Explanation of the Value parameter in Class constructor
Hello! I am following tutorials on Game Dev with C# and MonoGame to improve and practice my basic OOP programming knowledge with C#.
I am trying to understand which is the meaning of "value" in the following, which I suppose to be a constructor:
public ContentManager Content
{
get
{
return _content;
}
set
{
if (value == null)
{
throw new ArgumentNullException();
}
_content = value;
}
}
The class constructor pasted above is inside the class Game : IDisposable that comes from the Microsoft.Xna.Framework.
Specifically, I don't understand where the "value" comes from and how it is handled, as it seems to me to be a parameter passed inside such a class constructor:
At the same time, the constructor seems parameterless to me, as there is no () after the name of the constructor... I suppose I am missing some OOP key concepts in this regard; please help me to understand 🙂
More in general, this "Content {get;set;}" is called to load the content inside the game, in the LoadContent method of the Game.cs:
protected override void LoadContent()
{
target = Content.Load<Texture2D>("target");
target = Content.Load<Texture2D>("target");
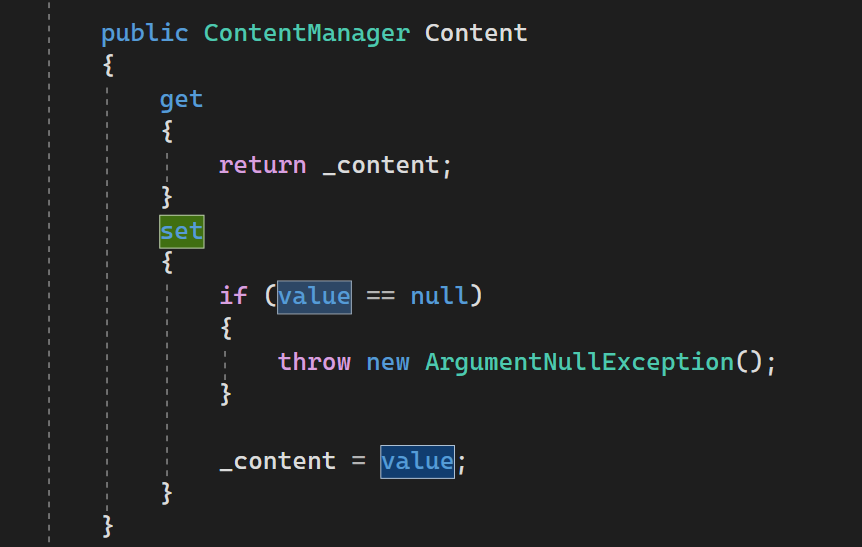
12 Replies
This is not a constructor, it is a property
A property is essentially two methods, a method which gets a value, and a method to set a value. In this case you have a field called
_content
, which this property gets and sets the value of.
$getsetdevolvecan be shortened to
can be shortened to
can be shortened to
can be shortened to
What this property is doing is in the setter it's confirming that the value passed into the property is not null, before actually setting the
_content
field to that value.Imagine
value
is a "parameter" of set
"method".
Your original code
You can imagine that get
is a method without parameter and set
is a method with a parameter value
of type ContentManager
as follows.
As it is just an imagination, the following does not compile!
Thank you so much for the explanations! These are really useful to me! 🙂
I have another doubt: this LoadContent method is where the Content property mentioned before is used.
By hovering with the mouse to see the root, I see these two class names (ContentManager and Game) with a space between them.
Game is the class where the Content property is defined, so it appears obvious to me that this is shown here. But what is the role of the ContentManager instead? Does the space imply a relationship between the two classes here?
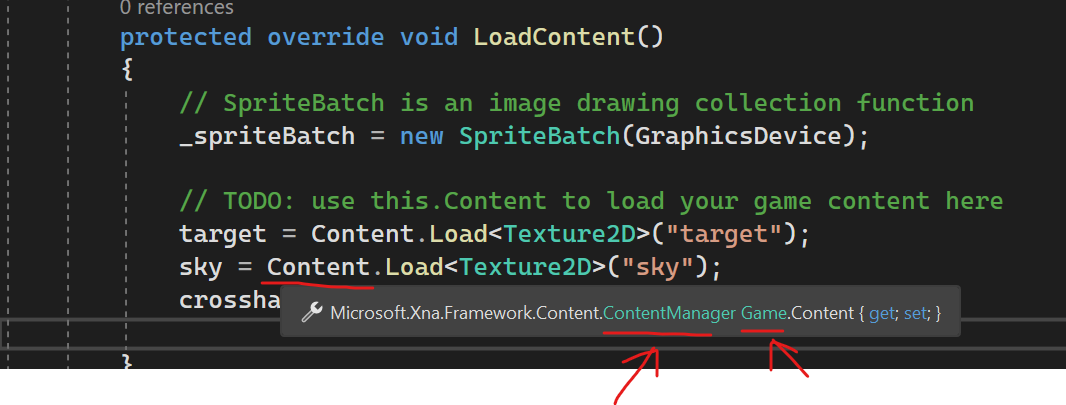
Edit:
I think this answer does not answer your question right above.
