Splitting a number into equal distances (COMPLEX)
Okay the picture looks a little complicated so im going to try and explain it
I want to use HSL
HSL hue has a max value of 360
I want to divide this 360 to segments
In the code I chose 3 segments
So the first three value would be
0, 120, 240
(360 / 3), (360 / 3 * 1), (360 / 3 * 2)
Now I want to choose a 3 more colors
the best next set of unique colors are
60, 180, 300
(the middle of segment a) (middle seg b) (middle seg c)
now I want the next 3 unique colors
36 156 276
(the other side)
The yellow values are the next unique color
The purple is just a location value
lets say you want to find the Hue value for Yellow2 (first value for the second segment)
The purple value for it is 9
so its value is 9x12 (12 being 360/30 [where 30 is the amount of desired segments])
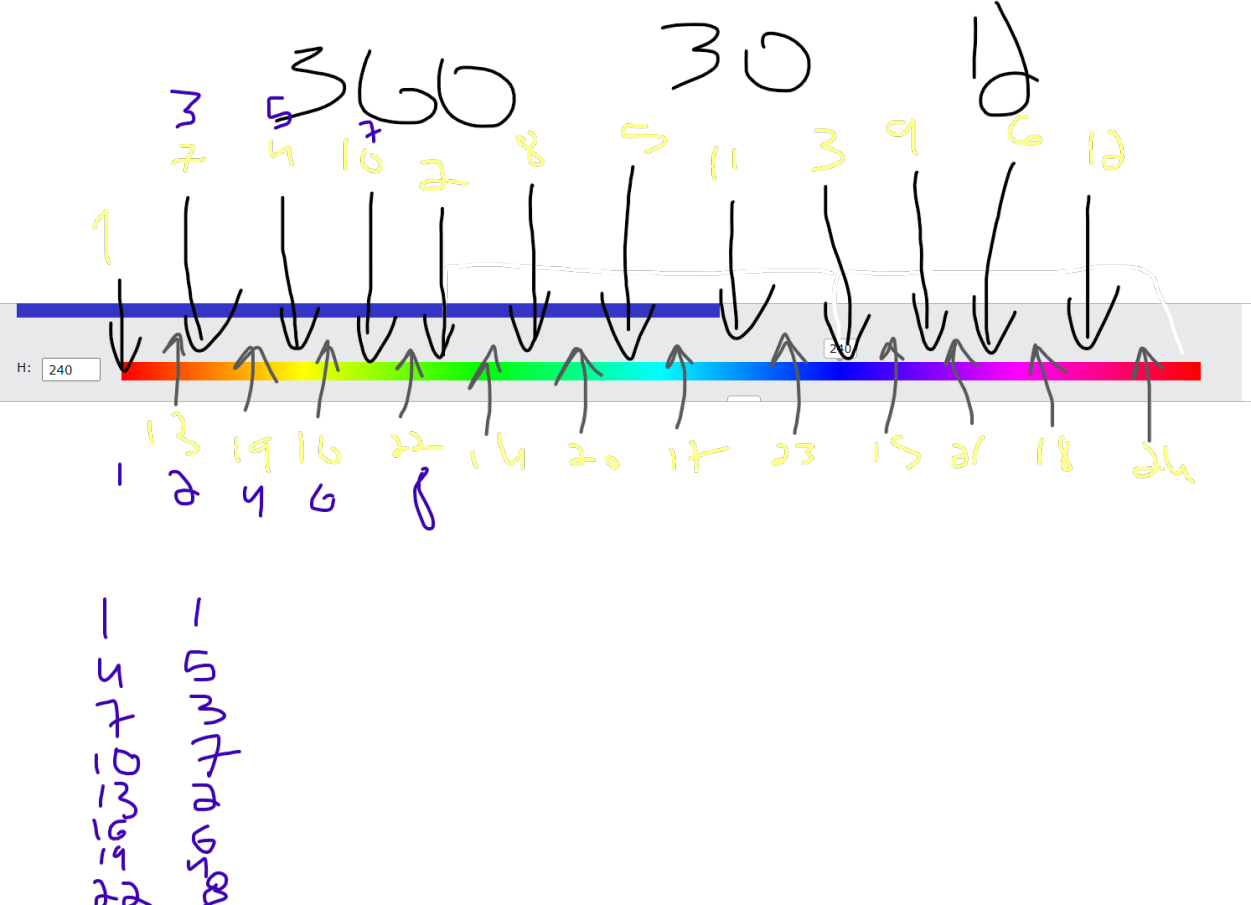
22 Replies
The code above prints
As you can see, the place where the value goes wrong is the second printed "36 156 276" which is i=5
Can anyone help me diagnose my code and make it work without my hack above?
