why i cant access field in child class when using inheritance?
hey guys. can smb explain this error? i dont understand what im doing wrong:
thanks in advance
106 Replies
⌛
This post has been reserved for your question.
Hey @bambyzas! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
by creating a variable of type
Animal
, you say that you know it is an Animal
but don't assume further information
you could also do
ok. but ive seen such cases where you do stuff like Animal dog1=new Dog();. i.e. you create instance of a child class but for some reason assign it to the parent type. whats the purpose of this?
breed is only on dog, and you declared it as animal
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
it means you create a dog and forget that it is a dog
you just know about it being an animal after that
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
It's still a dog, you just reference it as an animal
Like, "my friend has a pet, can't remember if it's a dog or a cat, but it's definitely a pet"
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
ok, but then again. using the more abstract data type wouldnt let you use functinalities from more specific data type
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
i dont get it
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
this
Ok so there are two things: Variable declaration and variable initialization
Declaration means you create a variable, e.g.
This decides what methods are available to you
initialization is something else - it sets the variable to another value, e.g.
But this doesn't influence which parts are usable for you
new ArrayList()
creates an ArrayList
object. If you put it somewhetre that expects any List
and doesn't care which one it is, that code cannot use ArrayList
-specific stuffok. so it means i can use functionalities, which ArrayList has, right?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
i know what polymorphism and inheritance is
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
wdym?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
inheritance - you have a parent class and you can create child class. And child then can receive methods/fields from its parent class. if Animal has eat(), Dog will also have eat().
polymorphism - has two forms. method overloading and overriding. overriding is if in Animal has eat(){yum yum} and Dog overrides this method and it becomes eat(){nom nom}. overloading is having the same name of method but different signature (return type, params type, params numbers). i can have void addition(int a, int b) and void addition (double a, double b)
so ur speculation that idk what polymorphism and inheritance is wrong
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
huh?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
doesnt matter. Animal a=new Dog(); means that var
a
can have access to Dog class fields and methods. bc a
var point to frickin Dog objectUnknown User•15mo ago
Message Not Public
Sign In & Join Server To View
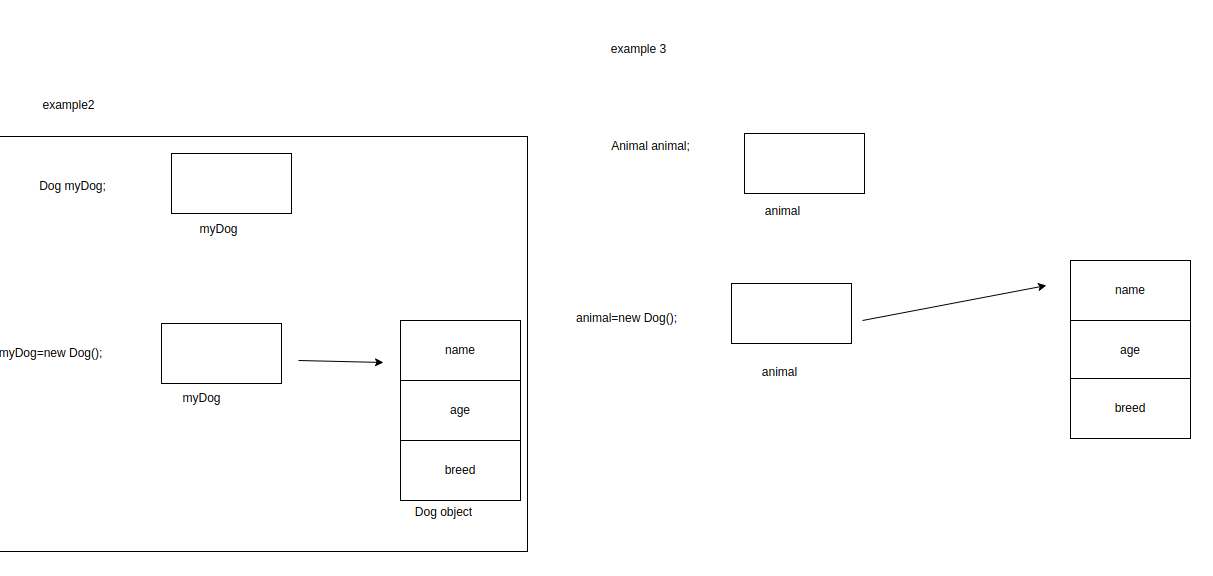
yes
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
ok. then ill ask it from another angle. these two are valid:
Animal animal=new Dog();
Dog dog=new Dog();
so are there any cases where id need to use animal var, that has less methods/fields?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
for example?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
but vice versta its compatible, right?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
All dogs are animals, but not all animals are dogs
crap
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
but i wasnt talking about generics yet
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
nope

Here we go with the full blown statement: Generics in Java are invariant (a pet peeve of mine). So what that means is that Java doesn't know if you're putting something in, or taking something out of a generic. If I had a List<Dog> I can add only dogs to it, but only get dogs out. If I have a list<animal> I can add dogs and cats, but only get animals out
So a List<Dog> is not a List<Animal>
So if I were to assign a List<Dog> to a List<Animal> all of a sudden I can put cats in there, which I shouldn't be able to do
In case you want to read more about that: https://stackoverflow.com/q/2745265/10871900
Stack Overflow
Is List a subclass of List? Why are Java generics not implicitly po...
I'm a bit confused about how Java generics handle inheritance / polymorphism.
