✅ How to serialize IEnumerable<T> to json?
I have following:
and I want to get following: " { "Key": "GetValueBytes()", ... } "
how can I do it?
and I want to get following: " { "Key": "GetValueBytes()", ... } "
how can I do it?
16 Replies
I can do this:
but it looks bad
JsonConverter serializes dictionaries as key-value pairs just fine
so perhaps make the collection type into a dictionary?
cleaner way would be to write a custom converter otherwise
iirc
IEnumerable<byte>
will be serialized into a json array of numbers,
byte[]
will result in a base64 encoded string,
the proper answer is a custom converterno I meant something on the line of exposing
Headers
as IReadOnlyDictionary<string, byte[]>
yeah, that would result in
"header1": "base64-encoded-byte-array"
, while ⤴️ would be "header1": "1,254,4,6,127"
ahhh so they do want it as a comma-separated list of values
why though
you'd need a custom converter then
why though
tbh i like neither variant 😂
i would prefer a simple hex string without delimiters for such stuff, but i guess base64 is at least not that a** long
hex string would get much longer than base64!
and I don't think it really adds any benefit apart from being easy to parse, so... why?
i was working on a project for a long time where i had to write some test packets (had to do with reverse engineering a network protocol),
guess i got used to hex in json because of that
was a lot of fiddling around, adding similar packets (if it were stored binary i would have taken a hex editor for that), where u simply edited the description, some bytes and that was it
later the guy i did work together on that wrote a whole GUI tool and stored the whole thing as binary blob but also with some more helpful visualization on the data
unfortunately I can't change IEnumerable to IReadOnlyDictionary
hm maybe I can do smth like..
Headers.ToDictionary(header => header.Key, header => header.GetValueBytes())
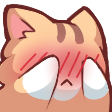
I would suggest using a custom converter
are you using S.T.Json?
Unknown User•16mo ago
Message Not Public
Sign In & Join Server To View
How to write custom converters for JSON serialization - .NET
Learn how to create custom converters for the JSON serialization classes that are provided in the System.Text.Json namespace.
ok, thanks!