Interpreter Design pattern
This is what I came up with so far it has issues and wrongly used
111 Replies
ā
This post has been reserved for your question.
Hey @Rag...JN š š¦” š½ š°! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
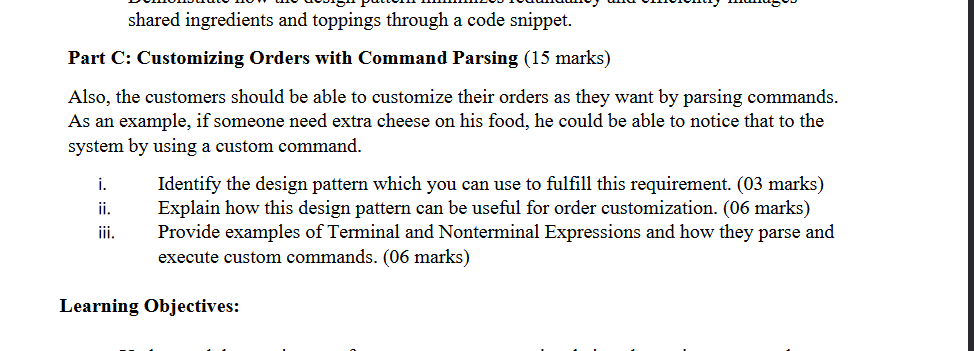
:hidethepain:
what would be the Non-terminal class would look like and its functionality here
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
I have did research on interpreter pattern
now I need some help with implementing it, like I would appreciate if you can give me some directions like we(Daniest and tjoener) had in the general chat
So the purpose of that question is to add the extra details to the food order right?
So when you click Extra Spice, and Extra Salt these two data will be attached to the Ordered Food Item
ChatGPT generated this code give a look
and the Client Usage
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
oh wait, my general discussion were about intepreter patterns
I was struggling to understand the concepts and in one example I didn't understand what postfix is
Those were related to interpreter pattern examples
This is the main question, I have to use Interpreter pattern So I started to study about interpreter pattern
now it's time to implement interpreter pattern to that question
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
yep
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
do you want to see the full question so you will get the context?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
so I guess the Customer will say
"Extra Cheese"
"Extra Spice"
"Low Oil"
"Low Spice"
These are the commands that needed to be interpreted if I understood it correctly
This is just part 1, second part is I have to actually build the Food Ordering system app in Java Swing with these patterns applied
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
so in Java We will have a Expression, TerminalExpression, NonTerminalExpression, and Context right
that's the structure right
Where does the rules/guidelines/etc comes into
can you explain with this diagram so I can easily grasp it
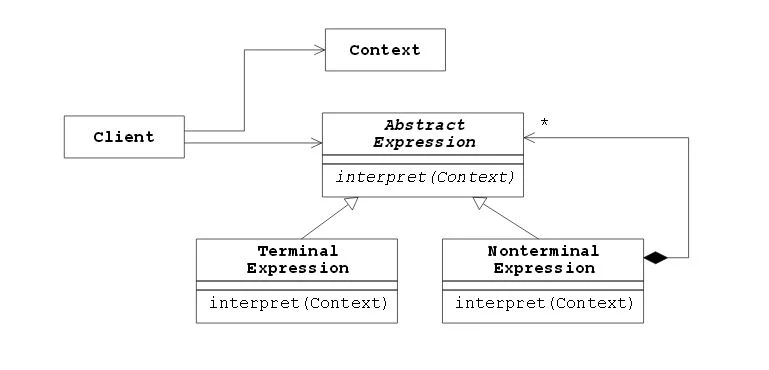
sorry if I am being rude
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
yah
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
ok
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
damn
so
extra, low, high, medium is like adjectives right
and
Cheese, Fat, Sugar, Oil, Salt are nouns
so this my syntax
correct?
adjective and noun
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
what I am expecting is much simpler expectations we can just stick to adjectives and nouns for now?
Because I am trying to think how the user will give commands
like extra cheese to an selected pizza or extra salt to a selected burger
in the Swing GUI
So if the user clicks
"Extra Cheese" button
my idea is this
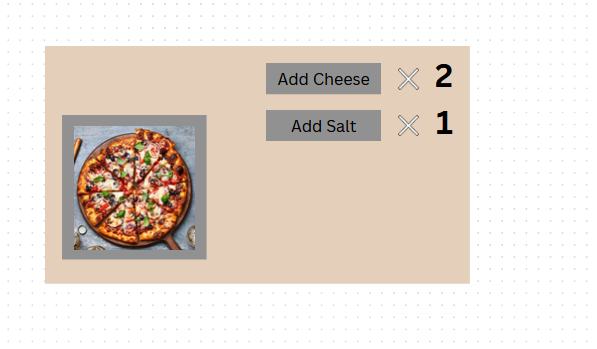
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
hmm
this is my friend's code how he implemented the interpreter pattern to his application
I hope this gives you what I am trying to interpret here :hidethepain:
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
can you explain? a little bit more
thank you so much for your time and kindness š š
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
yeah
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
t,i,c,a,o,e?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
ok?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
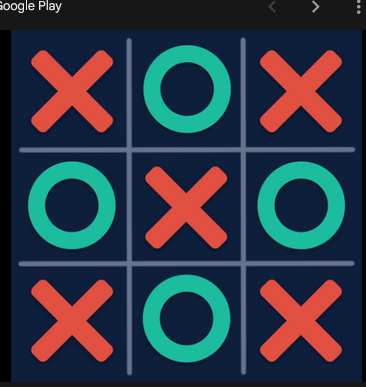
what do you mean by squares can hav 3 values?
