Trying to read from a binary file
New to c#, trying to validate a username and password from a binary file, but getting the issue 'Too many bytes in what should have been a 7-bit encoded integer. Not too familiar with binary readers and writers, can anyone help?
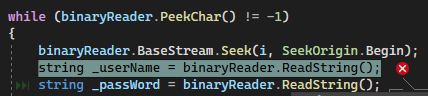
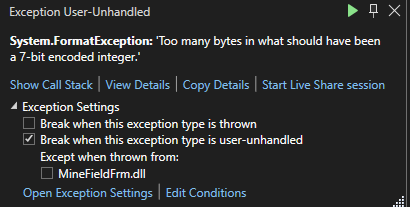
64 Replies
That some funky code there
especially since you rewind the stream before trying to read, in a loop
what is that loop and that rewind doing there?
I'm honestly not sure, haven't been taught how to do this yet and have little understanding of how it all works so this is cobbled together from the little I do know and various stack overflow questions ðŸ˜
is there a reason you're using a binary reader?
as part of the assignment we have to read and write from a binary file
alright so just walk us through exactly what you are trying to do here
yeah readstring expects the data to be in a specific format
namely length-prefixed with 7 bit characters
How was this binary file made?
BinaryWriter
?So in the binary file there are user details, username, password, then a few others. I want to take user input, which is fine, and then check it against the username and password stored in the binary file. The username written to the binary file was created by a binary writer writing a string, and the password by a binary writer writing an int (I hashed the password)
oh yeah i forgot there are multiple users details in a single file
so I need to loop through them all
can you show the write code?
so we know the format of the reading
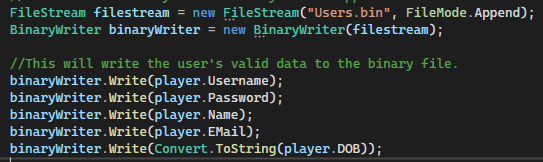
player is a class, Username is string and Password is an int
Mhm...
and you just... append to this bin file?
no lead int that tells you how many players the file contains?
yeah, this was the code we were given to do this
okay
okay, thats fine
a little less safe, but still doable
I'll begin with posting a little snippet of code
note how we must write and read in the exact same order, with the exact same types and the exact same count of things
binary wont let you skip around in the file, you need to know exactly what is where
so if you have an unknown amount of players, you'll need to read until the stream reaches its end, and you must read an entire full "player" struct at a time
right I think I'm understanding now, I use the read to iterate through what is in the file, and check against it, then move to the next line, and I need to read everything on a line before going to the next one
.. what?
maybe I'm not understanding give me a minute to see
thank you so much though this is helping a lot
write will uh... write to the file
:p
you dont wanna write when reading.
I meant to type read sorry
tired ahaha
changed it now, am i on the right tracks?
something along those lines
but there is no concept of "lines" in a binary file
its just a stream of binary data
BUT, since we know the structure of the data inside that stream, as long as we read one "block" at a time, we're good
I think i got it now, I'll probably be back here in 5 minutes to ask for me help however haha
thank you
I'm still getting this original issue
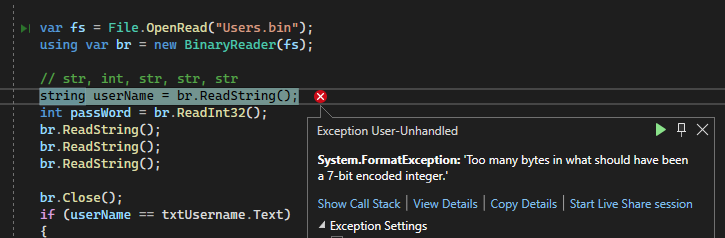
hm, your file must be malformed
I'd recommend re-generating it
ok I'll give that a go thanks
ReadString
reads a number from the file, which is how many chars to read for the string, then it reads that amount of chars. If that fails, you get this error
it most likely means your binary file didnt start with a string, or was somehow modified after it was created
binary is very hard to work with for this reason, and you cant just open the file in a text editor like with json etcthat sorted one issue and uncovered another
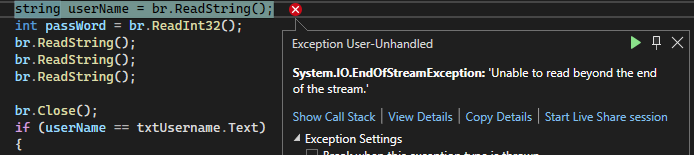
ah i opened it a few times with notepad that must have triggered it
that might be it yeah
hm, why would it try reading beyond the end of the stream...
do you have any loops or stuff going here? also when you write, you dont write any ints
you just write 5 strings
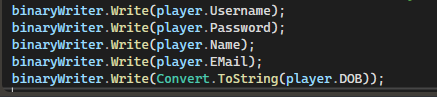
at least thats what you showed us before
player.Password is an integer
ah okay
because I hash the password
yeah I have a for loop going around it here one sec
show me da loop.
