Is Inside a polygon algorithm..
Does anyone know how to make an algorithm on how to check if a Vector3 position is inside a set of Vector3 corners?
I tried raycasting, but its not giving me the correct results.
So basicallyt the blue dots are the corners, and the red is how is where its "active" ( Where I get detected ).
The black lines is how i want it.
As said I tried raycasting and the results of that is in the image below.
I know that winding numbers would be accurate 100%, but I want to be able to have the corners in a randomized order, and not a clockwise or counterclockwise way.
Does anyone know how to do this?
Red = Zone I get detected.
Blue = The corners
Black = How I want it to work..
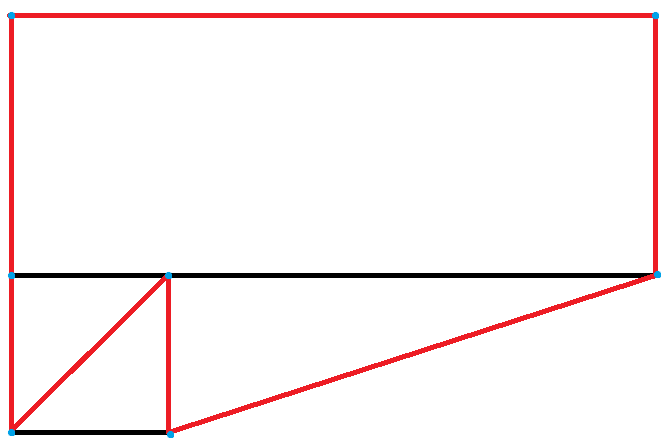
2 Replies
I believe it has something to do with how I am sorting the vertices based on the centroid.
u could simply choose a random direction from the point and calculate with that ray how many surface triangles it intersects,
if the number is odd the point is inside, if the number is even its outside