Just navigate to my answer right after this one.
I keep this for your future reference about
this
.
- this
word represents the instance of Game
being constructed.
- this
word can only be used inside the class (in this example, Game
class)
Still confused?
A Game
instance hahaha
created outside Game
class
A Game
instance this
created automatically inside Game
class
- this
is provided automatically and cannot be manually created!
- so any attempt to do this = new Game();
is illegal, it does not compile!
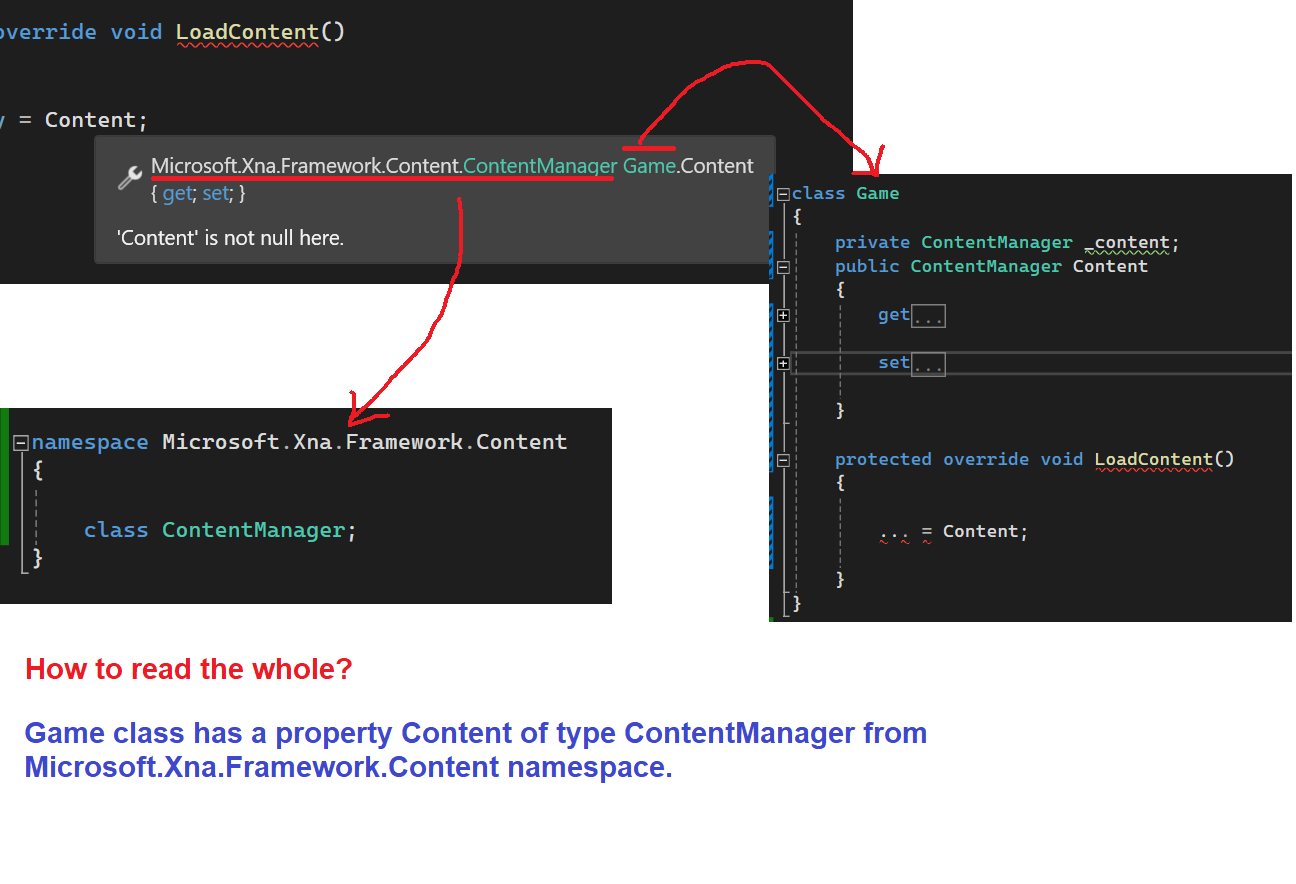
Great!!! Thank you so much @ABOUT ME explains my gameplay !!
Because I am not using Unity, I cannot answer with 100% accuracy for the meaning of
It can be any thing such as:
or
or
or others that I am lazy to write.

this is a property, not a constructor
oh, chat jump