Assume the following hierarchy -
Animal (Parent)
Dog - Cat (Children)
So suppose I have a method doSomething(List<
I miss in/out generics 😐
just do
extends
/super
when necessaryIt works (mostly) but it's a crutch
holy cow. thanks
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
@dan1st | Daniel but wait. i dont understand the issue here.
you put a cat in the list
and then you do
Dog dog = dogs.get(0);
so you take out the cat and assume it's a dogthe first two lines mean this?
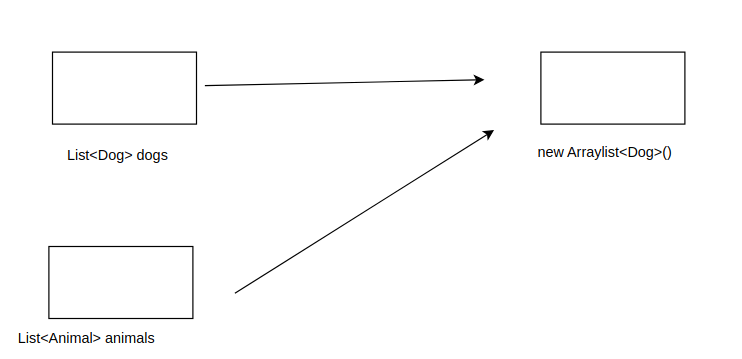
the first line means: create a list of dogs
The second one means: assume it's a list of animals (which is the issue)
but they both point to arraylist of dogs
it's the same list
but in one variable, you use it as a list of dogs which is fine
but in the other one, you use it as a list of animals which isn't ok because you can use it to insert a cat into the list of dogs
and if you have a list of dogs, you really don't want a cat in there
is the same as :
right?
Neitheŕ of them is allowed
wouldnt jvm do casting or smth like that under the hood? bc in my notes i have this fragment from the book:
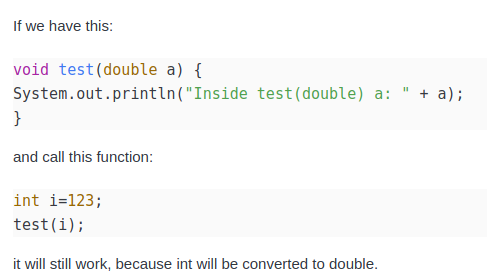
int/double is one thing but it doesn't work with generics
but i dont use generics. theres no <T> stuff
the List<Cat>/List<Dog> is about generics
Yes, people have explained this in your last 4 questions
again. i didnt use <T> syntax anywhere
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
But if i do List<String> thats also generics?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
but if i dont use objects. then its not generics, like List<int>?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
(which is using generics)
one last question fellas. in java theres List interface and ArrayList class. as we know, if u go up the inheritance hierarchy, u lose functionality/methods/fields. so
List<String>stringList=new ArrayList<>():
seems like a less capable solution. but for some reason its used more often than ArrayList<String>stringList=new ArrayList<>();
. why is that? why use less capable/powerful solution?Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
you misunderstand my question on purpose
He might but that stack overflow answer is exactly why
that explains exactly what you just asked
i know what does programming to an interface means but it doenst answer my question
and you keep telling me that it does
it's the reason you use
List
for the declaration instead of ArrayList
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
again, i said that you lose some functinality
yes, that's the point of programming against the interface
or a part of it
you only use the functionality specified in the interface
this is the same logic
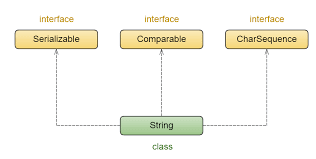
why use String, when you can use less powerful interface?
depends on which functionality you need
doesnt matter. yet still everyone uses String class all the time
if you just need a
CharSequence
, use thatUnknown User•15mo ago
Message Not Public
Sign In & Join Server To View
because in many cases, you actually need a
String
and different CharSequence
s do vastly different thingsi dont get it
when using ArrayList, u need for some reason to use less capable solution? doesnt make sense
oh wow. my code will be more flexible, but ill lose functionality.
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Do you need functionality of
ArrayList
that isn't in List
?
if you used CharSequence
instead of String
, you wouldn't be able to use substring
any moreits the same like asking
do u need functionality of String that isnt in CharSequence
. ofc. u always use String, instead of less capable CharSequence. yet u r telling me that I should use less capable List instead of ArrayListI need String specific functionality in most cases
ArrayList
doesn't provide more methods you'd need often
and then there's the thing that if you have a String
, you can be sure it's immutable etc
there isn't a good interface for String
and there's also no need for it since there are not alternative implementation of the same functionality (StringBuilder
has different functionality but if you want to write something working for both, use CharSequence
if possible)ArrayList has 31 method. List has 28 methods . u dont know ur stuff lol
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
I know
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
mhm. u cant even count lol
I haven't used any of the extra
ArrayList
methods for quite some timeUnknown User•15mo ago
Message Not Public
Sign In & Join Server To View
You need to use methods like ensureCapacity very rarely and most uses of it are useless/make something worse
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
No, that isn't necessary here
so if u use List, u get flexible code + basic/mostly needed functionalit. but if u use ArrayList u just get a couple of more extra methods. so that is the reasoning one would choose List over ArrayList?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
When was the last time you actually needed
ArrayList
functionality that isn't in List
?huih?
I think it's never because if you needed it at some point, you wouldn't say that
Post Closed
This post has been closed by <@611623200756989972>.