I am open to different approaches for this as long as the colors are as unique as they can be 🙂
this started as me just wanting to find the first 30 unique values but ended as a challenge I wanted to complete.
It was so difficult to me that I decided to extend that challenge here 😛
sorry for the bad pictures it looked fine when I did it on my pc 😮
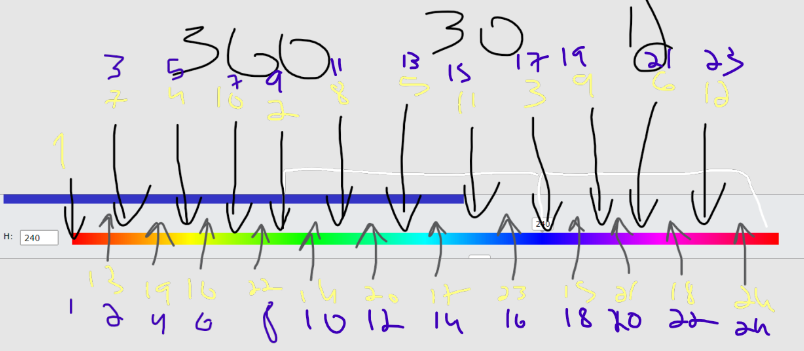
What's the purpose of doing this? You could achieve a similar result by dividing