yeah right?
if you had to create a game with System.out.printlns (with the Terminal)
how would you do it? you enter values which are strings
this is what I meant
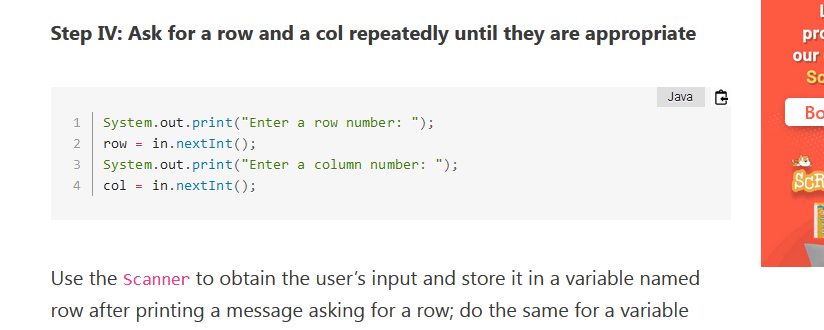
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
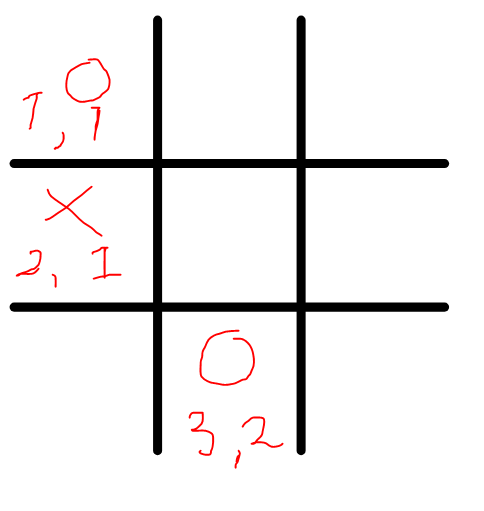
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
u just REPRESENTED the user input in a differant way
btw please have some water
if you haven'tUnknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
being able to click on the blocks means that typing numbers is not an incorrect way to play the game
did I get it wrong?
do I have ADHD? I literally processing every thing you have said. I know your concept is simple for some reason my brain can't process it
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
So I literally get it wrong?
no
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
btw I don't have ADHD like this could be a symptom
yep
Like you have the details of the car
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
no, the picture comes under the details of the car
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
ok that is a rule?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
ok ok yah
the photo is a representation of a car
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
what is the question here?
yah it also represents the car but it represents the exact details
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
Photo
I mean number plate or VIN will you some unique details but it's not enough to show it to your friend
hey this is my car and you show the Photo of the car not the number plate
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
no it's subjective
to show it to a friend online you represent the car with a Photo, to government work you must need a VIN and Number plate
even that some government related papers ask for vehicle color and stuff
Photo is not only 'correct' way to represent a car
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
The interpreter pattern is you defining how you will represent things
So I am representing the Car with Number Plate and Photo
Others can Communicate with your program regarding those things
They can read and get data from my photo and number plate
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
alright
š§
you can represent it in many ways
but others has to understand how you represented the thing?
so that's why in order to use/communicate your program they have to learn the syntax
ok where are we going finally?
you can hit me up if you are tired I can wait
===================
is this pattern does interpret?
https://www.tutorialspoint.com/design_pattern/interpreter_pattern.htm
Design Patterns - Interpreter Pattern
Design Patterns Interpreter Pattern - Interpreter pattern provides a way to evaluate language grammar or expression. This type of pattern comes under behavioral pattern. This pattern involves implementing an expression interface which tells to interpret a particular context. This pattern is used in SQL parsing, symbol processing engine
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
??
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
any reviews on this example
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
I have no idea what it interprets tbh
ok it tries interpret John
a male name in general
so if I inserted a lot of male names
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
yah
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
ok
It would be like
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
it's just a fixed method right?
the user has the option to enter any of the male names that are mentioned there?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
alright
can we try another approach?
would you try out your solution for original question in abstract way not too much detail then we can discuss it?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
like this?
