How to live update chat screen when a new chat is entered to the ChatHistory context (Static list)
In the Swing application
35 Replies
⌛
This post has been reserved for your question.
Hey @Rag...JN 🌌 🦡 👽 💰! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
There are two Actors Customer and Emplyee
Employee gets a UI to chat and also the customer
This is how the chats are managed across the application
To display the Chats for each Actor I use a method
which will load the chats
but the problem is this won't live update I have to manually refresh
or manually call the loadChats method
This is the mediator that manages the chats between actors
when should what change?
Also, in most cases, you want to use
ArrayList
instead of LinkedList
When someone send a message, it should update the other user's UI
like how we chat in discord
Are they on different devices?
Are they communicating over sockets?
no Just same instance
ah ok
One Swing application
Can you show the
Chat
class?yeah
This is the customer's UI after confirming the order
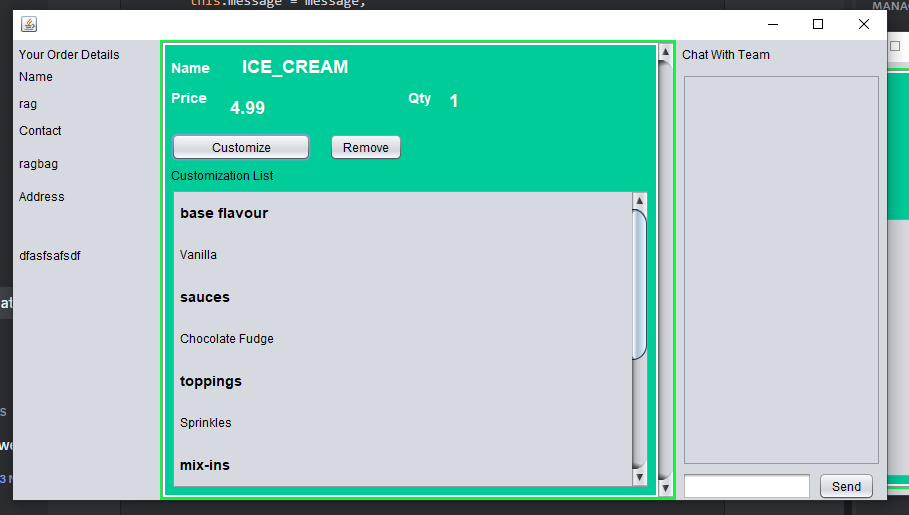
They get a Chat UI to chat
This is the UI what the employee get to chat with the customer
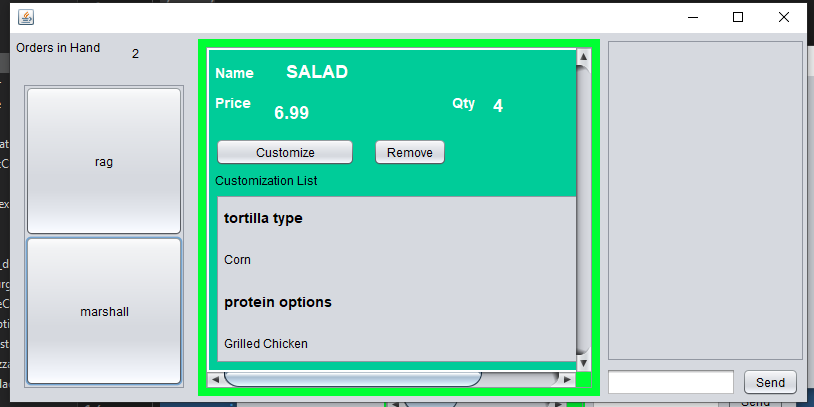
Can you show the
receiveMessage
method?Concreate Mediator calls the receiveMessage method
I meant the method itself
not how it's called
like inside the actors?
yah
What about updating the UI there?
I didn't really nothing inside
btw can you show the
Actor
interface?
I don't think you should use instanceof
Why the
?
You can just do
to.receiveMessage(message, from);
I thought about this so I thought it would be convenient in future to check the Actor instance
https://discord.com/channels/648956210850299986/1204332183209385984/1204351093434220565
What about updating the UI there?
I think the best way is to have a reference to the UI in your
ChatEmployee
(and ChatCustomer
)yah yah
and update it from there
but wouldn't that couple the UI?
you can use an interface
For example, you could create a
ChatUpdater
interface
with a method addChatMessage
or actually use a listener
that's better
You create an interface like that
because of the performance issue ?
yeah
and in your actors, you have a
List<ChatListener>
and in the receiveMessage
, you call the onChatMessageReceived
of all listeners
and the UI class can then do somethng like
ook alright
Would that work for you?
yah I will give a try and update you
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.