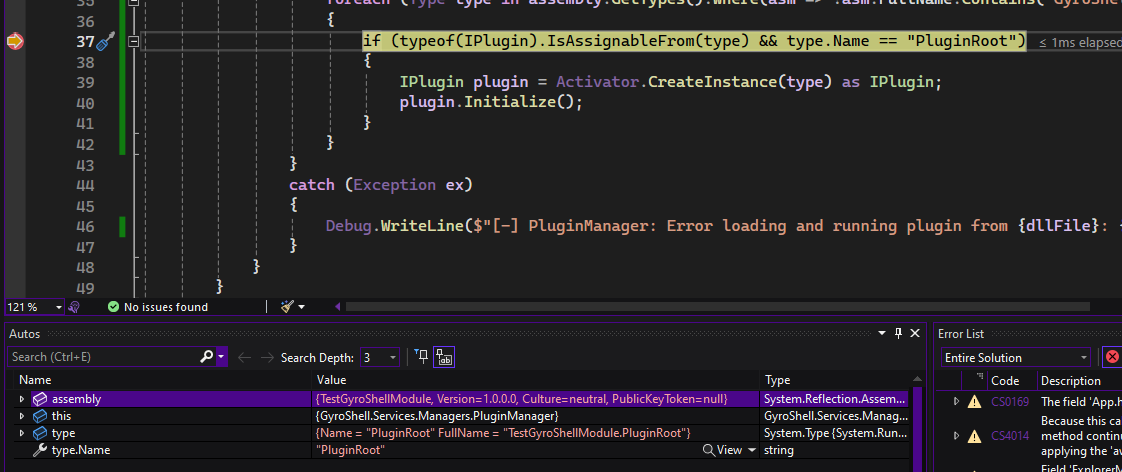
This next breakpoint is never hit

If you move next then it should try to run CreateInstance
or you could do that
It just fails at the if statement, it thinks that the PluginRoot isn't assignable to IPlugin
It won't run the CreateInstance
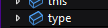
Click the little arrow thing on type
are you absolutely 100% sure there aren't multiple types in your project called
IPlugin
?Explore the interfaces
because what this is indicating is the IPlugin you're testing against and the IPlugin the type implements are different types

I'm certain that they're implementing the same interface
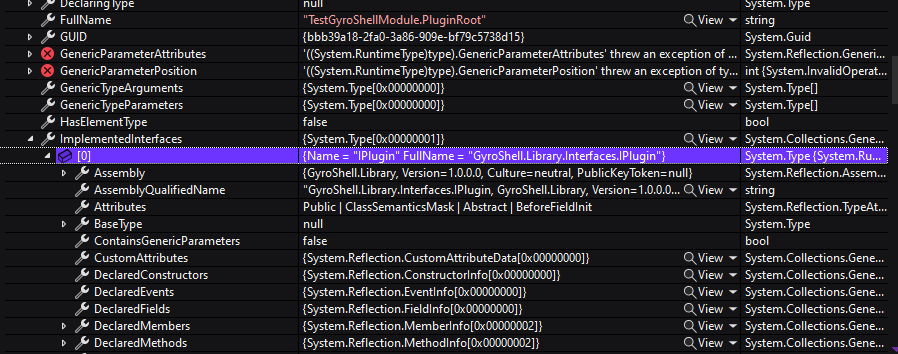
PluginRoot implements GyroShell.Library.Interfaces.IPlugin
I dunno then, the code I gave worked for me
Though I use an abstract class instead of an interface
should I do that instead?
Could try it
It doesn’t really matter which method I use, as long as it’s not some crude manual reflection thing
Then if that works try using an interface again
I’ll try an abstract later, I gotta go eat dinner
Thanks for the help though
dunno if it'll help but here's relevant code from the last plugin loader i was involved with https://github.com/TorchAPI/Torch/blob/master/Torch/Plugins/PluginManager.cs#L506-L573
Cool, I’ll look into it later. Thanks!
@br4kejet Hey, I'm back, and I tried an abstract class
same problem as before
I'll check the version of .NET and WASDK
Nope, they're on the same version of WASDK and .NET
oh wait what i just fixed it
for some reason, when the assembly loader attemped to load the file, it was also loading the reference library. i thought that was the issue earlier, but i fixed it at the wrong spot. the issue needs to be fixed before the file even loads

it's working now
weird behavior nonetheless but hey it works 🤷

there we go