360 / segments
and then segmentSize * i
.
Your code also isn't properly formatted.he tried
```csharpi don't know how it's not formatted, is it automatically escaped in some way?
alright working on it now 🙂
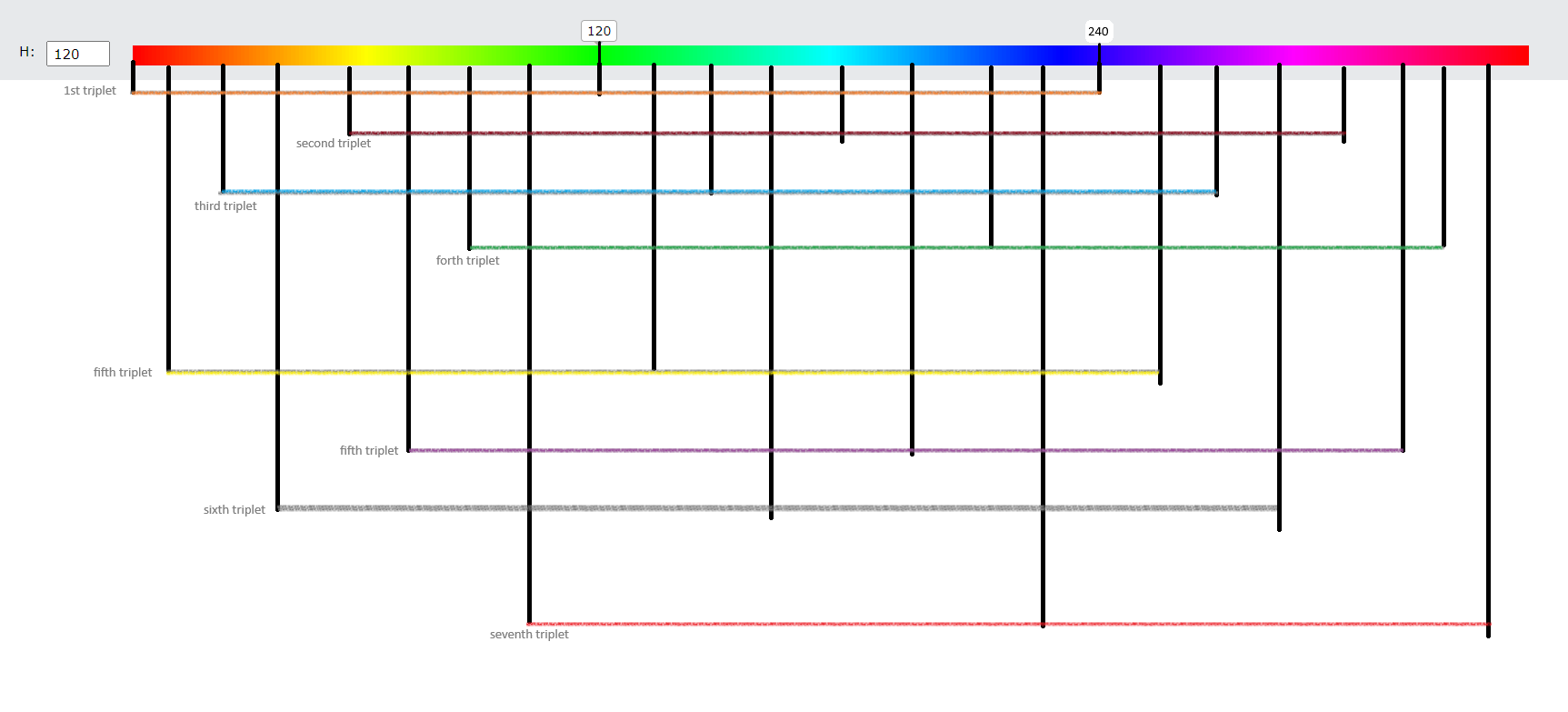
im adding numbrs now
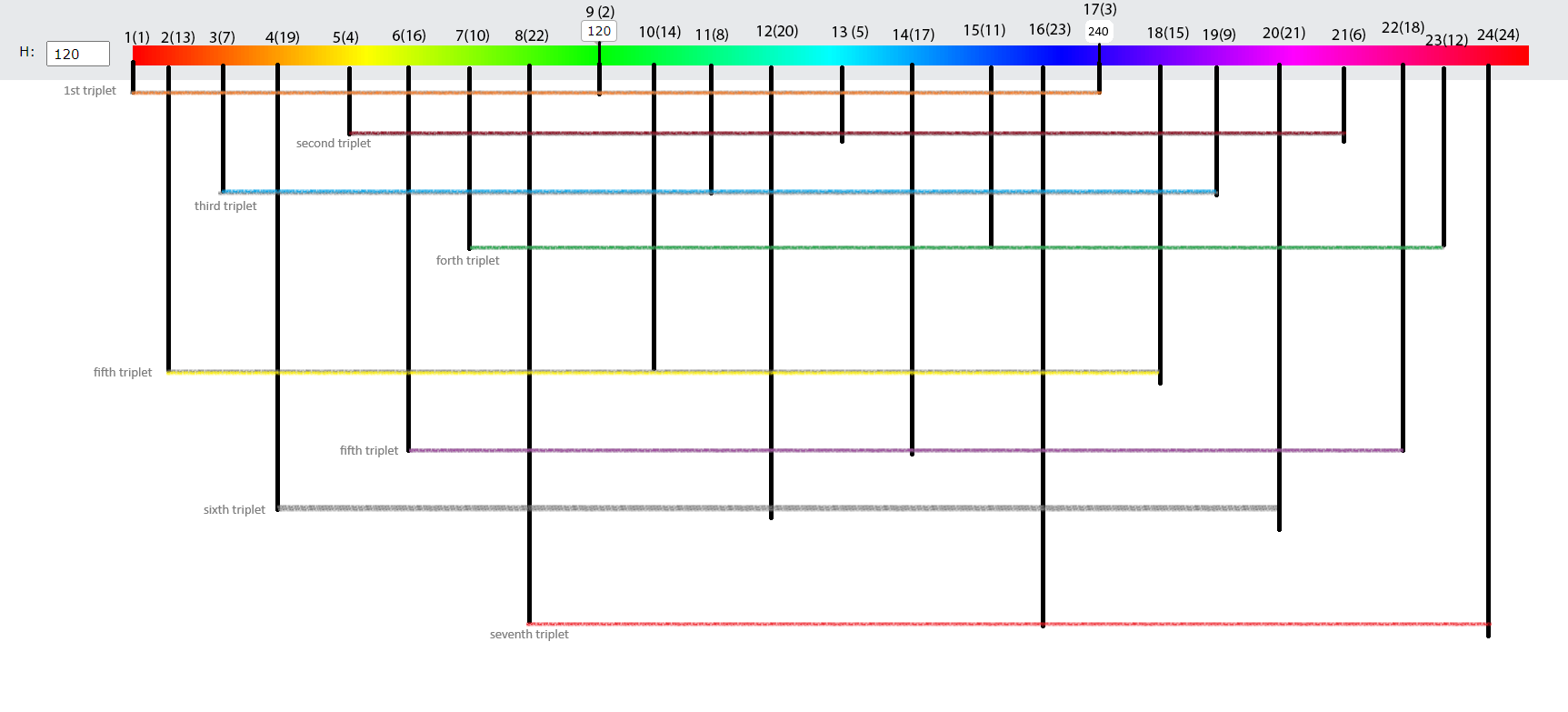
^ Testing if formatting works for me, it didnt yesterday for some reason
how would you order the different segments though?