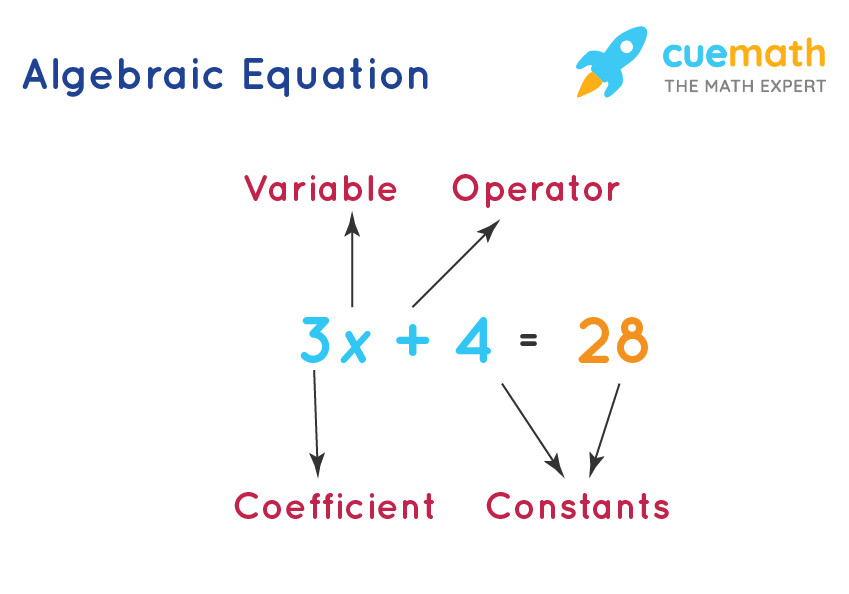
So if I type "3x + 4 = 28" it should print out the value? 8
let's start with the interface
which is obvious
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
4,3 are the terminal expressions
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
it does not take a context either right?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
The interface's interpret method should've taken a context in our case "3x + 4 = 28"
context will have something like this?
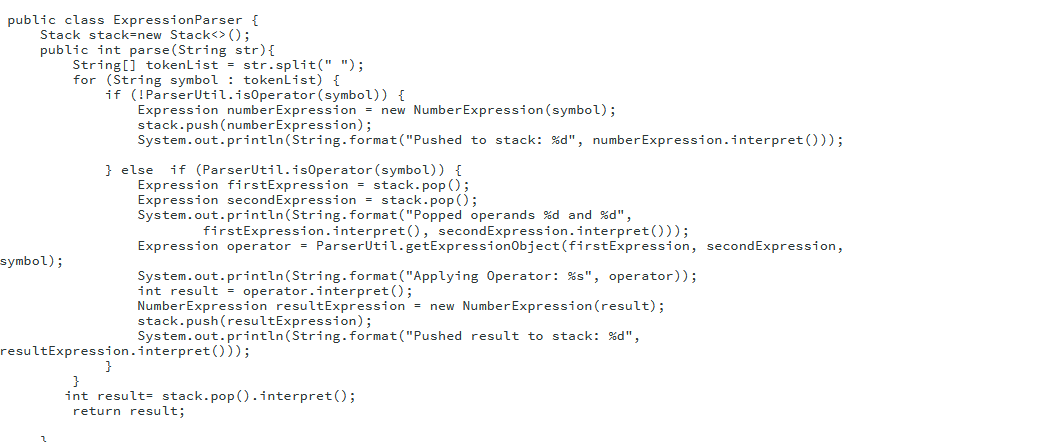
can you see it btw?
shall I send the code?
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
this is the full example code for it
but where is the context?
jt
Spring Framework Guru
Interpreter Pattern - Spring Framework Guru
Interpreter Pattern āGiven a language, define a representation for its grammar along with an interpreter that uses the representation to interpret sentences in the language.ā Design Patterns: Elements of Reusable Object-Oriented Software dInterpreter pattern is part of the Behavioral pattern family of the Gang of Four design patterns. Behavioral...
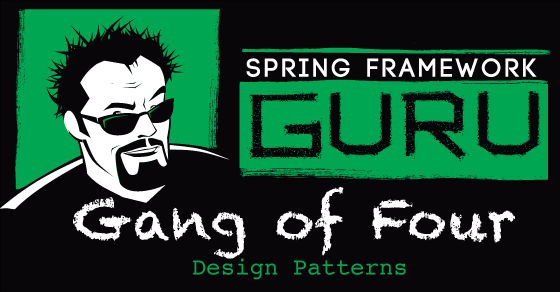
Unknown Userā¢15mo ago
Message Not Public
Sign In & Join Server To View
ok ok
I am going to take some break
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
/open
@borgrel
3x + 4 = 28 for this
the NonTerminal ones are
AdditionExpression?
SubstractionExpression
also I have to follow the BODMAS right?
The non terminal expressions we need
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
Figured it out finally
https://discord.com/channels/648956210850299986/653632542100160547/1203938798698762291
I guess I figured out the Interpreter Pattern for my application
That's not what terminal and non terminal mean
Non-terminal is a composite
You got it š
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.