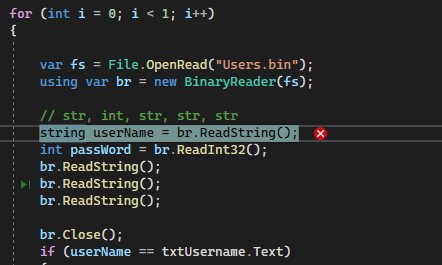
uuh
what
just one that should only run once to test
oh there are many things wrong here
you need to open the stream and reader before the loop
and close the reader AFTER the loop
also, here is a more appropriate "end of line" check:
while (br.BaseStream.Position < br.BaseStream.Length)
the "end of line" check is for the for loop yeah?
ye
yeah im still getting the same issue
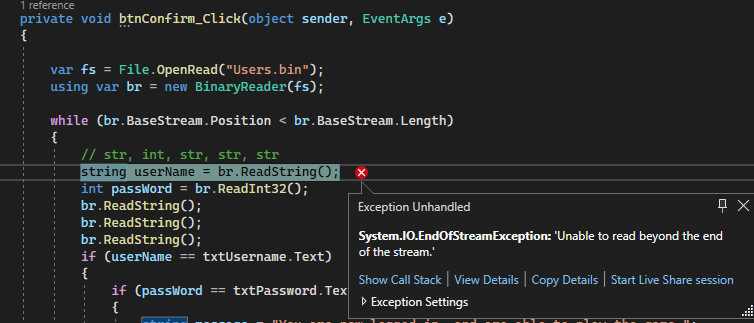
and you're sure
users.bin
is fine now?havent touched it since
hm
cause it all works fine on my end
remade it, made it by saving a blank file from notepad as a .bin in case that matters
oh no no
delete the file
don't ever open binary files with a text editor
how do i manually generate one then?
with your "write" program/method
yeah so deleted it and let it create through the program
and error still occurs
hm
might be time to install a hex editor and inspect your file
iirc vscode has one built in, if not there's an extension
not built in, but defo extensions
I personally use
ImHex
essentially turning it on and off again to see if it sorts itself
it didnt, just to check, it always accesses the file withing the project right?
and you are sure you are writing
1 string
1 int32
3 more strings, per user?
well, the file in your
bin/net8.0/
folder
if its generated and read at runtimeI'm using 5.0 for reference
not by choice
thats fine
but yeah, the file will be at
YourProjectHere\bin\Debug\net5.0\Users.bin
yeah just searched for writers and thats all I could see
wait i might be an idiot
these are words all programmers are very familiar with
damn I'm working on an older version where my username is stored as an int (I hashed it too for some reason), changed it recently but forgot to use that one ðŸ˜
let me run it now and see if it works
yeah that'd majorly fuck this up
it works!
thank you so much, sorry for wasting your time at the end there
can highly recommend imhex btw. I taught it to recognize a C# string and then my "player" structure
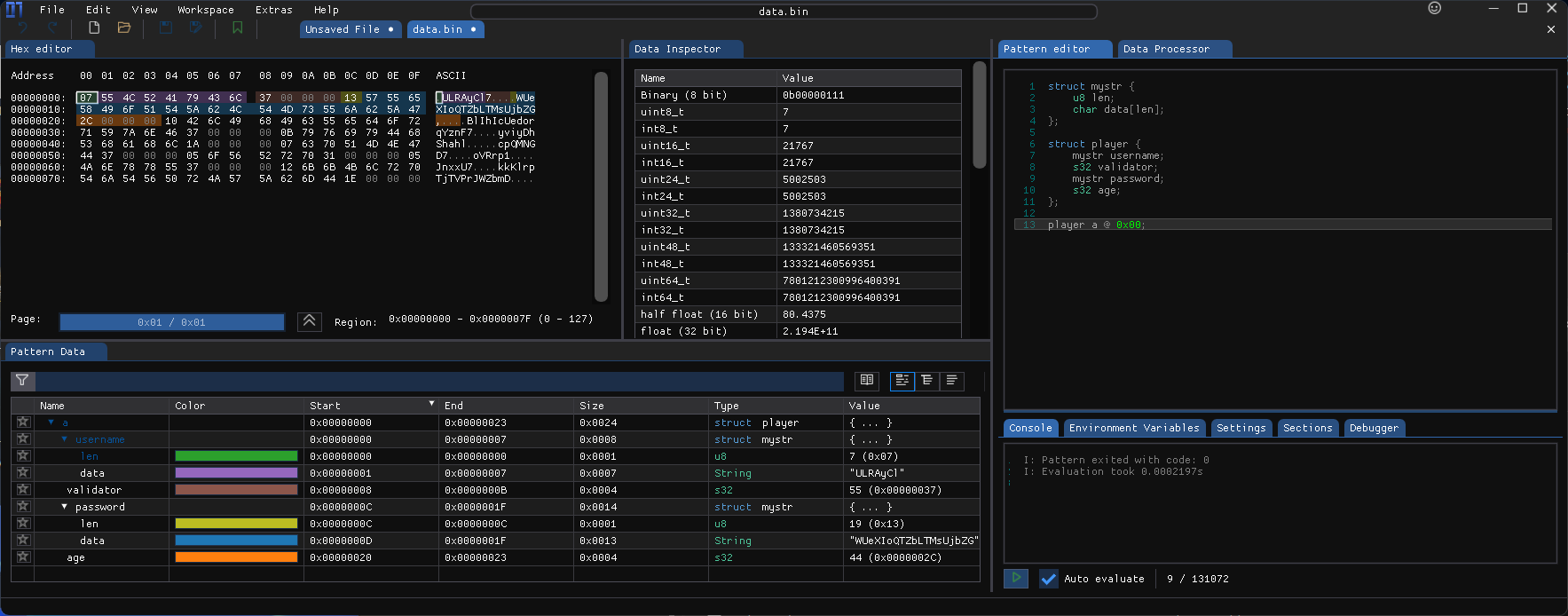