I want to grab the next unique color
thats my problem
if I seperate the first segment
0 -> 120
where 0 has a point and 120 has a point
The next most unique color is at
60
and in your output it say its 15
does that make sense?
same, i am breaking my head over the ordering lol
i feel like it has to do with the moudulus of the first value based on the row amount
but i still havent fully wrapped my head around it
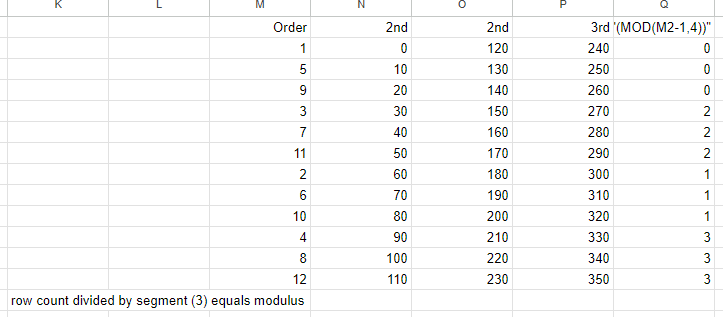
it only works on this 12 list though
i would sort the 4 groups based on the modulus
take the first 0
then the first 1
then the first 2
then the first 3
and repeat for the remaining
but I actually need the inverse of mod
because im using the sorted list to get the values
im not sure if that even works my brain is having a difficult time understanding LOL
@my profile explains my gameplay in case the above somehow helps you?
also changed your code a bit
now the segment count and point count are dynamic
oh you figured out the order? that is the part im stuck on 🙂
can I see it?
alright thank you for your time 🙂
any luck? 🙂
A
This works really great!
I have trouble modifiying it though
I want to specify the amount of points I want to return
See my above example (linking it here)
for how I changed the code so that the segment and point count are dynamic
I might want only 20 points
in which case your method returns extra colors which doesnt give the most unique colors for 20 points
the part I think its messing up on is the Math.Pow
but im having trouble understanding what its doing so I am not sure
n should be the amount of
c's
it would be better described as segments
instead of n
when I set n=2 in your code
shouldnt I expect
?
instead I get
same with n=1
i expect the result to be
instead of
not quite-
when n=5
i expect 15 numbers
5 * 3c's
meeaning 5 rows
instead I get
i should be getting
does that make sense?
what does your n mean?
i might be misunderstanding how to use your n
In my code, one component consists of 2^n segments.
I am focusing on the left component only.
- if
n=0
there is only 1 segment: 0
- if n=1
there are 2 segments: 0 and 60
- if n=2
there are 4 segments: 0, 30, 60, 90
- if n=3
there are 8 segments: 0 + 120*i/8
where 0<=i<8
.
- etcthis still fails at n=5
i should be seeing 5 rows
I made typos in the previous code, I wanted to type
Ceiling
but I typed Floor
.This is getting really close!
I think theres a calculation mistake though
If I put n=10
I expect 30 numbers
360/30 = 12
The numbers should start at 0
and all numbers should be a multiple of 12
but im seeing numbers like 67.5
This is close enough for me though, i greatly appriciate the help youve given me 🙂
At this point, having these numbers close enough to the real values is perfectly fine.
Just wanted to make that clear
Just focus on the left component, so when your
n=10
then each segment must be 120/10=12
unit apart.
But with this value, you no longer get evenly bisected segments.
Your scenario (n):
|---------------------------|
|------------|--------------|
|-------|----------|---------|
My scenario (2^n):
|---------------------------|
|------------|--------------|
|-----|------|-------|-------|i think its better to focus on the point count(amount of numbers) instead of categorizing it by segments
my original code has it by segments which mislead you possibly I apologize!
But it does not make sense to divide with your scenario. I will show the illustration.
How do you divide evenly?
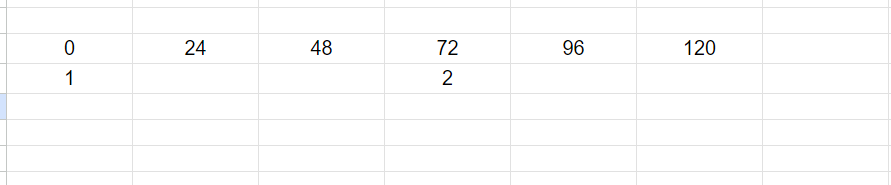
well in this case I would divide it by doing
0 24 48 72 96 120
1 4 2 5 3 6
It is not symmetrical.
In my scenario the number of dots always in the form of
1 + 2^n
its not symmetrical, but I dont want it to be
i want the most unique colors for all the points
Got a spiral sorter (ty chat gpt for the idea!)
Now I just need to connect this to the number generator 😛
oh nevermind its not sorting by MOST unique when it goes to bigger numbers ughhh
but its accurate with its numbers 😉